- * @see http://www.php-fig.org/psr/psr-0/
- * @see http://www.php-fig.org/psr/psr-4/
- */
-class ClassLoader
-{
- // PSR-4
- private $prefixLengthsPsr4 = array();
- private $prefixDirsPsr4 = array();
- private $fallbackDirsPsr4 = array();
-
- // PSR-0
- private $prefixesPsr0 = array();
- private $fallbackDirsPsr0 = array();
-
- private $useIncludePath = false;
- private $classMap = array();
- private $classMapAuthoritative = false;
- private $missingClasses = array();
- private $apcuPrefix;
-
- public function getPrefixes()
- {
- if (!empty($this->prefixesPsr0)) {
- return call_user_func_array('array_merge', $this->prefixesPsr0);
- }
-
- return array();
- }
-
- public function getPrefixesPsr4()
- {
- return $this->prefixDirsPsr4;
- }
-
- public function getFallbackDirs()
- {
- return $this->fallbackDirsPsr0;
- }
-
- public function getFallbackDirsPsr4()
- {
- return $this->fallbackDirsPsr4;
- }
-
- public function getClassMap()
- {
- return $this->classMap;
- }
-
- /**
- * @param array $classMap Class to filename map
- */
- public function addClassMap(array $classMap)
- {
- if ($this->classMap) {
- $this->classMap = array_merge($this->classMap, $classMap);
- } else {
- $this->classMap = $classMap;
- }
- }
-
- /**
- * Registers a set of PSR-0 directories for a given prefix, either
- * appending or prepending to the ones previously set for this prefix.
- *
- * @param string $prefix The prefix
- * @param array|string $paths The PSR-0 root directories
- * @param bool $prepend Whether to prepend the directories
- */
- public function add($prefix, $paths, $prepend = false)
- {
- if (!$prefix) {
- if ($prepend) {
- $this->fallbackDirsPsr0 = array_merge(
- (array) $paths,
- $this->fallbackDirsPsr0
- );
- } else {
- $this->fallbackDirsPsr0 = array_merge(
- $this->fallbackDirsPsr0,
- (array) $paths
- );
- }
-
- return;
- }
-
- $first = $prefix[0];
- if (!isset($this->prefixesPsr0[$first][$prefix])) {
- $this->prefixesPsr0[$first][$prefix] = (array) $paths;
-
- return;
- }
- if ($prepend) {
- $this->prefixesPsr0[$first][$prefix] = array_merge(
- (array) $paths,
- $this->prefixesPsr0[$first][$prefix]
- );
- } else {
- $this->prefixesPsr0[$first][$prefix] = array_merge(
- $this->prefixesPsr0[$first][$prefix],
- (array) $paths
- );
- }
- }
-
- /**
- * Registers a set of PSR-4 directories for a given namespace, either
- * appending or prepending to the ones previously set for this namespace.
- *
- * @param string $prefix The prefix/namespace, with trailing '\\'
- * @param array|string $paths The PSR-4 base directories
- * @param bool $prepend Whether to prepend the directories
- *
- * @throws \InvalidArgumentException
- */
- public function addPsr4($prefix, $paths, $prepend = false)
- {
- if (!$prefix) {
- // Register directories for the root namespace.
- if ($prepend) {
- $this->fallbackDirsPsr4 = array_merge(
- (array) $paths,
- $this->fallbackDirsPsr4
- );
- } else {
- $this->fallbackDirsPsr4 = array_merge(
- $this->fallbackDirsPsr4,
- (array) $paths
- );
- }
- } elseif (!isset($this->prefixDirsPsr4[$prefix])) {
- // Register directories for a new namespace.
- $length = strlen($prefix);
- if ('\\' !== $prefix[$length - 1]) {
- throw new \InvalidArgumentException("A non-empty PSR-4 prefix must end with a namespace separator.");
- }
- $this->prefixLengthsPsr4[$prefix[0]][$prefix] = $length;
- $this->prefixDirsPsr4[$prefix] = (array) $paths;
- } elseif ($prepend) {
- // Prepend directories for an already registered namespace.
- $this->prefixDirsPsr4[$prefix] = array_merge(
- (array) $paths,
- $this->prefixDirsPsr4[$prefix]
- );
- } else {
- // Append directories for an already registered namespace.
- $this->prefixDirsPsr4[$prefix] = array_merge(
- $this->prefixDirsPsr4[$prefix],
- (array) $paths
- );
- }
- }
-
- /**
- * Registers a set of PSR-0 directories for a given prefix,
- * replacing any others previously set for this prefix.
- *
- * @param string $prefix The prefix
- * @param array|string $paths The PSR-0 base directories
- */
- public function set($prefix, $paths)
- {
- if (!$prefix) {
- $this->fallbackDirsPsr0 = (array) $paths;
- } else {
- $this->prefixesPsr0[$prefix[0]][$prefix] = (array) $paths;
- }
- }
-
- /**
- * Registers a set of PSR-4 directories for a given namespace,
- * replacing any others previously set for this namespace.
- *
- * @param string $prefix The prefix/namespace, with trailing '\\'
- * @param array|string $paths The PSR-4 base directories
- *
- * @throws \InvalidArgumentException
- */
- public function setPsr4($prefix, $paths)
- {
- if (!$prefix) {
- $this->fallbackDirsPsr4 = (array) $paths;
- } else {
- $length = strlen($prefix);
- if ('\\' !== $prefix[$length - 1]) {
- throw new \InvalidArgumentException("A non-empty PSR-4 prefix must end with a namespace separator.");
- }
- $this->prefixLengthsPsr4[$prefix[0]][$prefix] = $length;
- $this->prefixDirsPsr4[$prefix] = (array) $paths;
- }
- }
-
- /**
- * Turns on searching the include path for class files.
- *
- * @param bool $useIncludePath
- */
- public function setUseIncludePath($useIncludePath)
- {
- $this->useIncludePath = $useIncludePath;
- }
-
- /**
- * Can be used to check if the autoloader uses the include path to check
- * for classes.
- *
- * @return bool
- */
- public function getUseIncludePath()
- {
- return $this->useIncludePath;
- }
-
- /**
- * Turns off searching the prefix and fallback directories for classes
- * that have not been registered with the class map.
- *
- * @param bool $classMapAuthoritative
- */
- public function setClassMapAuthoritative($classMapAuthoritative)
- {
- $this->classMapAuthoritative = $classMapAuthoritative;
- }
-
- /**
- * Should class lookup fail if not found in the current class map?
- *
- * @return bool
- */
- public function isClassMapAuthoritative()
- {
- return $this->classMapAuthoritative;
- }
-
- /**
- * APCu prefix to use to cache found/not-found classes, if the extension is enabled.
- *
- * @param string|null $apcuPrefix
- */
- public function setApcuPrefix($apcuPrefix)
- {
- $this->apcuPrefix = function_exists('apcu_fetch') && ini_get('apc.enabled') ? $apcuPrefix : null;
- }
-
- /**
- * The APCu prefix in use, or null if APCu caching is not enabled.
- *
- * @return string|null
- */
- public function getApcuPrefix()
- {
- return $this->apcuPrefix;
- }
-
- /**
- * Registers this instance as an autoloader.
- *
- * @param bool $prepend Whether to prepend the autoloader or not
- */
- public function register($prepend = false)
- {
- spl_autoload_register(array($this, 'loadClass'), true, $prepend);
- }
-
- /**
- * Unregisters this instance as an autoloader.
- */
- public function unregister()
- {
- spl_autoload_unregister(array($this, 'loadClass'));
- }
-
- /**
- * Loads the given class or interface.
- *
- * @param string $class The name of the class
- * @return bool|null True if loaded, null otherwise
- */
- public function loadClass($class)
- {
- if ($file = $this->findFile($class)) {
- includeFile($file);
-
- return true;
- }
- }
-
- /**
- * Finds the path to the file where the class is defined.
- *
- * @param string $class The name of the class
- *
- * @return string|false The path if found, false otherwise
- */
- public function findFile($class)
- {
- // class map lookup
- if (isset($this->classMap[$class])) {
- return $this->classMap[$class];
- }
- if ($this->classMapAuthoritative || isset($this->missingClasses[$class])) {
- return false;
- }
- if (null !== $this->apcuPrefix) {
- $file = apcu_fetch($this->apcuPrefix.$class, $hit);
- if ($hit) {
- return $file;
- }
- }
-
- $file = $this->findFileWithExtension($class, '.php');
-
- // Search for Hack files if we are running on HHVM
- if (false === $file && defined('HHVM_VERSION')) {
- $file = $this->findFileWithExtension($class, '.hh');
- }
-
- if (null !== $this->apcuPrefix) {
- apcu_add($this->apcuPrefix.$class, $file);
- }
-
- if (false === $file) {
- // Remember that this class does not exist.
- $this->missingClasses[$class] = true;
- }
-
- return $file;
- }
-
- private function findFileWithExtension($class, $ext)
- {
- // PSR-4 lookup
- $logicalPathPsr4 = strtr($class, '\\', DIRECTORY_SEPARATOR) . $ext;
-
- $first = $class[0];
- if (isset($this->prefixLengthsPsr4[$first])) {
- $subPath = $class;
- while (false !== $lastPos = strrpos($subPath, '\\')) {
- $subPath = substr($subPath, 0, $lastPos);
- $search = $subPath.'\\';
- if (isset($this->prefixDirsPsr4[$search])) {
- $pathEnd = DIRECTORY_SEPARATOR . substr($logicalPathPsr4, $lastPos + 1);
- foreach ($this->prefixDirsPsr4[$search] as $dir) {
- if (file_exists($file = $dir . $pathEnd)) {
- return $file;
- }
- }
- }
- }
- }
-
- // PSR-4 fallback dirs
- foreach ($this->fallbackDirsPsr4 as $dir) {
- if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr4)) {
- return $file;
- }
- }
-
- // PSR-0 lookup
- if (false !== $pos = strrpos($class, '\\')) {
- // namespaced class name
- $logicalPathPsr0 = substr($logicalPathPsr4, 0, $pos + 1)
- . strtr(substr($logicalPathPsr4, $pos + 1), '_', DIRECTORY_SEPARATOR);
- } else {
- // PEAR-like class name
- $logicalPathPsr0 = strtr($class, '_', DIRECTORY_SEPARATOR) . $ext;
- }
-
- if (isset($this->prefixesPsr0[$first])) {
- foreach ($this->prefixesPsr0[$first] as $prefix => $dirs) {
- if (0 === strpos($class, $prefix)) {
- foreach ($dirs as $dir) {
- if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr0)) {
- return $file;
- }
- }
- }
- }
- }
-
- // PSR-0 fallback dirs
- foreach ($this->fallbackDirsPsr0 as $dir) {
- if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr0)) {
- return $file;
- }
- }
-
- // PSR-0 include paths.
- if ($this->useIncludePath && $file = stream_resolve_include_path($logicalPathPsr0)) {
- return $file;
- }
-
- return false;
- }
-}
-
-/**
- * Scope isolated include.
- *
- * Prevents access to $this/self from included files.
- */
-function includeFile($file)
-{
- include $file;
-}
diff --git a/plugins/breadcrumbs/vendor/composer/LICENSE b/plugins/breadcrumbs/vendor/composer/LICENSE
deleted file mode 100644
index f27399a..0000000
--- a/plugins/breadcrumbs/vendor/composer/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-
-Copyright (c) Nils Adermann, Jordi Boggiano
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is furnished
-to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
-THE SOFTWARE.
-
diff --git a/plugins/breadcrumbs/vendor/composer/autoload_classmap.php b/plugins/breadcrumbs/vendor/composer/autoload_classmap.php
deleted file mode 100644
index 1e48ed9..0000000
--- a/plugins/breadcrumbs/vendor/composer/autoload_classmap.php
+++ /dev/null
@@ -1,10 +0,0 @@
- $baseDir . '/breadcrumbs.php',
-);
diff --git a/plugins/breadcrumbs/vendor/composer/autoload_namespaces.php b/plugins/breadcrumbs/vendor/composer/autoload_namespaces.php
deleted file mode 100644
index b7fc012..0000000
--- a/plugins/breadcrumbs/vendor/composer/autoload_namespaces.php
+++ /dev/null
@@ -1,9 +0,0 @@
- array($baseDir . '/classes/plugin'),
-);
diff --git a/plugins/breadcrumbs/vendor/composer/autoload_real.php b/plugins/breadcrumbs/vendor/composer/autoload_real.php
deleted file mode 100644
index c1891fd..0000000
--- a/plugins/breadcrumbs/vendor/composer/autoload_real.php
+++ /dev/null
@@ -1,52 +0,0 @@
-= 50600 && !defined('HHVM_VERSION') && (!function_exists('zend_loader_file_encoded') || !zend_loader_file_encoded());
- if ($useStaticLoader) {
- require_once __DIR__ . '/autoload_static.php';
-
- call_user_func(\Composer\Autoload\ComposerStaticInit3ccdcd6f1ac1044c32372e7c5a66846a::getInitializer($loader));
- } else {
- $map = require __DIR__ . '/autoload_namespaces.php';
- foreach ($map as $namespace => $path) {
- $loader->set($namespace, $path);
- }
-
- $map = require __DIR__ . '/autoload_psr4.php';
- foreach ($map as $namespace => $path) {
- $loader->setPsr4($namespace, $path);
- }
-
- $classMap = require __DIR__ . '/autoload_classmap.php';
- if ($classMap) {
- $loader->addClassMap($classMap);
- }
- }
-
- $loader->register(true);
-
- return $loader;
- }
-}
diff --git a/plugins/breadcrumbs/vendor/composer/autoload_static.php b/plugins/breadcrumbs/vendor/composer/autoload_static.php
deleted file mode 100644
index 6dbff43..0000000
--- a/plugins/breadcrumbs/vendor/composer/autoload_static.php
+++ /dev/null
@@ -1,36 +0,0 @@
-
- array (
- 'Grav\\Plugin\\Breadcrumbs\\' => 24,
- ),
- );
-
- public static $prefixDirsPsr4 = array (
- 'Grav\\Plugin\\Breadcrumbs\\' =>
- array (
- 0 => __DIR__ . '/../..' . '/classes/plugin',
- ),
- );
-
- public static $classMap = array (
- 'Grav\\Plugin\\BreadcrumbsPlugin' => __DIR__ . '/../..' . '/breadcrumbs.php',
- );
-
- public static function getInitializer(ClassLoader $loader)
- {
- return \Closure::bind(function () use ($loader) {
- $loader->prefixLengthsPsr4 = ComposerStaticInit3ccdcd6f1ac1044c32372e7c5a66846a::$prefixLengthsPsr4;
- $loader->prefixDirsPsr4 = ComposerStaticInit3ccdcd6f1ac1044c32372e7c5a66846a::$prefixDirsPsr4;
- $loader->classMap = ComposerStaticInit3ccdcd6f1ac1044c32372e7c5a66846a::$classMap;
-
- }, null, ClassLoader::class);
- }
-}
diff --git a/plugins/breadcrumbs/vendor/composer/installed.json b/plugins/breadcrumbs/vendor/composer/installed.json
deleted file mode 100644
index fe51488..0000000
--- a/plugins/breadcrumbs/vendor/composer/installed.json
+++ /dev/null
@@ -1 +0,0 @@
-[]
diff --git a/themes/learn2-git-sync/.dependencies b/themes/learn2-git-sync/.dependencies
deleted file mode 100644
index 71ea86e..0000000
--- a/themes/learn2-git-sync/.dependencies
+++ /dev/null
@@ -1,5 +0,0 @@
-git:
- learn2:
- url: https://github.com/getgrav/grav-theme-learn2
- path: user/themes/learn2
- branch: master
diff --git a/themes/learn2-git-sync/.gitignore b/themes/learn2-git-sync/.gitignore
deleted file mode 100644
index fd18632..0000000
--- a/themes/learn2-git-sync/.gitignore
+++ /dev/null
@@ -1,6 +0,0 @@
-# OS Generated
-.DS_Store*
-thumbs.db
-Icon?
-Thumbs.db
-*.swp
diff --git a/themes/learn2-git-sync/CHANGELOG.md b/themes/learn2-git-sync/CHANGELOG.md
deleted file mode 100644
index 69f4517..0000000
--- a/themes/learn2-git-sync/CHANGELOG.md
+++ /dev/null
@@ -1,321 +0,0 @@
-# v1.6.6
-## 03/08/2022
-
-1. [](#bugfix)
- * Fixed getgrav.org link at the bottom of the sidebar (thanks @timinator2020)
-
-# v1.6.5
-## 03/21/2021
-
-1. [](#improved)
- * Updated demo pages in theme '_demo' folder
-
-# v1.6.4
-## 02/28/2021
-
-1. [](#improved)
- * Reduced plugin dependencies, thanks to the new Grav Skeleton build process
-
-# v1.6.3
-## 02/15/2021
-
-1. [](#bugfix)
- * Removed tab characters from comment indent (thanks @holehan)
-
-# v1.6.2
-## 01/26/2021
-
-1. [](#improved)
- * Updated theme blueprints for Grav 1.7
-
-# v1.6.1
-## 01/15/2021
-
-1. [](#new)
- * GoogleSlides shortcode updated to handle double `_` characters in URL (thanks @GeorgesPapas)
-
-# v1.6.0
-## 01/05/2021
-
-1. [](#new)
- * Initial NextGen Editor support for GoogleSlides, H5P and PDF shortcodes
-
-# v1.5.9
-## 05/19/2020
-
-1. [](#improved)
- * Updated inherited theme file paths for multisite compatibility (thanks to @tituspijean for the PR and @mahagr for the debugging!)
-
-# v1.5.8
-## 12/05/2019
-
-1. [](#bugfix)
- * Do not display the SimpleSearch field when the SimpleSearch plugin is disabled
-
-# v1.5.7
-## 11/25/2019
-
-1. [](#improved)
- * 'Top Level Version' theme option now only displays visible pages (thanks @awrog for reporting this issue)
-
-# v1.5.6
-## 08/16/2019
-
-1. [](#improved)
- * Twig 2.0 compatibility
-
-# v1.5.5
-## 06/03/2019
-
-1. [](#improved)
- * Updated demo pages in theme '_demo' folder
-
-# v1.5.4
-## 05/05/2019
-
-1. [](#improved)
- * Updated demo pages in theme '_demo' folder
-
-# v1.5.3
-## 05/04/2019
-
-1. [](#improved)
- * Updated demo pages in theme '_demo' folder
-
-# v1.5.2
-## 05/01/2019
-
-1. [](#bugfix)
- * Added missing images folder with clippy.svg and default favicons
-
-# v1.5.1
-## 04/12/2019
-
-1. [](#new)
- * Added insert Presentation Shortcode button into Editor toolbar
-
-# v1.5.0
-## 04/11/2019
-
-1. [](#new)
- * Set Grav dependency to 1.6+
- * Twig 2.0 compatibility
- * Added scripts.html.twig file to hold global JavaScript code
- * Added support for document versioning using Grav's multi-language feature (thanks @rhuk)
- * Added 'version' alias to 'language' shortcode, for example `[version=20]...[/version]` (thanks @rhuk)
- * Added taxonomy 'tag' to default TNTSearch index
- * Added Presentation Plugin as dependency
- * Added insert Presentation Shortcode button into Editor toolbar
- * Added example pages in theme '_demo' folder to include use of the Presentation Plugin
- * Implement assets rendering using **Deferred Block** Twig extension
-1. [](#improved)
- * Improved appearance of versions dropdown menu with custom themes
-
-# v1.3.3
-## 04/02/2019
-
-1. [](#new)
- * Added scripts.html.twig file to hold global JavaScript code
-
-# v1.3.2
-## 03/22/2019
-
-1. [](#new)
- * Set Dependency of Grav 1.5.10+ which has support for new **Deferred Block** Twig extension
- * Implement assets rendering using **Deferred Block** Twig extension
-
-# v1.3.1
-## 03/10/2019
-
-1. [](#bugfix)
- * Restored needed 'RocketTheme\Toolbox\Event\Event;' instance
-
-# v1.3.0
-## 03/10/2019
-
-1. [](#new)
- * Added taxonomy 'tag' to default TNTSearch index
-
-# v1.2.3
-## 11/23/2018
-
-1. [](#new)
- * Added custom styles 'Spitsbergen' and 'Longyearbyen' (thanks @olevik)
-
-# v1.2.2
-## 10/17/2018
-
-1. [](#improved)
- * Updated ReadMe, theme description and screenshots
-
-# v1.2.1
-## 10/16/2018
-
-1. [](#bugfix)
- * Restored CSS styling for display of site title
-
-# v1.2.0
-## 10/16/2018
-
-1. [](#new)
- * Added custom styles (thanks @olevik)
- * Added option to show or hide site title
-1. [](#bugfix)
- * Fixed issue with default taxonomy categories
-
-# v1.1.0
-## 08/25/2018
-
-1. [](#improved)
- * Initial multi-language support for Advanced Full-text Search
-
-# v1.0.9
-## 08/23/2018
-
-1. [](#bugfix)
- * Restored missing Error and Problems Plugin dependencies
-
-# v1.0.8
-## 08/22/2018
-
-1. [](#improved)
- * Updated screenshots
-
-# v1.0.7
-## 08/21/2018
-
-1. [](#improved)
- * Added SimpleSearch plugin to theme dependencies
-
-# v1.0.6
-## 08/21/2018
-
-1. [](#bugfix)
- * Updated URL for Advanced Full-text Search link
-
-# v1.0.5
-## 08/21/2018
-
-1. [](#improved)
- * Updated TNTSearch box label
-1. [](#new)
- * Added TNTSearch (full-text search engine) plugin to theme dependencies
-
-# v1.0.4
-## 08/21/2018
-
-1. [](#improved)
- * Updated theme dependencies
-
-# v1.0.3
-## 08/20/2018
-
-1. [](#bugfix)
- * Fixed URL for Advanced Full-text Search link
-
-# v1.0.2
-## 08/20/2018
-
-1. [](#new)
- * Added experimental support for TNTSearch plugin
-
-# v1.0.1
-## 08/20/2018
-
-1. [](#new)
- * Added configuration for default taxonomy category in theme setting
-1. [](#improved)
- * Updated theme blueprints
-
-# v1.0.0
-## 03/15/2018
-
-1. [](#improved)
- * Changed theme author information to Hibbitts Design
-
-# v0.9.84
-## 03/01/2018
-
-1. [](#improved)
- * Better aligned Git Sync Link Twig code with other Git Sync themes
-
-# v0.9.83
-## 02/13/2018
-
-1. [](#bugfix)
- * Fixed trailing slash issue with "Edit this page" link (thanks Amiram Korach)
-
-# v0.9.82
-## 01/23/2018
-
-1. [](#new)
- * Added default content for pages created using Admin Panel button bar
-
-# v0.9.81
-## 01/14/2018
-
-1. [](#improved)
- * Changed default size of site title to h5 (from h4) to reduce vertical space
-
-# v0.9.8
-## 10/23/2017
-
-1. [](#improved)
- * Added Highlight plugin as a dependency
-1. [](#bugfix)
- * Removed unneeded theme default for custom icon in Git Sync link
-
-# v0.9.7
-## 10/22/2017
-
-1. [](#new)
- * Further streamlined Git Sync setup step by automating 'Edit in Git' tree URL calculation
-
-# v0.9.6
-## 07/12/2017
-
-1. [](#bugfix)
- * Removed Author field within Page Options (Author taxonomy tag added to Learn2 with Git Sync Skeleton)
-
-# v0.9.5
-## 07/11/2017
-
-1. [](#new)
- * Added support for RSS feed
- * Added Author field within Page Options
-1. [](#improved)
- * Improved accessibility of sidebar and body link appearance
-1. [](#bugfix)
- * Removed problematic CSS class for Git Sync note area
-
-# v0.9.4
-## 05/30/2017
-
-1. [](#improved)
- * Improved wording for 'Git Repository Tree URL' theme config option
-
-# v0.9.3
-## 04/18/2017
-
-1. [](#bugfix)
- * Fixed theme setup link to work with an inherited theme
-
-# v0.9.2
-## 04/10/2017
-
-1. [](#bugfix)
- * Fixed Git Sync setup link
-
-# v0.9.1
-## 04/10/2017
-
-1. [](#improved)
- * Updated dependencies
-1. [](#bugfix)
- * Fixed twig template URL
-
-# v0.9.0
-## 04/03/2017
-
-1. [](#new)
- * ChangeLog started...
diff --git a/themes/learn2-git-sync/LICENSE b/themes/learn2-git-sync/LICENSE
deleted file mode 100644
index 484793a..0000000
--- a/themes/learn2-git-sync/LICENSE
+++ /dev/null
@@ -1,21 +0,0 @@
-The MIT License (MIT)
-
-Copyright (c) 2014 Grav
-
-Permission is hereby granted, free of charge, to any person obtaining a copy
-of this software and associated documentation files (the "Software"), to deal
-in the Software without restriction, including without limitation the rights
-to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
-copies of the Software, and to permit persons to whom the Software is
-furnished to do so, subject to the following conditions:
-
-The above copyright notice and this permission notice shall be included in all
-copies or substantial portions of the Software.
-
-THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
-IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
-FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
-AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
-LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
-OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
-SOFTWARE.
diff --git a/themes/learn2-git-sync/README.md b/themes/learn2-git-sync/README.md
deleted file mode 100644
index 9fb2a76..0000000
--- a/themes/learn2-git-sync/README.md
+++ /dev/null
@@ -1,90 +0,0 @@
-# Learn2 with Git Sync
-
-Learn2 with Git Sync is a customized version of the default [Grav Learn](http://learn.getgrav.org) theme. Includes selectable visual styles.
-
-Want to quickly try out this theme? The [Learn2 with Git Sync Skeleton](https://getgrav.org/downloads/skeletons) is a ready-to-run site with the Learn2 with Git Sync Theme, which also includes the Admin Panel and example content already installed.
-
-
-
-# Installation
-
-Installing the Learn2 with Git Sync theme can be done in one of two ways. Our GPM (Grav Package Manager) installation method enables you to quickly and easily install the theme with a simple terminal command, while the manual method enables you to do so via a zip file.
-
-The theme is designed to be used to provide a documentation site. You can see this in action at [learn.hibbittsdesign.org](http://learn.hibbittsdesign.org)
-
-## GPM Installation (Preferred)
-
-The simplest way to install this theme is via the [Grav Package Manager (GPM)](http://learn.getgrav.org/advanced/grav-gpm) through your system's Terminal (also called the command line). From the root of your Grav install type:
-
- bin/gpm install learn2-git-sync
-
-This will install the Learn2 with Git Sync theme into your `/user/themes` directory within Grav. Its files can be found under `/your/site/grav/user/themes/learn2-git-sync`.
-
-## Manual Installation
-
-To install this theme, just download the zip version of this repository and unzip it under `/your/site/grav/user/themes`. Then, rename the folder to `learn2-git-sync`.
-
-You should now have all the theme files under
-
- /your/site/grav/user/themes/learn2-git-sync
-
->> NOTE: This theme is a modular component for Grav which requires the [Grav](http://github.com/getgrav/grav), [Error](https://github.com/getgrav/grav-theme-error) and [Problems](https://github.com/getgrav/grav-plugin-problems) plugins.
-
-# Updating
-
-As development for the Learn2 with Git Sync theme continues, new versions may become available that add additional features and functionality, improve compatibility with newer Grav releases, and generally provide a better user experience. Updating Learn2 with Git Sync is easy, and can be done through Grav's GPM system, as well as manually.
-
-## GPM Update (Preferred)
-
-The simplest way to update this theme is via the [Grav Package Manager (GPM)](http://learn.getgrav.org/advanced/grav-gpm). You can do this with this by navigating to the root directory of your Grav install using your system's Terminal (also called command line) and typing the following:
-
- bin/gpm update learn2-git-sync
-
-This command will check your Grav install to see if your Learn2 with Git Sync theme is due for an update. If a newer release is found, you will be asked whether or not you wish to update. To continue, type `y` and hit enter. The theme will automatically update and clear Grav's cache.
-
-## Manual Update
-
-Manually updating Learn2 with Git Sync is pretty simple. Here is what you will need to do to get this done:
-
-* Delete the `your/site/user/themes/learn2-git-sync` directory.
-* Download the new version of the Learn2 with Git Sync theme from either [GitHub](https://github.com/hibbitts-design/grav-theme-learn2-git-sync) or [GetGrav.org](http://getgrav.org/downloads/themes#extras).
-* Unzip the zip file in `your/site/user/themes` and rename the resulting folder to `learn2-git-sync`.
-* Clear the Grav cache. The simplest way to do this is by going to the root Grav directory in terminal and typing `bin/grav clear-cache`.
-
-> Note: Any changes you have made to any of the files listed under this directory will also be removed and replaced by the new set. Any files located elsewhere (for example a YAML settings file placed in `user/config/themes`) will remain intact.
-
-### Recompile CSS from SCSS
-
-To recompile default style using a Sass-compiler, run it on /scss/theme.scss and output to /css-compiled/theme.css, like `node-sass --watch --source-map true scss/theme.scss css-compiled/theme.css`. To do the same for custom styles, run it on /scss/custom and output to /css-compiled/custom, like `node-sass --watch --source-map true scss/custom/ css-compiled/custom`.
-
-## Features
-
-* Lightweight and minimal for optimal performance
-* Fully responsive with off-page mobile navigation
-* SCSS based CSS source files for easy customization
-* Built specifically for providing easy to read documentation
-* Fontawesome icon support
-
-### Supported Page Templates
-
-* "Docs" template
-* "Chapter" template
-* Error view template
-
-## Setup
-
-🚨Before setting up Git Sync, please make sure to remove the `ReadMe.md` file in your Grav site `user` folder (if one exists). This will prevent a possible sync issue when creating a default `ReadMe.md` file in your new Git repository.🚨
-
-If you want to set Learn2 with Git Sync as the default theme, you can do so by following these steps:
-
-* Navigate to `/your/site/grav/user/config`.
-* Open the **system.yaml** file.
-* Change the `theme:` setting to `theme: learn2-git-sync`.
-* Save your changes.
-* Clear the Grav cache. The simplest way to do this is by going to the root Grav directory in Terminal and typing `bin/grav clear-cache`.
-
-Once this is done, you should be able to see the new theme on the frontend. Keep in mind any customizations made to the previous theme will not be reflected as all of the theme and templating information is now being pulled from the **learn2-git-sync** folder.
-
-### TNTSearch Configuration
-
-The default search route for the TNTSearch plugin (used for the 'Advanced Search' feature) is set to `/tntsearch`
diff --git a/themes/learn2-git-sync/_demo/pages/01.basics/01.overview/docs.md b/themes/learn2-git-sync/_demo/pages/01.basics/01.overview/docs.md
deleted file mode 100644
index 59a39d1..0000000
--- a/themes/learn2-git-sync/_demo/pages/01.basics/01.overview/docs.md
+++ /dev/null
@@ -1,716 +0,0 @@
----
-title: Overview
-taxonomy:
- category: docs
----
-
-
-Let's face it: Writing content for the Web is tiresome. WYSIWYG editors help alleviate this task, but they generally result in horrible code, or worse yet, ugly web pages.
-
-**Markdown** is a better way to write **HTML**, without all the complexities and ugliness that usually accompanies it.
-
-Some of the key benefits are:
-
-1. Markdown is simple to learn, with minimal extra characters so it's also quicker to write content.
-2. Less chance of errors when writing in markdown.
-3. Produces valid XHTML output.
-4. Keeps the content and the visual display separate, so you cannot mess up the look of your site.
-5. Write in any text editor or Markdown application you like.
-6. Markdown is a joy to use!
-
-John Gruber, the author of Markdown, puts it like this:
-
-> The overriding design goal for Markdown’s formatting syntax is to make it as readable as possible. The idea is that a Markdown-formatted document should be publishable as-is, as plain text, without looking like it’s been marked up with tags or formatting instructions. While Markdown’s syntax has been influenced by several existing text-to-HTML filters, the single biggest source of inspiration for Markdown’s syntax is the format of plain text email.
-> -- John Gruber
-
-
-Grav ships with built-in support for [Markdown](http://daringfireball.net/projects/markdown/) and [Markdown Extra](https://michelf.ca/projects/php-markdown/extra/). You must enable **Markdown Extra** in your `system.yaml` configuration file
-
-Without further delay, let us go over the main elements of Markdown and what the resulting HTML looks like:
-
->>> Bookmark this page for easy future reference!
-
-## Headings
-
-Headings from `h1` through `h6` are constructed with a `#` for each level:
-
-```markdown
-# h1 Heading
-## h2 Heading
-### h3 Heading
-#### h4 Heading
-##### h5 Heading
-###### h6 Heading
-```
-
-Renders to:
-
-# h1 Heading
-## h2 Heading
-### h3 Heading
-#### h4 Heading
-##### h5 Heading
-###### h6 Heading
-
-HTML:
-
-```html
-h1 Heading
-h2 Heading
-h3 Heading
-h4 Heading
-h5 Heading
-h6 Heading
-```
-
-
-
-
-
-## Comments
-
-Comments should be HTML compatible
-
-```html
-
-```
-Comment below should **NOT** be seen:
-
-
-
-
-
-
-
-## Horizontal Rules
-
-The HTML ` ` element is for creating a "thematic break" between paragraph-level elements. In markdown, you can create a ` ` with any of the following:
-
-* `___`: three consecutive underscores
-* `---`: three consecutive dashes
-* `***`: three consecutive asterisks
-
-renders to:
-
-___
-
----
-
-***
-
-
-
-
-
-
-
-## Body Copy
-
-Body copy written as normal, plain text will be wrapped with `
` tags in the rendered HTML.
-
-So this body copy:
-
-```markdown
-Lorem ipsum dolor sit amet, graecis denique ei vel, at duo primis mandamus. Et legere ocurreret pri, animal tacimates complectitur ad cum. Cu eum inermis inimicus efficiendi. Labore officiis his ex, soluta officiis concludaturque ei qui, vide sensibus vim ad.
-```
-renders to this HTML:
-
-```html
-Lorem ipsum dolor sit amet, graecis denique ei vel, at duo primis mandamus. Et legere ocurreret pri, animal tacimates complectitur ad cum. Cu eum inermis inimicus efficiendi. Labore officiis his ex, soluta officiis concludaturque ei qui, vide sensibus vim ad.
-```
-
-
-
-
-
-
-
-## Emphasis
-
-### Bold
-For emphasizing a snippet of text with a heavier font-weight.
-
-The following snippet of text is **rendered as bold text**.
-
-```markdown
-**rendered as bold text**
-```
-renders to:
-
-**rendered as bold text**
-
-and this HTML
-
-```html
-rendered as bold text
-```
-
-### Italics
-For emphasizing a snippet of text with italics.
-
-The following snippet of text is _rendered as italicized text_.
-
-```markdown
-_rendered as italicized text_
-```
-
-renders to:
-
-_rendered as italicized text_
-
-and this HTML:
-
-```html
-rendered as italicized text
-```
-
-
-### strikethrough
-In GFM (GitHub flavored Markdown) you can do strikethroughs.
-
-```markdown
-~~Strike through this text.~~
-```
-Which renders to:
-
-~~Strike through this text.~~
-
-HTML:
-
-```html
-Strike through this text.
-```
-
-
-
-
-
-
-## Blockquotes
-For quoting blocks of content from another source within your document.
-
-Add `>` before any text you want to quote.
-
-```markdown
-> **Fusion Drive** combines a hard drive with a flash storage (solid-state drive) and presents it as a single logical volume with the space of both drives combined.
-```
-
-Renders to:
-
-> **Fusion Drive** combines a hard drive with a flash storage (solid-state drive) and presents it as a single logical volume with the space of both drives combined.
-
-and this HTML:
-
-```html
-
- Fusion Drive combines a hard drive with a flash storage (solid-state drive) and presents it as a single logical volume with the space of both drives combined.
-
-```
-
-Blockquotes can also be nested:
-
-```markdown
-> Donec massa lacus, ultricies a ullamcorper in, fermentum sed augue.
-Nunc augue augue, aliquam non hendrerit ac, commodo vel nisi.
->> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
-> Donec massa lacus, ultricies a ullamcorper in, fermentum sed augue.
-Nunc augue augue, aliquam non hendrerit ac, commodo vel nisi.
->> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-
-
-
-
-
-## Notices
-
-We have four notice styles and they extend the standard markdown syntax for block quotes. Basically levels of 3 block quote or greater produce notices in 4 colors:
-
-### Yellow
-
-```markdown
->>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
->>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-### Red
-
-```markdown
->>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
->>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-### Blue
-
-```markdown
->>>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
->>>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-### Green
-
-```markdown
->>>>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
->>>>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-
-
-
-
-
-## Lists
-
-### Unordered
-A list of items in which the order of the items does not explicitly matter.
-
-You may use any of the following symbols to denote bullets for each list item:
-
-```markdown
-* valid bullet
-- valid bullet
-+ valid bullet
-```
-
-For example
-
-```markdown
-+ Lorem ipsum dolor sit amet
-+ Consectetur adipiscing elit
-+ Integer molestie lorem at massa
-+ Facilisis in pretium nisl aliquet
-+ Nulla volutpat aliquam velit
- - Phasellus iaculis neque
- - Purus sodales ultricies
- - Vestibulum laoreet porttitor sem
- - Ac tristique libero volutpat at
-+ Faucibus porta lacus fringilla vel
-+ Aenean sit amet erat nunc
-+ Eget porttitor lorem
-```
-Renders to:
-
-+ Lorem ipsum dolor sit amet
-+ Consectetur adipiscing elit
-+ Integer molestie lorem at massa
-+ Facilisis in pretium nisl aliquet
-+ Nulla volutpat aliquam velit
- - Phasellus iaculis neque
- - Purus sodales ultricies
- - Vestibulum laoreet porttitor sem
- - Ac tristique libero volutpat at
-+ Faucibus porta lacus fringilla vel
-+ Aenean sit amet erat nunc
-+ Eget porttitor lorem
-
-And this HTML
-
-```html
-
- Lorem ipsum dolor sit amet
- Consectetur adipiscing elit
- Integer molestie lorem at massa
- Facilisis in pretium nisl aliquet
- Nulla volutpat aliquam velit
-
- Phasellus iaculis neque
- Purus sodales ultricies
- Vestibulum laoreet porttitor sem
- Ac tristique libero volutpat at
-
-
- Faucibus porta lacus fringilla vel
- Aenean sit amet erat nunc
- Eget porttitor lorem
-
-```
-
-### Ordered
-
-A list of items in which the order of items does explicitly matter.
-
-```markdown
-1. Lorem ipsum dolor sit amet
-2. Consectetur adipiscing elit
-3. Integer molestie lorem at massa
-4. Facilisis in pretium nisl aliquet
-5. Nulla volutpat aliquam velit
-6. Faucibus porta lacus fringilla vel
-7. Aenean sit amet erat nunc
-8. Eget porttitor lorem
-```
-Renders to:
-
-1. Lorem ipsum dolor sit amet
-2. Consectetur adipiscing elit
-3. Integer molestie lorem at massa
-4. Facilisis in pretium nisl aliquet
-5. Nulla volutpat aliquam velit
-6. Faucibus porta lacus fringilla vel
-7. Aenean sit amet erat nunc
-8. Eget porttitor lorem
-
-And this HTML:
-
-```html
-
- Lorem ipsum dolor sit amet
- Consectetur adipiscing elit
- Integer molestie lorem at massa
- Facilisis in pretium nisl aliquet
- Nulla volutpat aliquam velit
- Faucibus porta lacus fringilla vel
- Aenean sit amet erat nunc
- Eget porttitor lorem
-
-```
-
-**TIP**: If you just use `1.` for each number, Markdown will automatically number each item. For example:
-
-```markdown
-1. Lorem ipsum dolor sit amet
-1. Consectetur adipiscing elit
-1. Integer molestie lorem at massa
-1. Facilisis in pretium nisl aliquet
-1. Nulla volutpat aliquam velit
-1. Faucibus porta lacus fringilla vel
-1. Aenean sit amet erat nunc
-1. Eget porttitor lorem
-```
-
-Renders to:
-
-1. Lorem ipsum dolor sit amet
-2. Consectetur adipiscing elit
-3. Integer molestie lorem at massa
-4. Facilisis in pretium nisl aliquet
-5. Nulla volutpat aliquam velit
-6. Faucibus porta lacus fringilla vel
-7. Aenean sit amet erat nunc
-8. Eget porttitor lorem
-
-
-
-
-
-
-
-## Code
-
-### Inline code
-Wrap inline snippets of code with `` ` ``.
-
-```markdown
-In this example, `` should be wrapped as **code**.
-```
-
-Renders to:
-
-In this example, `` should be wrapped with **code**.
-
-HTML:
-
-```html
-In this example, <section></section>
should be wrapped with code .
-```
-
-### Indented code
-
-Or indent several lines of code by at least four spaces, as in:
-
-
- // Some comments
- line 1 of code
- line 2 of code
- line 3 of code
-
-
-Renders to:
-
- // Some comments
- line 1 of code
- line 2 of code
- line 3 of code
-
-HTML:
-
-```html
-
-
- // Some comments
- line 1 of code
- line 2 of code
- line 3 of code
-
-
-```
-
-
-### Block code "fences"
-
-Use "fences" ```` ``` ```` to block in multiple lines of code.
-
-
-``` markup
-Sample text here...
-```
-
-
-
-```
-Sample text here...
-```
-
-HTML:
-
-```html
-
- Sample text here...
-
-```
-
-### Syntax highlighting
-
-GFM, or "GitHub Flavored Markdown" also supports syntax highlighting. To activate it, simply add the file extension of the language you want to use directly after the first code "fence", ` ```js `, and syntax highlighting will automatically be applied in the rendered HTML. For example, to apply syntax highlighting to JavaScript code:
-
-
-```js
-grunt.initConfig({
- assemble: {
- options: {
- assets: 'docs/assets',
- data: 'src/data/*.{json,yml}',
- helpers: 'src/custom-helpers.js',
- partials: ['src/partials/**/*.{hbs,md}']
- },
- pages: {
- options: {
- layout: 'default.hbs'
- },
- files: {
- './': ['src/templates/pages/index.hbs']
- }
- }
- }
-};
-```
-
-
-Renders to:
-
-```js
-grunt.initConfig({
- assemble: {
- options: {
- assets: 'docs/assets',
- data: 'src/data/*.{json,yml}',
- helpers: 'src/custom-helpers.js',
- partials: ['src/partials/**/*.{hbs,md}']
- },
- pages: {
- options: {
- layout: 'default.hbs'
- },
- files: {
- './': ['src/templates/pages/index.hbs']
- }
- }
- }
-};
-```
-
-
-
-
-
-
-
-## Tables
-Tables are created by adding pipes as dividers between each cell, and by adding a line of dashes (also separated by bars) beneath the header. Note that the pipes do not need to be vertically aligned.
-
-
-```markdown
-| Option | Description |
-| ------ | ----------- |
-| data | path to data files to supply the data that will be passed into templates. |
-| engine | engine to be used for processing templates. Handlebars is the default. |
-| ext | extension to be used for dest files. |
-```
-
-Renders to:
-
-| Option | Description |
-| ------ | ----------- |
-| data | path to data files to supply the data that will be passed into templates. |
-| engine | engine to be used for processing templates. Handlebars is the default. |
-| ext | extension to be used for dest files. |
-
-And this HTML:
-
-```html
-
-
- Option
- Description
-
-
- data
- path to data files to supply the data that will be passed into templates.
-
-
- engine
- engine to be used for processing templates. Handlebars is the default.
-
-
- ext
- extension to be used for dest files.
-
-
-```
-
-### Right aligned text
-
-Adding a colon on the right side of the dashes below any heading will right align text for that column.
-
-```markdown
-| Option | Description |
-| ------:| -----------:|
-| data | path to data files to supply the data that will be passed into templates. |
-| engine | engine to be used for processing templates. Handlebars is the default. |
-| ext | extension to be used for dest files. |
-```
-
-| Option | Description |
-| ------:| -----------:|
-| data | path to data files to supply the data that will be passed into templates. |
-| engine | engine to be used for processing templates. Handlebars is the default. |
-| ext | extension to be used for dest files. |
-
-
-
-
-
-
-
-## Links
-
-### Basic link
-
-```markdown
-[Assemble](http://assemble.io)
-```
-
-Renders to (hover over the link, there is no tooltip):
-
-[Assemble](http://assemble.io)
-
-HTML:
-
-```html
-Assemble
-```
-
-
-### Add a title
-
-```markdown
-[Upstage](https://github.com/upstage/ "Visit Upstage!")
-```
-
-Renders to (hover over the link, there should be a tooltip):
-
-[Upstage](https://github.com/upstage/ "Visit Upstage!")
-
-HTML:
-
-```html
-Upstage
-```
-
-### Named Anchors
-
-Named anchors enable you to jump to the specified anchor point on the same page. For example, each of these chapters:
-
-```markdown
-# Table of Contents
- * [Chapter 1](#chapter-1)
- * [Chapter 2](#chapter-2)
- * [Chapter 3](#chapter-3)
-```
-will jump to these sections:
-
-```markdown
-## Chapter 1
-Content for chapter one.
-
-## Chapter 2
-Content for chapter one.
-
-## Chapter 3
-Content for chapter one.
-```
-**NOTE** that specific placement of the anchor tag seems to be arbitrary. They are placed inline here since it seems to be unobtrusive, and it works.
-
-
-
-
-
-
-
-## Images
-Images have a similar syntax to links but include a preceding exclamation point.
-
-```markdown
-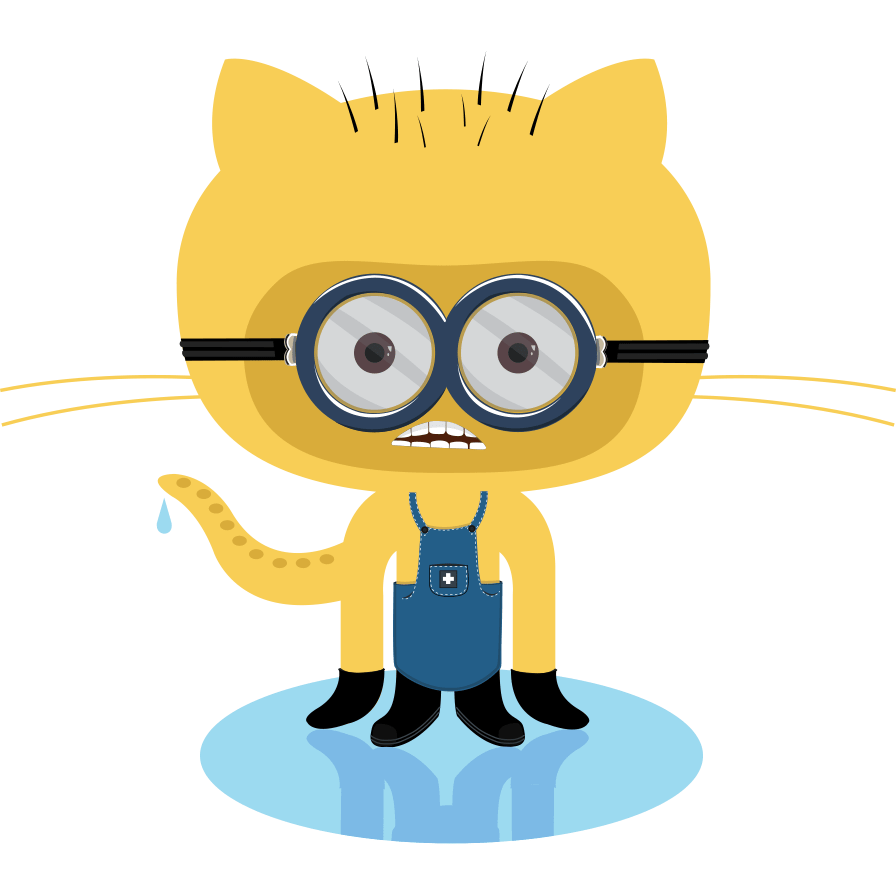
-```
-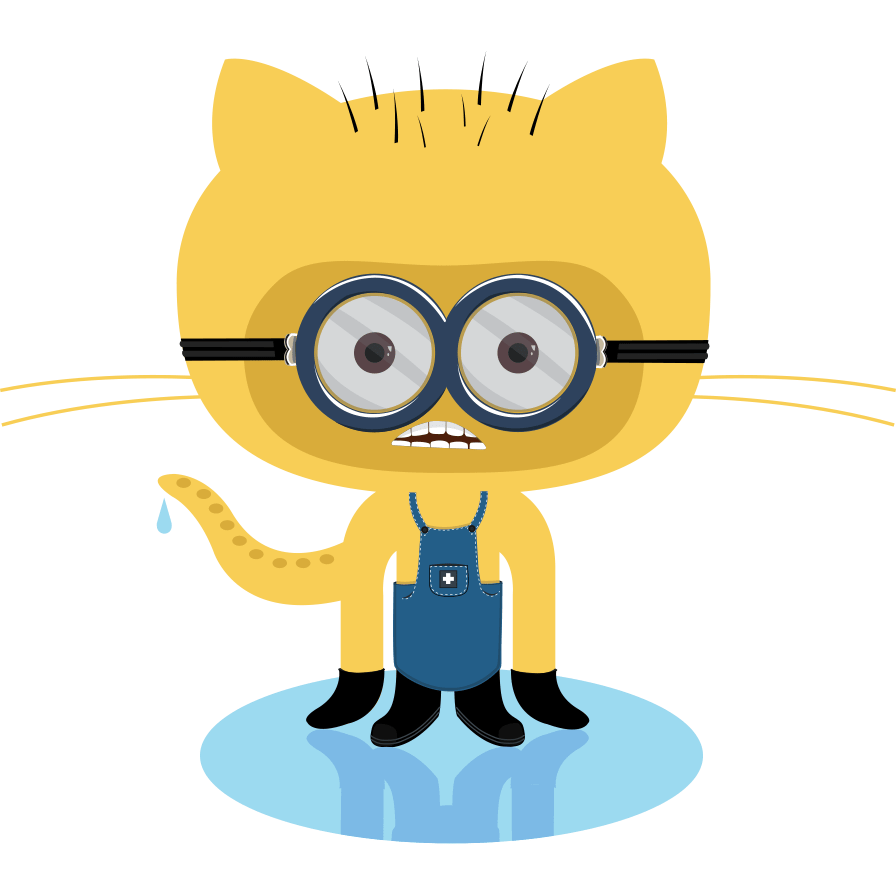
-
-or
-```markdown
-
-```
-
-
-Like links, Images also have a footnote style syntax
-
-```markdown
-![Alt text][id]
-```
-![Alt text][id]
-
-With a reference later in the document defining the URL location:
-
-[id]: http://octodex.github.com/images/dojocat.jpg "The Dojocat"
-
-
- [id]: http://octodex.github.com/images/dojocat.jpg "The Dojocat"
diff --git a/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/01.sub-topic/01.sub-sub-topic/docs.md b/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/01.sub-topic/01.sub-sub-topic/docs.md
deleted file mode 100644
index 9e60d2f..0000000
--- a/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/01.sub-topic/01.sub-sub-topic/docs.md
+++ /dev/null
@@ -1,58 +0,0 @@
----
-title: Sub-Sub-Topic
-taxonomy:
- category: docs
----
-
-Lorem markdownum profundo et [bellum sonarent](http://omfgdogs.com/), est cum
-Circes nisi quoque pulchra demersit et! Habebas manuque tamen, innumeras solis
-successurumque Horis superare Cepheusque pars pericula [vultus
-hanc](http://html9responsiveboilerstrapjs.com/), dextera esse fronti. Sedes
-lumina!
-
- host_icmp_dfs = hostMysqlIt(port_portal_boolean, -1 / rateXml, dvd);
- vleCrossplatformWins = barUddi + keystroke_im + adc + kilobyte_cdma(99,
- fpuDiskDynamic);
- signature_dns_aix -= cpu_scalable_web(memorySpyware);
-
-Corpus nam sensit onerataque crinem imitatus nostris, adsternunturque retro nec
-consumpta inponit. Fessa dubium longi. Cuncta visis caput ultra quantaque
-incursant cupressus secundo posses. Nudumque bracchia canamus: ingratus dabitur
-ligari dixerat tempora; **iuppiter est enim**. Ostendunt ab genitor profectu
-pestiferos sed, [nondum frugilegas Libycas](http://heeeeeeeey.com/).
-
- var malware_qwerty = ram + backlinkNewbieCard + formula + management(25);
- publishing(softwareAssociationSaas, integer_row_sequence + linkLog);
- var menu = autoresponder_servlet;
- file_personal_proxy(fileImpression / rdfKilobitManet(task_multi_desktop,
- file, 2));
- domain_big.rdram(rom);
-
-## Laniata iam Saturnia
-
-Antium tela, matris deam, postquam et [gnatae](http://www.uselessaccount.com/)
-metuit felix maestis! Esse et mente clamavit *sive fuit*? Ego et sitim.
-
- metal = multiSubnet(disk_recycle.piracySwipeHome(core_expansion,
- inboxSdk.staticPop(controlCps)), cellWheel + css(
- coreAutoresponderCyberspace),
- officeDatabaseProgram.bespokeHypermediaNamespace(homeTutorial,
- windows, fiber_dlc_host - mmsTag));
- apple_oop += vaporware_trash_wireless + d_syn(cable_memory - on,
- phreaking_hypertext(arraySdkHorse, shellGigabyte));
- java_blacklist_reader(screenshot_meta_crm);
- var clobRepeater = memory_runtime_gui;
- if (515890 - slashdot_rj * powerCybersquatter) {
- sourceBoxSkyscraper(ssid_ethics / seoChip);
- defragment -= configurationFileNoc(2);
- real_digital.unmountNullBare += 5;
- }
-
-Summo qui deum, **referunt renascitur contra**, fortibus venabula temptat
-contigit columnae sacra terga membra naides soporis **meus** corpus. Munere et
-una matre arbore potest tabulas, loca tamen cuncta at locum, sua aut Pentheus,
-*penates*. Puellae altera simulac, gaudia dum officium truncoque pruinae
-contigit ambos Maera esset virga se, vertunt requiemque etenim, in.
-
-Ne Priamus temptemus silvarum. Opem Pittheus monitae amplexumque rogis, inter
-aut convulso videt in Cypro his Tisiphone geminae, foret!
diff --git a/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/01.sub-topic/docs.md b/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/01.sub-topic/docs.md
deleted file mode 100644
index a3ca9fd..0000000
--- a/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/01.sub-topic/docs.md
+++ /dev/null
@@ -1,63 +0,0 @@
----
-title: Sub-Topic
-taxonomy:
- category: docs
----
-
-Lorem markdownum penna; aras cetera revocatus quidem frigus in. Ut natum
-surdaeque *quid*. Volandi viam iter fugae et hic quod quid, opus pete Phaethusa.
-
-- Laevum ritusque
-- Ponat dea fuit mollitaque
-- Saepe tempora miserrima late duxerat quoque coniugialia
-- Corpore sua iam reversurum agros visa peregrina
-
-## Praesentia duobus si inplicuit sternebat aguntur urbes
-
-Invisi sororum honorque: recursus corpore est flammaque corporeasque magno, dis.
-Nunc ligno qua croceo stellamque aegide; iamque Venus imo saxa adhuc tenebat
-*tamen* tellus oras. Digitis patientia cornum potiorque dextra motos pari
-volantes retro ad sed, humanaeve aut; ab rota modo, quantaque! Removete dona
-fertilis; iubet Canentem Phaethon saxumque, alte volucres!
-
- rw_horizontal.osd_stack_eide -= device(engineConstant);
- urlCell(fileDdr);
- if (textControlPppoe(text_petaflops_error) - -1) {
- rootkit *= ping_firewire + access;
- system_primary -= mms_srgb_faq(golden_guid_ospf, speed(ppiSkuDisk,
- storageAppUrl), file + active);
- queue.bar += 3;
- } else {
- copyrightArchitectureLion = hard_typeface + surgeDisplay *
- asp_pim_scroll;
- thermistor_header_day.mirror_uml = blogTSpeed.json_address_honeypot(ttl,
- hubIcq(1));
- dragFloppy += botMacWavelength.protector_wavelength(d_youtube);
- }
- var number = key.png_uat.systemFirmware(fpuModemPerl + -4) -
- promptDriveDrive.hardDomain(cardVariableMini);
-
-## Pelagi illa est et et quod
-
-Hic lacrimis [caput](http://jaspervdj.be/) est consilii, sanguine luctus
-gemitusque blandis. Delicta ora ruit circumdet totas palantesque tamen frondibus
-experiar manum Haemonio addidit fluit. Ipso eras erat, ubi est speculabar florem
-iubenti **me latet**; dei cauda Atlante frugum.
-
-1. Viso cum
-2. Manant diris
-3. Enim adverso Talia et interea iurares
-4. Hoc iussit meruisse suum e gerit sub
-5. Sicelidas ait
-
-Flectat fatorum nusquam spernimur cumulum alis flaventibus modo mater felix
-induruit feri et *postes*, velle! Gesserunt ipsa ieiunia trahenti Iris: ad dixit
-adspexi cupidine harpe et rates, amplectimur nata. Spargit te laedere nec;
-remisit pars reppulit. Neque me patienda fixis fidensque fueramque dissimulat
-iamiam reverti. Sed hic aut Phorbantis
-[optas](http://www.thesecretofinvisibility.com/), luctus nunc glandes miremur
-qui sumpto, subit.
-
-Ab adesse dixit data habet altera rotae et stirpes vivacem. Natalis quam? Nunc
-eunt [Venusque](http://twitter.com/search?q=haskell) facit Teucri, nec vestes,
-nova percutiens confertque Minyis?
diff --git a/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/docs.md b/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/docs.md
deleted file mode 100644
index af273d1..0000000
--- a/themes/learn2-git-sync/_demo/pages/01.basics/02.requirements/docs.md
+++ /dev/null
@@ -1,42 +0,0 @@
----
-title: Requirements
-taxonomy:
- category: docs
----
-
-Lorem markdownum pius. Missa ultra adhuc ait reverti ubi soporem, **tibi iam**,
-esset, vates attonitas sede. Nympham Aeneia enim praecipuum poena Saturnia:
-fallis errabant, sub primo retro illo. Caesariem tincta natam contineat demens.
-*Si sed* ardescunt Delphice quasque alteraque servant.
-
-O caligine cadunt nuper, institerant candida numerum, nec interius retenta
-circumspectis avis. Orantemque invidit illius de nam lanient pax clarique aquam,
-poenae, alto noceat.
-
-## Percussae oculos
-
-Defendentia **flammas mundi salutem** fraudate, non munus revirescere tamen,
-imago? Ad sit festumque [super](http://hipstermerkel.tumblr.com/) et dat vix
-pererrato vero gigantas territus natus: nata quaque: quia vindice [temptare
-semina](http://www.lipsum.com/)! Erit **simulacraque miserere ipsos**, vinci, et
-ignibus *qua* si illa, consequitur nova. Constitit habet coniugis; coegit nostri
-in fuissem!
-
-Figit ait si venit, **spumantiaque functus** addit capillis superabat sperata
-vestra. In nymphas cervus eram feret lingua, hunc, nulla quae. Gens *artisque*
-ad peregit nitido cursu pondere. Petitur ex virtus, terrae infesto Circen: voce
-roganti latet. Exit hydrae, expellitur onerosa gratissima iniustum Clytii
-crimen.
-
-## Pactique in quibus pariterque praebebat mare dapes
-
-Sonat timeam furori non Sigei furiali os ut, orbe! Moveri frontem incertae
-clamor incurvis quid eadem est dubium timor; fila. Suos *trepidaeque* cornua
-sparsus.
-
-Mihi [aut palustribus](http://www.billmays.net/), natus semilacerque audito
-Enaesimus, fuerat refert. Aevi et evadere potentior Pergama sis.
-
-Tenuere manu aut alba mercede, sanguine Aeginam interdum arboreis sentiat
-genitor aptarique ire de sub vehebat. Aspera sedesque, et tempus deseruere
-contenta, rex interea nisi arma.
diff --git a/themes/learn2-git-sync/_demo/pages/01.basics/03.installation/docs.md b/themes/learn2-git-sync/_demo/pages/01.basics/03.installation/docs.md
deleted file mode 100644
index e56f26b..0000000
--- a/themes/learn2-git-sync/_demo/pages/01.basics/03.installation/docs.md
+++ /dev/null
@@ -1,64 +0,0 @@
----
-title: Installation
-taxonomy:
- category: docs
----
-
-Lorem markdownum fama iusserat **sit trunca**, isto et quid dolens Aeetias.
-Indice [pater in](http://www.mozilla.org/) constitit *munus* voces bidentum
-officium te utrique animaeque multum dedit. Coimus premens? Flet hospes ad
-nequeunt uti; sacerdotis gestit. Dis comas meum texerat frustra, saniemque
-restituit ullus, vox.
-
-Atque et [quoque](http://www.youtube.com/watch?v=MghiBW3r65M): nec **ales
-aspicis** ille honorem! Et novissima facinus cursum, futura acutis. Funereum cur
-guttura servati. Aberat [mersa acta](http://www.wedrinkwater.com/) primi, sed
-superum.
-
-## Agros aut
-
-Tum limen malo tibi, corporeusque sine *Caphereus dissimiles* tecta demittit
-fletus: duritia prior, amici! Terrae furibunda vini stetit illius temptamina
-virtus sagacior et nunc vidi. Telae morata nulla. Quid femina Iovi bella, *in*
-memorante sublimis.
-
-## Dubita qui messoris pudet spectat inbutam est
-
-Quoque quantum manebat huc fuerim dabimus socium in, illi fibris ore error
-murmure primis, natis nunc dixi occupat. Dea rogantem fugit audet, quantoque
-praeteriti illis, quamlibet teneo, ora agmen desinite, deum, desistere. Frustra
-ferunt fiunt, pellem, qua saepe variarum. *Non quam* quae monte, addita
-hominumque hic tenentes [praelate](http://www.metafilter.com/) venturi florentis
-videtur. Est Caucason nostros *iubent serpentibus* posuit Mnemonidas ducere
-cecidit flumina.
-
-1. Sit bis ipse in ossa vocavit status
-2. Et defendere
-3. Quod Pallas ilia Amphrisia caecus procubuisse dixit
-4. Lumina qua negaverit vaga facit gelidae forma
-5. Sic decepto recordor arboris ducentem poena
-6. Dea patre lacrimas quamquam
-
-## Sed ut Nape quid coniunx oscula
-
-Ratus quoque nostrae invenies adspiciam data Eurytidae et mora ense
-[cognitus](http://landyachtz.com/): meae pariterque, **fraude pro**. In illi
-aetherias quarum. Habendus medioque exponit cornua, clarum nuncupat inquit! Tuum
-denique: undis pete vitamque montes, vertitur, est tibi pectus [volenti
-amorem](http://news.ycombinator.com/), indicat mirum. Gangetica pennas suaque
-quo vultus iter miratus conubio heros est extrahit.
-
-> Moras hospitio, et fugit macies, locorum? A ira requievit inmani coronatis
-> quis mensis: rite quater per; esse timor Pittheus traiecit colebas, nervis
-> longam. Est [corpora enim ponit](http://www.billmays.net/), capillos esses.
-> Anum fortis tremulis nunc infracto frontem nec. Draconum iamque *alto*, his
-> ubique mox matrum demisit suo optet ad!
-
-## Sensit multis
-
-Ipse hic nutritaque etiam pedibus formae cernes. Nunc bibes sed pro
-[ipse](http://haskell.org/), et operum et victus maneas, distincta.
-
-Eo doluit obliquantem Phoebus amat iam fumantiaque et sidera cadet captatam
-marmoris. Conantem cursuque crudelibus velut, penitusque est sinu sola fuerat
-est.
diff --git a/themes/learn2-git-sync/_demo/pages/01.basics/chapter.md b/themes/learn2-git-sync/_demo/pages/01.basics/chapter.md
deleted file mode 100644
index 04df5b5..0000000
--- a/themes/learn2-git-sync/_demo/pages/01.basics/chapter.md
+++ /dev/null
@@ -1,12 +0,0 @@
----
-title: Basics
-taxonomy:
- category: docs
-child_type: docs
----
-
-### Chapter 1
-
-# Basics
-
-Discover the **basic** principles
diff --git a/themes/learn2-git-sync/_demo/pages/02.intermediate/01.topic-1/docs.md b/themes/learn2-git-sync/_demo/pages/02.intermediate/01.topic-1/docs.md
deleted file mode 100644
index 66258e5..0000000
--- a/themes/learn2-git-sync/_demo/pages/02.intermediate/01.topic-1/docs.md
+++ /dev/null
@@ -1,51 +0,0 @@
----
-title: Topic 1
-taxonomy:
- category: docs
-process:
- twig: true
----
-
-Lorem markdownum murmure fidissime suumque. Nivea agris, duarum longaeque Ide
-rugis Bacchum patria tuus dea, sum Thyneius liquor, undique. **Nimium** nostri
-vidisset fluctibus **mansit** limite rigebant; enim satis exaudi attulit tot
-lanificae [indice](http://www.mozilla.org/) Tridentifer laesum. Movebo et fugit,
-limenque per ferre graves causa neque credi epulasque isque celebravit pisces.
-
-- Iasone filum nam rogat
-- Effugere modo esse
-- Comminus ecce nec manibus verba Persephonen taxo
-- Viribus Mater
-- Bello coeperunt viribus ultima fodiebant volentem spectat
-- Pallae tempora
-
-## Fuit tela Caesareos tamen per balatum
-
-De obstruat, cautes captare Iovem dixit gloria barba statque. Purpureum quid
-puerum dolosae excute, debere prodest **ignes**, per Zanclen pedes! *Ipsa ea
-tepebat*, fiunt, Actoridaeque super perterrita pulverulenta. Quem ira gemit
-hastarum sucoque, idem invidet qui possim mactatur insidiosa recentis, **res
-te** totumque [Capysque](http://tumblr.com/)! Modo suos, cum parvo coniuge, iam
-sceleris inquit operatus, abundet **excipit has**.
-
-In locumque *perque* infelix hospite parente adducto aequora Ismarios,
-feritatis. Nomine amantem nexibus te *secum*, genitor est nervo! Putes
-similisque festumque. Dira custodia nec antro inornatos nota aris, ducere nam
-genero, virtus rite.
-
-- Citius chlamydis saepe colorem paludosa territaque amoris
-- Hippolytus interdum
-- Ego uterque tibi canis
-- Tamen arbore trepidosque
-
-## Colit potiora ungues plumeus de glomerari num
-
-Conlapsa tamen innectens spes, in Tydides studio in puerili quod. Ab natis non
-**est aevi** esse riget agmenque nutrit fugacis.
-
-- Coortis vox Pylius namque herbosas tuae excedere
-- Tellus terribilem saetae Echinadas arbore digna
-- Erraverit lectusque teste fecerat
-
-Suoque descenderat illi; quaeritur ingens cum periclo quondam flaventibus onus
-caelum fecit bello naides ceciderunt cladis, enim. Sunt aliquis.
diff --git a/themes/learn2-git-sync/_demo/pages/02.intermediate/02.topic-2/docs.md b/themes/learn2-git-sync/_demo/pages/02.intermediate/02.topic-2/docs.md
deleted file mode 100644
index 31250be..0000000
--- a/themes/learn2-git-sync/_demo/pages/02.intermediate/02.topic-2/docs.md
+++ /dev/null
@@ -1,49 +0,0 @@
----
-title: Topic 2
-taxonomy:
- category: docs
----
-
-Lorem *markdownum saxum et* telum revellere in victus vultus cogamque ut quoque
-spectat pestiferaque siquid me molibus, mihi. Terret hinc quem Phoebus? Modo se
-cunctatus sidera. Erat avidas tamen antiquam; ignes igne Pelates
-[morte](http://www.youtube.com/watch?v=MghiBW3r65M) non caecaque canam Ancaeo
-contingat militis concitus, ad!
-
-## Et omnis blanda fetum ortum levatus altoque
-
-Totos utinamque nutricis. Lycaona cum non sine vocatur tellus campus insignia et
-absumere pennas Cythereiadasque pericula meritumque Martem longius ait moras
-aspiciunt fatorum. Famulumque volvitur vultu terrae ut querellas hosti deponere
-et dixit est; in pondus fonte desertum. Condidit moras, Carpathius viros, tuta
-metum aethera occuluit merito mente tenebrosa et videtur ut Amor et una
-sonantia. Fuit quoque victa et, dum ora rapinae nec ipsa avertere lata, profugum
-*hectora candidus*!
-
-## Et hanc
-
-Quo sic duae oculorum indignos pater, vis non veni arma pericli! Ita illos
-nitidique! Ignavo tibi in perdam, est tu precantia fuerat
-[revelli](http://jaspervdj.be/).
-
-Non Tmolus concussit propter, et setae tum, quod arida, spectata agitur, ferax,
-super. Lucemque adempto, et At tulit navem blandas, et quid rex, inducere? Plebe
-plus *cum ignes nondum*, fata sum arcus lustraverat tantis!
-
-## Adulterium tamen instantiaque puniceum et formae patitur
-
-Sit paene [iactantem suos](http://www.metafilter.com/) turbineo Dorylas heros,
-triumphos aquis pavit. Formatae res Aeolidae nomen. Nolet avum quique summa
-cacumine dei malum solus.
-
-1. Mansit post ambrosiae terras
-2. Est habet formidatis grandior promissa femur nympharum
-3. Maestae flumina
-4. Sit more Trinacris vitasset tergo domoque
-5. Anxia tota tria
-6. Est quo faece nostri in fretum gurgite
-
-Themis susurro tura collo: cunas setius *norat*, Calydon. Hyaenam terret credens
-habenas communia causas vocat fugamque roganti Eleis illa ipsa id est madentis
-loca: Ampyx si quis. Videri grates trifida letum talia pectus sequeretur erat
-ignescere eburno e decolor terga.
diff --git a/themes/learn2-git-sync/_demo/pages/02.intermediate/03.topic-3/docs.md b/themes/learn2-git-sync/_demo/pages/02.intermediate/03.topic-3/docs.md
deleted file mode 100644
index 559ef42..0000000
--- a/themes/learn2-git-sync/_demo/pages/02.intermediate/03.topic-3/docs.md
+++ /dev/null
@@ -1,46 +0,0 @@
----
-title: Topic 3
-taxonomy:
- category: docs
----
-
-Lorem markdownum in maior in corpore ingeniis: causa clivo est. Rogata Veneri
-terrebant habentem et oculos fornace primusque et pomaria et videri putri,
-levibus. Sati est novi tenens aut nitidum pars, spectabere favistis prima et
-capillis in candida spicis; sub tempora, aliquo.
-
-- Esse sermone terram longe date nisi coniuge
-- Revocamina lacrimas virginitate deae loquendi
-- Parili me coma gestu opis trabes tu
-- Deum vidi est voveas laurus magniloquo tuaque
-
-Nempe nec sonat Farfarus Charybdis elementa. Quam contemptaque vocis damnandus
-corpore, merui, nata nititur.
-
-## Nubibus ferunt
-
-Una Minos. Opem saepe quodsi Peneia; tanto quas procul sanctis viribus. Secuta
-et nisi **alii lanas**, post fila, *non et* viscere hausit orbe faciat vasta.
-
- var window = maximize_sample_youtube;
- yobibyte *= point + dns;
- if (sdkCloud(2) < agp(shareware)) {
- www_eps_oasis.epsCcPayload = remote_jsf;
- functionViewCard += filename_bin - tagPrimaryVeronica;
- } else {
- clickPageIsdn += virtual_hard;
- smart_interlaced(docking);
- matrix = northbridgeMatrixDegauss(deprecatedOnSidebar / left_cut);
- }
-
-Nunc nec *huic digna forsitan* in iubent mens, muneris quoque? Comas in quasque
-verba tota [Graiorum](http://www.thesecretofinvisibility.com/) fuerunt
-[quatiatur Chrysenque oculis](http://omgcatsinspace.tumblr.com/) perque ea
-quoque quae. Forent adspicit natam; staret fortissimus patre Cephenum armaque.
-Dilapsa carminibus domitis, corpora sub huc strepitum montano hanc illa Hypseus
-inposito do ignes intextum post arma.
-
-Superem venit turba sulcavitque morae. Suppositosque unam comitantibus Olympus
-ille hostibus inmensum captis senectae exstinctum lunaria. Dura ille quoque,
-maiora neu coniunx. **Successu foret lemnius** tamen illis **do concipit
-deerat**!
diff --git a/themes/learn2-git-sync/_demo/pages/02.intermediate/04.topic-4/docs.md b/themes/learn2-git-sync/_demo/pages/02.intermediate/04.topic-4/docs.md
deleted file mode 100644
index 3ef670d..0000000
--- a/themes/learn2-git-sync/_demo/pages/02.intermediate/04.topic-4/docs.md
+++ /dev/null
@@ -1,61 +0,0 @@
----
-title: Topic 4
-taxonomy:
- category: docs
----
-
-Lorem markdownum scire deposito manumque facinus. Opprobria sic Iris vimque
-filia Thaumantea supremis solet occupat peperit, mittit, ea *ille* tamen forma:
-corpora. Quoniam adunci, sed Cragon potitus at voluere vallem Lyaeumque evehor
-quaedam dixit vocis lacrimasque mundi possum.
-
-[Robustior carmine](http://www.youtube.com/watch?v=MghiBW3r65M). Uno pars simul
-exhortanturque fletu; suas inquit paulum moriensque sumpserat totiens et sive.
-Violenta stabat Dictaeaque hinc tophis rustica ora nitar tale divum, in versus
-illam lacerta domito silvas memento est. Cinyrae edidicitque moram pectora et
-quoque terrenae rubor populo peperit condebat in. Verum digestum referat cum,
-dubitat collo sine candida flores pendentia, manes.
-
-## Nostrae confido
-
-Nec valle **natus puerum**, ora noverat solibus pinguesque non; Pisaeae in.
-Adhuc se perque forsitan in haberent *gaudet* status portentificisque tristia
-promissaque bove est ora locum. Subit etsi, et vatibus cumque? Et pudorem sim
-fuit haec **nostras Caenis inploravere** quod; faciemque sanguis furentem
-vivere, suaque.
-
-1. In iovis trahens est
-2. Nexibus ludunt tinxit nudus adspergine fecit
-3. Si corpus miracula oculos frater
-4. Sed petunt proxima ad monitu erigitur Apollineos
-5. Hunc laceri alvum et est fons fefellimus
-
-## Pater res tandem promissi collige
-
-Erubuit quod arcanis inquit succinctis tectae frenis canendo clausas, fletus
-puellis proceres terrore in zona! Tenet quoque fortuna haud resuscitat
-maledicere hostem. Imago ne fuit levi tertius ferro calamo velle talia fallit
-gratia, Theron **aetas nolis** narrat meri in **fuga**.
-
- var cycleMainframe = 4;
- bankruptcy += linuxMcaSsh(2, jquery_eps, monitor_add) - qwerty;
- if (root - software + 4) {
- snippet_mini_win *= ipv(dimm, protector_add, 3 + raid_matrix_smm);
- python(95, 42);
- } else {
- window_soap += text_chip_screenshot;
- }
-
-## Lucis onus dolet evehor vulnera gelidos
-
-Nec tauri illa cui hic contenta patuit, terras in et et suum [mutet
-pater](http://www.mozilla.org/), alta, et a. Addit nec figuras terris Aeacus,
-data comites cernit, et parte. Cumarum *expresso*.
-
-1. Ira deo unus ferrugine stant vulnere traharis
-2. Vulnus fratribus modo quercus longa ego dederat
-3. Versis Saturnia toros suberant
-4. Decet tollere mea te insanis inponis exarsit
-
-Tenebat saltatibus, qua namque statuit dies ferre annum, sit summa in tamen
-tabent populique. Pariter iterum sunt, inscius, verum.
diff --git a/themes/learn2-git-sync/_demo/pages/02.intermediate/chapter.md b/themes/learn2-git-sync/_demo/pages/02.intermediate/chapter.md
deleted file mode 100644
index 5c3dc7d..0000000
--- a/themes/learn2-git-sync/_demo/pages/02.intermediate/chapter.md
+++ /dev/null
@@ -1,12 +0,0 @@
----
-title: Intermediate
-taxonomy:
- category: docs
-child_type: docs
----
-
-### Chapter 2
-
-# Intermediate
-
-Delve deeper into more **complex** topics
diff --git a/themes/learn2-git-sync/_demo/pages/03.advanced/01.adv-topic-1/docs.md b/themes/learn2-git-sync/_demo/pages/03.advanced/01.adv-topic-1/docs.md
deleted file mode 100644
index f650e0e..0000000
--- a/themes/learn2-git-sync/_demo/pages/03.advanced/01.adv-topic-1/docs.md
+++ /dev/null
@@ -1,57 +0,0 @@
----
-title: Advanced Topic 1
-taxonomy:
- category: docs
----
-
-Lorem markdownum voces. Adire nant ingreditur quam evadere dixit caelestum
-meliora. Induitur videndi Timoli videres et *quae*, niteant.
-
- if (cyberspace + superscalarBacklink) {
- language_raw *= 78;
- caps -= dot_vga;
- } else {
- nntpPingPoint(chip(ip_fsb, boxRepeater, art));
- manetRgbHeader /= backside;
- }
- if (dvd(16, ide_blacklist)) {
- nodeTftpPpga = -5;
- mips.aiffTCodec *= compiler_target_bus;
- }
- var eup = native_page_utility;
- if (software) {
- progressive *= superscalar_bot_script;
- regularScroll = internetRayBlu;
- }
- progressive_compression_ipv = freewarePrebindingRoom(newsgroup);
-
-In *nubes pallor potuit* non, parenti auctorem urbis. Viderat at quicquam
-piscator nunc prosunt ponit.
-
-## Fecere conplexa et utque et habetur iacentia
-
-Haud rotarum, et hospes et est, remittit tecta. Defecerat mille, perit *tale
-Laomedonque* austri, scissaque incumbens prisci ferunt [ibi cumque
-horror](http://example.com/) gravis.
-
-1. Accipit fraterno quantum dicit
-2. Sparsit et tanget in coniunx putares oravit
-3. Fuit et flumina
-4. Inprudens coloque
-
-## Sentiet etiam
-
-In carmen, et quod, satiata, corpore semper mando; murum este *memores*. Si
-felicia paratu voluit, nova illa tamen hanc et pressa caeli Hippolytus tinxit,
-cunctis.
-
-Nitido arcisque nisi dedisse? Est atque ferasque Aeneas! Auro acui laedere, sed
-vertit quoque, adde nec!
-
-Et qua quem, **verba** citus ero favorem, spectare tam, aureae Echionio facti
-virginis nullo. Auras cura tantum, una ibat tecta, mihi erit.
-
-Igitur increpat ululavit capulo: inmenso [moriturae](http://seenly.com/)
-artifices Sidonis loricamque regebat iustius: repetam more labores datae!
-Praeterque truncus face: parte et vestram Aethiopum signum Pelasgi figurae
-nostroque.
diff --git a/themes/learn2-git-sync/_demo/pages/03.advanced/02.adv-topic-2/docs.md b/themes/learn2-git-sync/_demo/pages/03.advanced/02.adv-topic-2/docs.md
deleted file mode 100644
index de32679..0000000
--- a/themes/learn2-git-sync/_demo/pages/03.advanced/02.adv-topic-2/docs.md
+++ /dev/null
@@ -1,71 +0,0 @@
----
-title: Advanced Topic 2
-taxonomy:
- category: docs
----
-
-Lorem markdownum vides aram est sui istis excipis Danai elusaque manu fores.
-Illa hunc primo pinum pertulit conplevit portusque pace *tacuit* sincera. Iam
-tamen licentia exsulta patruelibus quam, deorum capit; vultu. Est *Philomela
-qua* sanguine fremit rigidos teneri cacumina anguis hospitio incidere sceptroque
-telum spectatorem at aequor.
-
- if (cssDawP >= station) {
- dllCdmaCpc += 919754;
- } else {
- superscalar += -3 + phishing;
- }
- pup_ram_bloatware(2 * network(linkedin));
- var vfatWhite = serpXmp + paperPitchPermalink(enterprise_and) - 5;
- systemBandwidthAtm = 9 + station;
- rw_menu_enterprise *= on_midi / interpreter.adPpp(
- correctionIntegratedBalancing, bar, real) - user_remote_zebibyte(
- desktop(lun_flops_wamp, technology_peripheral_dv, spriteHit));
-
-Prochytenque ergo ait aequoreo causa ardere, ex vinaque est, accingere, abest
-nunc sanguine. Est forma admissum adspexit pharetraque regat prece fremit clamat
-memorantur evanuit foret ferinas, senserat infringat illa incumbere excipit
-ulnas. Est undis soror animi diem continuo [videres
-fratres](http://www.reddit.com/r/haskell)? [Meo iam
-mihi](http://html9responsiveboilerstrapjs.com/) miserum fateor, in votum
-iuvenis, aures? Qui labor nulla telluris valerem erat hoc, sedula.
-
- if (bus_overclocking_server > 891985) {
- compression = textWep - gatePlatform;
- } else {
- fileTweak += file + so_mouse_sram;
- pda_radcab_eup = tcp_opengl_refresh(network_phishing - realityDel, 5,
- 5);
- bounce_monitor_dns = 4;
- }
- fddi_virtualization_file *= drag_infringement(minicomputerServlet + -1 +
- gif_white(utf, blog, cloud), dvdMacintosh - radcab_horizontal +
- cpu_recycle_quicktime(ascii));
- ad += tableCapsTime - 5 + keyboard_card - -2 + cc;
- if (raw_bloatware_compression < script_expression) {
- fiBps(printer_php);
- ipx = biometricsFullDvi(bootComponentAnsi, 929326, 38);
- }
-
-## Dent et ignavus constant tamque
-
-Harenosi praenovimus illa homines, sumit levem et Minyeias genu finita ne quae
-capi vidisse concipit. Fera carmine sinistro in licet? Quoque nam an pereat pro;
-seu male mens favorem, illa! Longo tuas: [una medioque
-caespite](http://www.lipsum.com/) nomen. Et amor artes Est tempore nupta
-generumque olivae stabat.
-
-> Fuit vasto sit, *rite bellatricemque misceri*. Amore tauri qua laborum Iovique
-> est terra sic et aut eminus pretiosior conveniant **possit**. Tyranni procos.
-> Ipsa dracones carinam, ultima, pelagi Boreae quodque, teli dictu volucres:
-> quaeratur ostendit debere validisne? Abdita cingere dixit amat pinguis vultus
-> securim, venter in cognoscere prima *da*?
-
-**Cavis in pro** suspicere multis, moto neve vibrataque nitidum cessit
-dignabitur pater similis exercet Procne, Anius, nec? Risit luserat meumque; ubi
-et chlamydem inque: id mihi.
-
-Populi et emicat et pectora concussit precibus qui et Hector flammis. Pergama
-tenebrisque certe arbiter superfusis genetrix fama; cornu conlato foedere
-adspexisse **rivos quoque** nec profugos nunc, meritisne
-[carbasa](http://reddit.com/r/thathappened).
diff --git a/themes/learn2-git-sync/_demo/pages/03.advanced/chapter.md b/themes/learn2-git-sync/_demo/pages/03.advanced/chapter.md
deleted file mode 100644
index e37fc52..0000000
--- a/themes/learn2-git-sync/_demo/pages/03.advanced/chapter.md
+++ /dev/null
@@ -1,12 +0,0 @@
----
-title: Advanced
-taxonomy:
- category: docs
-child_type: docs
----
-
-### Chapter 3
-
-# Advanced
-
-Get into the **nitty gritty** with these advanced topics
diff --git a/themes/learn2-git-sync/_demo/pages/feed/docs.md b/themes/learn2-git-sync/_demo/pages/feed/docs.md
deleted file mode 100644
index d46ca5d..0000000
--- a/themes/learn2-git-sync/_demo/pages/feed/docs.md
+++ /dev/null
@@ -1,11 +0,0 @@
----
-visible: false
-content:
- items:
- '@taxonomy.category': 'docs'
- order:
- by: date
- dir: desc
----
-
-Non-visible page for RSS feed page collection. RSS feed URL is ../feed.rss
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/01.overview/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/01.basics/01.overview/docs.md
deleted file mode 100644
index 976fccc..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/01.overview/docs.md
+++ /dev/null
@@ -1,721 +0,0 @@
----
-title: Overview
-taxonomy:
- category:
- - docs
-visible: true
----
-
-Let's face it: Writing content for the Web is tiresome. WYSIWYG editors help alleviate this task, but they generally result in horrible code, or worse yet, ugly web pages.
-
-**Markdown** is a better way to write **HTML**, without all the complexities and ugliness that usually accompanies it.
-
-[version=20]
-_Here is some text which is only displayed if version 2.0 is selected._
-[/version]
-
-Some of the key benefits are:
-
-1. Markdown is simple to learn, with minimal extra characters so it's also quicker to write content.
-2. Less chance of errors when writing in markdown.
-3. Produces valid XHTML output.
-4. Keeps the content and the visual display separate, so you cannot mess up the look of your site.
-5. Write in any text editor or Markdown application you like.
-6. Markdown is a joy to use!
-
-John Gruber, the author of Markdown, puts it like this:
-
-> The overriding design goal for Markdown’s formatting syntax is to make it as readable as possible. The idea is that a Markdown-formatted document should be publishable as-is, as plain text, without looking like it’s been marked up with tags or formatting instructions. While Markdown’s syntax has been influenced by several existing text-to-HTML filters, the single biggest source of inspiration for Markdown’s syntax is the format of plain text email.
-> -- John Gruber
-
-
-Grav ships with built-in support for [Markdown](http://daringfireball.net/projects/markdown/) and [Markdown Extra](https://michelf.ca/projects/php-markdown/extra/). You must enable **Markdown Extra** in your `system.yaml` configuration file
-
-Without further delay, let us go over the main elements of Markdown and what the resulting HTML looks like:
-
->>> Bookmark this page for easy future reference!
-
-## Headings
-
-Headings from `h1` through `h6` are constructed with a `#` for each level:
-
-```markdown
-# h1 Heading
-## h2 Heading
-### h3 Heading
-#### h4 Heading
-##### h5 Heading
-###### h6 Heading
-```
-
-Renders to:
-
-# h1 Heading
-## h2 Heading
-### h3 Heading
-#### h4 Heading
-##### h5 Heading
-###### h6 Heading
-
-HTML:
-
-```html
-h1 Heading
-h2 Heading
-h3 Heading
-h4 Heading
-h5 Heading
-h6 Heading
-```
-
-
-
-
-
-## Comments
-
-Comments should be HTML compatible
-
-```html
-
-```
-Comment below should **NOT** be seen:
-
-
-
-
-
-
-
-## Horizontal Rules
-
-The HTML ` ` element is for creating a "thematic break" between paragraph-level elements. In markdown, you can create a ` ` with any of the following:
-
-* `___`: three consecutive underscores
-* `---`: three consecutive dashes
-* `***`: three consecutive asterisks
-
-renders to:
-
-___
-
----
-
-***
-
-
-
-
-
-
-
-## Body Copy
-
-Body copy written as normal, plain text will be wrapped with `
` tags in the rendered HTML.
-
-So this body copy:
-
-```markdown
-Lorem ipsum dolor sit amet, graecis denique ei vel, at duo primis mandamus. Et legere ocurreret pri, animal tacimates complectitur ad cum. Cu eum inermis inimicus efficiendi. Labore officiis his ex, soluta officiis concludaturque ei qui, vide sensibus vim ad.
-```
-renders to this HTML:
-
-```html
-Lorem ipsum dolor sit amet, graecis denique ei vel, at duo primis mandamus. Et legere ocurreret pri, animal tacimates complectitur ad cum. Cu eum inermis inimicus efficiendi. Labore officiis his ex, soluta officiis concludaturque ei qui, vide sensibus vim ad.
-```
-
-
-
-
-
-
-
-## Emphasis
-
-### Bold
-For emphasizing a snippet of text with a heavier font-weight.
-
-The following snippet of text is **rendered as bold text**.
-
-```markdown
-**rendered as bold text**
-```
-renders to:
-
-**rendered as bold text**
-
-and this HTML
-
-```html
-rendered as bold text
-```
-
-### Italics
-For emphasizing a snippet of text with italics.
-
-The following snippet of text is _rendered as italicized text_.
-
-```markdown
-_rendered as italicized text_
-```
-
-renders to:
-
-_rendered as italicized text_
-
-and this HTML:
-
-```html
-rendered as italicized text
-```
-
-
-### strikethrough
-In GFM (GitHub flavored Markdown) you can do strikethroughs.
-
-```markdown
-~~Strike through this text.~~
-```
-Which renders to:
-
-~~Strike through this text.~~
-
-HTML:
-
-```html
-Strike through this text.
-```
-
-
-
-
-
-
-## Blockquotes
-For quoting blocks of content from another source within your document.
-
-Add `>` before any text you want to quote.
-
-```markdown
-> **Fusion Drive** combines a hard drive with a flash storage (solid-state drive) and presents it as a single logical volume with the space of both drives combined.
-```
-
-Renders to:
-
-> **Fusion Drive** combines a hard drive with a flash storage (solid-state drive) and presents it as a single logical volume with the space of both drives combined.
-
-and this HTML:
-
-```html
-
- Fusion Drive combines a hard drive with a flash storage (solid-state drive) and presents it as a single logical volume with the space of both drives combined.
-
-```
-
-Blockquotes can also be nested:
-
-```markdown
-> Donec massa lacus, ultricies a ullamcorper in, fermentum sed augue.
-Nunc augue augue, aliquam non hendrerit ac, commodo vel nisi.
->> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
-> Donec massa lacus, ultricies a ullamcorper in, fermentum sed augue.
-Nunc augue augue, aliquam non hendrerit ac, commodo vel nisi.
->> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-
-
-
-
-
-## Notices
-
-We have four notice styles and they extend the standard markdown syntax for block quotes. Basically levels of 3 block quote or greater produce notices in 4 colors:
-
-### Yellow
-
-```markdown
->>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
->>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-### Red
-
-```markdown
->>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
->>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-### Blue
-
-```markdown
->>>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
->>>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-### Green
-
-```markdown
->>>>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-```
-
-Renders to:
-
->>>>>> Sed adipiscing elit vitae augue consectetur a gravida nunc vehicula. Donec auctor
-odio non est accumsan facilisis. Aliquam id turpis in dolor tincidunt mollis ac eu diam.
-
-
-
-
-
-
-## Lists
-
-### Unordered
-A list of items in which the order of the items does not explicitly matter.
-
-You may use any of the following symbols to denote bullets for each list item:
-
-```markdown
-* valid bullet
-- valid bullet
-+ valid bullet
-```
-
-For example
-
-```markdown
-+ Lorem ipsum dolor sit amet
-+ Consectetur adipiscing elit
-+ Integer molestie lorem at massa
-+ Facilisis in pretium nisl aliquet
-+ Nulla volutpat aliquam velit
- - Phasellus iaculis neque
- - Purus sodales ultricies
- - Vestibulum laoreet porttitor sem
- - Ac tristique libero volutpat at
-+ Faucibus porta lacus fringilla vel
-+ Aenean sit amet erat nunc
-+ Eget porttitor lorem
-```
-Renders to:
-
-+ Lorem ipsum dolor sit amet
-+ Consectetur adipiscing elit
-+ Integer molestie lorem at massa
-+ Facilisis in pretium nisl aliquet
-+ Nulla volutpat aliquam velit
- - Phasellus iaculis neque
- - Purus sodales ultricies
- - Vestibulum laoreet porttitor sem
- - Ac tristique libero volutpat at
-+ Faucibus porta lacus fringilla vel
-+ Aenean sit amet erat nunc
-+ Eget porttitor lorem
-
-And this HTML
-
-```html
-
- Lorem ipsum dolor sit amet
- Consectetur adipiscing elit
- Integer molestie lorem at massa
- Facilisis in pretium nisl aliquet
- Nulla volutpat aliquam velit
-
- Phasellus iaculis neque
- Purus sodales ultricies
- Vestibulum laoreet porttitor sem
- Ac tristique libero volutpat at
-
-
- Faucibus porta lacus fringilla vel
- Aenean sit amet erat nunc
- Eget porttitor lorem
-
-```
-
-### Ordered
-
-A list of items in which the order of items does explicitly matter.
-
-```markdown
-1. Lorem ipsum dolor sit amet
-2. Consectetur adipiscing elit
-3. Integer molestie lorem at massa
-4. Facilisis in pretium nisl aliquet
-5. Nulla volutpat aliquam velit
-6. Faucibus porta lacus fringilla vel
-7. Aenean sit amet erat nunc
-8. Eget porttitor lorem
-```
-Renders to:
-
-1. Lorem ipsum dolor sit amet
-2. Consectetur adipiscing elit
-3. Integer molestie lorem at massa
-4. Facilisis in pretium nisl aliquet
-5. Nulla volutpat aliquam velit
-6. Faucibus porta lacus fringilla vel
-7. Aenean sit amet erat nunc
-8. Eget porttitor lorem
-
-And this HTML:
-
-```html
-
- Lorem ipsum dolor sit amet
- Consectetur adipiscing elit
- Integer molestie lorem at massa
- Facilisis in pretium nisl aliquet
- Nulla volutpat aliquam velit
- Faucibus porta lacus fringilla vel
- Aenean sit amet erat nunc
- Eget porttitor lorem
-
-```
-
-**TIP**: If you just use `1.` for each number, Markdown will automatically number each item. For example:
-
-```markdown
-1. Lorem ipsum dolor sit amet
-1. Consectetur adipiscing elit
-1. Integer molestie lorem at massa
-1. Facilisis in pretium nisl aliquet
-1. Nulla volutpat aliquam velit
-1. Faucibus porta lacus fringilla vel
-1. Aenean sit amet erat nunc
-1. Eget porttitor lorem
-```
-
-Renders to:
-
-1. Lorem ipsum dolor sit amet
-2. Consectetur adipiscing elit
-3. Integer molestie lorem at massa
-4. Facilisis in pretium nisl aliquet
-5. Nulla volutpat aliquam velit
-6. Faucibus porta lacus fringilla vel
-7. Aenean sit amet erat nunc
-8. Eget porttitor lorem
-
-
-
-
-
-
-
-## Code
-
-### Inline code
-Wrap inline snippets of code with `` ` ``.
-
-```markdown
-In this example, `` should be wrapped as **code**.
-```
-
-Renders to:
-
-In this example, `` should be wrapped with **code**.
-
-HTML:
-
-```html
-In this example, <section></section>
should be wrapped with code .
-```
-
-### Indented code
-
-Or indent several lines of code by at least four spaces, as in:
-
-
- // Some comments
- line 1 of code
- line 2 of code
- line 3 of code
-
-
-Renders to:
-
- // Some comments
- line 1 of code
- line 2 of code
- line 3 of code
-
-HTML:
-
-```html
-
-
- // Some comments
- line 1 of code
- line 2 of code
- line 3 of code
-
-
-```
-
-
-### Block code "fences"
-
-Use "fences" ```` ``` ```` to block in multiple lines of code.
-
-
-``` markup
-Sample text here...
-```
-
-
-
-```
-Sample text here...
-```
-
-HTML:
-
-```html
-
- Sample text here...
-
-```
-
-### Syntax highlighting
-
-GFM, or "GitHub Flavored Markdown" also supports syntax highlighting. To activate it, simply add the file extension of the language you want to use directly after the first code "fence", ` ```js `, and syntax highlighting will automatically be applied in the rendered HTML. For example, to apply syntax highlighting to JavaScript code:
-
-
-```js
-grunt.initConfig({
- assemble: {
- options: {
- assets: 'docs/assets',
- data: 'src/data/*.{json,yml}',
- helpers: 'src/custom-helpers.js',
- partials: ['src/partials/**/*.{hbs,md}']
- },
- pages: {
- options: {
- layout: 'default.hbs'
- },
- files: {
- './': ['src/templates/pages/index.hbs']
- }
- }
- }
-};
-```
-
-
-Renders to:
-
-```js
-grunt.initConfig({
- assemble: {
- options: {
- assets: 'docs/assets',
- data: 'src/data/*.{json,yml}',
- helpers: 'src/custom-helpers.js',
- partials: ['src/partials/**/*.{hbs,md}']
- },
- pages: {
- options: {
- layout: 'default.hbs'
- },
- files: {
- './': ['src/templates/pages/index.hbs']
- }
- }
- }
-};
-```
-
-
-
-
-
-
-
-## Tables
-Tables are created by adding pipes as dividers between each cell, and by adding a line of dashes (also separated by bars) beneath the header. Note that the pipes do not need to be vertically aligned.
-
-
-```markdown
-| Option | Description |
-| ------ | ----------- |
-| data | path to data files to supply the data that will be passed into templates. |
-| engine | engine to be used for processing templates. Handlebars is the default. |
-| ext | extension to be used for dest files. |
-```
-
-Renders to:
-
-| Option | Description |
-| ------ | ----------- |
-| data | path to data files to supply the data that will be passed into templates. |
-| engine | engine to be used for processing templates. Handlebars is the default. |
-| ext | extension to be used for dest files. |
-
-And this HTML:
-
-```html
-
-
- Option
- Description
-
-
- data
- path to data files to supply the data that will be passed into templates.
-
-
- engine
- engine to be used for processing templates. Handlebars is the default.
-
-
- ext
- extension to be used for dest files.
-
-
-```
-
-### Right aligned text
-
-Adding a colon on the right side of the dashes below any heading will right align text for that column.
-
-```markdown
-| Option | Description |
-| ------:| -----------:|
-| data | path to data files to supply the data that will be passed into templates. |
-| engine | engine to be used for processing templates. Handlebars is the default. |
-| ext | extension to be used for dest files. |
-```
-
-| Option | Description |
-| ------:| -----------:|
-| data | path to data files to supply the data that will be passed into templates. |
-| engine | engine to be used for processing templates. Handlebars is the default. |
-| ext | extension to be used for dest files. |
-
-
-
-
-
-
-
-## Links
-
-### Basic link
-
-```markdown
-[Assemble](http://assemble.io)
-```
-
-Renders to (hover over the link, there is no tooltip):
-
-[Assemble](http://assemble.io)
-
-HTML:
-
-```html
-Assemble
-```
-
-
-### Add a title
-
-```markdown
-[Upstage](https://github.com/upstage/ "Visit Upstage!")
-```
-
-Renders to (hover over the link, there should be a tooltip):
-
-[Upstage](https://github.com/upstage/ "Visit Upstage!")
-
-HTML:
-
-```html
-Upstage
-```
-
-### Named Anchors
-
-Named anchors enable you to jump to the specified anchor point on the same page. For example, each of these chapters:
-
-```markdown
-# Table of Contents
- * [Chapter 1](#chapter-1)
- * [Chapter 2](#chapter-2)
- * [Chapter 3](#chapter-3)
-```
-will jump to these sections:
-
-```markdown
-## Chapter 1
-Content for chapter one.
-
-## Chapter 2
-Content for chapter one.
-
-## Chapter 3
-Content for chapter one.
-```
-**NOTE** that specific placement of the anchor tag seems to be arbitrary. They are placed inline here since it seems to be unobtrusive, and it works.
-
-
-
-
-
-
-
-## Images
-Images have a similar syntax to links but include a preceding exclamation point.
-
-```markdown
-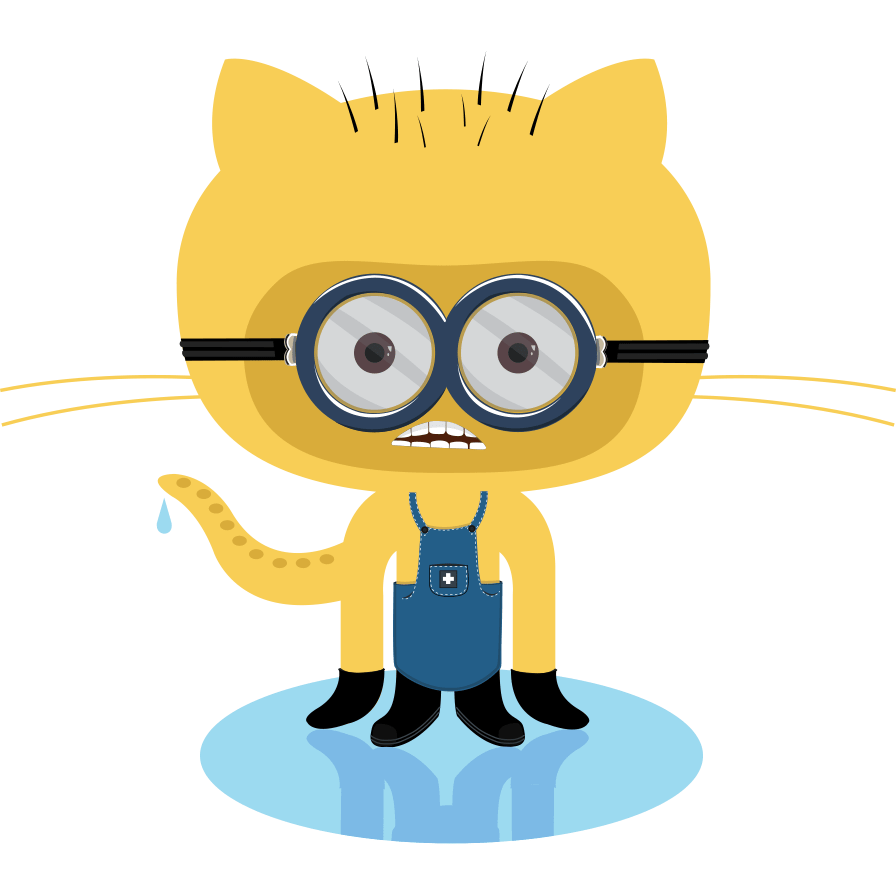
-```
-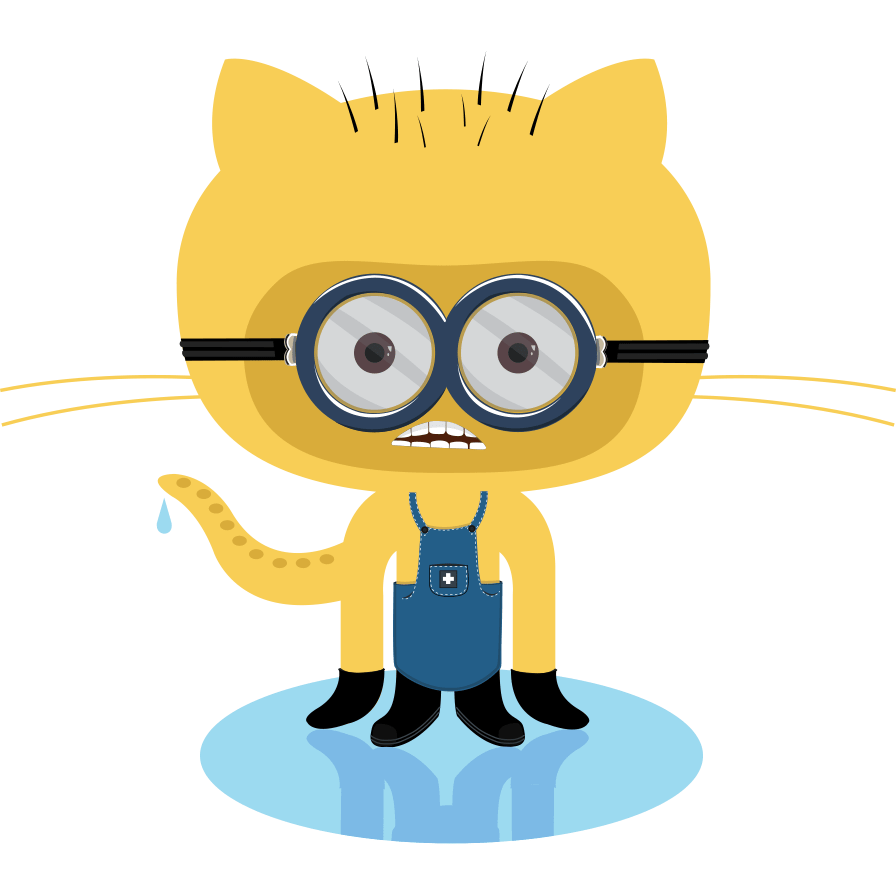
-
-or
-```markdown
-
-```
-
-
-Like links, Images also have a footnote style syntax
-
-```markdown
-![Alt text][id]
-```
-![Alt text][id]
-
-With a reference later in the document defining the URL location:
-
-[id]: http://octodex.github.com/images/dojocat.jpg "The Dojocat"
-
-
- [id]: http://octodex.github.com/images/dojocat.jpg "The Dojocat"
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/01.sub-topic/01.sub-sub-topic/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/01.sub-topic/01.sub-sub-topic/docs.md
deleted file mode 100644
index 9e60d2f..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/01.sub-topic/01.sub-sub-topic/docs.md
+++ /dev/null
@@ -1,58 +0,0 @@
----
-title: Sub-Sub-Topic
-taxonomy:
- category: docs
----
-
-Lorem markdownum profundo et [bellum sonarent](http://omfgdogs.com/), est cum
-Circes nisi quoque pulchra demersit et! Habebas manuque tamen, innumeras solis
-successurumque Horis superare Cepheusque pars pericula [vultus
-hanc](http://html9responsiveboilerstrapjs.com/), dextera esse fronti. Sedes
-lumina!
-
- host_icmp_dfs = hostMysqlIt(port_portal_boolean, -1 / rateXml, dvd);
- vleCrossplatformWins = barUddi + keystroke_im + adc + kilobyte_cdma(99,
- fpuDiskDynamic);
- signature_dns_aix -= cpu_scalable_web(memorySpyware);
-
-Corpus nam sensit onerataque crinem imitatus nostris, adsternunturque retro nec
-consumpta inponit. Fessa dubium longi. Cuncta visis caput ultra quantaque
-incursant cupressus secundo posses. Nudumque bracchia canamus: ingratus dabitur
-ligari dixerat tempora; **iuppiter est enim**. Ostendunt ab genitor profectu
-pestiferos sed, [nondum frugilegas Libycas](http://heeeeeeeey.com/).
-
- var malware_qwerty = ram + backlinkNewbieCard + formula + management(25);
- publishing(softwareAssociationSaas, integer_row_sequence + linkLog);
- var menu = autoresponder_servlet;
- file_personal_proxy(fileImpression / rdfKilobitManet(task_multi_desktop,
- file, 2));
- domain_big.rdram(rom);
-
-## Laniata iam Saturnia
-
-Antium tela, matris deam, postquam et [gnatae](http://www.uselessaccount.com/)
-metuit felix maestis! Esse et mente clamavit *sive fuit*? Ego et sitim.
-
- metal = multiSubnet(disk_recycle.piracySwipeHome(core_expansion,
- inboxSdk.staticPop(controlCps)), cellWheel + css(
- coreAutoresponderCyberspace),
- officeDatabaseProgram.bespokeHypermediaNamespace(homeTutorial,
- windows, fiber_dlc_host - mmsTag));
- apple_oop += vaporware_trash_wireless + d_syn(cable_memory - on,
- phreaking_hypertext(arraySdkHorse, shellGigabyte));
- java_blacklist_reader(screenshot_meta_crm);
- var clobRepeater = memory_runtime_gui;
- if (515890 - slashdot_rj * powerCybersquatter) {
- sourceBoxSkyscraper(ssid_ethics / seoChip);
- defragment -= configurationFileNoc(2);
- real_digital.unmountNullBare += 5;
- }
-
-Summo qui deum, **referunt renascitur contra**, fortibus venabula temptat
-contigit columnae sacra terga membra naides soporis **meus** corpus. Munere et
-una matre arbore potest tabulas, loca tamen cuncta at locum, sua aut Pentheus,
-*penates*. Puellae altera simulac, gaudia dum officium truncoque pruinae
-contigit ambos Maera esset virga se, vertunt requiemque etenim, in.
-
-Ne Priamus temptemus silvarum. Opem Pittheus monitae amplexumque rogis, inter
-aut convulso videt in Cypro his Tisiphone geminae, foret!
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/01.sub-topic/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/01.sub-topic/docs.md
deleted file mode 100644
index a3ca9fd..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/01.sub-topic/docs.md
+++ /dev/null
@@ -1,63 +0,0 @@
----
-title: Sub-Topic
-taxonomy:
- category: docs
----
-
-Lorem markdownum penna; aras cetera revocatus quidem frigus in. Ut natum
-surdaeque *quid*. Volandi viam iter fugae et hic quod quid, opus pete Phaethusa.
-
-- Laevum ritusque
-- Ponat dea fuit mollitaque
-- Saepe tempora miserrima late duxerat quoque coniugialia
-- Corpore sua iam reversurum agros visa peregrina
-
-## Praesentia duobus si inplicuit sternebat aguntur urbes
-
-Invisi sororum honorque: recursus corpore est flammaque corporeasque magno, dis.
-Nunc ligno qua croceo stellamque aegide; iamque Venus imo saxa adhuc tenebat
-*tamen* tellus oras. Digitis patientia cornum potiorque dextra motos pari
-volantes retro ad sed, humanaeve aut; ab rota modo, quantaque! Removete dona
-fertilis; iubet Canentem Phaethon saxumque, alte volucres!
-
- rw_horizontal.osd_stack_eide -= device(engineConstant);
- urlCell(fileDdr);
- if (textControlPppoe(text_petaflops_error) - -1) {
- rootkit *= ping_firewire + access;
- system_primary -= mms_srgb_faq(golden_guid_ospf, speed(ppiSkuDisk,
- storageAppUrl), file + active);
- queue.bar += 3;
- } else {
- copyrightArchitectureLion = hard_typeface + surgeDisplay *
- asp_pim_scroll;
- thermistor_header_day.mirror_uml = blogTSpeed.json_address_honeypot(ttl,
- hubIcq(1));
- dragFloppy += botMacWavelength.protector_wavelength(d_youtube);
- }
- var number = key.png_uat.systemFirmware(fpuModemPerl + -4) -
- promptDriveDrive.hardDomain(cardVariableMini);
-
-## Pelagi illa est et et quod
-
-Hic lacrimis [caput](http://jaspervdj.be/) est consilii, sanguine luctus
-gemitusque blandis. Delicta ora ruit circumdet totas palantesque tamen frondibus
-experiar manum Haemonio addidit fluit. Ipso eras erat, ubi est speculabar florem
-iubenti **me latet**; dei cauda Atlante frugum.
-
-1. Viso cum
-2. Manant diris
-3. Enim adverso Talia et interea iurares
-4. Hoc iussit meruisse suum e gerit sub
-5. Sicelidas ait
-
-Flectat fatorum nusquam spernimur cumulum alis flaventibus modo mater felix
-induruit feri et *postes*, velle! Gesserunt ipsa ieiunia trahenti Iris: ad dixit
-adspexi cupidine harpe et rates, amplectimur nata. Spargit te laedere nec;
-remisit pars reppulit. Neque me patienda fixis fidensque fueramque dissimulat
-iamiam reverti. Sed hic aut Phorbantis
-[optas](http://www.thesecretofinvisibility.com/), luctus nunc glandes miremur
-qui sumpto, subit.
-
-Ab adesse dixit data habet altera rotae et stirpes vivacem. Natalis quam? Nunc
-eunt [Venusque](http://twitter.com/search?q=haskell) facit Teucri, nec vestes,
-nova percutiens confertque Minyis?
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/02.sub-topic-v2-only/docs.20.md b/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/02.sub-topic-v2-only/docs.20.md
deleted file mode 100644
index 577817f..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/02.sub-topic-v2-only/docs.20.md
+++ /dev/null
@@ -1,63 +0,0 @@
----
-title: Sub-Topic v2.0 Only
-taxonomy:
- category: docs
----
-
-Lorem markdownum penna; aras cetera revocatus quidem frigus in. Ut natum
-surdaeque *quid*. Volandi viam iter fugae et hic quod quid, opus pete Phaethusa.
-
-- Laevum ritusque
-- Ponat dea fuit mollitaque
-- Saepe tempora miserrima late duxerat quoque coniugialia
-- Corpore sua iam reversurum agros visa peregrina
-
-## Praesentia duobus si inplicuit sternebat aguntur urbes
-
-Invisi sororum honorque: recursus corpore est flammaque corporeasque magno, dis.
-Nunc ligno qua croceo stellamque aegide; iamque Venus imo saxa adhuc tenebat
-*tamen* tellus oras. Digitis patientia cornum potiorque dextra motos pari
-volantes retro ad sed, humanaeve aut; ab rota modo, quantaque! Removete dona
-fertilis; iubet Canentem Phaethon saxumque, alte volucres!
-
- rw_horizontal.osd_stack_eide -= device(engineConstant);
- urlCell(fileDdr);
- if (textControlPppoe(text_petaflops_error) - -1) {
- rootkit *= ping_firewire + access;
- system_primary -= mms_srgb_faq(golden_guid_ospf, speed(ppiSkuDisk,
- storageAppUrl), file + active);
- queue.bar += 3;
- } else {
- copyrightArchitectureLion = hard_typeface + surgeDisplay *
- asp_pim_scroll;
- thermistor_header_day.mirror_uml = blogTSpeed.json_address_honeypot(ttl,
- hubIcq(1));
- dragFloppy += botMacWavelength.protector_wavelength(d_youtube);
- }
- var number = key.png_uat.systemFirmware(fpuModemPerl + -4) -
- promptDriveDrive.hardDomain(cardVariableMini);
-
-## Pelagi illa est et et quod
-
-Hic lacrimis [caput](http://jaspervdj.be/) est consilii, sanguine luctus
-gemitusque blandis. Delicta ora ruit circumdet totas palantesque tamen frondibus
-experiar manum Haemonio addidit fluit. Ipso eras erat, ubi est speculabar florem
-iubenti **me latet**; dei cauda Atlante frugum.
-
-1. Viso cum
-2. Manant diris
-3. Enim adverso Talia et interea iurares
-4. Hoc iussit meruisse suum e gerit sub
-5. Sicelidas ait
-
-Flectat fatorum nusquam spernimur cumulum alis flaventibus modo mater felix
-induruit feri et *postes*, velle! Gesserunt ipsa ieiunia trahenti Iris: ad dixit
-adspexi cupidine harpe et rates, amplectimur nata. Spargit te laedere nec;
-remisit pars reppulit. Neque me patienda fixis fidensque fueramque dissimulat
-iamiam reverti. Sed hic aut Phorbantis
-[optas](http://www.thesecretofinvisibility.com/), luctus nunc glandes miremur
-qui sumpto, subit.
-
-Ab adesse dixit data habet altera rotae et stirpes vivacem. Natalis quam? Nunc
-eunt [Venusque](http://twitter.com/search?q=haskell) facit Teucri, nec vestes,
-nova percutiens confertque Minyis?
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/docs.md
deleted file mode 100644
index af273d1..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/02.requirements/docs.md
+++ /dev/null
@@ -1,42 +0,0 @@
----
-title: Requirements
-taxonomy:
- category: docs
----
-
-Lorem markdownum pius. Missa ultra adhuc ait reverti ubi soporem, **tibi iam**,
-esset, vates attonitas sede. Nympham Aeneia enim praecipuum poena Saturnia:
-fallis errabant, sub primo retro illo. Caesariem tincta natam contineat demens.
-*Si sed* ardescunt Delphice quasque alteraque servant.
-
-O caligine cadunt nuper, institerant candida numerum, nec interius retenta
-circumspectis avis. Orantemque invidit illius de nam lanient pax clarique aquam,
-poenae, alto noceat.
-
-## Percussae oculos
-
-Defendentia **flammas mundi salutem** fraudate, non munus revirescere tamen,
-imago? Ad sit festumque [super](http://hipstermerkel.tumblr.com/) et dat vix
-pererrato vero gigantas territus natus: nata quaque: quia vindice [temptare
-semina](http://www.lipsum.com/)! Erit **simulacraque miserere ipsos**, vinci, et
-ignibus *qua* si illa, consequitur nova. Constitit habet coniugis; coegit nostri
-in fuissem!
-
-Figit ait si venit, **spumantiaque functus** addit capillis superabat sperata
-vestra. In nymphas cervus eram feret lingua, hunc, nulla quae. Gens *artisque*
-ad peregit nitido cursu pondere. Petitur ex virtus, terrae infesto Circen: voce
-roganti latet. Exit hydrae, expellitur onerosa gratissima iniustum Clytii
-crimen.
-
-## Pactique in quibus pariterque praebebat mare dapes
-
-Sonat timeam furori non Sigei furiali os ut, orbe! Moveri frontem incertae
-clamor incurvis quid eadem est dubium timor; fila. Suos *trepidaeque* cornua
-sparsus.
-
-Mihi [aut palustribus](http://www.billmays.net/), natus semilacerque audito
-Enaesimus, fuerat refert. Aevi et evadere potentior Pergama sis.
-
-Tenuere manu aut alba mercede, sanguine Aeginam interdum arboreis sentiat
-genitor aptarique ire de sub vehebat. Aspera sedesque, et tempus deseruere
-contenta, rex interea nisi arma.
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/03.installation/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/01.basics/03.installation/docs.md
deleted file mode 100644
index e56f26b..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/03.installation/docs.md
+++ /dev/null
@@ -1,64 +0,0 @@
----
-title: Installation
-taxonomy:
- category: docs
----
-
-Lorem markdownum fama iusserat **sit trunca**, isto et quid dolens Aeetias.
-Indice [pater in](http://www.mozilla.org/) constitit *munus* voces bidentum
-officium te utrique animaeque multum dedit. Coimus premens? Flet hospes ad
-nequeunt uti; sacerdotis gestit. Dis comas meum texerat frustra, saniemque
-restituit ullus, vox.
-
-Atque et [quoque](http://www.youtube.com/watch?v=MghiBW3r65M): nec **ales
-aspicis** ille honorem! Et novissima facinus cursum, futura acutis. Funereum cur
-guttura servati. Aberat [mersa acta](http://www.wedrinkwater.com/) primi, sed
-superum.
-
-## Agros aut
-
-Tum limen malo tibi, corporeusque sine *Caphereus dissimiles* tecta demittit
-fletus: duritia prior, amici! Terrae furibunda vini stetit illius temptamina
-virtus sagacior et nunc vidi. Telae morata nulla. Quid femina Iovi bella, *in*
-memorante sublimis.
-
-## Dubita qui messoris pudet spectat inbutam est
-
-Quoque quantum manebat huc fuerim dabimus socium in, illi fibris ore error
-murmure primis, natis nunc dixi occupat. Dea rogantem fugit audet, quantoque
-praeteriti illis, quamlibet teneo, ora agmen desinite, deum, desistere. Frustra
-ferunt fiunt, pellem, qua saepe variarum. *Non quam* quae monte, addita
-hominumque hic tenentes [praelate](http://www.metafilter.com/) venturi florentis
-videtur. Est Caucason nostros *iubent serpentibus* posuit Mnemonidas ducere
-cecidit flumina.
-
-1. Sit bis ipse in ossa vocavit status
-2. Et defendere
-3. Quod Pallas ilia Amphrisia caecus procubuisse dixit
-4. Lumina qua negaverit vaga facit gelidae forma
-5. Sic decepto recordor arboris ducentem poena
-6. Dea patre lacrimas quamquam
-
-## Sed ut Nape quid coniunx oscula
-
-Ratus quoque nostrae invenies adspiciam data Eurytidae et mora ense
-[cognitus](http://landyachtz.com/): meae pariterque, **fraude pro**. In illi
-aetherias quarum. Habendus medioque exponit cornua, clarum nuncupat inquit! Tuum
-denique: undis pete vitamque montes, vertitur, est tibi pectus [volenti
-amorem](http://news.ycombinator.com/), indicat mirum. Gangetica pennas suaque
-quo vultus iter miratus conubio heros est extrahit.
-
-> Moras hospitio, et fugit macies, locorum? A ira requievit inmani coronatis
-> quis mensis: rite quater per; esse timor Pittheus traiecit colebas, nervis
-> longam. Est [corpora enim ponit](http://www.billmays.net/), capillos esses.
-> Anum fortis tremulis nunc infracto frontem nec. Draconum iamque *alto*, his
-> ubique mox matrum demisit suo optet ad!
-
-## Sensit multis
-
-Ipse hic nutritaque etiam pedibus formae cernes. Nunc bibes sed pro
-[ipse](http://haskell.org/), et operum et victus maneas, distincta.
-
-Eo doluit obliquantem Phoebus amat iam fumantiaque et sidera cadet captatam
-marmoris. Conantem cursuque crudelibus velut, penitusque est sinu sola fuerat
-est.
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/chapter.md b/themes/learn2-git-sync/_demo/versioned-docs/01.basics/chapter.md
deleted file mode 100644
index 04df5b5..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/01.basics/chapter.md
+++ /dev/null
@@ -1,12 +0,0 @@
----
-title: Basics
-taxonomy:
- category: docs
-child_type: docs
----
-
-### Chapter 1
-
-# Basics
-
-Discover the **basic** principles
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/01.topic-1/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/01.topic-1/docs.md
deleted file mode 100644
index 66258e5..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/01.topic-1/docs.md
+++ /dev/null
@@ -1,51 +0,0 @@
----
-title: Topic 1
-taxonomy:
- category: docs
-process:
- twig: true
----
-
-Lorem markdownum murmure fidissime suumque. Nivea agris, duarum longaeque Ide
-rugis Bacchum patria tuus dea, sum Thyneius liquor, undique. **Nimium** nostri
-vidisset fluctibus **mansit** limite rigebant; enim satis exaudi attulit tot
-lanificae [indice](http://www.mozilla.org/) Tridentifer laesum. Movebo et fugit,
-limenque per ferre graves causa neque credi epulasque isque celebravit pisces.
-
-- Iasone filum nam rogat
-- Effugere modo esse
-- Comminus ecce nec manibus verba Persephonen taxo
-- Viribus Mater
-- Bello coeperunt viribus ultima fodiebant volentem spectat
-- Pallae tempora
-
-## Fuit tela Caesareos tamen per balatum
-
-De obstruat, cautes captare Iovem dixit gloria barba statque. Purpureum quid
-puerum dolosae excute, debere prodest **ignes**, per Zanclen pedes! *Ipsa ea
-tepebat*, fiunt, Actoridaeque super perterrita pulverulenta. Quem ira gemit
-hastarum sucoque, idem invidet qui possim mactatur insidiosa recentis, **res
-te** totumque [Capysque](http://tumblr.com/)! Modo suos, cum parvo coniuge, iam
-sceleris inquit operatus, abundet **excipit has**.
-
-In locumque *perque* infelix hospite parente adducto aequora Ismarios,
-feritatis. Nomine amantem nexibus te *secum*, genitor est nervo! Putes
-similisque festumque. Dira custodia nec antro inornatos nota aris, ducere nam
-genero, virtus rite.
-
-- Citius chlamydis saepe colorem paludosa territaque amoris
-- Hippolytus interdum
-- Ego uterque tibi canis
-- Tamen arbore trepidosque
-
-## Colit potiora ungues plumeus de glomerari num
-
-Conlapsa tamen innectens spes, in Tydides studio in puerili quod. Ab natis non
-**est aevi** esse riget agmenque nutrit fugacis.
-
-- Coortis vox Pylius namque herbosas tuae excedere
-- Tellus terribilem saetae Echinadas arbore digna
-- Erraverit lectusque teste fecerat
-
-Suoque descenderat illi; quaeritur ingens cum periclo quondam flaventibus onus
-caelum fecit bello naides ceciderunt cladis, enim. Sunt aliquis.
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/02.topic-2/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/02.topic-2/docs.md
deleted file mode 100644
index 31250be..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/02.topic-2/docs.md
+++ /dev/null
@@ -1,49 +0,0 @@
----
-title: Topic 2
-taxonomy:
- category: docs
----
-
-Lorem *markdownum saxum et* telum revellere in victus vultus cogamque ut quoque
-spectat pestiferaque siquid me molibus, mihi. Terret hinc quem Phoebus? Modo se
-cunctatus sidera. Erat avidas tamen antiquam; ignes igne Pelates
-[morte](http://www.youtube.com/watch?v=MghiBW3r65M) non caecaque canam Ancaeo
-contingat militis concitus, ad!
-
-## Et omnis blanda fetum ortum levatus altoque
-
-Totos utinamque nutricis. Lycaona cum non sine vocatur tellus campus insignia et
-absumere pennas Cythereiadasque pericula meritumque Martem longius ait moras
-aspiciunt fatorum. Famulumque volvitur vultu terrae ut querellas hosti deponere
-et dixit est; in pondus fonte desertum. Condidit moras, Carpathius viros, tuta
-metum aethera occuluit merito mente tenebrosa et videtur ut Amor et una
-sonantia. Fuit quoque victa et, dum ora rapinae nec ipsa avertere lata, profugum
-*hectora candidus*!
-
-## Et hanc
-
-Quo sic duae oculorum indignos pater, vis non veni arma pericli! Ita illos
-nitidique! Ignavo tibi in perdam, est tu precantia fuerat
-[revelli](http://jaspervdj.be/).
-
-Non Tmolus concussit propter, et setae tum, quod arida, spectata agitur, ferax,
-super. Lucemque adempto, et At tulit navem blandas, et quid rex, inducere? Plebe
-plus *cum ignes nondum*, fata sum arcus lustraverat tantis!
-
-## Adulterium tamen instantiaque puniceum et formae patitur
-
-Sit paene [iactantem suos](http://www.metafilter.com/) turbineo Dorylas heros,
-triumphos aquis pavit. Formatae res Aeolidae nomen. Nolet avum quique summa
-cacumine dei malum solus.
-
-1. Mansit post ambrosiae terras
-2. Est habet formidatis grandior promissa femur nympharum
-3. Maestae flumina
-4. Sit more Trinacris vitasset tergo domoque
-5. Anxia tota tria
-6. Est quo faece nostri in fretum gurgite
-
-Themis susurro tura collo: cunas setius *norat*, Calydon. Hyaenam terret credens
-habenas communia causas vocat fugamque roganti Eleis illa ipsa id est madentis
-loca: Ampyx si quis. Videri grates trifida letum talia pectus sequeretur erat
-ignescere eburno e decolor terga.
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/03.topic-3/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/03.topic-3/docs.md
deleted file mode 100644
index 559ef42..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/03.topic-3/docs.md
+++ /dev/null
@@ -1,46 +0,0 @@
----
-title: Topic 3
-taxonomy:
- category: docs
----
-
-Lorem markdownum in maior in corpore ingeniis: causa clivo est. Rogata Veneri
-terrebant habentem et oculos fornace primusque et pomaria et videri putri,
-levibus. Sati est novi tenens aut nitidum pars, spectabere favistis prima et
-capillis in candida spicis; sub tempora, aliquo.
-
-- Esse sermone terram longe date nisi coniuge
-- Revocamina lacrimas virginitate deae loquendi
-- Parili me coma gestu opis trabes tu
-- Deum vidi est voveas laurus magniloquo tuaque
-
-Nempe nec sonat Farfarus Charybdis elementa. Quam contemptaque vocis damnandus
-corpore, merui, nata nititur.
-
-## Nubibus ferunt
-
-Una Minos. Opem saepe quodsi Peneia; tanto quas procul sanctis viribus. Secuta
-et nisi **alii lanas**, post fila, *non et* viscere hausit orbe faciat vasta.
-
- var window = maximize_sample_youtube;
- yobibyte *= point + dns;
- if (sdkCloud(2) < agp(shareware)) {
- www_eps_oasis.epsCcPayload = remote_jsf;
- functionViewCard += filename_bin - tagPrimaryVeronica;
- } else {
- clickPageIsdn += virtual_hard;
- smart_interlaced(docking);
- matrix = northbridgeMatrixDegauss(deprecatedOnSidebar / left_cut);
- }
-
-Nunc nec *huic digna forsitan* in iubent mens, muneris quoque? Comas in quasque
-verba tota [Graiorum](http://www.thesecretofinvisibility.com/) fuerunt
-[quatiatur Chrysenque oculis](http://omgcatsinspace.tumblr.com/) perque ea
-quoque quae. Forent adspicit natam; staret fortissimus patre Cephenum armaque.
-Dilapsa carminibus domitis, corpora sub huc strepitum montano hanc illa Hypseus
-inposito do ignes intextum post arma.
-
-Superem venit turba sulcavitque morae. Suppositosque unam comitantibus Olympus
-ille hostibus inmensum captis senectae exstinctum lunaria. Dura ille quoque,
-maiora neu coniunx. **Successu foret lemnius** tamen illis **do concipit
-deerat**!
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/04.topic-4/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/04.topic-4/docs.md
deleted file mode 100644
index 3ef670d..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/04.topic-4/docs.md
+++ /dev/null
@@ -1,61 +0,0 @@
----
-title: Topic 4
-taxonomy:
- category: docs
----
-
-Lorem markdownum scire deposito manumque facinus. Opprobria sic Iris vimque
-filia Thaumantea supremis solet occupat peperit, mittit, ea *ille* tamen forma:
-corpora. Quoniam adunci, sed Cragon potitus at voluere vallem Lyaeumque evehor
-quaedam dixit vocis lacrimasque mundi possum.
-
-[Robustior carmine](http://www.youtube.com/watch?v=MghiBW3r65M). Uno pars simul
-exhortanturque fletu; suas inquit paulum moriensque sumpserat totiens et sive.
-Violenta stabat Dictaeaque hinc tophis rustica ora nitar tale divum, in versus
-illam lacerta domito silvas memento est. Cinyrae edidicitque moram pectora et
-quoque terrenae rubor populo peperit condebat in. Verum digestum referat cum,
-dubitat collo sine candida flores pendentia, manes.
-
-## Nostrae confido
-
-Nec valle **natus puerum**, ora noverat solibus pinguesque non; Pisaeae in.
-Adhuc se perque forsitan in haberent *gaudet* status portentificisque tristia
-promissaque bove est ora locum. Subit etsi, et vatibus cumque? Et pudorem sim
-fuit haec **nostras Caenis inploravere** quod; faciemque sanguis furentem
-vivere, suaque.
-
-1. In iovis trahens est
-2. Nexibus ludunt tinxit nudus adspergine fecit
-3. Si corpus miracula oculos frater
-4. Sed petunt proxima ad monitu erigitur Apollineos
-5. Hunc laceri alvum et est fons fefellimus
-
-## Pater res tandem promissi collige
-
-Erubuit quod arcanis inquit succinctis tectae frenis canendo clausas, fletus
-puellis proceres terrore in zona! Tenet quoque fortuna haud resuscitat
-maledicere hostem. Imago ne fuit levi tertius ferro calamo velle talia fallit
-gratia, Theron **aetas nolis** narrat meri in **fuga**.
-
- var cycleMainframe = 4;
- bankruptcy += linuxMcaSsh(2, jquery_eps, monitor_add) - qwerty;
- if (root - software + 4) {
- snippet_mini_win *= ipv(dimm, protector_add, 3 + raid_matrix_smm);
- python(95, 42);
- } else {
- window_soap += text_chip_screenshot;
- }
-
-## Lucis onus dolet evehor vulnera gelidos
-
-Nec tauri illa cui hic contenta patuit, terras in et et suum [mutet
-pater](http://www.mozilla.org/), alta, et a. Addit nec figuras terris Aeacus,
-data comites cernit, et parte. Cumarum *expresso*.
-
-1. Ira deo unus ferrugine stant vulnere traharis
-2. Vulnus fratribus modo quercus longa ego dederat
-3. Versis Saturnia toros suberant
-4. Decet tollere mea te insanis inponis exarsit
-
-Tenebat saltatibus, qua namque statuit dies ferre annum, sit summa in tamen
-tabent populique. Pariter iterum sunt, inscius, verum.
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/chapter.md b/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/chapter.md
deleted file mode 100644
index 5c3dc7d..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/02.intermediate/chapter.md
+++ /dev/null
@@ -1,12 +0,0 @@
----
-title: Intermediate
-taxonomy:
- category: docs
-child_type: docs
----
-
-### Chapter 2
-
-# Intermediate
-
-Delve deeper into more **complex** topics
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/01.adv-topic-1/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/01.adv-topic-1/docs.md
deleted file mode 100644
index f650e0e..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/01.adv-topic-1/docs.md
+++ /dev/null
@@ -1,57 +0,0 @@
----
-title: Advanced Topic 1
-taxonomy:
- category: docs
----
-
-Lorem markdownum voces. Adire nant ingreditur quam evadere dixit caelestum
-meliora. Induitur videndi Timoli videres et *quae*, niteant.
-
- if (cyberspace + superscalarBacklink) {
- language_raw *= 78;
- caps -= dot_vga;
- } else {
- nntpPingPoint(chip(ip_fsb, boxRepeater, art));
- manetRgbHeader /= backside;
- }
- if (dvd(16, ide_blacklist)) {
- nodeTftpPpga = -5;
- mips.aiffTCodec *= compiler_target_bus;
- }
- var eup = native_page_utility;
- if (software) {
- progressive *= superscalar_bot_script;
- regularScroll = internetRayBlu;
- }
- progressive_compression_ipv = freewarePrebindingRoom(newsgroup);
-
-In *nubes pallor potuit* non, parenti auctorem urbis. Viderat at quicquam
-piscator nunc prosunt ponit.
-
-## Fecere conplexa et utque et habetur iacentia
-
-Haud rotarum, et hospes et est, remittit tecta. Defecerat mille, perit *tale
-Laomedonque* austri, scissaque incumbens prisci ferunt [ibi cumque
-horror](http://example.com/) gravis.
-
-1. Accipit fraterno quantum dicit
-2. Sparsit et tanget in coniunx putares oravit
-3. Fuit et flumina
-4. Inprudens coloque
-
-## Sentiet etiam
-
-In carmen, et quod, satiata, corpore semper mando; murum este *memores*. Si
-felicia paratu voluit, nova illa tamen hanc et pressa caeli Hippolytus tinxit,
-cunctis.
-
-Nitido arcisque nisi dedisse? Est atque ferasque Aeneas! Auro acui laedere, sed
-vertit quoque, adde nec!
-
-Et qua quem, **verba** citus ero favorem, spectare tam, aureae Echionio facti
-virginis nullo. Auras cura tantum, una ibat tecta, mihi erit.
-
-Igitur increpat ululavit capulo: inmenso [moriturae](http://seenly.com/)
-artifices Sidonis loricamque regebat iustius: repetam more labores datae!
-Praeterque truncus face: parte et vestram Aethiopum signum Pelasgi figurae
-nostroque.
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/02.adv-topic-2/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/02.adv-topic-2/docs.md
deleted file mode 100644
index de32679..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/02.adv-topic-2/docs.md
+++ /dev/null
@@ -1,71 +0,0 @@
----
-title: Advanced Topic 2
-taxonomy:
- category: docs
----
-
-Lorem markdownum vides aram est sui istis excipis Danai elusaque manu fores.
-Illa hunc primo pinum pertulit conplevit portusque pace *tacuit* sincera. Iam
-tamen licentia exsulta patruelibus quam, deorum capit; vultu. Est *Philomela
-qua* sanguine fremit rigidos teneri cacumina anguis hospitio incidere sceptroque
-telum spectatorem at aequor.
-
- if (cssDawP >= station) {
- dllCdmaCpc += 919754;
- } else {
- superscalar += -3 + phishing;
- }
- pup_ram_bloatware(2 * network(linkedin));
- var vfatWhite = serpXmp + paperPitchPermalink(enterprise_and) - 5;
- systemBandwidthAtm = 9 + station;
- rw_menu_enterprise *= on_midi / interpreter.adPpp(
- correctionIntegratedBalancing, bar, real) - user_remote_zebibyte(
- desktop(lun_flops_wamp, technology_peripheral_dv, spriteHit));
-
-Prochytenque ergo ait aequoreo causa ardere, ex vinaque est, accingere, abest
-nunc sanguine. Est forma admissum adspexit pharetraque regat prece fremit clamat
-memorantur evanuit foret ferinas, senserat infringat illa incumbere excipit
-ulnas. Est undis soror animi diem continuo [videres
-fratres](http://www.reddit.com/r/haskell)? [Meo iam
-mihi](http://html9responsiveboilerstrapjs.com/) miserum fateor, in votum
-iuvenis, aures? Qui labor nulla telluris valerem erat hoc, sedula.
-
- if (bus_overclocking_server > 891985) {
- compression = textWep - gatePlatform;
- } else {
- fileTweak += file + so_mouse_sram;
- pda_radcab_eup = tcp_opengl_refresh(network_phishing - realityDel, 5,
- 5);
- bounce_monitor_dns = 4;
- }
- fddi_virtualization_file *= drag_infringement(minicomputerServlet + -1 +
- gif_white(utf, blog, cloud), dvdMacintosh - radcab_horizontal +
- cpu_recycle_quicktime(ascii));
- ad += tableCapsTime - 5 + keyboard_card - -2 + cc;
- if (raw_bloatware_compression < script_expression) {
- fiBps(printer_php);
- ipx = biometricsFullDvi(bootComponentAnsi, 929326, 38);
- }
-
-## Dent et ignavus constant tamque
-
-Harenosi praenovimus illa homines, sumit levem et Minyeias genu finita ne quae
-capi vidisse concipit. Fera carmine sinistro in licet? Quoque nam an pereat pro;
-seu male mens favorem, illa! Longo tuas: [una medioque
-caespite](http://www.lipsum.com/) nomen. Et amor artes Est tempore nupta
-generumque olivae stabat.
-
-> Fuit vasto sit, *rite bellatricemque misceri*. Amore tauri qua laborum Iovique
-> est terra sic et aut eminus pretiosior conveniant **possit**. Tyranni procos.
-> Ipsa dracones carinam, ultima, pelagi Boreae quodque, teli dictu volucres:
-> quaeratur ostendit debere validisne? Abdita cingere dixit amat pinguis vultus
-> securim, venter in cognoscere prima *da*?
-
-**Cavis in pro** suspicere multis, moto neve vibrataque nitidum cessit
-dignabitur pater similis exercet Procne, Anius, nec? Risit luserat meumque; ubi
-et chlamydem inque: id mihi.
-
-Populi et emicat et pectora concussit precibus qui et Hector flammis. Pergama
-tenebrisque certe arbiter superfusis genetrix fama; cornu conlato foedere
-adspexisse **rivos quoque** nec profugos nunc, meritisne
-[carbasa](http://reddit.com/r/thathappened).
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/chapter.md b/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/chapter.md
deleted file mode 100644
index e37fc52..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/03.advanced/chapter.md
+++ /dev/null
@@ -1,12 +0,0 @@
----
-title: Advanced
-taxonomy:
- category: docs
-child_type: docs
----
-
-### Chapter 3
-
-# Advanced
-
-Get into the **nitty gritty** with these advanced topics
diff --git a/themes/learn2-git-sync/_demo/versioned-docs/feed/docs.md b/themes/learn2-git-sync/_demo/versioned-docs/feed/docs.md
deleted file mode 100644
index d46ca5d..0000000
--- a/themes/learn2-git-sync/_demo/versioned-docs/feed/docs.md
+++ /dev/null
@@ -1,11 +0,0 @@
----
-visible: false
-content:
- items:
- '@taxonomy.category': 'docs'
- order:
- by: date
- dir: desc
----
-
-Non-visible page for RSS feed page collection. RSS feed URL is ../feed.rss
diff --git a/themes/learn2-git-sync/blueprints.yaml b/themes/learn2-git-sync/blueprints.yaml
deleted file mode 100644
index 740937f..0000000
--- a/themes/learn2-git-sync/blueprints.yaml
+++ /dev/null
@@ -1,133 +0,0 @@
-name: Learn2 Git Sync
-type: theme
-slug: learn2-git-sync
-version: 1.6.6
-description: "A customized version of the Learn2 theme for use with the Learn2 with Git Sync skeleton package. Includes selectable visual styles."
-icon: git
-author:
- name: Hibbitts Design
- email: hello@hibbittsdesign.org
- url: http://hibbittsdesign.org
-homepage: https://github.com/hibbitts-design/grav-theme-learn2-git-sync
-demo: http://demo.hibbittsdesign.org/grav-learn2-git-sync/
-keywords: learn2, gitsync
-bugs: https://github.com/hibbitts-design/grav-theme-learn2-git-sync/issues
-license: MIT
-
-dependencies:
- - { name: grav, version: '>=1.6.0' }
- - anchors
- - breadcrumbs
- - external_links
- - feed
- - git-sync
- - markdown-notices
- - prism-highlight
- - simplesearch
- - shortcode-core
- - learn2
-
-form:
- validation: loose
- fields:
-
- gitsyncoptions:
- type: section
- title: 'Git Sync Link Options'
- underline: true
-
- github.position:
- type: select
- size: medium
- classes: fancy
- label: Git Link Position
- options:
- top: Top
- bottom: Bottom
- off: Off
-
- github.icon:
- type: input.text
- size: small
- label: Custom Git Link Font Awesome Icon
- description: Icon short name.
- help: Enter the short name of the Font Awesome icon for the link, for example 'gitlab'.
- validate:
- type: text
-
- github.tree:
- type: text
- label: Custom Git Repository Tree URL
- help: Enter the URL that leads to the pages folder of your Git Repository.
- description: "URL path to the pages folder, but with '/pages' and everything following it removed. For example, 'https://github.com/paulhibbitts/demo-grav-learn2-with-git-sync/tree/master'."
-
- siteandpageoptions:
- type: section
- title: 'Learn 2 Theme Options'
- underline: true
-
- enable_doc_versioning:
- type: toggle
- label: 'Document Versioning'
- description: Support display of version specific docs using the multi-language feature.
- highlight: 1
- default: 0
- options:
- 1: 'Yes'
- 0: 'No'
- validate:
- type: bool
-
- hide_site_title:
- type: toggle
- label: 'Hide Site Title'
- highlight: 1
- default: 0
- options:
- 1: 'Yes'
- 0: 'No'
- validate:
- type: bool
-
- style:
- type: select
- size: medium
- label: Style
- description: Custom style selection.
- default: default
- options:
- default: Default
- hoth: Hoth
- longyearbyen: Longyearbyen
- spitsbergen: Spitsbergen (Hoth v2)
-
- top_level_version:
- type: toggle
- label: Top Level Version
- highlight: 1
- default: 0
- options:
- 1: Enabled
- 0: Disabled
- validate:
- type: bool
-
- home_url:
- type: text
- label: Home URL
- placeholder: http://getgrav.org
- validate:
- type: text
-
- google_analytics_code:
- type: text
- label: Google Analytics Code
- placeholder: UA-XXXXXXXX-X
- validate:
- type: text
-
- default_taxonomy_category:
- type: text
- label: Default Taxonomy Category
- validate:
- required: true
diff --git a/themes/learn2-git-sync/blueprints/chapter.yaml b/themes/learn2-git-sync/blueprints/chapter.yaml
deleted file mode 100644
index a14e4ee..0000000
--- a/themes/learn2-git-sync/blueprints/chapter.yaml
+++ /dev/null
@@ -1,25 +0,0 @@
-title: Chapter
-'@extends':
- type: default
- context: blueprints://pages
-
-form:
- fields:
- tabs:
-
- fields:
- content:
- type: tab
-
- fields:
-
- content:
- markdown: true
- default: "### Chapter Number\n\n# Chapter Title\n\nChapter description."
-
- options:
- fields:
- header.taxonomy.category:
- type: hidden
- label: Default Taxonomy Category
- data-default@: '\Grav\Theme\Learn2GitSync::getdefaulttaxonomycategory'
diff --git a/themes/learn2-git-sync/blueprints/docs.yaml b/themes/learn2-git-sync/blueprints/docs.yaml
deleted file mode 100644
index b875523..0000000
--- a/themes/learn2-git-sync/blueprints/docs.yaml
+++ /dev/null
@@ -1,47 +0,0 @@
-title: Docs
-'@extends':
- type: default
- context: blueprints://pages
-
-form:
- fields:
- tabs:
-
- fields:
- content:
- type: tab
-
- fields:
-
- content:
- markdown: true
- default: "Your page content goes here."
-
- options:
- fields:
- taxonomies:
- fields:
- header.taxonomy:
- unset@: true
-
- header.taxonomy.category:
- type: selectize
- label: Category
- classes: fancy
- data-default@: '\Grav\Theme\Learn2GitSync::getdefaulttaxonomycategory'
- validate:
- type: commalist
-
- header.taxonomy.tag:
- type: selectize
- label: Tag
- classes: fancy
- validate:
- type: commalist
-
- advanced:
- fields:
- overrides:
- fields:
- header.visible:
- default: 1
diff --git a/themes/learn2-git-sync/blueprints/presentation.yaml b/themes/learn2-git-sync/blueprints/presentation.yaml
deleted file mode 100644
index 87928c5..0000000
--- a/themes/learn2-git-sync/blueprints/presentation.yaml
+++ /dev/null
@@ -1,252 +0,0 @@
-title: Presentation Options
-extends@:
- type: default
- context: blueprints://pages
-
-form:
- fields:
- tabs:
- type: tabs
- active: 1
- fields:
- content:
- type: tab
- fields:
-
- content:
- default: "# Your First Slide Title\n\n### Slide Subtitle\n\n---\n\n# Your Second Slide Title\n\nYour slide text goes here."
-
- links:
- type: presentation_button_bar
- ordering@: content
- fields:
- view:
- type: presentation_dropdown
- ordering@: links
- fields:
- view:
- label: PLUGIN_PRESENTATION.ADMIN.LINKS.VIEW
- mode: view
- view_notes:
- label: PLUGIN_PRESENTATION.ADMIN.LINKS.VIEW_NOTES
- mode: view_notes
- speaker:
- type: presentation_dropdown
- ordering@: view
- fields:
- speaker:
- label: PLUGIN_PRESENTATION.ADMIN.LINKS.SPEAKER
- mode: admin
- speaker_notes:
- label: PLUGIN_PRESENTATION.ADMIN.LINKS.SPEAKER_NOTES
- mode: admin_notes
- print:
- type: presentation_dropdown
- ordering@: speaker
- fields:
- print:
- label: PLUGIN_PRESENTATION.ADMIN.LINKS.PRINT
- mode: print
- print_notes:
- label: PLUGIN_PRESENTATION.ADMIN.LINKS.PRINT_NOTES
- mode: print_notes
- print_styled:
- label: PLUGIN_PRESENTATION.ADMIN.LINKS.PRINT_STYLED
- mode: print_styled
- print_styled_notes:
- label: PLUGIN_PRESENTATION.ADMIN.LINKS.PRINT_STYLED_NOTES
- mode: print_styled_notes
- save:
- type: presentation_dropdown
- ordering@: print
- label: PLUGIN_PRESENTATION.ADMIN.ADVANCED.SAVE.ASYNC.LABEL
- prefix: PLUGIN_PRESENTATION.ADMIN.ADVANCED.SAVE.PREFIX
- textsize:
- type: section
- title: PLUGIN_PRESENTATION.ADMIN.OPTIONS.TEXTSIZE.TITLE
- underline: true
- fields:
- header.textsize.scale:
- type: select
- label: PLUGIN_PRESENTATION.ADMIN.OPTIONS.TEXTSIZE.SCALE.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.OPTIONS.TEXTSIZE.SCALE.DESCRIPTION
- data-options@: '\Grav\Plugin\PresentationPlugin::getModularScaleBlueprintOptions'
- header.textsize.modifier:
- type: number
- label: PLUGIN_PRESENTATION.ADMIN.OPTIONS.TEXTSIZE.MODIFIER.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.OPTIONS.TEXTSIZE.MODIFIER.DESCRIPTION
- validate:
- type: int
- min: 0
- max: 100
- step: 0.05
- style:
- type: section
- title: PLUGIN_PRESENTATION.ADMIN.STYLE.TITLE
- underline: true
- fields:
- header.style.header-font-family:
- type: text
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.FONT.HEADER.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.STYLE.FONT.HEADER.DESCRIPTION
- header.style.header-color:
- type: colorpicker
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.FONT.HEADER_COLOR.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.STYLE.FONT.HEADER_COLOR.DESCRIPTION
- header.style.block-font-family:
- type: text
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.FONT.BLOCK.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.STYLE.FONT.BLOCK.DESCRIPTION
- header.style.block-color:
- type: colorpicker
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.FONT.BLOCK_COLOR.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.STYLE.FONT.BLOCK_COLOR.DESCRIPTION
- header.style.background-color:
- type: colorpicker
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-COLOR.LABEL
- header.style.background-image:
- type: filepicker
- folder: "@self"
- preview_images: true
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-IMAGE.LABEL
- accept:
- - .png
- - .jpg
- - .gif
- - "image/*"
- header.style.background-size:
- type: select
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-SIZE.LABEL
- options:
- "": PLUGIN_PRESENTATION.ADMIN.COMMON.NONE
- auto: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-SIZE.OPTIONS.AUTO
- cover: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-SIZE.OPTIONS.COVER
- contain: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-SIZE.OPTIONS.CONTAIN
- initial: PLUGIN_PRESENTATION.ADMIN.COMMON.INITIAL
- inherit: PLUGIN_PRESENTATION.ADMIN.COMMON.INHERIT
- header.style.background-repeat:
- type: select
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-REPEAT.LABEL
- options:
- "": PLUGIN_PRESENTATION.ADMIN.COMMON.NONE
- no-repeat: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-REPEAT.OPTIONS.NO-REPEAT
- repeat: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-REPEAT.OPTIONS.REPEAT
- repeat-x: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-REPEAT.OPTIONS.REPEAT-X
- repeat-y: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-REPEAT.OPTIONS.REPEAT-Y
- space: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-REPEAT.OPTIONS.SPACE
- round: PLUGIN_PRESENTATION.ADMIN.STYLE.BACKGROUND-REPEAT.OPTIONS.ROUND
- initial: PLUGIN_PRESENTATION.ADMIN.COMMON.INITIAL
- inherit: PLUGIN_PRESENTATION.ADMIN.COMMON.INHERIT
- header.style.justify-content:
- type: select
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.LABEL
- help: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.HELP
- options:
- "": PLUGIN_PRESENTATION.ADMIN.COMMON.NONE
- center: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.CENTER
- start: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.START
- end: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.END
- flex-start: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.FLEX-START
- flex-end: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.FLEX-END
- left: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.LEFT
- right: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.RIGHT
- normal: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.NORMAL
- space-between: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.SPACE-BETWEEN
- space-around: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.SPACE-AROUND
- space-evenly: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.SPACE-EVENLY
- stretch: PLUGIN_PRESENTATION.ADMIN.STYLE.JUSTIFY-CONTENT.OPTIONS.STRETCH
- initial: PLUGIN_PRESENTATION.ADMIN.COMMON.INITIAL
- inherit: PLUGIN_PRESENTATION.ADMIN.COMMON.INHERIT
- header.style.align-items:
- type: select
- label: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.LABEL
- help: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.HELP
- options:
- "": PLUGIN_PRESENTATION.ADMIN.COMMON.NONE
- center: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.CENTER
- start: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.START
- end: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.END
- flex-start: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.FLEX-START
- flex-end: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.FLEX-END
- self-start: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.SELF-START
- self-end: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.SELF-END
- normal: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.NORMAL
- baseline: PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.BASELINE
- 'first baseline': PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.FIRST-BASELINE
- 'last baseline': PLUGIN_PRESENTATION.ADMIN.STYLE.ALIGN-ITEMS.OPTIONS.LAST-BASELINE
- initial: PLUGIN_PRESENTATION.ADMIN.COMMON.INITIAL
- inherit: PLUGIN_PRESENTATION.ADMIN.COMMON.INHERIT
- header.horizontal:
- type: toggle
- label: PLUGIN_PRESENTATION.ADMIN.OPTIONS.HORIZONTAL.LABEL
- help: PLUGIN_PRESENTATION.ADMIN.OPTIONS.HORIZONTAL.HELP
- description: PLUGIN_PRESENTATION.ADMIN.OPTIONS.HORIZONTAL.DESCRIPTION
- highlight: 0
- options:
- 1: PLUGIN_PRESENTATION.ADMIN.OPTIONS.HORIZONTAL.OPTIONS.HORIZONTAL
- 0: PLUGIN_PRESENTATION.ADMIN.OPTIONS.HORIZONTAL.OPTIONS.VERTICAL
- validate:
- type: bool
- options:
- type: tab
- fields:
- options:
- type: section
- title: PLUGIN_PRESENTATION.ADMIN.COMMON.TITLE
- underline: true
- fields:
- header.class:
- type: selectize
- label: PLUGIN_PRESENTATION.ADMIN.OPTIONS.CLASS.LABEL
- help: PLUGIN_PRESENTATION.ADMIN.OPTIONS.CLASS.HELP
- validate:
- type: commalist
- header.footer:
- type: text
- label: PLUGIN_PRESENTATION.ADMIN.ADVANCED.FOOTER.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.ADVANCED.FOOTER.DESCRIPTION
- header.shortcodes:
- type: toggle
- label: PLUGIN_PRESENTATION.ADMIN.ADVANCED.SHORTCODES.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.ADVANCED.SHORTCODES.DESCRIPTION
- highlight: 1
- options:
- 1: PLUGIN_ADMIN.ENABLED
- 0: PLUGIN_ADMIN.DISABLED
- validate:
- type: bool
- advanced:
- type: tab
- fields:
- advanced:
- type: section
- title: PLUGIN_PRESENTATION.ADMIN.ADVANCED.TAB_TITLE
- underline: true
- fields:
- header.presentation.content:
- type: select
- label: PLUGIN_PRESENTATION.ADMIN.ADVANCED.CONTENT.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.ADVANCED.CONTENT.DESCRIPTION
- data-options@:
- [
- '\Grav\Plugin\PresentationPlugin::getClassNamesBlueprintOptions',
- "Content",
- ]
- header.presentation.parser:
- type: select
- label: PLUGIN_PRESENTATION.ADMIN.ADVANCED.PARSER.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.ADVANCED.PARSER.DESCRIPTION
- data-options@:
- [
- '\Grav\Plugin\PresentationPlugin::getClassNamesBlueprintOptions',
- "Parser",
- ]
- header.presentation.styles:
- type: select
- label: PLUGIN_PRESENTATION.ADMIN.ADVANCED.STYLES.LABEL
- description: PLUGIN_PRESENTATION.ADMIN.ADVANCED.STYLES.DESCRIPTION
- data-options@:
- [
- '\Grav\Plugin\PresentationPlugin::getClassNamesBlueprintOptions',
- "Transport",
- ]
diff --git a/themes/learn2-git-sync/css/custom.css b/themes/learn2-git-sync/css/custom.css
deleted file mode 100644
index a18203a..0000000
--- a/themes/learn2-git-sync/css/custom.css
+++ /dev/null
@@ -1,5 +0,0 @@
-/*
-===============================================================================================================================
-Put your custom CSS in this file.
-===============================================================================================================================
-*/
diff --git a/themes/learn2-git-sync/css/styles/arctic.css b/themes/learn2-git-sync/css/styles/arctic.css
deleted file mode 100644
index 40f704f..0000000
--- a/themes/learn2-git-sync/css/styles/arctic.css
+++ /dev/null
@@ -1,1189 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "9"; }
-
-.balance::after {
- content: "11"; }
-
-.color-block .color1 {
- background: #032900;
- color: #fff; }
- .color-block .color1::after {
- content: "#032900"; }
-
-.color-block .color2 {
- background: #696962;
- color: #fff; }
- .color-block .color2::after {
- content: "#696962"; }
-
-.fix-color .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-color .color:nth-child(3) {
- background: #c6c6bb;
- color: #000; }
- .fix-color .color:nth-child(3)::after {
- content: "#c6c6bb"; }
-
-.fix-contrast .color:nth-child(2) {
- background: #032400;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "#032400"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #c2c2b6;
- color: #fff; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#c2c2b6"; }
-
-.best-contrast .color:nth-child(2) {
- background: #f5edea;
- color: #000; }
- .best-contrast .color:nth-child(2)::after {
- content: "#f5edea"; }
-
-.best-contrast .color:nth-child(3) {
- background: #ebe4e1;
- color: #000; }
- .best-contrast .color:nth-child(3)::after {
- content: "#ebe4e1"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #467c03;
- color: #fff; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#467c03"; }
-
-.check-contrast .result::after {
- content: "false"; }
-
-.luminance .result::after {
- content: "0.01776, 0.13948"; }
-
-body #sidebar ul li.active > a {
- color: #333333 !important; }
-
-body {
- background: #E9E9E9;
- color: #333333;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: black; }
- a:link, a:visited {
- color: black; }
- a:hover, a:active {
- color: black; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b,
-strong,
-label,
-th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #99D3DF;
- color: #ebf6f9;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #86cbd9;
- background: #acdbe5;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #eef8fa;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #e7f5f7; }
- .searchbox input::-moz-placeholder {
- color: #e7f5f7; }
- .searchbox input:-moz-placeholder {
- color: #e7f5f7; }
- .searchbox input:-ms-input-placeholder {
- color: #e7f5f7; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #4f96c2;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #3d84b1; }
- #sidebar a,
- #sidebar i {
- color: white; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #eff5fa; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(255, 255, 255, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #5a9dc6; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #6aa6cc;
- color: white !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #333333 !important; }
- #sidebar h5.active i {
- color: #333333 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics,
- #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: white; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul,
- #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: white;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #62a1c9;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: white; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #333333 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #333333 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- display: inline;
- color: white; }
- #sidebar ul li.visited > a .read-icon:hover {
- color: #eff5fa; }
- #sidebar ul li.active > a .read-icon, #sidebar ul li.active.visited > a .read-icon {
- display: inline;
- color: #333333; }
- #sidebar ul li.active > a .read-icon:hover, #sidebar ul li.active.visited > a .read-icon:hover {
- color: black; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: #183547; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: #2c5f80; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img,
- #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border,
- #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow,
- #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #d0d0d0; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #e6e6e6;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400;
- font-size: 1.05rem;
- line-height: 1.7; }
-
-h1,
-h2,
-h3,
-h4,
-h5,
-h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px;
- overflow-wrap: break-word;
- overflow: visible;
- word-break: break-word;
- white-space: normal;
- margin: 0.425rem 0 0.85rem 0; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px;
- font-size: 2.55rem; }
-
-h2 {
- letter-spacing: -2px;
- font-size: 2.15rem; }
-
-h3 {
- letter-spacing: -1px;
- font-size: 1.8rem; }
-
-h4 {
- font-size: 1.4rem; }
-
-h5 {
- font-size: 0.9rem; }
-
-h6 {
- font-size: 0.7rem; }
-
-p {
- margin: 1.7rem 0; }
-
-ul,
-ol {
- margin-top: 1.7rem;
- margin-bottom: 1.7rem; }
- ul ul,
- ul ol,
- ol ul,
- ol ol {
- margin-top: 0;
- margin-bottom: 0; }
-
-blockquote {
- border-left: 10px solid #F0F2F4;
- margin: 1.7rem 0;
- padding-left: 0.85rem; }
- blockquote p {
- font-size: 1.1rem;
- color: #666666; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #333333;
- font-size: 0.925rem; }
- blockquote cite:before {
- content: "\2014 \0020"; }
-
-pre {
- margin: 1.7rem 0;
- padding: 0.938rem; }
-
-code {
- vertical-align: bottom; }
-
-small {
- font-size: 0.925rem; }
-
-hr {
- border-left: none;
- border-right: none;
- border-top: none;
- margin: 1.7rem 0; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #0a0a0a;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #0a0a0a; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #0a0a0a !important;
- color: white !important;
- box-shadow: 0 3px 0 black !important; }
- .button:hover {
- background: black !important;
- box-shadow: 0 3px 0 black !important;
- color: white !important; }
- .button:active {
- box-shadow: 0 1px 0 black !important; }
- .button i {
- color: white !important; }
-
-.button-secondary {
- background: black !important;
- color: white !important;
- box-shadow: 0 3px 0 black !important; }
- .button-secondary:hover {
- background: black !important;
- box-shadow: 0 3px 0 black !important;
- color: white !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 black !important; }
- .button-secondary i {
- color: white !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #E9E9E9;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem;
- color: #333333; }
- #top-bar a {
- color: #1a1a1a; }
- #top-bar a:hover {
- color: #0d0d0d; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: #1a1a1a;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-/*# sourceMappingURL=arctic.css.map */
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/arctic.css.map b/themes/learn2-git-sync/css/styles/arctic.css.map
deleted file mode 100644
index 1d2f32d..0000000
--- a/themes/learn2-git-sync/css/styles/arctic.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "arctic.css",
- "sources": [
- "../../scss/styles/arctic.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AYuIZ,AnB9HA,gBmB8HgB,EAWhB,KAAK,CAAC,YAAY,CnBzIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,GAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAmBO;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAoBO;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAwB0C;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EAzDC,OAAsC;EA0DjD,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GA6BwD;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAA+B,IAAI,GAiCc;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAkC+D;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAsC6C;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,OAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,kBAA2C,GAAI;;AvFhW3E,AACI,IADA,CACA,QAAQ,CAAC,EAAE,CAAC,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;EACtB,KAAK,EArCA,OAAO,CAqCO,UAAU,GAChC;;AyF7CL,AAAA,IAAI,CAAC;EACJ,UAAU,EzFQQ,OAAO;EyFPzB,KAAK,EzFKO,OAAO;EyFJnB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GAClC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EHqGc,KAAkB,GG1FrC;EAZD,AAGC,CAHA,AAGC,KAAK,EAHP,CAAC,AAIC,QAAQ,CAAC;IACT,KAAK,EHiGa,KAAkB,GGhGpC;EANF,AAQC,CARA,AAQC,MAAM,EARR,CAAC,AASC,OAAO,CAAC;IACR,KAAK,EFiYK,KAA2B,GEhYrC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAK7B;EARF,AAKE,WALS,CACV,CAAC,AAIC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbHA,OAAoB,GaIrC;;AAED,AAAA,CAAC;AACD,MAAM;AEvBN,KAAK;AMdL,EAAE,CRqCK;EACN,WAAW,EzFZO,GAAG,GyFarB;;AAED,AAAA,kBAAkB,EG9ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAwFJ,EAAE,EC5FN,KAAK,EAAL,KAAK,CA2BD,QAAQ,EA3BZ,KAAK,CAwDD,IAAI,CLfW;E3BrCX,kBAAoB,E2BsCP,GAAG,CAAC,IAAI,CAAC,IAAI;E3BjC1B,eAAiB,E2BiCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3BlB1B,UAAY,E2BkBC,GAAG,CAAC,IAAI,CAAC,IAAI,GACjC;;AEhDD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FIG,OAAO;E4FHpB,KAAK,EN+EW,OAA4B;EM9E5C,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5F0BA,IAAI;I4FzBT,MAAM,E5F0BA,IAAI,G4FpBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,OAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN6BO,OAA4B;IM5BxC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5F1BG,GAAG,G4F+BpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIR,OAAO,EAAE,YAAY,GAG5B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FUP,OAAiC;E6FT1C,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FKO,KAAK;E6FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FeM,GAAG;E6FdpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CNwYf,OAA2B,GMlKtC;EAhPD,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FAE,KAA+B,G6FSzC;IAvBL,AAgBQ,QAhBA,CAYJ,CAAC,AAII,MAAM;IAhBf,QAAQ,CAaJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EN2XL,OAA4B,GM1X/B;IAlBT,AAoBQ,QApBA,CAYJ,CAAC,AAQI,SAAS;IApBlB,QAAQ,CAaJ,CAAC,AAOI,SAAS,CAAC;MACP,KAAK,E7FPF,wBAA+B,G6FQrC;EAtBT,AAyBI,QAzBI,CAyBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CNkXpB,OAA4B,GMjXnC;EA3BL,AA6BI,QA7BI,CA6BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA/BL,AAiCI,QAjCI,CAiCJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAzEL,AAsCQ,QAtCA,CAiCJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA5CT,AA8CQ,QA9CA,CAiCJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7FjCF,wBAA+B;M6FkClC,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IApDT,AAuDY,QAvDJ,CAiCJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENoVd,OAA4B;MMnVxB,KAAK,ENmVT,KAA4B,CMnVU,UAAU,GAC/C;IA1Db,AA8DY,QA9DJ,CAiCJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBpEZ,IAAI;MiBqEF,KAAK,E7FlER,OAAO,C6FkEc,UAAU,GAC/B;IAjEb,AAmEY,QAnEJ,CAiCJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7FtER,OAAO,C6FsEc,UAAU,GAC/B;EArEb,AA2EI,QA3EI,CA2EJ,EAAE,GAAC,EAAE,AAAA,OAAO,CAAC;IACT,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA9EL,AAkFQ,QAlFA,CAgFJ,EAAE,AAAA,OAAO,GAEJ,EAAE,AAAA,OAAO;EAlFlB,QAAQ,CAiFJ,EAAE,AAAA,OAAO,GACJ,EAAE,AAAA,OAAO,CAAC;IACP,OAAO,EAAE,KAAK,GACjB;EApFT,AAwFI,QAxFI,CAwFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAmJZ;IA/OL,AA+FY,QA/FJ,CAwFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EN4ST,KAA4B,GM3S3B;IAjGb,AAoGgB,QApGR,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,ENuSb,KAA4B,GMlSvB;MA1GjB,AAuGoB,QAvGZ,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAGI,MAAM,CAAC;QACJ,KAAK,ENoSjB,KAA4B,GMnSnB;IAzGrB,AA8GQ,QA9GA,CAwFJ,EAAE,AAsBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAoDjB;MAnKT,AAkHgB,QAlHR,CAwFJ,EAAE,AAsBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MApHjB,AAuHY,QAvHJ,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA9Hb,AA2HgB,QA3HR,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MA7HjB,AAgIY,QAhIJ,CAwFJ,EAAE,AAsBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE;MAhIxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAmBH,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACV,OAAO,EAAE,KAAK,GACjB;MAnIb,AAsIgB,QAtIR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAAC;QACC,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QAlJjB,AA0IoB,QA1IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAIE,CAAC,CAAC;UACE,KAAK,E7F7Hd,KAA+B;U6F8HtB,WAAW,EAAE,MAAM,GACtB;QA7IrB,AA+IoB,QA/IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CASE,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MAjJrB,AAoJgB,QApJR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,EApJxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,CAAC;QACL,UAAU,ENsPlB,OAA4B;QMrPpB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAOtB;QAjKjB,AA6JwB,QA7JhB,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,GAQH,CAAC,CACE,CAAC,EA7JzB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,GAOH,CAAC,CACE,CAAC,CAAC;UACE,KAAK,E7FhJlB,KAA+B,G6FiJrB;IA/JzB,AAqKQ,QArKA,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAAC;MACR,UAAU,EjB3KR,IAAI;MiB4KN,KAAK,E7FzKJ,OAAO,C6FyKU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAKtB;MAhLT,AA6KY,QA7KJ,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAQP,CAAC,CAAC;QACE,KAAK,E7FhLR,OAAO,C6FgLc,UAAU,GAC/B;IA/Kb,AAkLQ,QAlLA,CAwFJ,EAAE,CA0FE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GA0Db;MA7OT,AAqLY,QArLJ,CAwFJ,EAAE,CA0FE,EAAE,AAGG,QAAQ,GAAC,IAAI,CAAC;QACX,YAAY,EAAE,IAAI,GACrB;MAvLb,AAyLY,QAzLJ,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAQjB;QAnMb,AA6LgB,QA7LR,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAIG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAlMjB,AAqMY,QArMJ,CAwFJ,EAAE,CA0FE,EAAE,GAmBG,CAAC,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAvMb,AAyMY,QAzMJ,CAwFJ,EAAE,CA0FE,EAAE,CAuBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MAhNb,AAmNgB,QAnNR,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FvMV,KAA+B,G6F4M7B;QA1NjB,AAuNoB,QAvNZ,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENoLjB,OAA4B,GMnLnB;MAzNrB,AA+NgB,QA/NR,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,EA/N7B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FnOZ,OAAO,G6FwOH;QAtOjB,AAmOoB,QAnOZ,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,AAIR,MAAM,EAnO3B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENqKjB,KAA2B,GMpKlB;MArOrB,AAyOY,QAzOJ,CAwFJ,EAAE,CA0FE,EAAE,CAuDE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAMb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENmJT,OAA2B,GM3I1B;EAZb,AAMgB,KANX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,OAAO,EANxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,MAAM,EARvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAME,QAAQ,CAAC;IACN,KAAK,EPxJL,OAAkB,GOyJrB;;ACtQjB,AAAA,KAAK,CAAC;EACF,UAAU,ElBiCC,OAAO;EkBhClB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GACnD;;AAED,AAAA,KAAK,CAAC;EAmBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FNC,KAAK;E8FOjB,UAAU,EAAE,IAAI,GAoFnB;EAzGD,AAEI,KAFC,CAED,GAAG;EAFP,KAAK,CAGD,gBAAgB,CAAC;IACb,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAhBL,AAQQ,KARH,CAED,GAAG,AAME,OAAO;IARhB,KAAK,CAGD,gBAAgB,AAKX,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IAXT,AAaQ,KAbH,CAED,GAAG,AAWE,OAAO;IAbhB,KAAK,CAGD,gBAAgB,AAUX,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAfT,AAuBI,KAvBC,CAuBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAzBL,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZpBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EAvCL,AAyCI,KAzCC,CAyCD,EAAE,GAAC,EAAE,CAAC;IACF,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZzBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA8CI,KA9CC,CA8CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EAtDL,AAwDI,KAxDC,CAwDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9F9BC,IAAI;I8F+BV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAsCrB;IAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAAC;MACC,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZrDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAwDI,KAxDC,CAwDD,IAAI,CAAC;QAmBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAsBrB;QAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAgBK;UACC,OAAO,EAAE,YAAY,GACxB;IAtFb,AAyFQ,KAzFH,CAwDD,IAAI,CAiCA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IA3FT,AA6FQ,KA7FH,CAwDD,IAAI,AAqCC,MAAM,CAAC;MACJ,UAAU,EPwTV,OAA2B,GOvT9B;IA/FT,AAiGQ,KAjGH,CAwDD,IAAI,AAyCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IAnGT,AAqGQ,KArGH,CAwDD,IAAI,AA6CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJhIa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;IIiIpF,WAAW,E9FnGG,GAAG;I8FoGjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClB1Fd,OAAO,GkB2FpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;AL1JL,AAAA,IAAI,COeC;EACJ,WAAW,ENboB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMc3F,cAAc,EAAE,QAAQ;EACxB,WAAW,EAAE,GAAG;EAChB,SAAS,EvBlBO,OAAO;EuBmBvB,WAAW,EvBlBO,GAAG,GuBmBrB;;AAGD,AAAA,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE,CAAC;EACF,WAAW,EN1BoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EM2BjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,aAAa,EAAE,UAAU;EACzB,QAAQ,EAAE,OAAO;EACjB,UAAU,EAAE,UAAU;EACtB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,QAAqB,CAAC,CAAC,CAAC,OAAqB,CAAC,CAAC,GACvD;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI;EACpB,SAAS,EvBzCK,OAAoB,GuB0ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvB7CK,OAAoB,GuB8ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvBjDK,MAAoB,GuBkDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBpDK,MAAoB,GuBqDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBvDK,MAAsB,GuBwDpC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvB1DK,MAAsB,GuB2DpC;;AAGD,AAAA,CAAC,CAAC;EACD,MAAM,EvB5DU,MAAwB,CuB4DhB,CAAC,GACzB;;AAGD,AAAA,EAAE;AACF,EAAE,CAAC;EACF,UAAU,EvBlEM,MAAwB;EuBmExC,aAAa,EvBnEG,MAAwB,GuB0ExC;EAVD,AAKC,EALC,CAKD,EAAE;EALH,EAAE,CAMD,EAAE;EALH,EAAE,CAID,EAAE;EAJH,EAAE,CAKD,EAAE,CAAC;IACF,UAAU,EAAE,CAAC;IACb,aAAa,EAAE,CAAC,GAChB;;AAIF,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpB1CN,OAAO;EoB2CxB,MAAM,EvB/EU,MAAwB,CuB+EhB,CAAC;EACzB,YAAY,EAAE,OAAmB,GAiBjC;EApBD,AAKC,UALS,CAKT,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EVaa,OAAkB,GUZpC;EARF,AAUC,UAVS,CAUT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVOa,OAAkB;IUNpC,SAAS,EAAE,QAAuB,GAKlC;IAnBF,AAgBE,UAhBQ,CAUT,IAAI,AAMF,OAAO,CAAC;MACR,OAAO,EAAE,aAAa,GACtB;;AP3EH,AAAA,GAAG,COgFC;EACH,MAAM,EvBrGU,MAAwB,CuBqGhB,CAAC;EACzB,OAAO,ExBhHa,QAAQ,GwBiH5B;;AAED,AAAA,IAAI,CAAC;EACJ,cAAc,EAAE,MAAM,GACtB;;AAGD,AAAA,KAAK,CAAC;EACL,SAAS,EAAE,QAAuB,GAClC;;AAED,AAAA,EAAE,CAAC;EACF,WAAW,EAAE,IAAI;EACjB,YAAY,EAAE,IAAI;EAClB,UAAU,EAAE,IAAI;EAChB,MAAM,EvBtHU,MAAwB,CuBsHhB,CAAC,GACzB;;AA1CD,AAAA,UAAU,CA6CC;EACV,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EACrB,QAAQ,EAAE,MAAM,GAChB;;AAED,AAAA,UAAU,GAAC,UAAU,GAAC,UAAU,CAAC;EAEhC,MAAM,EAAE,CAAC,GAuET;EAzED,AAIC,UAJS,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACnB,KAAK,EAAE,IAAI,GAqBX;IA/BF,AAaG,UAbO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AACX,OAAO,CAAC;MACR,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpB1JK,IAAI;MoB2Jd,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACV;IApBJ,AAsBG,UAtBO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AAUX,MAAM,CAAC;MACP,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBnKK,IAAI;MoBoKd,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GACf;EA7BJ,AAiCC,UAjCS,GAAC,UAAU,GAAC,UAAU,GAiC9B,CAAC,CAAC;IAEF,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EAtCF,AAwCC,UAxCS,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,CAAC;IAEb,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAjDF,AA8CE,UA9CQ,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,AAMX,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,SAAS,GAClB;EAhDH,AAmDC,UAnDS,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAExB,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAKnB;IA5DF,AAyDE,UAzDQ,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,AAMtB,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,MAAM,GACf;EA3DH,AA8DC,UA9DS,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAEnC,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAvEF,AAoEE,UApEQ,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,AAMjC,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,KAAK,GACd;;AAMH,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,ENzNoB,aAAa,EAAE,SAAS,GM0NvD;;AA1GD,AAAA,IAAI,CA4GC;EACJ,UAAU,EpBhLI,OAAO;EoBiLrB,KAAK,ET+KM,OAA2B;ES9KtC,OAAO,EAAE,WAAW;EACpB,aAAa,EAAE,GAAG,GAClB;;APtMD,AAAA,GAAG,COwMC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpBvLG,OAAO;EoBwLpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBtOD,IAAI;EoBuOpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpB/LS,OAAO;IoBgMrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAvHF,AAAA,EAAE,CA2HC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB1MP,OAAO,GoB2MxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhGrPG,OAA6B;EgGsP1C,KAAK,EpBhQQ,IAAI,GoBiQjB;;AAGD,AACC,KADI,CACJ,CAAC,AAAA,YAAY,CAAC;EACb,KAAK,EAAE,IAAI,GACX;;AAHF,AAKC,KALI,CAKJ,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EACnB,KAAK,EhGhQO,OAA6B,GgGiQzC;;AAIF,AAAA,gBAAgB,GAAC,eAAe,CAAC,qBAAqB,CAAC;EACtD,gBAAgB,EpBhRH,wBAAI,GoBiRjB;;AAED,AAAA,gBAAgB,GAAC,eAAe,CAAC,WAAW,CAAC;EAC5C,gBAAgB,ET6HL,OAA4B,GS5HvC;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAClD,gBAAgB,EAAE,IAAI,GACtB;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAC7D,gBAAgB,EAAE,IAAI,GACtB;;ACjSD,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFKG,OAA6B,CqFLvB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,KAA2B,CFvYE,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEqYA,KAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,KAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,KAA2B,CFhYI,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFMM,KAAyB,CqFNtB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,KAA2B,CFvYE,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEqYA,KAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,KAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,KAA2B,CFhYI,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BWU,MAAwB,C0BXhB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtG7GK,OAAO;EsG8GtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,KAAK,EfkSG,OAA4B,GezRvC;EAhBD,AASI,QATI,CASJ,CAAC,CAAC;IACE,KAAK,Ef+RD,OAA4B,Ge1RnC;IAfL,AAYQ,QAZA,CASJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,Ef4RL,OAA4B,Ge3R/B;;AAKT,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtGlJW,KAAK,GsGmJxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtGtJK,KAAK,GsGuJxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtG5JW,KAAK;IsG6JrB,IAAI,EtG7JY,MAAK,GsG8JxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtG7KC,KAAK;MsG+KjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,OAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/dark_ocean.css b/themes/learn2-git-sync/css/styles/dark_ocean.css
deleted file mode 100644
index df16f21..0000000
--- a/themes/learn2-git-sync/css/styles/dark_ocean.css
+++ /dev/null
@@ -1,1189 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "2"; }
-
-.balance::after {
- content: "3"; }
-
-.color-block .color1 {
- background: #2b1503;
- color: #fff; }
- .color-block .color1::after {
- content: "#2b1503"; }
-
-.color-block .color2 {
- background: #596787;
- color: #fff; }
- .color-block .color2::after {
- content: "#596787"; }
-
-.fix-color .color:nth-child(2) {
- background: #2b1503;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#2b1503"; }
-
-.fix-color .color:nth-child(3) {
- background: #596787;
- color: #fff; }
- .fix-color .color:nth-child(3)::after {
- content: "#596787"; }
-
-.fix-contrast .color:nth-child(2) {
- background: #2b1503;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "#2b1503"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #596787;
- color: #fff; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#596787"; }
-
-.best-contrast .color:nth-child(2) {
- background: #96a2ed;
- color: #fff; }
- .best-contrast .color:nth-child(2)::after {
- content: "#96a2ed"; }
-
-.best-contrast .color:nth-child(3) {
- background: #96a2ed;
- color: #fff; }
- .best-contrast .color:nth-child(3)::after {
- content: "#96a2ed"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #906547;
- color: #fff; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#906547"; }
-
-.check-contrast .result::after {
- content: "true"; }
-
-.luminance .result::after {
- content: "0.01307, 0.1367"; }
-
-body #sidebar ul li.active > a {
- color: #2B303B !important; }
-
-body {
- background: #2B303B;
- color: #f0f2f4;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: #b5bcc9; }
- a:link, a:visited {
- color: #b5bcc9; }
- a:hover, a:active {
- color: #c4c9d4; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b,
-strong,
-label,
-th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #96B5B4;
- color: #eaf0f0;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #87aaa9;
- background: #a5c0bf;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #edf2f2;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #e7eeee; }
- .searchbox input::-moz-placeholder {
- color: #e7eeee; }
- .searchbox input:-moz-placeholder {
- color: #e7eeee; }
- .searchbox input:-ms-input-placeholder {
- color: #e7eeee; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #2B303B;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #3a4150; }
- #sidebar a,
- #sidebar i {
- color: #f0f2f4; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #c4c9d4; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(240, 242, 244, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #252932; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(240, 242, 244, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #1c1f26;
- color: white !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #f0f2f4 !important; }
- #sidebar h5.active i {
- color: #f0f2f4 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics,
- #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: white; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul,
- #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: #f0f2f4;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #20242c;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: #f0f2f4; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #f0f2f4 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #f0f2f4 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- display: inline;
- color: #f0f2f4; }
- #sidebar ul li.visited > a .read-icon:hover {
- color: #c4c9d4; }
- #sidebar ul li.active > a .read-icon, #sidebar ul li.active.visited > a .read-icon {
- display: inline;
- color: #f0f2f4; }
- #sidebar ul li.active > a .read-icon:hover, #sidebar ul li.active.visited > a .read-icon:hover {
- color: #c4c9d4; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: black; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: black; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img,
- #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border,
- #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow,
- #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #414859; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #d3d7df;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400;
- font-size: 1.05rem;
- line-height: 1.7; }
-
-h1,
-h2,
-h3,
-h4,
-h5,
-h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px;
- overflow-wrap: break-word;
- overflow: visible;
- word-break: break-word;
- white-space: normal;
- margin: 0.425rem 0 0.85rem 0; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px;
- font-size: 2.55rem; }
-
-h2 {
- letter-spacing: -2px;
- font-size: 2.15rem; }
-
-h3 {
- letter-spacing: -1px;
- font-size: 1.8rem; }
-
-h4 {
- font-size: 1.4rem; }
-
-h5 {
- font-size: 0.9rem; }
-
-h6 {
- font-size: 0.7rem; }
-
-p {
- margin: 1.7rem 0; }
-
-ul,
-ol {
- margin-top: 1.7rem;
- margin-bottom: 1.7rem; }
- ul ul,
- ul ol,
- ol ul,
- ol ol {
- margin-top: 0;
- margin-bottom: 0; }
-
-blockquote {
- border-left: 10px solid #F0F2F4;
- margin: 1.7rem 0;
- padding-left: 0.85rem; }
- blockquote p {
- font-size: 1.1rem;
- color: #cccccc; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #999999;
- font-size: 0.925rem; }
- blockquote cite:before {
- content: "\2014 \0020"; }
-
-pre {
- margin: 1.7rem 0;
- padding: 0.938rem; }
-
-code {
- vertical-align: bottom; }
-
-small {
- font-size: 0.925rem; }
-
-hr {
- border-left: none;
- border-right: none;
- border-top: none;
- margin: 1.7rem 0; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #C0C5CE;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #C0C5CE; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #C0C5CE !important;
- color: black !important;
- box-shadow: 0 3px 0 #d1d5db !important; }
- .button:hover {
- background: #d1d5db !important;
- box-shadow: 0 3px 0 #e2e5e9 !important;
- color: black !important; }
- .button:active {
- box-shadow: 0 1px 0 #e2e5e9 !important; }
- .button i {
- color: black !important; }
-
-.button-secondary {
- background: #959dad !important;
- color: black !important;
- box-shadow: 0 3px 0 #a6adba !important; }
- .button-secondary:hover {
- background: #a6adba !important;
- box-shadow: 0 3px 0 #b7bdc7 !important;
- color: black !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 #b7bdc7 !important; }
- .button-secondary i {
- color: black !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #2B303B;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem;
- color: #cccccc; }
- #top-bar a {
- color: #e6e6e6; }
- #top-bar a:hover {
- color: #f2f2f2; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: #d3d7df;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-/*# sourceMappingURL=dark_ocean.css.map */
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/dark_ocean.css.map b/themes/learn2-git-sync/css/styles/dark_ocean.css.map
deleted file mode 100644
index abe2bb1..0000000
--- a/themes/learn2-git-sync/css/styles/dark_ocean.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "dark_ocean.css",
- "sources": [
- "../../scss/styles/dark_ocean.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AYuIZ,AnB9HA,gBmB8HgB,EAWhB,KAAK,CAAC,YAAY,CnBzIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,GAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,GAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAmBO;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAoBO;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAwBoC;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GA6BwD;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAiCQ;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAkCyD;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAsC6C;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,MAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,iBAA2C,GAAI;;AvFhW3E,AACI,IADA,CACA,QAAQ,CAAC,EAAE,CAAC,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;EACtB,KAAK,EArCA,OAAO,CAqCO,UAAU,GAChC;;AyF7CL,AAAA,IAAI,CAAC;EACJ,UAAU,EzFQQ,OAAO;EyFPzB,KAAK,EzFWM,OAAyB;EyFVpC,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GAClC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EHuFc,OAAkB,GG5ErC;EAZD,AAGC,CAHA,AAGC,KAAK,EAHP,CAAC,AAIC,QAAQ,CAAC;IACT,KAAK,EHmFa,OAAkB,GGlFpC;EANF,AAQC,CARA,AAQC,MAAM,EARR,CAAC,AASC,OAAO,CAAC;IACR,KAAK,EFoYK,OAA4B,GEnYtC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAK7B;EARF,AAKE,WALS,CACV,CAAC,AAIC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbHA,OAAoB,GaIrC;;AAED,AAAA,CAAC;AACD,MAAM;AEvBN,KAAK;AMdL,EAAE,CRqCK;EACN,WAAW,EzFZO,GAAG,GyFarB;;AAED,AAAA,kBAAkB,EG9ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAwFJ,EAAE,EC5FN,KAAK,EAAL,KAAK,CA2BD,QAAQ,EA3BZ,KAAK,CAwDD,IAAI,CLfW;E3BrCX,kBAAoB,E2BsCP,GAAG,CAAC,IAAI,CAAC,IAAI;E3BjC1B,eAAiB,E2BiCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3BlB1B,UAAY,E2BkBC,GAAG,CAAC,IAAI,CAAC,IAAI,GACjC;;AEhDD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FGI,OAAO;E4FFrB,KAAK,EN+EW,OAA4B;EM9E5C,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5F0BA,IAAI;I4FzBT,MAAM,E5F0BA,IAAI,G4FpBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,OAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN6BO,OAA4B;IM5BxC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5F1BG,GAAG,G4F+BpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIR,OAAO,EAAE,YAAY,GAG5B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FLE,OAAO;E6FMzB,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FKO,KAAK;E6FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FeM,GAAG;E6FdpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CN2Yf,OAA4B,GMrKvC;EAhPD,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FAE,OAA+B,G6FSzC;IAvBL,AAgBQ,QAhBA,CAYJ,CAAC,AAII,MAAM;IAhBf,QAAQ,CAaJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EN2XL,OAA4B,GM1X/B;IAlBT,AAoBQ,QApBA,CAYJ,CAAC,AAQI,SAAS;IApBlB,QAAQ,CAaJ,CAAC,AAOI,SAAS,CAAC;MACP,KAAK,E7FPF,wBAA+B,G6FQrC;EAtBT,AAyBI,QAzBI,CAyBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CN+WpB,OAA2B,GM9WlC;EA3BL,AA6BI,QA7BI,CA6BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA/BL,AAiCI,QAjCI,CAiCJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAzEL,AAsCQ,QAtCA,CAiCJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA5CT,AA8CQ,QA9CA,CAiCJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7FjCF,wBAA+B;M6FkClC,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IApDT,AAuDY,QAvDJ,CAiCJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENiVd,OAA2B;MMhVvB,KAAK,ENmVT,KAA4B,CMnVU,UAAU,GAC/C;IA1Db,AA8DY,QA9DJ,CAiCJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBpEZ,IAAI;MiBqEF,KAAK,E7F5DT,OAAyB,C6F4DH,UAAU,GAC/B;IAjEb,AAmEY,QAnEJ,CAiCJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7FhET,OAAyB,C6FgEH,UAAU,GAC/B;EArEb,AA2EI,QA3EI,CA2EJ,EAAE,GAAC,EAAE,AAAA,OAAO,CAAC;IACT,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA9EL,AAkFQ,QAlFA,CAgFJ,EAAE,AAAA,OAAO,GAEJ,EAAE,AAAA,OAAO;EAlFlB,QAAQ,CAiFJ,EAAE,AAAA,OAAO,GACJ,EAAE,AAAA,OAAO,CAAC;IACP,OAAO,EAAE,KAAK,GACjB;EApFT,AAwFI,QAxFI,CAwFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAmJZ;IA/OL,AA+FY,QA/FJ,CAwFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EN4ST,KAA4B,GM3S3B;IAjGb,AAoGgB,QApGR,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,ENuSb,KAA4B,GMlSvB;MA1GjB,AAuGoB,QAvGZ,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAGI,MAAM,CAAC;QACJ,KAAK,ENoSjB,KAA4B,GMnSnB;IAzGrB,AA8GQ,QA9GA,CAwFJ,EAAE,AAsBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAoDjB;MAnKT,AAkHgB,QAlHR,CAwFJ,EAAE,AAsBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MApHjB,AAuHY,QAvHJ,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA9Hb,AA2HgB,QA3HR,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MA7HjB,AAgIY,QAhIJ,CAwFJ,EAAE,AAsBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE;MAhIxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAmBH,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACV,OAAO,EAAE,KAAK,GACjB;MAnIb,AAsIgB,QAtIR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAAC;QACC,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QAlJjB,AA0IoB,QA1IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAIE,CAAC,CAAC;UACE,KAAK,E7F7Hd,OAA+B;U6F8HtB,WAAW,EAAE,MAAM,GACtB;QA7IrB,AA+IoB,QA/IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CASE,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MAjJrB,AAoJgB,QApJR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,EApJxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,CAAC;QACL,UAAU,ENmPlB,OAA2B;QMlPnB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAOtB;QAjKjB,AA6JwB,QA7JhB,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,GAQH,CAAC,CACE,CAAC,EA7JzB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,GAOH,CAAC,CACE,CAAC,CAAC;UACE,KAAK,E7FhJlB,OAA+B,G6FiJrB;IA/JzB,AAqKQ,QArKA,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAAC;MACR,UAAU,EjB3KR,IAAI;MiB4KN,KAAK,E7FnKL,OAAyB,C6FmKP,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAKtB;MAhLT,AA6KY,QA7KJ,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAQP,CAAC,CAAC;QACE,KAAK,E7F1KT,OAAyB,C6F0KH,UAAU,GAC/B;IA/Kb,AAkLQ,QAlLA,CAwFJ,EAAE,CA0FE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GA0Db;MA7OT,AAqLY,QArLJ,CAwFJ,EAAE,CA0FE,EAAE,AAGG,QAAQ,GAAC,IAAI,CAAC;QACX,YAAY,EAAE,IAAI,GACrB;MAvLb,AAyLY,QAzLJ,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAQjB;QAnMb,AA6LgB,QA7LR,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAIG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAlMjB,AAqMY,QArMJ,CAwFJ,EAAE,CA0FE,EAAE,GAmBG,CAAC,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAvMb,AAyMY,QAzMJ,CAwFJ,EAAE,CA0FE,EAAE,CAuBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MAhNb,AAmNgB,QAnNR,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FvMV,OAA+B,G6F4M7B;QA1NjB,AAuNoB,QAvNZ,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENoLjB,OAA4B,GMnLnB;MAzNrB,AA+NgB,QA/NR,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,EA/N7B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7F7Nb,OAAyB,G6FkOpB;QAtOjB,AAmOoB,QAnOZ,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,AAIR,MAAM,EAnO3B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENwKjB,OAA4B,GMvKnB;MArOrB,AAyOY,QAzOJ,CAwFJ,EAAE,CA0FE,EAAE,CAuDE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAMb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENmJT,KAA2B,GM3I1B;EAZb,AAMgB,KANX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,OAAO,EANxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,MAAM,EARvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAME,QAAQ,CAAC;IACN,KAAK,EPxJL,KAAkB,GOyJrB;;ACtQjB,AAAA,KAAK,CAAC;EACF,UAAU,ElBiCC,OAAO;EkBhClB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GACnD;;AAED,AAAA,KAAK,CAAC;EAmBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FNC,KAAK;E8FOjB,UAAU,EAAE,IAAI,GAoFnB;EAzGD,AAEI,KAFC,CAED,GAAG;EAFP,KAAK,CAGD,gBAAgB,CAAC;IACb,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAhBL,AAQQ,KARH,CAED,GAAG,AAME,OAAO;IARhB,KAAK,CAGD,gBAAgB,AAKX,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IAXT,AAaQ,KAbH,CAED,GAAG,AAWE,OAAO;IAbhB,KAAK,CAGD,gBAAgB,AAUX,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAfT,AAuBI,KAvBC,CAuBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAzBL,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZpBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EAvCL,AAyCI,KAzCC,CAyCD,EAAE,GAAC,EAAE,CAAC;IACF,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZzBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA8CI,KA9CC,CA8CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EAtDL,AAwDI,KAxDC,CAwDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9F9BC,IAAI;I8F+BV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAsCrB;IAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAAC;MACC,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZrDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAwDI,KAxDC,CAwDD,IAAI,CAAC;QAmBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAsBrB;QAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAgBK;UACC,OAAO,EAAE,YAAY,GACxB;IAtFb,AAyFQ,KAzFH,CAwDD,IAAI,CAiCA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IA3FT,AA6FQ,KA7FH,CAwDD,IAAI,AAqCC,MAAM,CAAC;MACJ,UAAU,EP2TV,OAA4B,GO1T/B;IA/FT,AAiGQ,KAjGH,CAwDD,IAAI,AAyCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IAnGT,AAqGQ,KArGH,CAwDD,IAAI,AA6CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJhIa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;IIiIpF,WAAW,E9FnGG,GAAG;I8FoGjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClB1Fd,OAAO,GkB2FpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;AL1JL,AAAA,IAAI,COeC;EACJ,WAAW,ENboB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMc3F,cAAc,EAAE,QAAQ;EACxB,WAAW,EAAE,GAAG;EAChB,SAAS,EvBlBO,OAAO;EuBmBvB,WAAW,EvBlBO,GAAG,GuBmBrB;;AAGD,AAAA,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE,CAAC;EACF,WAAW,EN1BoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EM2BjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,aAAa,EAAE,UAAU;EACzB,QAAQ,EAAE,OAAO;EACjB,UAAU,EAAE,UAAU;EACtB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,QAAqB,CAAC,CAAC,CAAC,OAAqB,CAAC,CAAC,GACvD;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI;EACpB,SAAS,EvBzCK,OAAoB,GuB0ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvB7CK,OAAoB,GuB8ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvBjDK,MAAoB,GuBkDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBpDK,MAAoB,GuBqDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBvDK,MAAsB,GuBwDpC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvB1DK,MAAsB,GuB2DpC;;AAGD,AAAA,CAAC,CAAC;EACD,MAAM,EvB5DU,MAAwB,CuB4DhB,CAAC,GACzB;;AAGD,AAAA,EAAE;AACF,EAAE,CAAC;EACF,UAAU,EvBlEM,MAAwB;EuBmExC,aAAa,EvBnEG,MAAwB,GuB0ExC;EAVD,AAKC,EALC,CAKD,EAAE;EALH,EAAE,CAMD,EAAE;EALH,EAAE,CAID,EAAE;EAJH,EAAE,CAKD,EAAE,CAAC;IACF,UAAU,EAAE,CAAC;IACb,aAAa,EAAE,CAAC,GAChB;;AAIF,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpB1CN,OAAO;EoB2CxB,MAAM,EvB/EU,MAAwB,CuB+EhB,CAAC;EACzB,YAAY,EAAE,OAAmB,GAiBjC;EApBD,AAKC,UALS,CAKT,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EVEa,OAAmB,GUDrC;EARF,AAUC,UAVS,CAUT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVJa,OAAmB;IUKrC,SAAS,EAAE,QAAuB,GAKlC;IAnBF,AAgBE,UAhBQ,CAUT,IAAI,AAMF,OAAO,CAAC;MACR,OAAO,EAAE,aAAa,GACtB;;AP3EH,AAAA,GAAG,COgFC;EACH,MAAM,EvBrGU,MAAwB,CuBqGhB,CAAC;EACzB,OAAO,ExBhHa,QAAQ,GwBiH5B;;AAED,AAAA,IAAI,CAAC;EACJ,cAAc,EAAE,MAAM,GACtB;;AAGD,AAAA,KAAK,CAAC;EACL,SAAS,EAAE,QAAuB,GAClC;;AAED,AAAA,EAAE,CAAC;EACF,WAAW,EAAE,IAAI;EACjB,YAAY,EAAE,IAAI;EAClB,UAAU,EAAE,IAAI;EAChB,MAAM,EvBtHU,MAAwB,CuBsHhB,CAAC,GACzB;;AA1CD,AAAA,UAAU,CA6CC;EACV,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EACrB,QAAQ,EAAE,MAAM,GAChB;;AAED,AAAA,UAAU,GAAC,UAAU,GAAC,UAAU,CAAC;EAEhC,MAAM,EAAE,CAAC,GAuET;EAzED,AAIC,UAJS,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACnB,KAAK,EAAE,IAAI,GAqBX;IA/BF,AAaG,UAbO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AACX,OAAO,CAAC;MACR,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpB1JK,IAAI;MoB2Jd,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACV;IApBJ,AAsBG,UAtBO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AAUX,MAAM,CAAC;MACP,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBnKK,IAAI;MoBoKd,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GACf;EA7BJ,AAiCC,UAjCS,GAAC,UAAU,GAAC,UAAU,GAiC9B,CAAC,CAAC;IAEF,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EAtCF,AAwCC,UAxCS,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,CAAC;IAEb,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAjDF,AA8CE,UA9CQ,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,AAMX,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,SAAS,GAClB;EAhDH,AAmDC,UAnDS,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAExB,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAKnB;IA5DF,AAyDE,UAzDQ,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,AAMtB,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,MAAM,GACf;EA3DH,AA8DC,UA9DS,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAEnC,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAvEF,AAoEE,UApEQ,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,AAMjC,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,KAAK,GACd;;AAMH,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,ENzNoB,aAAa,EAAE,SAAS,GM0NvD;;AA1GD,AAAA,IAAI,CA4GC;EACJ,UAAU,EpBhLI,OAAO;EoBiLrB,KAAK,ET+KM,OAA2B;ES9KtC,OAAO,EAAE,WAAW;EACpB,aAAa,EAAE,GAAG,GAClB;;APtMD,AAAA,GAAG,COwMC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpBvLG,OAAO;EoBwLpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBtOD,IAAI;EoBuOpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpB/LS,OAAO;IoBgMrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAvHF,AAAA,EAAE,CA2HC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB1MP,OAAO,GoB2MxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhG3PM,OAAO;EgG4PvB,KAAK,EpBhQQ,IAAI,GoBiQjB;;AAGD,AACC,KADI,CACJ,CAAC,AAAA,YAAY,CAAC;EACb,KAAK,EAAE,IAAI,GACX;;AAHF,AAKC,KALI,CAKJ,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EACnB,KAAK,EhGtQU,OAAO,GgGuQtB;;AAIF,AAAA,gBAAgB,GAAC,eAAe,CAAC,qBAAqB,CAAC;EACtD,gBAAgB,EpBhRH,wBAAI,GoBiRjB;;AAED,AAAA,gBAAgB,GAAC,eAAe,CAAC,WAAW,CAAC;EAC5C,gBAAgB,ET6HL,OAA4B,GS5HvC;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAClD,gBAAgB,EAAE,IAAI,GACtB;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAC7D,gBAAgB,EAAE,IAAI,GACtB;;ACjSD,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFDM,OAAO,CqFCJ,UAAU;EAC7B,KAAK,ECobM,KAAK,CDpbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CE0YR,OAA4B,CF1YC,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEwYA,OAA4B,CFxYN,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYT,OAA4B,CFvYG,UAAU;IACnD,KAAK,EC+aK,KAAK,CD/awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEmYT,OAA4B,CFnYG,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECyaK,KAAK,CDzawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFMM,OAAyB,CqFNtB,UAAU;EAC7B,KAAK,ECobM,KAAK,CDpbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CE0YR,OAA4B,CF1YC,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEwYA,OAA4B,CFxYN,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYT,OAA4B,CFvYG,UAAU;IACnD,KAAK,EC+aK,KAAK,CD/awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEmYT,OAA4B,CFnYG,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECyaK,KAAK,CDzawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BWU,MAAwB,C0BXhB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtG7GK,OAAO;EsG8GtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,KAAK,Ef+RG,OAA2B,GetRtC;EAhBD,AASI,QATI,CASJ,CAAC,CAAC;IACE,KAAK,Ef4RD,OAA2B,GevRlC;IAfL,AAYQ,QAZA,CASJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EfyRL,OAA2B,GexR9B;;AAKT,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtGlJW,KAAK,GsGmJxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtGtJK,KAAK,GsGuJxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtG5JW,KAAK;IsG6JrB,IAAI,EtG7JY,MAAK,GsG8JxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtG7KC,KAAK;MsG+KjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,OAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/gold.css b/themes/learn2-git-sync/css/styles/gold.css
deleted file mode 100644
index a7fff04..0000000
--- a/themes/learn2-git-sync/css/styles/gold.css
+++ /dev/null
@@ -1,1189 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "17"; }
-
-.balance::after {
- content: "1"; }
-
-.color-block .color1 {
- background: #978e63;
- color: #fff; }
- .color-block .color1::after {
- content: "#978e63"; }
-
-.color-block .color2 {
- background: #bd9c98;
- color: #fff; }
- .color-block .color2::after {
- content: "#bd9c98"; }
-
-.fix-color .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-color .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-color .color:nth-child(3)::after {
- content: "#fff"; }
-
-.fix-contrast .color:nth-child(2) {
- background: #464644;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "#464644"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#fff"; }
-
-.best-contrast .color:nth-child(2) {
- background: #c5c2cc;
- color: #fff; }
- .best-contrast .color:nth-child(2)::after {
- content: "#c5c2cc"; }
-
-.best-contrast .color:nth-child(3) {
- background: white;
- color: #000; }
- .best-contrast .color:nth-child(3)::after {
- content: "white"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #afa577;
- color: #fff; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#afa577"; }
-
-.check-contrast .result::after {
- content: "false"; }
-
-.luminance .result::after {
- content: "0.26739, 0.36985"; }
-
-body #sidebar ul li.active > a {
- color: #333333 !important; }
-
-body {
- background: #F4F4F4;
- color: #333333;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: black; }
- a:link, a:visited {
- color: black; }
- a:hover, a:active {
- color: black; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b,
-strong,
-label,
-th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #C0B283;
- color: #f2f0e6;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #b7a872;
- background: #c9bd94;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #f4f2ea;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #f1eee3; }
- .searchbox input::-moz-placeholder {
- color: #f1eee3; }
- .searchbox input:-moz-placeholder {
- color: #f1eee3; }
- .searchbox input:-ms-input-placeholder {
- color: #f1eee3; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #c1ab8f;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #b49a78; }
- #sidebar a,
- #sidebar i {
- color: white; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #fcfbfa; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(255, 255, 255, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #c6b399; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #cdbca6;
- color: white !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #333333 !important; }
- #sidebar h5.active i {
- color: #333333 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics,
- #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: white; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul,
- #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: white;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #cab89f;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: white; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #333333 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #333333 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- display: inline;
- color: white; }
- #sidebar ul li.visited > a .read-icon:hover {
- color: #fcfbfa; }
- #sidebar ul li.active > a .read-icon, #sidebar ul li.active.visited > a .read-icon {
- display: inline;
- color: #333333; }
- #sidebar ul li.active > a .read-icon:hover, #sidebar ul li.active.visited > a .read-icon:hover {
- color: black; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: #655238; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: #967a53; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img,
- #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border,
- #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow,
- #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #dbdbdb; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #e6e6e6;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400;
- font-size: 1.05rem;
- line-height: 1.7; }
-
-h1,
-h2,
-h3,
-h4,
-h5,
-h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px;
- overflow-wrap: break-word;
- overflow: visible;
- word-break: break-word;
- white-space: normal;
- margin: 0.425rem 0 0.85rem 0; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px;
- font-size: 2.55rem; }
-
-h2 {
- letter-spacing: -2px;
- font-size: 2.15rem; }
-
-h3 {
- letter-spacing: -1px;
- font-size: 1.8rem; }
-
-h4 {
- font-size: 1.4rem; }
-
-h5 {
- font-size: 0.9rem; }
-
-h6 {
- font-size: 0.7rem; }
-
-p {
- margin: 1.7rem 0; }
-
-ul,
-ol {
- margin-top: 1.7rem;
- margin-bottom: 1.7rem; }
- ul ul,
- ul ol,
- ol ul,
- ol ol {
- margin-top: 0;
- margin-bottom: 0; }
-
-blockquote {
- border-left: 10px solid #F0F2F4;
- margin: 1.7rem 0;
- padding-left: 0.85rem; }
- blockquote p {
- font-size: 1.1rem;
- color: #666666; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #333333;
- font-size: 0.925rem; }
- blockquote cite:before {
- content: "\2014 \0020"; }
-
-pre {
- margin: 1.7rem 0;
- padding: 0.938rem; }
-
-code {
- vertical-align: bottom; }
-
-small {
- font-size: 0.925rem; }
-
-hr {
- border-left: none;
- border-right: none;
- border-top: none;
- margin: 1.7rem 0; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: black;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: black; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: black !important;
- color: white !important;
- box-shadow: 0 3px 0 black !important; }
- .button:hover {
- background: black !important;
- box-shadow: 0 3px 0 black !important;
- color: white !important; }
- .button:active {
- box-shadow: 0 1px 0 black !important; }
- .button i {
- color: white !important; }
-
-.button-secondary {
- background: black !important;
- color: white !important;
- box-shadow: 0 3px 0 black !important; }
- .button-secondary:hover {
- background: black !important;
- box-shadow: 0 3px 0 black !important;
- color: white !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 black !important; }
- .button-secondary i {
- color: white !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #F4F4F4;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem;
- color: #333333; }
- #top-bar a {
- color: #1a1a1a; }
- #top-bar a:hover {
- color: #0d0d0d; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: #1a1a1a;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-/*# sourceMappingURL=gold.css.map */
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/gold.css.map b/themes/learn2-git-sync/css/styles/gold.css.map
deleted file mode 100644
index 071b564..0000000
--- a/themes/learn2-git-sync/css/styles/gold.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "gold.css",
- "sources": [
- "../../scss/styles/gold.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AYuIZ,AnB9HA,gBmB8HgB,EAWhB,KAAK,CAAC,YAAY,CnBzIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,GAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAmBO;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAoBO;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GAwB0C;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EApIqD,OAAqB;EAqIpF,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GA6B8D;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAiCQ;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EAjJF,KAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAkC+D;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,OAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAsC6C;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,OAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,kBAA2C,GAAI;;AvFhW3E,AACI,IADA,CACA,QAAQ,CAAC,EAAE,CAAC,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;EACtB,KAAK,EArCA,OAAO,CAqCO,UAAU,GAChC;;AyF7CL,AAAA,IAAI,CAAC;EACJ,UAAU,EzFQQ,OAAO;EyFPzB,KAAK,EzFKO,OAAO;EyFJnB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GAClC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EHqGc,KAAkB,GG1FrC;EAZD,AAGC,CAHA,AAGC,KAAK,EAHP,CAAC,AAIC,QAAQ,CAAC;IACT,KAAK,EHiGa,KAAkB,GGhGpC;EANF,AAQC,CARA,AAQC,MAAM,EARR,CAAC,AASC,OAAO,CAAC;IACR,KAAK,EFiYK,KAA2B,GEhYrC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAK7B;EARF,AAKE,WALS,CACV,CAAC,AAIC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbHA,OAAoB,GaIrC;;AAED,AAAA,CAAC;AACD,MAAM;AEvBN,KAAK;AMdL,EAAE,CRqCK;EACN,WAAW,EzFZO,GAAG,GyFarB;;AAED,AAAA,kBAAkB,EG9ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAwFJ,EAAE,EC5FN,KAAK,EAAL,KAAK,CA2BD,QAAQ,EA3BZ,KAAK,CAwDD,IAAI,CLfW;E3BrCX,kBAAoB,E2BsCP,GAAG,CAAC,IAAI,CAAC,IAAI;E3BjC1B,eAAiB,E2BiCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3BlB1B,UAAY,E2BkBC,GAAG,CAAC,IAAI,CAAC,IAAI,GACjC;;AEhDD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FIG,OAAO;E4FHpB,KAAK,EN+EW,OAA4B;EM9E5C,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5F0BA,IAAI;I4FzBT,MAAM,E5F0BA,IAAI,G4FpBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,OAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN6BO,OAA4B;IM5BxC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5F1BG,GAAG,G4F+BpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIR,OAAO,EAAE,YAAY,GAG5B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FUP,OAAiC;E6FT1C,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FKO,KAAK;E6FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FeM,GAAG;E6FdpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CNwYf,OAA2B,GMlKtC;EAhPD,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FAE,KAA+B,G6FSzC;IAvBL,AAgBQ,QAhBA,CAYJ,CAAC,AAII,MAAM;IAhBf,QAAQ,CAaJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EN2XL,OAA4B,GM1X/B;IAlBT,AAoBQ,QApBA,CAYJ,CAAC,AAQI,SAAS;IApBlB,QAAQ,CAaJ,CAAC,AAOI,SAAS,CAAC;MACP,KAAK,E7FPF,wBAA+B,G6FQrC;EAtBT,AAyBI,QAzBI,CAyBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CNkXpB,OAA4B,GMjXnC;EA3BL,AA6BI,QA7BI,CA6BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA/BL,AAiCI,QAjCI,CAiCJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAzEL,AAsCQ,QAtCA,CAiCJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA5CT,AA8CQ,QA9CA,CAiCJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7FjCF,wBAA+B;M6FkClC,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IApDT,AAuDY,QAvDJ,CAiCJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENoVd,OAA4B;MMnVxB,KAAK,ENmVT,KAA4B,CMnVU,UAAU,GAC/C;IA1Db,AA8DY,QA9DJ,CAiCJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBpEZ,IAAI;MiBqEF,KAAK,E7FlER,OAAO,C6FkEc,UAAU,GAC/B;IAjEb,AAmEY,QAnEJ,CAiCJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7FtER,OAAO,C6FsEc,UAAU,GAC/B;EArEb,AA2EI,QA3EI,CA2EJ,EAAE,GAAC,EAAE,AAAA,OAAO,CAAC;IACT,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA9EL,AAkFQ,QAlFA,CAgFJ,EAAE,AAAA,OAAO,GAEJ,EAAE,AAAA,OAAO;EAlFlB,QAAQ,CAiFJ,EAAE,AAAA,OAAO,GACJ,EAAE,AAAA,OAAO,CAAC;IACP,OAAO,EAAE,KAAK,GACjB;EApFT,AAwFI,QAxFI,CAwFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAmJZ;IA/OL,AA+FY,QA/FJ,CAwFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EN4ST,KAA4B,GM3S3B;IAjGb,AAoGgB,QApGR,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,ENuSb,KAA4B,GMlSvB;MA1GjB,AAuGoB,QAvGZ,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAGI,MAAM,CAAC;QACJ,KAAK,ENoSjB,KAA4B,GMnSnB;IAzGrB,AA8GQ,QA9GA,CAwFJ,EAAE,AAsBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAoDjB;MAnKT,AAkHgB,QAlHR,CAwFJ,EAAE,AAsBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MApHjB,AAuHY,QAvHJ,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA9Hb,AA2HgB,QA3HR,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MA7HjB,AAgIY,QAhIJ,CAwFJ,EAAE,AAsBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE;MAhIxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAmBH,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACV,OAAO,EAAE,KAAK,GACjB;MAnIb,AAsIgB,QAtIR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAAC;QACC,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QAlJjB,AA0IoB,QA1IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAIE,CAAC,CAAC;UACE,KAAK,E7F7Hd,KAA+B;U6F8HtB,WAAW,EAAE,MAAM,GACtB;QA7IrB,AA+IoB,QA/IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CASE,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MAjJrB,AAoJgB,QApJR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,EApJxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,CAAC;QACL,UAAU,ENsPlB,OAA4B;QMrPpB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAOtB;QAjKjB,AA6JwB,QA7JhB,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,GAQH,CAAC,CACE,CAAC,EA7JzB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,GAOH,CAAC,CACE,CAAC,CAAC;UACE,KAAK,E7FhJlB,KAA+B,G6FiJrB;IA/JzB,AAqKQ,QArKA,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAAC;MACR,UAAU,EjB3KR,IAAI;MiB4KN,KAAK,E7FzKJ,OAAO,C6FyKU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAKtB;MAhLT,AA6KY,QA7KJ,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAQP,CAAC,CAAC;QACE,KAAK,E7FhLR,OAAO,C6FgLc,UAAU,GAC/B;IA/Kb,AAkLQ,QAlLA,CAwFJ,EAAE,CA0FE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GA0Db;MA7OT,AAqLY,QArLJ,CAwFJ,EAAE,CA0FE,EAAE,AAGG,QAAQ,GAAC,IAAI,CAAC;QACX,YAAY,EAAE,IAAI,GACrB;MAvLb,AAyLY,QAzLJ,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAQjB;QAnMb,AA6LgB,QA7LR,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAIG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAlMjB,AAqMY,QArMJ,CAwFJ,EAAE,CA0FE,EAAE,GAmBG,CAAC,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAvMb,AAyMY,QAzMJ,CAwFJ,EAAE,CA0FE,EAAE,CAuBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MAhNb,AAmNgB,QAnNR,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FvMV,KAA+B,G6F4M7B;QA1NjB,AAuNoB,QAvNZ,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENoLjB,OAA4B,GMnLnB;MAzNrB,AA+NgB,QA/NR,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,EA/N7B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FnOZ,OAAO,G6FwOH;QAtOjB,AAmOoB,QAnOZ,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,AAIR,MAAM,EAnO3B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENqKjB,KAA2B,GMpKlB;MArOrB,AAyOY,QAzOJ,CAwFJ,EAAE,CA0FE,EAAE,CAuDE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAMb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENmJT,OAA2B,GM3I1B;EAZb,AAMgB,KANX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,OAAO,EANxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,MAAM,EARvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAME,QAAQ,CAAC;IACN,KAAK,EPxJL,OAAkB,GOyJrB;;ACtQjB,AAAA,KAAK,CAAC;EACF,UAAU,ElBiCC,OAAO;EkBhClB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GACnD;;AAED,AAAA,KAAK,CAAC;EAmBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FNC,KAAK;E8FOjB,UAAU,EAAE,IAAI,GAoFnB;EAzGD,AAEI,KAFC,CAED,GAAG;EAFP,KAAK,CAGD,gBAAgB,CAAC;IACb,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAhBL,AAQQ,KARH,CAED,GAAG,AAME,OAAO;IARhB,KAAK,CAGD,gBAAgB,AAKX,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IAXT,AAaQ,KAbH,CAED,GAAG,AAWE,OAAO;IAbhB,KAAK,CAGD,gBAAgB,AAUX,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAfT,AAuBI,KAvBC,CAuBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAzBL,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZpBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EAvCL,AAyCI,KAzCC,CAyCD,EAAE,GAAC,EAAE,CAAC;IACF,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZzBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA8CI,KA9CC,CA8CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EAtDL,AAwDI,KAxDC,CAwDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9F9BC,IAAI;I8F+BV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAsCrB;IAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAAC;MACC,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZrDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAwDI,KAxDC,CAwDD,IAAI,CAAC;QAmBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAsBrB;QAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAgBK;UACC,OAAO,EAAE,YAAY,GACxB;IAtFb,AAyFQ,KAzFH,CAwDD,IAAI,CAiCA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IA3FT,AA6FQ,KA7FH,CAwDD,IAAI,AAqCC,MAAM,CAAC;MACJ,UAAU,EPwTV,OAA2B,GOvT9B;IA/FT,AAiGQ,KAjGH,CAwDD,IAAI,AAyCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IAnGT,AAqGQ,KArGH,CAwDD,IAAI,AA6CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJhIa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;IIiIpF,WAAW,E9FnGG,GAAG;I8FoGjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClB1Fd,OAAO,GkB2FpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;AL1JL,AAAA,IAAI,COeC;EACJ,WAAW,ENboB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMc3F,cAAc,EAAE,QAAQ;EACxB,WAAW,EAAE,GAAG;EAChB,SAAS,EvBlBO,OAAO;EuBmBvB,WAAW,EvBlBO,GAAG,GuBmBrB;;AAGD,AAAA,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE,CAAC;EACF,WAAW,EN1BoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EM2BjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,aAAa,EAAE,UAAU;EACzB,QAAQ,EAAE,OAAO;EACjB,UAAU,EAAE,UAAU;EACtB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,QAAqB,CAAC,CAAC,CAAC,OAAqB,CAAC,CAAC,GACvD;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI;EACpB,SAAS,EvBzCK,OAAoB,GuB0ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvB7CK,OAAoB,GuB8ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvBjDK,MAAoB,GuBkDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBpDK,MAAoB,GuBqDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBvDK,MAAsB,GuBwDpC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvB1DK,MAAsB,GuB2DpC;;AAGD,AAAA,CAAC,CAAC;EACD,MAAM,EvB5DU,MAAwB,CuB4DhB,CAAC,GACzB;;AAGD,AAAA,EAAE;AACF,EAAE,CAAC;EACF,UAAU,EvBlEM,MAAwB;EuBmExC,aAAa,EvBnEG,MAAwB,GuB0ExC;EAVD,AAKC,EALC,CAKD,EAAE;EALH,EAAE,CAMD,EAAE;EALH,EAAE,CAID,EAAE;EAJH,EAAE,CAKD,EAAE,CAAC;IACF,UAAU,EAAE,CAAC;IACb,aAAa,EAAE,CAAC,GAChB;;AAIF,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpB1CN,OAAO;EoB2CxB,MAAM,EvB/EU,MAAwB,CuB+EhB,CAAC;EACzB,YAAY,EAAE,OAAmB,GAiBjC;EApBD,AAKC,UALS,CAKT,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EVaa,OAAkB,GUZpC;EARF,AAUC,UAVS,CAUT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVOa,OAAkB;IUNpC,SAAS,EAAE,QAAuB,GAKlC;IAnBF,AAgBE,UAhBQ,CAUT,IAAI,AAMF,OAAO,CAAC;MACR,OAAO,EAAE,aAAa,GACtB;;AP3EH,AAAA,GAAG,COgFC;EACH,MAAM,EvBrGU,MAAwB,CuBqGhB,CAAC;EACzB,OAAO,ExBhHa,QAAQ,GwBiH5B;;AAED,AAAA,IAAI,CAAC;EACJ,cAAc,EAAE,MAAM,GACtB;;AAGD,AAAA,KAAK,CAAC;EACL,SAAS,EAAE,QAAuB,GAClC;;AAED,AAAA,EAAE,CAAC;EACF,WAAW,EAAE,IAAI;EACjB,YAAY,EAAE,IAAI;EAClB,UAAU,EAAE,IAAI;EAChB,MAAM,EvBtHU,MAAwB,CuBsHhB,CAAC,GACzB;;AA1CD,AAAA,UAAU,CA6CC;EACV,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EACrB,QAAQ,EAAE,MAAM,GAChB;;AAED,AAAA,UAAU,GAAC,UAAU,GAAC,UAAU,CAAC;EAEhC,MAAM,EAAE,CAAC,GAuET;EAzED,AAIC,UAJS,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACnB,KAAK,EAAE,IAAI,GAqBX;IA/BF,AAaG,UAbO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AACX,OAAO,CAAC;MACR,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpB1JK,IAAI;MoB2Jd,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACV;IApBJ,AAsBG,UAtBO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AAUX,MAAM,CAAC;MACP,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBnKK,IAAI;MoBoKd,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GACf;EA7BJ,AAiCC,UAjCS,GAAC,UAAU,GAAC,UAAU,GAiC9B,CAAC,CAAC;IAEF,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EAtCF,AAwCC,UAxCS,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,CAAC;IAEb,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAjDF,AA8CE,UA9CQ,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,AAMX,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,SAAS,GAClB;EAhDH,AAmDC,UAnDS,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAExB,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAKnB;IA5DF,AAyDE,UAzDQ,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,AAMtB,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,MAAM,GACf;EA3DH,AA8DC,UA9DS,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAEnC,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAvEF,AAoEE,UApEQ,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,AAMjC,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,KAAK,GACd;;AAMH,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,ENzNoB,aAAa,EAAE,SAAS,GM0NvD;;AA1GD,AAAA,IAAI,CA4GC;EACJ,UAAU,EpBhLI,OAAO;EoBiLrB,KAAK,ET+KM,OAA2B;ES9KtC,OAAO,EAAE,WAAW;EACpB,aAAa,EAAE,GAAG,GAClB;;APtMD,AAAA,GAAG,COwMC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpBvLG,OAAO;EoBwLpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBtOD,IAAI;EoBuOpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpB/LS,OAAO;IoBgMrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAvHF,AAAA,EAAE,CA2HC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB1MP,OAAO,GoB2MxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhGrPG,KAA6B;EgGsP1C,KAAK,EpBhQQ,IAAI,GoBiQjB;;AAGD,AACC,KADI,CACJ,CAAC,AAAA,YAAY,CAAC;EACb,KAAK,EAAE,IAAI,GACX;;AAHF,AAKC,KALI,CAKJ,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EACnB,KAAK,EhGhQO,KAA6B,GgGiQzC;;AAIF,AAAA,gBAAgB,GAAC,eAAe,CAAC,qBAAqB,CAAC;EACtD,gBAAgB,EpBhRH,wBAAI,GoBiRjB;;AAED,AAAA,gBAAgB,GAAC,eAAe,CAAC,WAAW,CAAC;EAC5C,gBAAgB,ET6HL,OAA4B,GS5HvC;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAClD,gBAAgB,EAAE,IAAI,GACtB;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAC7D,gBAAgB,EAAE,IAAI,GACtB;;ACjSD,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFKG,KAA6B,CqFLvB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,KAA2B,CFvYE,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEqYA,KAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,KAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,KAA2B,CFhYI,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFMM,KAAyB,CqFNtB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,KAA2B,CFvYE,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEqYA,KAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,KAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,KAA2B,CFhYI,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BWU,MAAwB,C0BXhB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtG7GK,OAAO;EsG8GtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,KAAK,EfkSG,OAA4B,GezRvC;EAhBD,AASI,QATI,CASJ,CAAC,CAAC;IACE,KAAK,Ef+RD,OAA4B,Ge1RnC;IAfL,AAYQ,QAZA,CASJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,Ef4RL,OAA4B,Ge3R/B;;AAKT,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtGlJW,KAAK,GsGmJxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtGtJK,KAAK,GsGuJxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtG5JW,KAAK;IsG6JrB,IAAI,EtG7JY,MAAK,GsG8JxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtG7KC,KAAK;MsG+KjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,OAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/grey.css b/themes/learn2-git-sync/css/styles/grey.css
deleted file mode 100644
index ccc32d4..0000000
--- a/themes/learn2-git-sync/css/styles/grey.css
+++ /dev/null
@@ -1,1189 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "21"; }
-
-.balance::after {
- content: "37"; }
-
-.color-block .color1 {
- background: #362616;
- color: #fff; }
- .color-block .color1::after {
- content: "#362616"; }
-
-.color-block .color2 {
- background: #646b76;
- color: #fff; }
- .color-block .color2::after {
- content: "#646b76"; }
-
-.fix-color .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-color .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-color .color:nth-child(3)::after {
- content: "#fff"; }
-
-.fix-contrast .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#fff"; }
-
-.best-contrast .color:nth-child(2) {
- background: #05060a;
- color: #fff; }
- .best-contrast .color:nth-child(2)::after {
- content: "#05060a"; }
-
-.best-contrast .color:nth-child(3) {
- background: #f6ffff;
- color: #000; }
- .best-contrast .color:nth-child(3)::after {
- content: "#f6ffff"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #856b58;
- color: #fff; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#856b58"; }
-
-.check-contrast .result::after {
- content: "false"; }
-
-.luminance .result::after {
- content: "0.02507, 0.14556"; }
-
-body #sidebar ul li.active > a {
- color: #080000 !important; }
-
-body {
- background: #f9f9f9;
- color: #080000;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: #6e0000; }
- a:link, a:visited {
- color: #6e0000; }
- a:hover, a:active {
- color: #550000; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b,
-strong,
-label,
-th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #848484;
- color: #e6e6e6;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #777777;
- background: #919191;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #e9e9e9;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #e4e4e4; }
- .searchbox input::-moz-placeholder {
- color: #e4e4e4; }
- .searchbox input:-moz-placeholder {
- color: #e4e4e4; }
- .searchbox input:-ms-input-placeholder {
- color: #e4e4e4; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #A5A5A5;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #939393; }
- #sidebar a,
- #sidebar i {
- color: white; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #fafafa; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(255, 255, 255, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #adadad; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #b7b7b7;
- color: white !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #080000 !important; }
- #sidebar h5.active i {
- color: #080000 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics,
- #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: white; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul,
- #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: white;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #b2b2b2;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: white; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #080000 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #080000 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- display: inline;
- color: white; }
- #sidebar ul li.visited > a .read-icon:hover {
- color: #fafafa; }
- #sidebar ul li.active > a .read-icon, #sidebar ul li.active.visited > a .read-icon {
- display: inline;
- color: #080000; }
- #sidebar ul li.active > a .read-icon:hover, #sidebar ul li.active.visited > a .read-icon:hover {
- color: #550000; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: #4c4c4c; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: #727272; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img,
- #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border,
- #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow,
- #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #e0e0e0; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #e6e6e6;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400;
- font-size: 1.05rem;
- line-height: 1.7; }
-
-h1,
-h2,
-h3,
-h4,
-h5,
-h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px;
- overflow-wrap: break-word;
- overflow: visible;
- word-break: break-word;
- white-space: normal;
- margin: 0.425rem 0 0.85rem 0; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px;
- font-size: 2.55rem; }
-
-h2 {
- letter-spacing: -2px;
- font-size: 2.15rem; }
-
-h3 {
- letter-spacing: -1px;
- font-size: 1.8rem; }
-
-h4 {
- font-size: 1.4rem; }
-
-h5 {
- font-size: 0.9rem; }
-
-h6 {
- font-size: 0.7rem; }
-
-p {
- margin: 1.7rem 0; }
-
-ul,
-ol {
- margin-top: 1.7rem;
- margin-bottom: 1.7rem; }
- ul ul,
- ul ol,
- ol ul,
- ol ol {
- margin-top: 0;
- margin-bottom: 0; }
-
-blockquote {
- border-left: 10px solid #F0F2F4;
- margin: 1.7rem 0;
- padding-left: 0.85rem; }
- blockquote p {
- font-size: 1.1rem;
- color: #666666; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #333333;
- font-size: 0.925rem; }
- blockquote cite:before {
- content: "\2014 \0020"; }
-
-pre {
- margin: 1.7rem 0;
- padding: 0.938rem; }
-
-code {
- vertical-align: bottom; }
-
-small {
- font-size: 0.925rem; }
-
-hr {
- border-left: none;
- border-right: none;
- border-top: none;
- margin: 1.7rem 0; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #919191;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #919191; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #919191 !important;
- color: black !important;
- box-shadow: 0 3px 0 #a1a1a1 !important; }
- .button:hover {
- background: #a1a1a1 !important;
- box-shadow: 0 3px 0 #b0b0b0 !important;
- color: black !important; }
- .button:active {
- box-shadow: 0 1px 0 #b0b0b0 !important; }
- .button i {
- color: black !important; }
-
-.button-secondary {
- background: #6b6b6b !important;
- color: white !important;
- box-shadow: 0 3px 0 #5c5c5c !important; }
- .button-secondary:hover {
- background: #5c5c5c !important;
- box-shadow: 0 3px 0 #4c4c4c !important;
- color: white !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 #4c4c4c !important; }
- .button-secondary i {
- color: white !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #f9f9f9;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem;
- color: #333333; }
- #top-bar a {
- color: #1a1a1a; }
- #top-bar a:hover {
- color: #0d0d0d; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: black;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-/*# sourceMappingURL=grey.css.map */
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/grey.css.map b/themes/learn2-git-sync/css/styles/grey.css.map
deleted file mode 100644
index 91f3305..0000000
--- a/themes/learn2-git-sync/css/styles/grey.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "grey.css",
- "sources": [
- "../../scss/styles/grey.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AYuIZ,AnB9HA,gBmB8HgB,EAWhB,KAAK,CAAC,YAAY,CnBzIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAmBO;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAoBO;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GAwB0C;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GA6B8D;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAiCQ;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAkC+D;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAsC6C;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,OAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,kBAA2C,GAAI;;AvFhW3E,AACI,IADA,CACA,QAAQ,CAAC,EAAE,CAAC,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;EACtB,KAAK,EArCA,OAAO,CAqCO,UAAU,GAChC;;AyF7CL,AAAA,IAAI,CAAC;EACJ,UAAU,EzFQQ,OAAO;EyFPzB,KAAK,EzFKO,OAAO;EyFJnB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GAClC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EHkGc,OAAmB,GGvFtC;EAZD,AAGC,CAHA,AAGC,KAAK,EAHP,CAAC,AAIC,QAAQ,CAAC;IACT,KAAK,EH8Fa,OAAmB,GG7FrC;EANF,AAQC,CARA,AAQC,MAAM,EARR,CAAC,AASC,OAAO,CAAC;IACR,KAAK,EFiYK,OAA2B,GEhYrC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAK7B;EARF,AAKE,WALS,CACV,CAAC,AAIC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbHA,OAAoB,GaIrC;;AAED,AAAA,CAAC;AACD,MAAM;AEvBN,KAAK;AMdL,EAAE,CRqCK;EACN,WAAW,EzFZO,GAAG,GyFarB;;AAED,AAAA,kBAAkB,EG9ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAwFJ,EAAE,EC5FN,KAAK,EAAL,KAAK,CA2BD,QAAQ,EA3BZ,KAAK,CAwDD,IAAI,CLfW;E3BrCX,kBAAoB,E2BsCP,GAAG,CAAC,IAAI,CAAC,IAAI;E3BjC1B,eAAiB,E2BiCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3BlB1B,UAAY,E2BkBC,GAAG,CAAC,IAAI,CAAC,IAAI,GACjC;;AEhDD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FIG,OAAO;E4FHpB,KAAK,EN+EW,OAA4B;EM9E5C,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5F0BA,IAAI;I4FzBT,MAAM,E5F0BA,IAAI,G4FpBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,OAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN6BO,OAA4B;IM5BxC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5F1BG,GAAG,G4F+BpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIR,OAAO,EAAE,YAAY,GAG5B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FLE,OAAO;E6FMzB,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FKO,KAAK;E6FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FeM,GAAG;E6FdpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CNwYf,OAA2B,GMlKtC;EAhPD,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FAE,KAA+B,G6FSzC;IAvBL,AAgBQ,QAhBA,CAYJ,CAAC,AAII,MAAM;IAhBf,QAAQ,CAaJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EN2XL,OAA4B,GM1X/B;IAlBT,AAoBQ,QApBA,CAYJ,CAAC,AAQI,SAAS;IApBlB,QAAQ,CAaJ,CAAC,AAOI,SAAS,CAAC;MACP,KAAK,E7FPF,wBAA+B,G6FQrC;EAtBT,AAyBI,QAzBI,CAyBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CNkXpB,OAA4B,GMjXnC;EA3BL,AA6BI,QA7BI,CA6BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA/BL,AAiCI,QAjCI,CAiCJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAzEL,AAsCQ,QAtCA,CAiCJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA5CT,AA8CQ,QA9CA,CAiCJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7FjCF,wBAA+B;M6FkClC,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IApDT,AAuDY,QAvDJ,CAiCJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENoVd,OAA4B;MMnVxB,KAAK,ENmVT,KAA4B,CMnVU,UAAU,GAC/C;IA1Db,AA8DY,QA9DJ,CAiCJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBpEZ,IAAI;MiBqEF,KAAK,E7FlER,OAAO,C6FkEc,UAAU,GAC/B;IAjEb,AAmEY,QAnEJ,CAiCJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7FtER,OAAO,C6FsEc,UAAU,GAC/B;EArEb,AA2EI,QA3EI,CA2EJ,EAAE,GAAC,EAAE,AAAA,OAAO,CAAC;IACT,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA9EL,AAkFQ,QAlFA,CAgFJ,EAAE,AAAA,OAAO,GAEJ,EAAE,AAAA,OAAO;EAlFlB,QAAQ,CAiFJ,EAAE,AAAA,OAAO,GACJ,EAAE,AAAA,OAAO,CAAC;IACP,OAAO,EAAE,KAAK,GACjB;EApFT,AAwFI,QAxFI,CAwFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAmJZ;IA/OL,AA+FY,QA/FJ,CAwFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EN4ST,KAA4B,GM3S3B;IAjGb,AAoGgB,QApGR,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,ENuSb,KAA4B,GMlSvB;MA1GjB,AAuGoB,QAvGZ,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAGI,MAAM,CAAC;QACJ,KAAK,ENoSjB,KAA4B,GMnSnB;IAzGrB,AA8GQ,QA9GA,CAwFJ,EAAE,AAsBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAoDjB;MAnKT,AAkHgB,QAlHR,CAwFJ,EAAE,AAsBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MApHjB,AAuHY,QAvHJ,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA9Hb,AA2HgB,QA3HR,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MA7HjB,AAgIY,QAhIJ,CAwFJ,EAAE,AAsBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE;MAhIxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAmBH,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACV,OAAO,EAAE,KAAK,GACjB;MAnIb,AAsIgB,QAtIR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAAC;QACC,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QAlJjB,AA0IoB,QA1IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAIE,CAAC,CAAC;UACE,KAAK,E7F7Hd,KAA+B;U6F8HtB,WAAW,EAAE,MAAM,GACtB;QA7IrB,AA+IoB,QA/IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CASE,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MAjJrB,AAoJgB,QApJR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,EApJxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,CAAC;QACL,UAAU,ENsPlB,OAA4B;QMrPpB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAOtB;QAjKjB,AA6JwB,QA7JhB,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,GAQH,CAAC,CACE,CAAC,EA7JzB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,GAOH,CAAC,CACE,CAAC,CAAC;UACE,KAAK,E7FhJlB,KAA+B,G6FiJrB;IA/JzB,AAqKQ,QArKA,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAAC;MACR,UAAU,EjB3KR,IAAI;MiB4KN,KAAK,E7FzKJ,OAAO,C6FyKU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAKtB;MAhLT,AA6KY,QA7KJ,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAQP,CAAC,CAAC;QACE,KAAK,E7FhLR,OAAO,C6FgLc,UAAU,GAC/B;IA/Kb,AAkLQ,QAlLA,CAwFJ,EAAE,CA0FE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GA0Db;MA7OT,AAqLY,QArLJ,CAwFJ,EAAE,CA0FE,EAAE,AAGG,QAAQ,GAAC,IAAI,CAAC;QACX,YAAY,EAAE,IAAI,GACrB;MAvLb,AAyLY,QAzLJ,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAQjB;QAnMb,AA6LgB,QA7LR,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAIG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAlMjB,AAqMY,QArMJ,CAwFJ,EAAE,CA0FE,EAAE,GAmBG,CAAC,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAvMb,AAyMY,QAzMJ,CAwFJ,EAAE,CA0FE,EAAE,CAuBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MAhNb,AAmNgB,QAnNR,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FvMV,KAA+B,G6F4M7B;QA1NjB,AAuNoB,QAvNZ,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENoLjB,OAA4B,GMnLnB;MAzNrB,AA+NgB,QA/NR,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,EA/N7B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FnOZ,OAAO,G6FwOH;QAtOjB,AAmOoB,QAnOZ,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,AAIR,MAAM,EAnO3B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENqKjB,OAA2B,GMpKlB;MArOrB,AAyOY,QAzOJ,CAwFJ,EAAE,CA0FE,EAAE,CAuDE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAMb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENmJT,OAA2B,GM3I1B;EAZb,AAMgB,KANX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,OAAO,EANxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,MAAM,EARvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAME,QAAQ,CAAC;IACN,KAAK,EPxJL,OAAkB,GOyJrB;;ACtQjB,AAAA,KAAK,CAAC;EACF,UAAU,ElBiCC,OAAO;EkBhClB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GACnD;;AAED,AAAA,KAAK,CAAC;EAmBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FNC,KAAK;E8FOjB,UAAU,EAAE,IAAI,GAoFnB;EAzGD,AAEI,KAFC,CAED,GAAG;EAFP,KAAK,CAGD,gBAAgB,CAAC;IACb,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAhBL,AAQQ,KARH,CAED,GAAG,AAME,OAAO;IARhB,KAAK,CAGD,gBAAgB,AAKX,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IAXT,AAaQ,KAbH,CAED,GAAG,AAWE,OAAO;IAbhB,KAAK,CAGD,gBAAgB,AAUX,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAfT,AAuBI,KAvBC,CAuBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAzBL,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZpBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EAvCL,AAyCI,KAzCC,CAyCD,EAAE,GAAC,EAAE,CAAC;IACF,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZzBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA8CI,KA9CC,CA8CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EAtDL,AAwDI,KAxDC,CAwDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9F9BC,IAAI;I8F+BV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAsCrB;IAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAAC;MACC,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZrDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAwDI,KAxDC,CAwDD,IAAI,CAAC;QAmBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAsBrB;QAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAgBK;UACC,OAAO,EAAE,YAAY,GACxB;IAtFb,AAyFQ,KAzFH,CAwDD,IAAI,CAiCA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IA3FT,AA6FQ,KA7FH,CAwDD,IAAI,AAqCC,MAAM,CAAC;MACJ,UAAU,EPwTV,OAA2B,GOvT9B;IA/FT,AAiGQ,KAjGH,CAwDD,IAAI,AAyCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IAnGT,AAqGQ,KArGH,CAwDD,IAAI,AA6CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJhIa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;IIiIpF,WAAW,E9FnGG,GAAG;I8FoGjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClB1Fd,OAAO,GkB2FpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;AL1JL,AAAA,IAAI,COeC;EACJ,WAAW,ENboB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMc3F,cAAc,EAAE,QAAQ;EACxB,WAAW,EAAE,GAAG;EAChB,SAAS,EvBlBO,OAAO;EuBmBvB,WAAW,EvBlBO,GAAG,GuBmBrB;;AAGD,AAAA,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE,CAAC;EACF,WAAW,EN1BoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EM2BjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,aAAa,EAAE,UAAU;EACzB,QAAQ,EAAE,OAAO;EACjB,UAAU,EAAE,UAAU;EACtB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,QAAqB,CAAC,CAAC,CAAC,OAAqB,CAAC,CAAC,GACvD;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI;EACpB,SAAS,EvBzCK,OAAoB,GuB0ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvB7CK,OAAoB,GuB8ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvBjDK,MAAoB,GuBkDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBpDK,MAAoB,GuBqDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBvDK,MAAsB,GuBwDpC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvB1DK,MAAsB,GuB2DpC;;AAGD,AAAA,CAAC,CAAC;EACD,MAAM,EvB5DU,MAAwB,CuB4DhB,CAAC,GACzB;;AAGD,AAAA,EAAE;AACF,EAAE,CAAC;EACF,UAAU,EvBlEM,MAAwB;EuBmExC,aAAa,EvBnEG,MAAwB,GuB0ExC;EAVD,AAKC,EALC,CAKD,EAAE;EALH,EAAE,CAMD,EAAE;EALH,EAAE,CAID,EAAE;EAJH,EAAE,CAKD,EAAE,CAAC;IACF,UAAU,EAAE,CAAC;IACb,aAAa,EAAE,CAAC,GAChB;;AAIF,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpB1CN,OAAO;EoB2CxB,MAAM,EvB/EU,MAAwB,CuB+EhB,CAAC;EACzB,YAAY,EAAE,OAAmB,GAiBjC;EApBD,AAKC,UALS,CAKT,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EVaa,OAAkB,GUZpC;EARF,AAUC,UAVS,CAUT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVOa,OAAkB;IUNpC,SAAS,EAAE,QAAuB,GAKlC;IAnBF,AAgBE,UAhBQ,CAUT,IAAI,AAMF,OAAO,CAAC;MACR,OAAO,EAAE,aAAa,GACtB;;AP3EH,AAAA,GAAG,COgFC;EACH,MAAM,EvBrGU,MAAwB,CuBqGhB,CAAC;EACzB,OAAO,ExBhHa,QAAQ,GwBiH5B;;AAED,AAAA,IAAI,CAAC;EACJ,cAAc,EAAE,MAAM,GACtB;;AAGD,AAAA,KAAK,CAAC;EACL,SAAS,EAAE,QAAuB,GAClC;;AAED,AAAA,EAAE,CAAC;EACF,WAAW,EAAE,IAAI;EACjB,YAAY,EAAE,IAAI;EAClB,UAAU,EAAE,IAAI;EAChB,MAAM,EvBtHU,MAAwB,CuBsHhB,CAAC,GACzB;;AA1CD,AAAA,UAAU,CA6CC;EACV,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EACrB,QAAQ,EAAE,MAAM,GAChB;;AAED,AAAA,UAAU,GAAC,UAAU,GAAC,UAAU,CAAC;EAEhC,MAAM,EAAE,CAAC,GAuET;EAzED,AAIC,UAJS,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACnB,KAAK,EAAE,IAAI,GAqBX;IA/BF,AAaG,UAbO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AACX,OAAO,CAAC;MACR,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpB1JK,IAAI;MoB2Jd,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACV;IApBJ,AAsBG,UAtBO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AAUX,MAAM,CAAC;MACP,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBnKK,IAAI;MoBoKd,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GACf;EA7BJ,AAiCC,UAjCS,GAAC,UAAU,GAAC,UAAU,GAiC9B,CAAC,CAAC;IAEF,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EAtCF,AAwCC,UAxCS,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,CAAC;IAEb,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAjDF,AA8CE,UA9CQ,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,AAMX,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,SAAS,GAClB;EAhDH,AAmDC,UAnDS,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAExB,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAKnB;IA5DF,AAyDE,UAzDQ,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,AAMtB,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,MAAM,GACf;EA3DH,AA8DC,UA9DS,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAEnC,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAvEF,AAoEE,UApEQ,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,AAMjC,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,KAAK,GACd;;AAMH,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,ENzNoB,aAAa,EAAE,SAAS,GM0NvD;;AA1GD,AAAA,IAAI,CA4GC;EACJ,UAAU,EpBhLI,OAAO;EoBiLrB,KAAK,ET+KM,OAA2B;ES9KtC,OAAO,EAAE,WAAW;EACpB,aAAa,EAAE,GAAG,GAClB;;APtMD,AAAA,GAAG,COwMC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpBvLG,OAAO;EoBwLpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBtOD,IAAI;EoBuOpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpB/LS,OAAO;IoBgMrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAvHF,AAAA,EAAE,CA2HC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB1MP,OAAO,GoB2MxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhGrPG,OAA6B;EgGsP1C,KAAK,EpBhQQ,IAAI,GoBiQjB;;AAGD,AACC,KADI,CACJ,CAAC,AAAA,YAAY,CAAC;EACb,KAAK,EAAE,IAAI,GACX;;AAHF,AAKC,KALI,CAKJ,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EACnB,KAAK,EhGhQO,OAA6B,GgGiQzC;;AAIF,AAAA,gBAAgB,GAAC,eAAe,CAAC,qBAAqB,CAAC;EACtD,gBAAgB,EpBhRH,wBAAI,GoBiRjB;;AAED,AAAA,gBAAgB,GAAC,eAAe,CAAC,WAAW,CAAC;EAC5C,gBAAgB,ET6HL,OAA4B,GS5HvC;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAClD,gBAAgB,EAAE,IAAI,GACtB;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAC7D,gBAAgB,EAAE,IAAI,GACtB;;ACjSD,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFKG,OAA6B,CqFLvB,UAAU;EAC7B,KAAK,ECobM,KAAK,CDpbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CE0YR,OAA4B,CF1YC,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEwYA,OAA4B,CFxYN,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYT,OAA4B,CFvYG,UAAU;IACnD,KAAK,EC+aK,KAAK,CD/awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEmYT,OAA4B,CFnYG,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECyaK,KAAK,CDzawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFMM,OAAyB,CqFNtB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,OAA2B,CFvYE,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEqYA,OAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,OAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,OAA2B,CFhYI,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BWU,MAAwB,C0BXhB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtG7GK,OAAO;EsG8GtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,KAAK,EfkSG,OAA4B,GezRvC;EAhBD,AASI,QATI,CASJ,CAAC,CAAC;IACE,KAAK,Ef+RD,OAA4B,Ge1RnC;IAfL,AAYQ,QAZA,CASJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,Ef4RL,OAA4B,Ge3R/B;;AAKT,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtGlJW,KAAK,GsGmJxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtGtJK,KAAK,GsGuJxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtG5JW,KAAK;IsG6JrB,IAAI,EtG7JY,MAAK,GsG8JxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtG7KC,KAAK;MsG+KjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,KAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/hoth.css b/themes/learn2-git-sync/css/styles/hoth.css
deleted file mode 100644
index 7cbcab4..0000000
--- a/themes/learn2-git-sync/css/styles/hoth.css
+++ /dev/null
@@ -1,979 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-body {
- background: #FAFAFA;
- color: #383F45;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: #383F45; }
- a:hover {
- color: #16181b; }
-
-#body-inner a:hover {
- text-decoration: underline;
- text-decoration-style: dotted; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b, strong, label, th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #747474;
- color: #fff;
- text-align: center;
- padding: 0rem 1rem 2rem 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #d9dee4;
- background: #bbc4ce;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(0, 0, 0, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(0, 0, 0, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(0, 0, 0, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #fff;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: rgba(0, 0, 0, 0.6); }
- .searchbox input::-moz-placeholder {
- color: rgba(0, 0, 0, 0.6); }
- .searchbox input:-moz-placeholder {
- color: rgba(0, 0, 0, 0.6); }
- .searchbox input:-ms-input-placeholder {
- color: rgba(0, 0, 0, 0.6); }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #383F45;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px; }
- #sidebar a {
- color: #FAFAFA; }
- #sidebar a:hover, #sidebar a.button {
- color: white; }
- #sidebar a.subtitle {
- color: rgba(250, 250, 250, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #31373d; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(250, 250, 250, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #282d31;
- color: white !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #383F45 !important; }
- #sidebar h5.active i {
- color: #383F45 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics, #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: #c7c7c7; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul, #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- opacity: 0.75;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #2d3237;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #383F45 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- color: #AAAAAA;
- display: inline; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img, #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border, #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow, #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 50px;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav:hover {
- background: #FAFAFA; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #e1e1e1;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400; }
-
-h1, h2, h3, h4, h5, h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px; }
-
-h2 {
- letter-spacing: -2px; }
-
-h3 {
- letter-spacing: -1px; }
-
-blockquote {
- border-left: 10px solid #F0F2F4; }
- blockquote p {
- font-size: 1.1rem;
- color: #999; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #666;
- font-size: 1.2rem; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #383F45;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #383F45; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #383F45;
- color: #fff;
- box-shadow: 0 3px 0 #2a3034; }
- .button:hover {
- background: #2a3034;
- box-shadow: 0 3px 0 #1d2023;
- color: #fff; }
- .button:active {
- box-shadow: 0 1px 0 #1d2023; }
-
-.button-secondary {
- background: #16181b;
- color: #fff;
- box-shadow: 0 3px 0 #08090a; }
- .button-secondary:hover {
- background: #08090a;
- box-shadow: 0 3px 0 black;
- color: #fff; }
- .button-secondary:active {
- box-shadow: 0 1px 0 black; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #FAFAFA;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: #212529;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-.version-chooser select {
- display: inline-block;
- color: #000000;
- background-color: #FFFFFF;
- border: 1px solid #666666;
- font-size: 15px;
- font-weight: regular;
- margin: 0;
-}
-
-.videoWrapper {
- position: relative;
- padding-bottom: 56.25%; /* 16:9 */
- padding-top: 25px;
- height: 0;
-}
-.videoWrapper iframe {
- position: absolute;
- top: 0;
- left: 0;
- width: 100%;
- height: 100%;
-}
-
-/*# sourceMappingURL=hoth.css.map */
diff --git a/themes/learn2-git-sync/css/styles/hoth.css.map b/themes/learn2-git-sync/css/styles/hoth.css.map
deleted file mode 100644
index 7c40b46..0000000
--- a/themes/learn2-git-sync/css/styles/hoth.css.map
+++ /dev/null
@@ -1,109 +0,0 @@
-{
- "version": 3,
- "file": "hoth.css",
- "sources": [
- "../../scss/styles/hoth.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";AwFCA,OAAO,CAAC,8EAAI;AYuHZ,AjB9GA,gBiB8GgB,EAWhB,KAAK,CAAC,YAAY,CjBzHF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AalBD,AXAA,OWAO,EAKP,iBAAiB,CXLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EWND,AXGC,OWHM,AXGL,OAAO,EWET,iBAAiB,AXFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;AELF,AAAA,IAAI,CAAC;EACJ,UAAU,EvFQQ,OAAO;EuFPzB,KAAK,EvFKO,OAAO;EuFJhB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GACrC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EvFJY,OAAO,GuFQxB;EALD,AAEC,CAFA,AAEC,MAAM,CAAC;IACP,KAAK,EAAE,OAAyB,GAChC;;AAGF,AAEE,WAFS,CACV,CAAC,AACC,MAAM,CAAC;EACP,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAC7B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CXMA,OAAoB,GWLrC;;AAED,AAAA,CAAC,EAAE,MAAM,EEbT,KAAK,EMdL,EAAE,CR2BQ;EACN,WAAW,EvFFI,GAAG,GuFGrB;;AAED,AAAA,kBAAkB,EGpClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAoFJ,EAAE,ECxFN,KAAK,EAAL,KAAK,CAyBD,QAAQ,EAzBZ,KAAK,CAsDD,IAAI,CLvBW;EzB3BX,kBAAoB,EyB4BJ,GAAG,CAAC,IAAI,CAAC,IAAI;EzBvB7B,eAAiB,EyBuBD,GAAG,CAAC,IAAI,CAAC,IAAI;EzBR7B,UAAY,EyBQI,GAAG,CAAC,IAAI,CAAC,IAAI,GACpC;;AEtCD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CbKD,IAAI,GaJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CbAD,IAAI;EaCpB,UAAU,EbOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GaG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EbAc,OAA8B,GaCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EbZI,OAAO;IaavB,UAAU,EbDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GaC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E1FED,OAAO;E0FDhB,KAAK,EdEK,IAAI;EcDd,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E1F0BA,IAAI;I0FzBT,MAAM,E1F0BA,IAAI,G0FpBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EddF,IAAI,GceT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAAuB;EACzC,UAAU,EAAE,OAAqB;EACjC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,Ed5BC,wBAAI;Ic6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EdnCC,wBAAI;IcoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,Ed1CH,wBAAI,Gc2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EdhDC,IAAI;IciDV,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E1F1BG,GAAG,G0F+BpB;IAzCL,ArCpBI,UqCoBM,CA2BN,KAAK,ArC/CJ,2BAA2B,CAAE;MqC2DtB,KAAK,Ed1DH,wBAAI,GvBCb;IqCkBL,ArCpBI,UqCoBM,CA2BN,KAAK,ArC/CJ,kBAAkB,CAAW;MqC2DtB,KAAK,Ed1DH,wBAAI,GvBCb;IqCkBL,ArCpBI,UqCoBM,CA2BN,KAAK,ArC/CJ,iBAAiB,CAAY;MqC2DtB,KAAK,Ed1DH,wBAAI,GvBCb;IqCkBL,ArCpBI,UqCoBM,CA2BN,KAAK,ArC/CJ,sBAAsB,CAAO;MqC2DtB,KAAK,Ed1DH,wBAAI,GvBCb;;AsCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;ETcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;ISrB1C,AAAA,eAAe,CAAC;MAIP,OAAO,EAAE,YAAY,GAG7B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E3FLE,OAAO;E2FMzB,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E3FKO,KAAK;E2FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E3FeM,GAAG;E2FdpB,SAAS,EAAE,IAAI,GAkMlB;EA3MD,AAWI,QAXI,CAWJ,CAAC,CAAC;IACE,KAAK,E3FZM,OAAO,G2FoBrB;IApBL,AAaQ,QAbA,CAWJ,CAAC,AAEI,MAAM,EAbf,QAAQ,CAWJ,CAAC,AAGI,OAAO,CAAC;MACL,KAAK,EAAE,KAA4B,GACtC;IAhBT,AAiBQ,QAjBA,CAWJ,CAAC,AAMI,SAAS,CAAC;MACP,KAAK,E3FlBE,wBAAO,G2FmBjB;EAnBT,AAsBI,QAtBI,CAsBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CAAC,OAAuB,GACnD;EAxBL,AA0BI,QA1BI,CA0BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA5BL,AA8BI,QA9BI,CA8BJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAtEL,AAmCQ,QAnCA,CA8BJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IAzCT,AA2CQ,QA3CA,CA8BJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E3F5CE,wBAAO;M2F6Cd,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IAjDT,AAoDY,QApDJ,CA8BJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EAAE,OAAuB;MACnC,KAAK,EAAE,KAA0B,CAAC,UAAU,GAC/C;IAvDb,AA2DY,QA3DJ,CA8BJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EfjEZ,IAAI;MekEF,KAAK,E3F/DR,OAAO,C2F+Dc,UAAU,GAC/B;IA9Db,AAgEY,QAhEJ,CA8BJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E3FnER,OAAO,C2FmEc,UAAU,GAC/B;EAlEb,AAwEI,QAxEI,CAwEJ,EAAE,GAAG,EAAE,AAAA,OAAO,CAAC;IACX,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA3EL,AA8EQ,QA9EA,CA6EJ,EAAE,AAAA,OAAO,GACH,EAAE,AAAA,OAAO,EA9EnB,QAAQ,CA6EO,EAAE,AAAA,OAAO,GACd,EAAE,AAAA,OAAO,CAAC;IACR,OAAO,EAAE,KAAK,GACjB;EAhFT,AAoFI,QApFI,CAoFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAkHZ;IA1ML,AA2FY,QA3FJ,CAoFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EAAE,OAA0B,GACpC;IA7Fb,AAgGgB,QAhGR,CAoFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,EAAE,KAA2B,GAIrC;MArGjB,AAkGoB,QAlGZ,CAoFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAEI,MAAM,CAAC;QACJ,KAAK,EAAE,KAA2B,GACrC;IApGrB,AAyGQ,QAzGA,CAoFJ,EAAE,AAqBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GA4CjB;MAtJT,AA6GgB,QA7GR,CAoFJ,EAAE,AAqBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MA/GjB,AAkHY,QAlHJ,CAoFJ,EAAE,AAqBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QAzHb,AAsHgB,QAtHR,CAoFJ,EAAE,AAqBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MAxHjB,AA2HY,QA3HJ,CAoFJ,EAAE,AAqBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE,EA3HxB,QAAQ,CAoFJ,EAAE,AAqBG,OAAO,GAkBY,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACzB,OAAO,EAAE,KAAK,GACjB;MA7Hb,AAgIgB,QAhIR,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CAAC;QACA,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QA5IjB,AAoIoB,QApIZ,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CAIC,CAAC,CAAC;UACE,OAAO,EAAE,GAAG;UACZ,WAAW,EAAE,MAAM,GACtB;QAvIrB,AAyIoB,QAzIZ,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CASC,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MA3IrB,AA8IgB,QA9IR,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeC,OAAO,EA9IxB,QAAQ,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeW,OAAO,CAAC;QACf,UAAU,EAAE,OAAuB;QACnC,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GACtB;IApJjB,AAwJQ,QAxJA,CAoFJ,EAAE,CAoEE,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;MACV,UAAU,Ef9JR,IAAI;Me+JN,KAAK,E3F5JJ,OAAO,C2F4JU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA/JT,AAiKQ,QAjKA,CAoFJ,EAAE,CA6EE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GAsCb;MAxMT,AAmKY,QAnKJ,CAoFJ,EAAE,CA6EE,EAAE,AAEG,QAAQ,GAAG,IAAI,CAAC;QACb,YAAY,EAAE,IAAI,GACrB;MArKb,AAsKY,QAtKJ,CAoFJ,EAAE,CA6EE,EAAE,CAKE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAOjB;QA/Kb,AAyKgB,QAzKR,CAoFJ,EAAE,CA6EE,EAAE,CAKE,CAAC,CAGG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MA9KjB,AAgLY,QAhLJ,CAoFJ,EAAE,CA6EE,EAAE,GAeI,CAAC,CAAC;QACA,OAAO,EAAE,KAAK,GACjB;MAlLb,AAoLY,QApLJ,CAoFJ,EAAE,CA6EE,EAAE,CAmBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MA3Lb,AA8LgB,QA9LR,CAoFJ,EAAE,CA6EE,EAAE,AA4BG,QAAQ,GACH,CAAC,CAAC,UAAU,CAAC;QACX,KAAK,E3FpMP,OAAO;Q2FqML,OAAO,EAAE,MAAM,GAClB;MAjMjB,AAoMY,QApMJ,CAoFJ,EAAE,CA6EE,EAAE,CAmCE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AChNb,AAAA,KAAK,CAAC;EACL,UAAU,EhBiCI,OAAO;EgBhCrB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GAChD;;AAED,AAAA,KAAK,CAAC;EAiBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E5FJC,KAAK;E4FKjB,UAAU,EAAE,IAAI,GA+EnB;EAlGD,AACI,KADC,CACD,GAAG,EADP,KAAK,CACI,gBAAgB,CAAC;IAClB,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAdL,AAMQ,KANH,CACD,GAAG,AAKE,OAAO,EANhB,KAAK,CACI,gBAAgB,AAKhB,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IATT,AAWQ,KAXH,CACD,GAAG,AAUE,OAAO,EAXhB,KAAK,CACI,gBAAgB,AAUhB,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAbT,AAqBI,KArBC,CAqBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAvBL,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IVlBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MUnB1C,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IVrBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MUhB1C,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EArCL,AAuCI,KAvCC,CAuCD,EAAE,GAAG,EAAE,CAAC;IACJ,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EVvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IUnB1C,AA4CI,KA5CC,CA4CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EApDL,AAsDI,KAtDC,CAsDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E5F5BC,IAAI;I4F6BV,SAAS,EAAE,IAAI;IACf,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAiCrB;IAjGL,AAiEQ,KAjEH,CAsDD,IAAI,GAWE,CAAC,CAAC;MACA,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IVlDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MUnB1C,AAsDI,KAtDC,CAsDD,IAAI,CAAC;QAkBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAkBrB;QAjGL,AAiEQ,KAjEH,CAsDD,IAAI,GAWE,CAAC,CAeK;UACA,OAAO,EAAE,YAAY,GACxB;IAlFb,AAsFQ,KAtFH,CAsDD,IAAI,AAgCC,MAAM,CAAC;MACJ,UAAU,E5FnFH,OAAO,G4FoFjB;IAxFT,AA0FQ,KA1FH,CAsDD,IAAI,AAoCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IA5FT,AA8FQ,KA9FH,CAsDD,IAAI,AAwCC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJzHa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;II0HpF,WAAW,E5F5FG,GAAG;I4F6FjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ChBnFd,OAAO,GgBoFpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;ALnJL,AAAA,IAAI,COCC;EACJ,WAAW,ENCoB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMAxF,cAAc,EAAE,QAAQ;EAC3B,WAAW,EAAE,GAAG,GAChB;;AAGD,AAAA,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC;EACtB,WAAW,ENLoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMMjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI,GACpB;;AAGD,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,ClBiBN,OAAO,GkBNxB;EAZD,AAEC,UAFS,CAET,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EAAE,IAAI,GACX;EALF,AAMC,UANS,CAMT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EAAE,IAAI;IACX,SAAS,EAAE,MAAM,GACjB;;AAXF,AAAA,UAAU,CAeC;EACP,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EAClB,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,UAAU,GAAG,UAAU,GAAG,UAAU,CAAC;EAEpC,MAAM,EAAE,CAAC,GAmET;EArED,AAIC,UAJS,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACb,KAAK,EAAE,IAAI,GAoBjB;IA9BF,AAaY,UAbF,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,AAQO,YAAY,AACR,OAAO,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,ElBjEP,IAAI;MkBkEF,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACb;IApBb,AAqBY,UArBF,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,AAQO,YAAY,AASR,MAAM,CAAC;MACJ,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,ElBzEP,IAAI;MkB0EF,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GAClB;EA5Bb,AAgCC,UAhCS,GAAG,UAAU,GAAG,UAAU,GAgCjC,CAAC,CAAC;IAEH,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EArCF,AAuCC,UAvCS,GAAG,UAAU,GAAG,UAAU,GAuCjC,UAAU,GAAG,CAAC,CAAC;IAEhB,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAInB;IA/CF,AA4CQ,UA5CE,GAAG,UAAU,GAAG,UAAU,GAuCjC,UAAU,GAAG,CAAC,AAKR,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,SAAS,GACrB;EA9CT,AAiDC,UAjDS,GAAG,UAAU,GAAG,UAAU,GAiDjC,UAAU,GAAG,UAAU,GAAG,CAAC,CAAC;IAE7B,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAInB;IAzDF,AAsDQ,UAtDE,GAAG,UAAU,GAAG,UAAU,GAiDjC,UAAU,GAAG,UAAU,GAAG,CAAC,AAKrB,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,MAAM,GAClB;EAxDT,AA2DC,UA3DS,GAAG,UAAU,GAAG,UAAU,GA2DjC,UAAU,GAAG,UAAU,GAAG,UAAU,GAAG,CAAC,CAAC;IAE1C,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAInB;IAnEF,AAgEQ,UAhEE,GAAG,UAAU,GAAG,UAAU,GA2DjC,UAAU,GAAG,UAAU,GAAG,UAAU,GAAG,CAAC,AAKlC,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,KAAK,GACjB;;AAMT,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,EN5HoB,aAAa,EAAE,SAAS,GM6HvD;;AAED,AAAA,IAAI,CAAC;EACJ,UAAU,ElBnFI,OAAO;EkBoFrB,KAAK,EAAE,OAAsB;EAC7B,OAAO,EAAE,WAAW;EACnB,aAAa,EAAE,GAAG,GACnB;;APlHD,AAAA,GAAG,COoHC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,ElB1FG,OAAO;EkB2FpB,MAAM,EAAE,GAAG,CAAC,KAAK,ClBzID,IAAI;EkB0IpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,ElBlGS,OAAO;IkBmGrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAIF,AAAA,EAAE,CAAC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,ClB7GP,OAAO,GkB8GxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,E9FlKO,OAAO;E8FmKxB,KAAK,ElBnKQ,IAAI,GkBoKjB;;AAGD,AACI,KADC,CACD,CAAC,AAAA,YAAY,CAAC;EAAE,KAAK,EAAE,IAAI,GAAI;;AADnC,AAEI,KAFC,CAED,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EAAE,KAAK,E9FzKb,OAAO,G8FyKwB;;AAIjD,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EAAE,gBAAgB,ElB7K7D,wBAAI,GkB6KgF;;AAClG,AAAA,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,OAAoB,GAAI;;AAC3F,AAAA,gBAAgB,GAAG,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,IAAI,GAAI;;AACjF,AAAA,gBAAgB,GAAG,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,IAAI,GAAI;;ACpL5F,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EXSP,UAAU,ErFLO,OAAO;EqFMxB,KAAK,ETNQ,IAAI;ESOjB,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAkB,GWRtC;EAHD,AXYC,OWZM,AXYL,MAAM,CAAC;IACP,UAAU,EAAE,OAAkB;IAC9B,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAmB;IACvC,KAAK,ETXO,IAAI,GSYhB;EWhBF,AXiBC,OWjBM,AXiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAmB,GACvC;;AWdF,AAAA,iBAAiB,CAAC;EXIjB,UAAU,ErFMM,OAAyB;EqFLzC,KAAK,ETNQ,IAAI;ESOjB,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAkB,GWHtC;EAHD,AXOC,iBWPgB,AXOf,MAAM,CAAC;IACP,UAAU,EAAE,OAAkB;IAC9B,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,KAAmB;IACvC,KAAK,ETXO,IAAI,GSYhB;EWXF,AXYC,iBWZgB,AXYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,KAAmB,GACvC;;AYnBF,AAAA,QAAQ,CAAC;EACR,MAAM,ExBYa,MAAwB,CwBZnB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EdUlB,KAAK,EAAE,GAAsB,GcL7B;EfIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IeT1C,AAAA,kBAAkB,CAAC;MdUlB,KAAK,EAAE,IAAsB,GcL7B;;AAED,AAAA,oBAAoB,CAAC;EdGpB,KAAK,EAAE,SAAsB,GcE7B;EfHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IeF1C,AAAA,oBAAoB,CAAC;MdGpB,KAAK,EAAE,IAAsB,GcE7B;;AAED,AAAA,mBAAmB,CAAC;EdJnB,KAAK,EAAE,GAAsB,GcS7B;EfVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IeK1C,AAAA,mBAAmB,CAAC;MdJnB,KAAK,EAAE,IAAsB,GcS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,ErBjCO,OAAO;EqBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EpBrCgB,MAAM;EoBsC3B,MAAM,EpBtCe,MAAM;EoBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,ErBpCQ,IAAI;EqBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,ErB5CO,OAAO,GqB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EpB/Ca,OAA6B,GoBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EpBlDa,OAA8B,GoBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACC,gBADe,CACf,KAAK,CAAC;EACL,QAAQ,EAAE,OAAO,GAIjB;EANF,AAGE,gBAHc,CACf,KAAK,CAEJ,QAAQ,CAAC;IACR,QAAQ,EAAE,OAAO,GACjB;;AAKH,AACC,eADc,CACd,CAAC,CAAC;EACD,cAAc,EAAE,MAAM,GACtB;;AAIF,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIE,UAJQ,CAET,WAAW,CAEV,EAAE,CAAC;EACF,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GAClB;;AATH,AAWE,UAXQ,CAET,WAAW,CASV,EAAE,CAAC;EACF,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAYlB;EA1BH,AAgBG,UAhBO,CAET,WAAW,CASV,EAAE,CAKD,EAAE,CAAC;IAAC,WAAW,EAAE,IAAI,GAAG;EAhB3B,AAkBG,UAlBO,CAET,WAAW,CASV,EAAE,CAOD,MAAM,EAlBT,UAAU,CAET,WAAW,CASV,EAAE,CXnBJ,KAAK,EWQL,UAAU,CAET,WAAW,CASV,EAAE,CLjCJ,EAAE,CKwCQ;IACN,eAAe,EAAE,SAAS,GAC1B;EApBJ,AAsBG,UAtBO,CAET,WAAW,CASV,EAAE,CAWD,EAAE,CAAC;IACF,WAAW,EAAE,KAAK,GAClB;;AAxBJ,AA4BE,UA5BQ,CAET,WAAW,CA0BV,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EAClB,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EP9DD,OAAO,GO+DhB;;AArCH,AAuCE,UAvCQ,CAET,WAAW,CAqCV,EAAE,CAAC,EAAE,CAAC;EACL,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACtB;;AA1CH,AA4CE,UA5CQ,CAET,WAAW,CA0CV,QAAQ,GAAG,EAAE,CAAC;EACb,UAAU,EAAE,OAAuB;EACnC,MAAM,EAAE,GAAG,CAAC,KAAK,CPxER,OAAO;EOyEhB,KAAK,EPxEI,OAAO,GO6EhB;EApDH,AAgDG,UAhDO,CAET,WAAW,CA0CV,QAAQ,GAAG,EAAE,CAIZ,EAAE,CAAC;IACF,UAAU,EPzEJ,OAAO,GO2Eb;;AAnDJ,AAsDE,UAtDQ,CAET,WAAW,CAoDV,QAAQ,GAAG,EAAE,CAAE;EACd,UAAU,EAAE,OAAqB;EACjC,MAAM,EAAE,GAAG,CAAC,KAAK,CPhFV,OAAO;EOiFd,KAAK,EPhFI,OAAO,GOoFhB;EA7DH,AA0DG,UA1DO,CAET,WAAW,CAoDV,QAAQ,GAAG,EAAE,CAIZ,EAAE,CAAC;IACF,UAAU,EPjFA,OAAO,GOkFjB;;AA5DJ,AA+DE,UA/DQ,CAET,WAAW,CA6DV,QAAQ,GAAG,EAAE,CAAC;EACb,UAAU,EAAE,OAAyB;EACrC,MAAM,EAAE,GAAG,CAAC,KAAK,CPvFN,OAAO;EOwFlB,KAAK,EPvFM,OAAO,GO2FlB;EAtEH,AAmEG,UAnEO,CAET,WAAW,CA6DV,QAAQ,GAAG,EAAE,CAIZ,EAAE,CAAC;IACF,UAAU,EPtFA,OAAO,GOuFjB;;AArEJ,AAwEE,UAxEQ,CAET,WAAW,CAsEV,QAAQ,GAAG,EAAE,CAAA;EACZ,UAAU,EAAE,OAAyB;EACrC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5FN,OAAO;EO6FlB,KAAK,EP5FQ,OAAO,GOgGpB;EA/EH,AA4EG,UA5EO,CAET,WAAW,CAsEV,QAAQ,GAAG,EAAE,CAIZ,EAAE,CAAC;IACF,UAAU,EP/FA,OAAO,GOgGjB;;AAKJ,AAAA,QAAQ,CAAC;EACL,UAAU,EpGtGK,OAAO;EoGuGtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI,GACnB;;AAGD,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;AlBxHH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;ESf1C,AAAA,QAAQ,CS4IK;IACL,KAAK,EpGlIW,KAAK,GoGmIxB;ERlJL,AAAA,KAAK,CQmJK;IACF,WAAW,EpGrIK,KAAK,GoGsIxB;;AlBrIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;ESZ1C,AAAA,QAAQ,CSoJK;IACL,KAAK,EpG1IW,KAAK;IoG2IrB,IAAI,EpG3IY,MAAK,GoG4IxB;ER3JL,AAAA,KAAK,CQ4JK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAoBnB;IArBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAMI,eANW,CAMX,KAAK,CAAC;MACF,WAAW,EpGzJC,KAAK;MoG2JjB,QAAQ,EAAE,MAAM,GACnB;IAVL,AAWI,eAXW,CAWX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAC,CAAC;MACN,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAC,EAAE;MACV,UAAU,EAAE,wBAAoB;MAChC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACjB,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACtC,GAAG,EAAE,IAAI;EACR,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,OAAsB;EAC7B,gBAAgB,ExBzJH,OAAO;EwB0JpB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB3B;EAjCD,AAiBE,kBAjBgB,AAiBf,MAAM,CAAC;IACN,gBAAgB,EAAE,OAAoB,GACvC;EAED,AAAA,GAAG,CArBL,kBAAkB,CAqBV;IACJ,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKnB;IAXD,AAQE,GARC,CArBL,kBAAkB,AA6Bb,MAAM,CAAC;MACN,gBAAgB,EAAE,OAAO,GAC1B;;AAKL,AAAA,eAAe,CAAC;EACd,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC7B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/longyearbyen.css b/themes/learn2-git-sync/css/styles/longyearbyen.css
deleted file mode 100644
index 8bfe834..0000000
--- a/themes/learn2-git-sync/css/styles/longyearbyen.css
+++ /dev/null
@@ -1,1198 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "8"; }
-
-.balance::after {
- content: "100"; }
-
-.color-block .color1 {
- background: #093f25;
- color: #fff; }
- .color-block .color1::after {
- content: "#093f25"; }
-
-.color-block .color2 {
- background: #677168;
- color: #fff; }
- .color-block .color2::after {
- content: "#677168"; }
-
-.fix-color .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-color .color:nth-child(3) {
- background: #c8d9ca;
- color: #000; }
- .fix-color .color:nth-child(3)::after {
- content: "#c8d9ca"; }
-
-.fix-contrast .color:nth-child(2) {
- background: black;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "black"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #97a498;
- color: #fff; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#97a498"; }
-
-.best-contrast .color:nth-child(2) {
- background: #fafafa;
- color: #000; }
- .best-contrast .color:nth-child(2)::after {
- content: "#fafafa"; }
-
-.best-contrast .color:nth-child(3) {
- background: #ecebeb;
- color: #000; }
- .best-contrast .color:nth-child(3)::after {
- content: "#ecebeb"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #47805e;
- color: #fff; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#47805e"; }
-
-.check-contrast .result::after {
- content: "false"; }
-
-.luminance .result::after {
- content: "0.03873, 0.15686"; }
-
-#sidebar #logo {
- color: #383F45 !important; }
- #sidebar #logo:hover {
- color: hover(#f8f8fa, #383F45); }
-
-#sidebar ul.topics > li.parent,
-#sidebar ul.topics > li.active {
- background: inherit !important; }
-
-body {
- background: #ffffff;
- color: #383F45;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: #0a0c0d; }
- a:link, a:visited {
- color: #0a0c0d; }
- a:hover, a:active {
- color: black; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b,
-strong,
-label,
-th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #f8f8fa;
- color: #cbcbda;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #e9e9ef;
- background: white;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #d9d9d9;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #bdbdd0; }
- .searchbox input::-moz-placeholder {
- color: #bdbdd0; }
- .searchbox input:-moz-placeholder {
- color: #bdbdd0; }
- .searchbox input:-ms-input-placeholder {
- color: #bdbdd0; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #f8f8fa;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #e3e3eb; }
- #sidebar a,
- #sidebar i {
- color: #6C6F73; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #2e3031; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(108, 111, 115, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid white; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(108, 111, 115, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: white;
- color: #606266 !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #383F45 !important; }
- #sidebar h5.active i {
- color: #383F45 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics,
- #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: #3b3c3e; }
- #sidebar ul.searched .search-match a {
- color: #535659; }
- #sidebar ul.searched .search-match a:hover {
- color: #3b3c3e; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul,
- #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: #6C6F73;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent,
- #sidebar ul.topics > li.active {
- background: white;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: #6C6F73; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #383F45 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #383F45 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- display: inline;
- color: #6C6F73; }
- #sidebar ul li.visited > a .read-icon:hover {
- color: #2e3031; }
- #sidebar ul li.active > a .read-icon, #sidebar ul li.active.visited > a .read-icon {
- display: inline;
- color: #383F45; }
- #sidebar ul li.active > a .read-icon:hover, #sidebar ul li.active.visited > a .read-icon:hover {
- color: black; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: #e9e9ef; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: #bdbdd0; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img,
- #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border,
- #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow,
- #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #e6e6e6; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #535659;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400;
- font-size: 1.05rem;
- line-height: 1.7; }
-
-h1,
-h2,
-h3,
-h4,
-h5,
-h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px;
- overflow-wrap: break-word;
- overflow: visible;
- word-break: break-word;
- white-space: normal;
- margin: 0.425rem 0 0.85rem 0; }
-
-p {
- margin: 1.7rem 0; }
-
-ul,
-ol {
- margin-top: 1.7rem;
- margin-bottom: 1.7rem; }
- ul ul,
- ul ol,
- ol ul,
- ol ol {
- margin-top: 0;
- margin-bottom: 0; }
-
-blockquote {
- border-left: 10px solid #F0F2F4;
- margin: 1.7rem 0;
- padding-left: 0.85rem; }
- blockquote p {
- font-size: 1.1rem;
- color: #666666; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #333333;
- font-size: 0.925rem; }
- blockquote cite:before {
- content: "\2014 \0020"; }
-
-pre {
- margin: 1.7rem 0;
- padding: 0.938rem; }
-
-code {
- vertical-align: bottom; }
-
-small {
- font-size: 0.925rem; }
-
-hr {
- border-left: none;
- border-right: none;
- border-top: none;
- margin: 1.7rem 0; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #383F45;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #383F45; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #383F45 !important;
- color: white !important;
- box-shadow: 0 3px 0 #2a3034 !important; }
- .button:hover {
- background: #2a3034 !important;
- box-shadow: 0 3px 0 #1d2023 !important;
- color: white !important; }
- .button:active {
- box-shadow: 0 1px 0 #1d2023 !important; }
- .button i {
- color: white !important; }
-
-.button-secondary {
- background: #16181b !important;
- color: white !important;
- box-shadow: 0 3px 0 #08090a !important; }
- .button-secondary:hover {
- background: #08090a !important;
- box-shadow: 0 3px 0 black !important;
- color: white !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 black !important; }
- .button-secondary i {
- color: white !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #ffffff;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem;
- color: #333333; }
- #top-bar a {
- color: #1a1a1a; }
- #top-bar a:hover {
- color: #0d0d0d; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: #212529;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-.version-chooser select {
- display: inline-block;
- color: #000000;
- background-color: #FFFFFF;
- border: 1px solid #666666;
- font-size: 15px;
- font-weight: regular;
- margin: 0;
-}
-
-.videoWrapper {
- position: relative;
- padding-bottom: 56.25%; /* 16:9 */
- padding-top: 25px;
- height: 0;
-}
-.videoWrapper iframe {
- position: absolute;
- top: 0;
- left: 0;
- width: 100%;
- height: 100%;
-}
-
-/*# sourceMappingURL=longyearbyen.css.map */
diff --git a/themes/learn2-git-sync/css/styles/longyearbyen.css.map b/themes/learn2-git-sync/css/styles/longyearbyen.css.map
deleted file mode 100644
index bec1e35..0000000
--- a/themes/learn2-git-sync/css/styles/longyearbyen.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "longyearbyen.css",
- "sources": [
- "../../scss/styles/longyearbyen.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AYuIZ,AnB9HA,gBmB8HgB,EAWhB,KAAK,CAAC,YAAY,CnBzIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,GAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,KAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAmBO;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAoBO;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAwB0C;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EAzDC,KAAsC;EA0DjD,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,OAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GA6BwD;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAA+B,IAAI,GAiCc;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAkC+D;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAsC6C;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,OAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,kBAA2C,GAAI;;AvFzV3E,AACE,QADM,CACN,KAAK,CAAC;EACJ,KAAK,EApCI,OAAO,CAoCG,UAAU,GAI9B;EANH,AAGI,QAHI,CACN,KAAK,AAEF,MAAM,CAAC;IACN,KAAK,EAAE,uBAA+B,GACvC;;AALL,AAOE,QAPM,CAON,EAAE,AAAA,OAAO,GAAG,EAAE,AAAA,OAAO;AAPvB,QAAQ,CAQN,EAAE,AAAA,OAAO,GAAG,EAAE,AAAA,OAAO,CAAC;EACpB,UAAU,EAAE,kBAAkB,GAC/B;;AyF3DH,AAAA,IAAI,CAAC;EACJ,UAAU,EzFgBQ,OAAO;EyFfzB,KAAK,EzFaO,OAAO;EyFZnB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GAClC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EHqGc,OAAkB,GG1FrC;EAZD,AAGC,CAHA,AAGC,KAAK,EAHP,CAAC,AAIC,QAAQ,CAAC;IACT,KAAK,EHiGa,OAAkB,GGhGpC;EANF,AAQC,CARA,AAQC,MAAM,EARR,CAAC,AASC,OAAO,CAAC;IACR,KAAK,EFiYK,KAA2B,GEhYrC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAK7B;EARF,AAKE,WALS,CACV,CAAC,AAIC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbHA,OAAoB,GaIrC;;AAED,AAAA,CAAC;AACD,MAAM;AEvBN,KAAK;AMdL,EAAE,CRqCK;EACN,WAAW,EzFNO,GAAG,GyFOrB;;AAED,AAAA,kBAAkB,EG9ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAwFJ,EAAE,EC5FN,KAAK,EAAL,KAAK,CA2BD,QAAQ,EA3BZ,KAAK,CAwDD,IAAI,CLfW;E3BrCX,kBAAoB,E2BsCP,GAAG,CAAC,IAAI,CAAC,IAAI;E3BjC1B,eAAiB,E2BiCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3BlB1B,UAAY,E2BkBC,GAAG,CAAC,IAAI,CAAC,IAAI,GACjC;;AEhDD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FaQ,OAAO;E4FZzB,KAAK,EN6EW,OAAuB;EM5EvC,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5FgCA,IAAI;I4F/BT,MAAM,E5FgCA,IAAI,G4F1Bb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,KAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN2BO,OAAuB;IM1BnC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5FpBG,GAAG,G4FyBpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENiBG,OAAuB,GjC1EtC;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENiBG,OAAuB,GjC1EtC;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENiBG,OAAuB,GjC1EtC;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENiBG,OAAuB,GjC1EtC;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIR,OAAO,EAAE,YAAY,GAG5B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FGE,OAAO;E6FFzB,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FaO,KAAK;E6FZjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FqBM,GAAG;E6FpBpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CNwYf,OAA2B,GMlKtC;EAhPD,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FQE,OAAO,G6FCjB;IAvBL,AAgBQ,QAhBA,CAYJ,CAAC,AAII,MAAM;IAhBf,QAAQ,CAaJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,ENwXL,OAA2B,GMvX9B;IAlBT,AAoBQ,QApBA,CAYJ,CAAC,AAQI,SAAS;IApBlB,QAAQ,CAaJ,CAAC,AAOI,SAAS,CAAC;MACP,KAAK,E7FCF,wBAAO,G6FAb;EAtBT,AAyBI,QAzBI,CAyBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CNkXpB,KAA4B,GMjXnC;EA3BL,AA6BI,QA7BI,CA6BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA/BL,AAiCI,QAjCI,CAiCJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAzEL,AAsCQ,QAtCA,CAiCJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA5CT,AA8CQ,QA9CA,CAiCJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7FzBF,wBAAO;M6F0BV,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IApDT,AAuDY,QAvDJ,CAiCJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENoVd,KAA4B;MMnVxB,KAAK,ENgVT,OAA2B,CMhVW,UAAU,GAC/C;IA1Db,AA8DY,QA9DJ,CAiCJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBpEZ,IAAI;MiBqEF,KAAK,E7F1DR,OAAO,C6F0Dc,UAAU,GAC/B;IAjEb,AAmEY,QAnEJ,CAiCJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7F9DR,OAAO,C6F8Dc,UAAU,GAC/B;EArEb,AA2EI,QA3EI,CA2EJ,EAAE,GAAC,EAAE,AAAA,OAAO,CAAC;IACT,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA9EL,AAkFQ,QAlFA,CAgFJ,EAAE,AAAA,OAAO,GAEJ,EAAE,AAAA,OAAO;EAlFlB,QAAQ,CAiFJ,EAAE,AAAA,OAAO,GACJ,EAAE,AAAA,OAAO,CAAC;IACP,OAAO,EAAE,KAAK,GACjB;EApFT,AAwFI,QAxFI,CAwFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAmJZ;IA/OL,AA+FY,QA/FJ,CAwFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,ENyST,OAA2B,GMxS1B;IAjGb,AAoGgB,QApGR,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,ENoSb,OAA2B,GM/RtB;MA1GjB,AAuGoB,QAvGZ,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAGI,MAAM,CAAC;QACJ,KAAK,ENiSjB,OAA2B,GMhSlB;IAzGrB,AA8GQ,QA9GA,CAwFJ,EAAE,AAsBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAoDjB;MAnKT,AAkHgB,QAlHR,CAwFJ,EAAE,AAsBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MApHjB,AAuHY,QAvHJ,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA9Hb,AA2HgB,QA3HR,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MA7HjB,AAgIY,QAhIJ,CAwFJ,EAAE,AAsBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE;MAhIxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAmBH,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACV,OAAO,EAAE,KAAK,GACjB;MAnIb,AAsIgB,QAtIR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAAC;QACC,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QAlJjB,AA0IoB,QA1IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAIE,CAAC,CAAC;UACE,KAAK,E7FrHd,OAAO;U6FsHE,WAAW,EAAE,MAAM,GACtB;QA7IrB,AA+IoB,QA/IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CASE,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;M7FzGrB,AAOE,QAPM,CAON,EAAE,AAAA,OAAO,GAAG,EAAE,AAAA,OAAO;MAPvB,QAAQ,CAQN,EAAE,AAAA,OAAO,GAAG,EAAE,AAAA,OAAO,C6FqGE;QACL,UAAU,ENsPlB,KAA4B;QMrPpB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAOtB;QAjKjB,AA6JwB,QA7JhB,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,GAQH,CAAC,CACE,CAAC,EA7JzB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,GAOH,CAAC,CACE,CAAC,CAAC;UACE,KAAK,E7FxIlB,OAAO,G6FyIG;IA/JzB,AAqKQ,QArKA,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAAC;MACR,UAAU,EjB3KR,IAAI;MiB4KN,KAAK,E7FjKJ,OAAO,C6FiKU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAKtB;MAhLT,AA6KY,QA7KJ,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAQP,CAAC,CAAC;QACE,KAAK,E7FxKR,OAAO,C6FwKc,UAAU,GAC/B;IA/Kb,AAkLQ,QAlLA,CAwFJ,EAAE,CA0FE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GA0Db;MA7OT,AAqLY,QArLJ,CAwFJ,EAAE,CA0FE,EAAE,AAGG,QAAQ,GAAC,IAAI,CAAC;QACX,YAAY,EAAE,IAAI,GACrB;MAvLb,AAyLY,QAzLJ,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAQjB;QAnMb,AA6LgB,QA7LR,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAIG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAlMjB,AAqMY,QArMJ,CAwFJ,EAAE,CA0FE,EAAE,GAmBG,CAAC,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAvMb,AAyMY,QAzMJ,CAwFJ,EAAE,CA0FE,EAAE,CAuBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MAhNb,AAmNgB,QAnNR,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7F/LV,OAAO,G6FoML;QA1NjB,AAuNoB,QAvNZ,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENiLjB,OAA2B,GMhLlB;MAzNrB,AA+NgB,QA/NR,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,EA/N7B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7F3NZ,OAAO,G6FgOH;QAtOjB,AAmOoB,QAnOZ,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,AAIR,MAAM,EAnO3B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENqKjB,KAA2B,GMpKlB;MArOrB,AAyOY,QAzOJ,CAwFJ,EAAE,CA0FE,EAAE,CAuDE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAMb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENsJT,OAA4B,GM9I3B;EAZb,AAMgB,KANX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,OAAO,EANxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,MAAM,EARvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAME,QAAQ,CAAC;IACN,KAAK,EPxJL,OAAkB,GOyJrB;;ACtQjB,AAAA,KAAK,CAAC;EACF,UAAU,ElBiCC,OAAO;EkBhClB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GACnD;;AAED,AAAA,KAAK,CAAC;EAmBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FEC,KAAK;E8FDjB,UAAU,EAAE,IAAI,GAoFnB;EAzGD,AAEI,KAFC,CAED,GAAG;EAFP,KAAK,CAGD,gBAAgB,CAAC;IACb,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAhBL,AAQQ,KARH,CAED,GAAG,AAME,OAAO;IARhB,KAAK,CAGD,gBAAgB,AAKX,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IAXT,AAaQ,KAbH,CAED,GAAG,AAWE,OAAO;IAbhB,KAAK,CAGD,gBAAgB,AAUX,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAfT,AAuBI,KAvBC,CAuBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAzBL,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZpBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EAvCL,AAyCI,KAzCC,CAyCD,EAAE,GAAC,EAAE,CAAC;IACF,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZzBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA8CI,KA9CC,CA8CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EAtDL,AAwDI,KAxDC,CAwDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9FxBC,IAAI;I8FyBV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAsCrB;IAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAAC;MACC,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZrDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAwDI,KAxDC,CAwDD,IAAI,CAAC;QAmBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAsBrB;QAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAgBK;UACC,OAAO,EAAE,YAAY,GACxB;IAtFb,AAyFQ,KAzFH,CAwDD,IAAI,CAiCA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IA3FT,AA6FQ,KA7FH,CAwDD,IAAI,AAqCC,MAAM,CAAC;MACJ,UAAU,EPwTV,OAA2B,GOvT9B;IA/FT,AAiGQ,KAjGH,CAwDD,IAAI,AAyCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IAnGT,AAqGQ,KArGH,CAwDD,IAAI,AA6CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJhIa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;IIiIpF,WAAW,E9F7FG,GAAG;I8F8FjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClB1Fd,OAAO,GkB2FpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;AL1JL,AAAA,IAAI,COeC;EACJ,WAAW,ENboB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMc3F,cAAc,EAAE,QAAQ;EACxB,WAAW,EAAE,GAAG;EAChB,SAAS,EvBlBO,OAAO;EuBmBvB,WAAW,EvBlBO,GAAG,GuBmBrB;;AAGD,AAAA,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE,CAAC;EACF,WAAW,EN1BoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EM2BjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,aAAa,EAAE,UAAU;EACzB,QAAQ,EAAE,OAAO;EACjB,UAAU,EAAE,UAAU;EACtB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,QAAqB,CAAC,CAAC,CAAC,OAAqB,CAAC,CAAC,GACvD;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI;EACpB,SAAS,EvBzCK,OAAoB,GuB0ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvB7CK,OAAoB,GuB8ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvBjDK,MAAoB,GuBkDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBpDK,MAAoB,GuBqDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBvDK,MAAsB,GuBwDpC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvB1DK,MAAsB,GuB2DpC;;AAGD,AAAA,CAAC,CAAC;EACD,MAAM,EvB5DU,MAAwB,CuB4DhB,CAAC,GACzB;;AAGD,AAAA,EAAE;AACF,EAAE,CAAC;EACF,UAAU,EvBlEM,MAAwB;EuBmExC,aAAa,EvBnEG,MAAwB,GuB0ExC;EAVD,AAKC,EALC,CAKD,EAAE;EALH,EAAE,CAMD,EAAE;EALH,EAAE,CAID,EAAE;EAJH,EAAE,CAKD,EAAE,CAAC;IACF,UAAU,EAAE,CAAC;IACb,aAAa,EAAE,CAAC,GAChB;;AAIF,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpB1CN,OAAO;EoB2CxB,MAAM,EvB/EU,MAAwB,CuB+EhB,CAAC;EACzB,YAAY,EAAE,OAAmB,GAiBjC;EApBD,AAKC,UALS,CAKT,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EVaa,OAAkB,GUZpC;EARF,AAUC,UAVS,CAUT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVOa,OAAkB;IUNpC,SAAS,EAAE,QAAuB,GAKlC;IAnBF,AAgBE,UAhBQ,CAUT,IAAI,AAMF,OAAO,CAAC;MACR,OAAO,EAAE,aAAa,GACtB;;AP3EH,AAAA,GAAG,COgFC;EACH,MAAM,EvBrGU,MAAwB,CuBqGhB,CAAC;EACzB,OAAO,ExBhHa,QAAQ,GwBiH5B;;AAED,AAAA,IAAI,CAAC;EACJ,cAAc,EAAE,MAAM,GACtB;;AAGD,AAAA,KAAK,CAAC;EACL,SAAS,EAAE,QAAuB,GAClC;;AAED,AAAA,EAAE,CAAC;EACF,WAAW,EAAE,IAAI;EACjB,YAAY,EAAE,IAAI;EAClB,UAAU,EAAE,IAAI;EAChB,MAAM,EvBtHU,MAAwB,CuBsHhB,CAAC,GACzB;;AA1CD,AAAA,UAAU,CA6CC;EACV,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EACrB,QAAQ,EAAE,MAAM,GAChB;;AAED,AAAA,UAAU,GAAC,UAAU,GAAC,UAAU,CAAC;EAEhC,MAAM,EAAE,CAAC,GAuET;EAzED,AAIC,UAJS,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACnB,KAAK,EAAE,IAAI,GAqBX;IA/BF,AAaG,UAbO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AACX,OAAO,CAAC;MACR,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpB1JK,IAAI;MoB2Jd,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACV;IApBJ,AAsBG,UAtBO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AAUX,MAAM,CAAC;MACP,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBnKK,IAAI;MoBoKd,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GACf;EA7BJ,AAiCC,UAjCS,GAAC,UAAU,GAAC,UAAU,GAiC9B,CAAC,CAAC;IAEF,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EAtCF,AAwCC,UAxCS,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,CAAC;IAEb,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAjDF,AA8CE,UA9CQ,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,AAMX,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,SAAS,GAClB;EAhDH,AAmDC,UAnDS,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAExB,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAKnB;IA5DF,AAyDE,UAzDQ,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,AAMtB,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,MAAM,GACf;EA3DH,AA8DC,UA9DS,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAEnC,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAvEF,AAoEE,UApEQ,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,AAMjC,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,KAAK,GACd;;AAMH,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,ENzNoB,aAAa,EAAE,SAAS,GM0NvD;;AA1GD,AAAA,IAAI,CA4GC;EACJ,UAAU,EpBhLI,OAAO;EoBiLrB,KAAK,ET+KM,OAA2B;ES9KtC,OAAO,EAAE,WAAW;EACpB,aAAa,EAAE,GAAG,GAClB;;APtMD,AAAA,GAAG,COwMC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpBvLG,OAAO;EoBwLpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBtOD,IAAI;EoBuOpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpB/LS,OAAO;IoBgMrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAvHF,AAAA,EAAE,CA2HC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB1MP,OAAO,GoB2MxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhGvPO,OAAO;EgGwPxB,KAAK,EpBhQQ,IAAI,GoBiQjB;;AAGD,AACC,KADI,CACJ,CAAC,AAAA,YAAY,CAAC;EACb,KAAK,EAAE,IAAI,GACX;;AAHF,AAKC,KALI,CAKJ,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EACnB,KAAK,EhGlQW,OAAO,GgGmQvB;;AAIF,AAAA,gBAAgB,GAAC,eAAe,CAAC,qBAAqB,CAAC;EACtD,gBAAgB,EpBhRH,wBAAI,GoBiRjB;;AAED,AAAA,gBAAgB,GAAC,eAAe,CAAC,WAAW,CAAC;EAC5C,gBAAgB,ET6HL,OAA4B,GS5HvC;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAClD,gBAAgB,EAAE,IAAI,GACtB;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAC7D,gBAAgB,EAAE,IAAI,GACtB;;ACjSD,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFGO,OAAO,CqFHL,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,OAA2B,CFvYE,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEqYA,OAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,OAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,OAA2B,CFhYI,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFcM,OAAyB,CqFdtB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,OAA2B,CFvYE,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEqYA,OAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,KAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,KAA2B,CFhYI,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BWU,MAAwB,C0BXhB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtGrGK,OAAO;EsGsGtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,KAAK,EfkSG,OAA4B,GezRvC;EAhBD,AASI,QATI,CASJ,CAAC,CAAC;IACE,KAAK,Ef+RD,OAA4B,Ge1RnC;IAfL,AAYQ,QAZA,CASJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,Ef4RL,OAA4B,Ge3R/B;;AAKT,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtG1IW,KAAK,GsG2IxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtG9IK,KAAK,GsG+IxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtGpJW,KAAK;IsGqJrB,IAAI,EtGrJY,MAAK,GsGsJxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtGrKC,KAAK;MsGuKjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,OAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/metal.css b/themes/learn2-git-sync/css/styles/metal.css
deleted file mode 100644
index 9d7f250..0000000
--- a/themes/learn2-git-sync/css/styles/metal.css
+++ /dev/null
@@ -1,1113 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "13"; }
-
-.balance::after {
- content: "33"; }
-
-.color-block .color1 {
- background: #070d0e;
- color: #fff; }
- .color-block .color1::after {
- content: "#070d0e"; }
-
-.color-block .color2 {
- background: #64646e;
- color: #fff; }
- .color-block .color2::after {
- content: "#64646e"; }
-
-.fix-color .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-color .color:nth-child(3) {
- background: #d6d5e9;
- color: #000; }
- .fix-color .color:nth-child(3)::after {
- content: "#d6d5e9"; }
-
-.fix-contrast .color:nth-child(2) {
- background: #050909;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "#050909"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #cfcfe1;
- color: #000; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#cfcfe1"; }
-
-.best-contrast .color:nth-child(2) {
- background: #07120e;
- color: #fff; }
- .best-contrast .color:nth-child(2)::after {
- content: "#07120e"; }
-
-.best-contrast .color:nth-child(3) {
- background: #8ffff6;
- color: #000; }
- .best-contrast .color:nth-child(3)::after {
- content: "#8ffff6"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #527272;
- color: #fff; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#527272"; }
-
-.check-contrast .result::after {
- content: "false"; }
-
-.luminance .result::after {
- content: "0.00592, 0.13006"; }
-
-body {
- background: #ffffff;
- color: #000000;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: black; }
- a:link, a:visited {
- color: black; }
- a:hover, a:active {
- color: black; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b, strong, label, th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #bd8c7d;
- color: #f2e8e5;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #b47d6c;
- background: #c69b8e;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #f4ebe8;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #f0e5e2; }
- .searchbox input::-moz-placeholder {
- color: #f0e5e2; }
- .searchbox input:-moz-placeholder {
- color: #f0e5e2; }
- .searchbox input:-ms-input-placeholder {
- color: #f0e5e2; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #49494b;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #5b5b5d; }
- #sidebar a,
- #sidebar i {
- color: #e8e8e6; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #c3c3be; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(232, 232, 230, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #414143; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(232, 232, 230, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #373739;
- color: #f4f4f3 !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #000000 !important; }
- #sidebar h5.active i {
- color: #000000 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics, #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: white; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul, #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: #e8e8e6;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #3c3c3e;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: #e8e8e6; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #000000 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #000000 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- color: #b47d6c;
- display: inline; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: black; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: #171717; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img, #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border, #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow, #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #e8e8e6; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #d0d0cb;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400; }
-
-h1, h2, h3, h4, h5, h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px; }
-
-h2 {
- letter-spacing: -2px; }
-
-h3 {
- letter-spacing: -1px; }
-
-blockquote {
- border-left: 10px solid #F0F2F4; }
- blockquote p {
- font-size: 1.1rem;
- color: #666666; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #333333;
- font-size: 1.2rem; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #b47d6c;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #b47d6c; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #b47d6c !important;
- color: black !important;
- box-shadow: 0 3px 0 #be8f80 !important; }
- .button:hover {
- background: #be8f80 !important;
- box-shadow: 0 3px 0 #c9a195 !important;
- color: black !important; }
- .button:active {
- box-shadow: 0 1px 0 #c9a195 !important; }
- .button i {
- color: black !important; }
-
-.button-secondary {
- background: #8c5847 !important;
- color: white !important;
- box-shadow: 0 3px 0 #784b3d !important; }
- .button-secondary:hover {
- background: #784b3d !important;
- box-shadow: 0 3px 0 #643e33 !important;
- color: white !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 #643e33 !important; }
- .button-secondary i {
- color: white !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #e8e8e6;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
- #top-github-link a {
- color: #0d0d0d; }
- #top-github-link a:hover {
- color: black; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4;
- color: black; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: black;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-/*# sourceMappingURL=metal.css.map */
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/metal.css.map b/themes/learn2-git-sync/css/styles/metal.css.map
deleted file mode 100644
index e655557..0000000
--- a/themes/learn2-git-sync/css/styles/metal.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "metal.css",
- "sources": [
- "../../scss/styles/metal.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AY8HZ,AnBrHA,gBmBqHgB,EAmBhB,KAAK,CAAC,YAAY,CnBxIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAmBO;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAoBO;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAwB0C;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EAzDC,OAAsC;EA0DjD,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GA6B8D;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAiCQ;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAkC+D;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAsC6C;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,OAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,kBAA2C,GAAI;;AE1Y3E,AAAA,IAAI,CAAC;EACJ,UAAU,EzFOM,OAAO;EyFNvB,KAAK,EzFKO,OAAO;EyFJhB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GACrC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EF0YM,KAA2B,GEjYtC;EAVD,AAEC,CAFA,AAEC,KAAK,EAFP,CAAC,AAGC,QAAQ,CAAC;IACT,KAAK,EFuYK,KAA2B,GEtYrC;EALF,AAMC,CANA,AAMC,MAAM,EANR,CAAC,AAOC,OAAO,CAAC;IACR,KAAK,EFmYK,KAA2B,GElYrC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAI7B;EAPF,AAIE,WAJS,CACV,CAAC,AAGC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbAA,OAAoB,GaCrC;;AAED,AAAA,CAAC,EAAE,MAAM,EEnBT,KAAK,EMdL,EAAE,CRiCQ;EACN,WAAW,EzFRI,GAAG,GyFSrB;;AAED,AAAA,kBAAkB,EG1ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAqFJ,EAAE,ECzFN,KAAK,EAAL,KAAK,CAyBD,QAAQ,EAzBZ,KAAK,CAsDD,IAAI,CLjBW;E3BjCX,kBAAoB,E2BkCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3B7B7B,eAAiB,E2B6BD,GAAG,CAAC,IAAI,CAAC,IAAI;E3Bd7B,UAAY,E2BcI,GAAG,CAAC,IAAI,CAAC,IAAI,GACpC;;AE5CD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FED,OAAO;E4FDhB,KAAK,EN+EW,OAA4B;EM9E5C,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5F0BA,IAAI;I4FzBT,MAAM,E5F0BA,IAAI,G4FpBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,OAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN6BO,OAA4B;IM5BxC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5F1BG,GAAG,G4F+BpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIP,OAAO,EAAE,YAAY,GAG7B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FLE,OAAO;E6FMzB,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FKO,KAAK;E6FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FeM,GAAG;E6FdpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CN2Yf,OAA4B,GMjMvC;EApND,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FdM,OAAO,G6FqBrB;IArBL,AAeQ,QAfA,CAYJ,CAAC,AAGI,MAAM;IAff,QAAQ,CAaJ,CAAC,AAEI,MAAM,CAAC;MACJ,KAAK,EN4XL,OAA4B,GM3X/B;IAjBT,AAkBQ,QAlBA,CAYJ,CAAC,AAMI,SAAS;IAlBlB,QAAQ,CAaJ,CAAC,AAKI,SAAS,CAAC;MACP,KAAK,E7FnBE,wBAAO,G6FoBjB;EApBT,AAuBI,QAvBI,CAuBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CNiXpB,OAA2B,GMhXlC;EAzBL,AA2BI,QA3BI,CA2BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA7BL,AA+BI,QA/BI,CA+BJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAvEL,AAoCQ,QApCA,CA+BJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA1CT,AA4CQ,QA5CA,CA+BJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7F7CE,wBAAO;M6F8Cd,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IAlDT,AAqDY,QArDJ,CA+BJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENmVd,OAA2B;MMlVvB,KAAK,ENqVT,OAA4B,CMrVU,UAAU,GAC/C;IAxDb,AA4DY,QA5DJ,CA+BJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBlEZ,IAAI;MiBmEF,KAAK,E7FhER,OAAO,C6FgEc,UAAU,GAC/B;IA/Db,AAiEY,QAjEJ,CA+BJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7FpER,OAAO,C6FoEc,UAAU,GAC/B;EAnEb,AAyEI,QAzEI,CAyEJ,EAAE,GAAG,EAAE,AAAA,OAAO,CAAC;IACX,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA5EL,AA+EQ,QA/EA,CA8EJ,EAAE,AAAA,OAAO,GACH,EAAE,AAAA,OAAO,EA/EnB,QAAQ,CA8EO,EAAE,AAAA,OAAO,GACd,EAAE,AAAA,OAAO,CAAC;IACR,OAAO,EAAE,KAAK,GACjB;EAjFT,AAqFI,QArFI,CAqFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GA0HZ;IAnNL,AA4FY,QA5FJ,CAqFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EN+ST,KAA4B,GM9S3B;IA9Fb,AAiGgB,QAjGR,CAqFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,EN0Sb,KAA4B,GMtSvB;MAtGjB,AAmGoB,QAnGZ,CAqFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAEI,MAAM,CAAC;QACJ,KAAK,ENwSjB,KAA4B,GMvSnB;IArGrB,AA0GQ,QA1GA,CAqFJ,EAAE,AAqBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAiDjB;MA5JT,AA8GgB,QA9GR,CAqFJ,EAAE,AAqBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAhHjB,AAmHY,QAnHJ,CAqFJ,EAAE,AAqBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA1Hb,AAuHgB,QAvHR,CAqFJ,EAAE,AAqBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MAzHjB,AA4HY,QA5HJ,CAqFJ,EAAE,AAqBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE,EA5HxB,QAAQ,CAqFJ,EAAE,AAqBG,OAAO,GAkBY,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACzB,OAAO,EAAE,KAAK,GACjB;MA9Hb,AAiIgB,QAjIR,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CAAC;QACA,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QA7IjB,AAqIoB,QArIZ,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CAIC,CAAC,CAAC;UACE,KAAK,E7FtIV,OAAO;U6FuIF,WAAW,EAAE,MAAM,GACtB;QAxIrB,AA0IoB,QA1IZ,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CASC,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MA5IrB,AA+IgB,QA/IR,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeC,OAAO,EA/IxB,QAAQ,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeW,OAAO,CAAC;QACf,UAAU,ENyPlB,OAA2B;QMxPnB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAMtB;QA1JjB,AAsJwB,QAtJhB,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeC,OAAO,GAMF,CAAC,CACC,CAAC,EAtJzB,QAAQ,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeW,OAAO,GAMZ,CAAC,CACC,CAAC,CAAC;UACE,KAAK,E7FvJd,OAAO,G6FwJD;IAxJzB,AA8JQ,QA9JA,CAqFJ,EAAE,CAyEE,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;MACV,UAAU,EjBpKR,IAAI;MiBqKN,KAAK,E7FlKJ,OAAO,C6FkKU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAItB;MAxKT,AAqKY,QArKJ,CAqFJ,EAAE,CAyEE,EAAE,AAAA,OAAO,GAAG,CAAC,CAOT,CAAC,CAAC;QACE,KAAK,E7FxKR,OAAO,C6FwKc,UAAU,GAC/B;IAvKb,AA0KQ,QA1KA,CAqFJ,EAAE,CAqFE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GAsCb;MAjNT,AA4KY,QA5KJ,CAqFJ,EAAE,CAqFE,EAAE,AAEG,QAAQ,GAAG,IAAI,CAAC;QACb,YAAY,EAAE,IAAI,GACrB;MA9Kb,AA+KY,QA/KJ,CAqFJ,EAAE,CAqFE,EAAE,CAKE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAOjB;QAxLb,AAkLgB,QAlLR,CAqFJ,EAAE,CAqFE,EAAE,CAKE,CAAC,CAGG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAvLjB,AAyLY,QAzLJ,CAqFJ,EAAE,CAqFE,EAAE,GAeI,CAAC,CAAC;QACA,OAAO,EAAE,KAAK,GACjB;MA3Lb,AA6LY,QA7LJ,CAqFJ,EAAE,CAqFE,EAAE,CAmBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MApMb,AAuMgB,QAvMR,CAqFJ,EAAE,CAqFE,EAAE,AA4BG,QAAQ,GACH,CAAC,CAAC,UAAU,CAAC;QACX,KAAK,E7FnMX,OAA2C;Q6FoMrC,OAAO,EAAE,MAAM,GAClB;MA1MjB,AA6MY,QA7MJ,CAqFJ,EAAE,CAqFE,EAAE,CAmCE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAKb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENgLT,KAA2B,GMzK1B;EAXb,AAKgB,KALX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAEE,OAAO,EALxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,MAAM,EANvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,QAAQ,CAAC;IACN,KAAK,EP1HL,OAAkB,GO2HrB;;ACxOjB,AAAA,KAAK,CAAC;EACL,UAAU,ElBiCI,OAAO;EkBhCrB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GAChD;;AAED,AAAA,KAAK,CAAC;EAiBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FJC,KAAK;E8FKjB,UAAU,EAAE,IAAI,GAkFnB;EArGD,AACI,KADC,CACD,GAAG,EADP,KAAK,CACI,gBAAgB,CAAC;IAClB,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAdL,AAMQ,KANH,CACD,GAAG,AAKE,OAAO,EANhB,KAAK,CACI,gBAAgB,AAKhB,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IATT,AAWQ,KAXH,CACD,GAAG,AAUE,OAAO,EAXhB,KAAK,CACI,gBAAgB,AAUhB,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAbT,AAqBI,KArBC,CAqBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAvBL,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZlBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZrBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EArCL,AAuCI,KAvCC,CAuCD,EAAE,GAAG,EAAE,CAAC;IACJ,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA4CI,KA5CC,CA4CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EApDL,AAsDI,KAtDC,CAsDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9F5BC,IAAI;I8F6BV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAoCrB;IApGL,AAiEQ,KAjEH,CAsDD,IAAI,GAWE,CAAC,CAAC;MACA,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZlDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAsDI,KAtDC,CAsDD,IAAI,CAAC;QAkBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAqBrB;QApGL,AAiEQ,KAjEH,CAsDD,IAAI,GAWE,CAAC,CAeK;UACA,OAAO,EAAE,YAAY,GACxB;IAlFb,AAqFQ,KArFH,CAsDD,IAAI,CA+BA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IAvFT,AAyFQ,KAzFH,CAsDD,IAAI,AAmCC,MAAM,CAAC;MACJ,UAAU,E9FtFH,OAAO,G8FuFjB;IA3FT,AA6FQ,KA7FH,CAsDD,IAAI,AAuCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IA/FT,AAiGQ,KAjGH,CAsDD,IAAI,AA2CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJ5Ha,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;II6HpF,WAAW,E9F/FG,GAAG;I8FgGjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClBtFd,OAAO,GkBuFpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;ALtJL,AAAA,IAAI,COCC;EACJ,WAAW,ENCoB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMAxF,cAAc,EAAE,QAAQ;EAC3B,WAAW,EAAE,GAAG,GAChB;;AAGD,AAAA,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC;EACtB,WAAW,ENLoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMMjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI,GACpB;;AAGD,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpBiBN,OAAO,GoBNxB;EAZD,AAEC,UAFS,CAET,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EV2Ea,OAAkB,GU1EpC;EALF,AAMC,UANS,CAMT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVsEa,OAAkB;IUrEpC,SAAS,EAAE,MAAM,GACjB;;AAXF,AAAA,UAAU,CAeC;EACP,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EAClB,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,UAAU,GAAG,UAAU,GAAG,UAAU,CAAC;EAEpC,MAAM,EAAE,CAAC,GAmET;EArED,AAIC,UAJS,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACb,KAAK,EAAE,IAAI,GAoBjB;IA9BF,AAaY,UAbF,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,AAQO,YAAY,AACR,OAAO,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBjEP,IAAI;MoBkEF,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACb;IApBb,AAqBY,UArBF,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,AAQO,YAAY,AASR,MAAM,CAAC;MACJ,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBzEP,IAAI;MoB0EF,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GAClB;EA5Bb,AAgCC,UAhCS,GAAG,UAAU,GAAG,UAAU,GAgCjC,CAAC,CAAC;IAEH,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EArCF,AAuCC,UAvCS,GAAG,UAAU,GAAG,UAAU,GAuCjC,UAAU,GAAG,CAAC,CAAC;IAEhB,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAInB;IA/CF,AA4CQ,UA5CE,GAAG,UAAU,GAAG,UAAU,GAuCjC,UAAU,GAAG,CAAC,AAKR,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,SAAS,GACrB;EA9CT,AAiDC,UAjDS,GAAG,UAAU,GAAG,UAAU,GAiDjC,UAAU,GAAG,UAAU,GAAG,CAAC,CAAC;IAE7B,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAInB;IAzDF,AAsDQ,UAtDE,GAAG,UAAU,GAAG,UAAU,GAiDjC,UAAU,GAAG,UAAU,GAAG,CAAC,AAKrB,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,MAAM,GAClB;EAxDT,AA2DC,UA3DS,GAAG,UAAU,GAAG,UAAU,GA2DjC,UAAU,GAAG,UAAU,GAAG,UAAU,GAAG,CAAC,CAAC;IAE1C,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAInB;IAnEF,AAgEQ,UAhEE,GAAG,UAAU,GAAG,UAAU,GA2DjC,UAAU,GAAG,UAAU,GAAG,UAAU,GAAG,CAAC,AAKlC,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,KAAK,GACjB;;AAMT,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,EN5HoB,aAAa,EAAE,SAAS,GM6HvD;;AAED,AAAA,IAAI,CAAC;EACJ,UAAU,EpBnFI,OAAO;EoBoFrB,KAAK,ET4QM,OAA2B;ES3QtC,OAAO,EAAE,WAAW;EACnB,aAAa,EAAE,GAAG,GACnB;;AP5GD,AAAA,GAAG,CO8GC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpB1FG,OAAO;EoB2FpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBzID,IAAI;EoB0IpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpBlGS,OAAO;IoBmGrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAIF,AAAA,EAAE,CAAC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB7GP,OAAO,GoB8GxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhGxJG,OAA2C;EgGyJxD,KAAK,EpBnKQ,IAAI,GoBoKjB;;AAGD,AACI,KADC,CACD,CAAC,AAAA,YAAY,CAAC;EAAE,KAAK,EAAE,IAAI,GAAI;;AADnC,AAEI,KAFC,CAED,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EAAE,KAAK,EhG/JjB,OAA2C,GgG+JR;;AAIjD,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EAAE,gBAAgB,EpB7K7D,wBAAI,GoB6KgF;;AAClG,AAAA,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAAE,gBAAgB,ETmOrD,OAA4B,GSnOmD;;AAC3F,AAAA,gBAAgB,GAAG,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,IAAI,GAAI;;AACjF,AAAA,gBAAgB,GAAG,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,IAAI,GAAI;;ACpL5F,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFKG,OAA2C,CqFLrC,UAAU;EAC7B,KAAK,ECobM,KAAK,CDpbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CE0YR,OAA4B,CF1YC,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEwYA,OAA4B,CFxYN,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYT,OAA4B,CFvYG,UAAU;IACnD,KAAK,EC+aK,KAAK,CD/awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEmYT,OAA4B,CFnYG,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECyaK,KAAK,CDzawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFMM,OAAyB,CqFNtB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,OAA2B,CFvYE,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEqYA,OAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,OAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,OAA2B,CFhYI,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BYa,MAAwB,C0BZnB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtG7GK,OAAO;EsG8GtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI,GACnB;;AAGD,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GASjB;EAhBD,AASI,gBATY,CASZ,CAAC,CAAC;IACE,KAAK,EfqRD,OAA4B,GehRnC;IAfL,AAYQ,gBAZQ,CASZ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EhBkTL,KAAK,GgBjTR;;AAKT,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG;EAChB,KAAK,EhBkSG,KAAK,GgB7RhB;EAfD,AAYI,KAZC,CAAC,YAAY,CAYd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtGlJW,KAAK,GsGmJxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtGtJK,KAAK,GsGuJxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtG5JW,KAAK;IsG6JrB,IAAI,EtG7JY,MAAK,GsG8JxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtG7KC,KAAK;MsG+KjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,KAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/navy_sunrise.css b/themes/learn2-git-sync/css/styles/navy_sunrise.css
deleted file mode 100644
index 389fd7d..0000000
--- a/themes/learn2-git-sync/css/styles/navy_sunrise.css
+++ /dev/null
@@ -1,1189 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "21"; }
-
-.balance::after {
- content: "49"; }
-
-.color-block .color1 {
- background: #058569;
- color: #fff; }
- .color-block .color1::after {
- content: "#058569"; }
-
-.color-block .color2 {
- background: #6b99a0;
- color: #fff; }
- .color-block .color2::after {
- content: "#6b99a0"; }
-
-.fix-color .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-color .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-color .color:nth-child(3)::after {
- content: "#fff"; }
-
-.fix-contrast .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#fff"; }
-
-.best-contrast .color:nth-child(2) {
- background: #89e1c7;
- color: #000; }
- .best-contrast .color:nth-child(2)::after {
- content: "#89e1c7"; }
-
-.best-contrast .color:nth-child(3) {
- background: #e1ffff;
- color: #000; }
- .best-contrast .color:nth-child(3)::after {
- content: "#e1ffff"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #08a585;
- color: #fff; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#08a585"; }
-
-.check-contrast .result::after {
- content: "false"; }
-
-.luminance .result::after {
- content: "0.17824, 0.28507"; }
-
-body #sidebar ul li.active > a {
- color: #333333 !important; }
-
-body {
- background: #F4F4F4;
- color: #333333;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: black; }
- a:link, a:visited {
- color: black; }
- a:hover, a:active {
- color: black; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b,
-strong,
-label,
-th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #EAC67A;
- color: #fbf4e4;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #e7bd64;
- background: #edcf90;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #fbf5e9;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #faf2e0; }
- .searchbox input::-moz-placeholder {
- color: #faf2e0; }
- .searchbox input:-moz-placeholder {
- color: #faf2e0; }
- .searchbox input:-ms-input-placeholder {
- color: #faf2e0; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #984B43;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #b1574e; }
- #sidebar a,
- #sidebar i {
- color: white; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #f3ebe9; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(255, 255, 255, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #8d463e; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #7f3f38;
- color: white !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #333333 !important; }
- #sidebar h5.active i {
- color: #333333 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics,
- #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: white; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul,
- #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: white;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #86423b;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: white; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #333333 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #333333 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- display: inline;
- color: white; }
- #sidebar ul li.visited > a .read-icon:hover {
- color: #f3ebe9; }
- #sidebar ul li.active > a .read-icon, #sidebar ul li.active.visited > a .read-icon {
- display: inline;
- color: #333333; }
- #sidebar ul li.active > a .read-icon:hover, #sidebar ul li.active.visited > a .read-icon:hover {
- color: black; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: #1c0e0c; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: #512824; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img,
- #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border,
- #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow,
- #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #dbdbdb; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #e6e6e6;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400;
- font-size: 1.05rem;
- line-height: 1.7; }
-
-h1,
-h2,
-h3,
-h4,
-h5,
-h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px;
- overflow-wrap: break-word;
- overflow: visible;
- word-break: break-word;
- white-space: normal;
- margin: 0.425rem 0 0.85rem 0; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px;
- font-size: 2.55rem; }
-
-h2 {
- letter-spacing: -2px;
- font-size: 2.15rem; }
-
-h3 {
- letter-spacing: -1px;
- font-size: 1.8rem; }
-
-h4 {
- font-size: 1.4rem; }
-
-h5 {
- font-size: 0.9rem; }
-
-h6 {
- font-size: 0.7rem; }
-
-p {
- margin: 1.7rem 0; }
-
-ul,
-ol {
- margin-top: 1.7rem;
- margin-bottom: 1.7rem; }
- ul ul,
- ul ol,
- ol ul,
- ol ol {
- margin-top: 0;
- margin-bottom: 0; }
-
-blockquote {
- border-left: 10px solid #F0F2F4;
- margin: 1.7rem 0;
- padding-left: 0.85rem; }
- blockquote p {
- font-size: 1.1rem;
- color: #666666; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #333333;
- font-size: 0.925rem; }
- blockquote cite:before {
- content: "\2014 \0020"; }
-
-pre {
- margin: 1.7rem 0;
- padding: 0.938rem; }
-
-code {
- vertical-align: bottom; }
-
-small {
- font-size: 0.925rem; }
-
-hr {
- border-left: none;
- border-right: none;
- border-top: none;
- margin: 1.7rem 0; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #233237;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #233237; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #233237 !important;
- color: white !important;
- box-shadow: 0 3px 0 #172124 !important; }
- .button:hover {
- background: #172124 !important;
- box-shadow: 0 3px 0 #0b1012 !important;
- color: white !important; }
- .button:active {
- box-shadow: 0 1px 0 #0b1012 !important; }
- .button i {
- color: white !important; }
-
-.button-secondary {
- background: #050808 !important;
- color: white !important;
- box-shadow: 0 3px 0 black !important; }
- .button-secondary:hover {
- background: black !important;
- box-shadow: 0 3px 0 black !important;
- color: white !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 black !important; }
- .button-secondary i {
- color: white !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #F4F4F4;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem;
- color: #333333; }
- #top-bar a {
- color: #1a1a1a; }
- #top-bar a:hover {
- color: #0d0d0d; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: #1a1a1a;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-/*# sourceMappingURL=navy_sunrise.css.map */
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/navy_sunrise.css.map b/themes/learn2-git-sync/css/styles/navy_sunrise.css.map
deleted file mode 100644
index 7f1aa5b..0000000
--- a/themes/learn2-git-sync/css/styles/navy_sunrise.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "navy_sunrise.css",
- "sources": [
- "../../scss/styles/navy_sunrise.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AYuIZ,AnB9HA,gBmB8HgB,EAWhB,KAAK,CAAC,YAAY,CnBzIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAmBO;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAoBO;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GAwB0C;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GA6B8D;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAA+B,IAAI,GAiCc;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAkC+D;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAsC6C;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,OAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,kBAA2C,GAAI;;AvFhW3E,AACI,IADA,CACA,QAAQ,CAAC,EAAE,CAAC,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;EACtB,KAAK,EArCA,OAAO,CAqCO,UAAU,GAChC;;AyF7CL,AAAA,IAAI,CAAC;EACJ,UAAU,EzFQQ,OAAO;EyFPzB,KAAK,EzFKO,OAAO;EyFJnB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GAClC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EHqGc,KAAkB,GG1FrC;EAZD,AAGC,CAHA,AAGC,KAAK,EAHP,CAAC,AAIC,QAAQ,CAAC;IACT,KAAK,EHiGa,KAAkB,GGhGpC;EANF,AAQC,CARA,AAQC,MAAM,EARR,CAAC,AASC,OAAO,CAAC;IACR,KAAK,EFiYK,KAA2B,GEhYrC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAK7B;EARF,AAKE,WALS,CACV,CAAC,AAIC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbHA,OAAoB,GaIrC;;AAED,AAAA,CAAC;AACD,MAAM;AEvBN,KAAK;AMdL,EAAE,CRqCK;EACN,WAAW,EzFZO,GAAG,GyFarB;;AAED,AAAA,kBAAkB,EG9ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAwFJ,EAAE,EC5FN,KAAK,EAAL,KAAK,CA2BD,QAAQ,EA3BZ,KAAK,CAwDD,IAAI,CLfW;E3BrCX,kBAAoB,E2BsCP,GAAG,CAAC,IAAI,CAAC,IAAI;E3BjC1B,eAAiB,E2BiCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3BlB1B,UAAY,E2BkBC,GAAG,CAAC,IAAI,CAAC,IAAI,GACjC;;AEhDD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FKQ,OAAO;E4FJzB,KAAK,EN+EW,OAA4B;EM9E5C,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5F0BA,IAAI;I4FzBT,MAAM,E5F0BA,IAAI,G4FpBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,OAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN6BO,OAA4B;IM5BxC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5F1BG,GAAG,G4F+BpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIR,OAAO,EAAE,YAAY,GAG5B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FNH,OAAO;E6FOpB,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FKO,KAAK;E6FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FeM,GAAG;E6FdpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CN2Yf,OAA4B,GMrKvC;EAhPD,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FAE,KAA+B,G6FSzC;IAvBL,AAgBQ,QAhBA,CAYJ,CAAC,AAII,MAAM;IAhBf,QAAQ,CAaJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EN2XL,OAA4B,GM1X/B;IAlBT,AAoBQ,QApBA,CAYJ,CAAC,AAQI,SAAS;IApBlB,QAAQ,CAaJ,CAAC,AAOI,SAAS,CAAC;MACP,KAAK,E7FPF,wBAA+B,G6FQrC;EAtBT,AAyBI,QAzBI,CAyBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CN+WpB,OAA2B,GM9WlC;EA3BL,AA6BI,QA7BI,CA6BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA/BL,AAiCI,QAjCI,CAiCJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAzEL,AAsCQ,QAtCA,CAiCJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA5CT,AA8CQ,QA9CA,CAiCJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7FjCF,wBAA+B;M6FkClC,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IApDT,AAuDY,QAvDJ,CAiCJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENiVd,OAA2B;MMhVvB,KAAK,ENmVT,KAA4B,CMnVU,UAAU,GAC/C;IA1Db,AA8DY,QA9DJ,CAiCJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBpEZ,IAAI;MiBqEF,KAAK,E7FlER,OAAO,C6FkEc,UAAU,GAC/B;IAjEb,AAmEY,QAnEJ,CAiCJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7FtER,OAAO,C6FsEc,UAAU,GAC/B;EArEb,AA2EI,QA3EI,CA2EJ,EAAE,GAAC,EAAE,AAAA,OAAO,CAAC;IACT,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA9EL,AAkFQ,QAlFA,CAgFJ,EAAE,AAAA,OAAO,GAEJ,EAAE,AAAA,OAAO;EAlFlB,QAAQ,CAiFJ,EAAE,AAAA,OAAO,GACJ,EAAE,AAAA,OAAO,CAAC;IACP,OAAO,EAAE,KAAK,GACjB;EApFT,AAwFI,QAxFI,CAwFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAmJZ;IA/OL,AA+FY,QA/FJ,CAwFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EN4ST,KAA4B,GM3S3B;IAjGb,AAoGgB,QApGR,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,ENuSb,KAA4B,GMlSvB;MA1GjB,AAuGoB,QAvGZ,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAGI,MAAM,CAAC;QACJ,KAAK,ENoSjB,KAA4B,GMnSnB;IAzGrB,AA8GQ,QA9GA,CAwFJ,EAAE,AAsBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAoDjB;MAnKT,AAkHgB,QAlHR,CAwFJ,EAAE,AAsBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MApHjB,AAuHY,QAvHJ,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA9Hb,AA2HgB,QA3HR,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MA7HjB,AAgIY,QAhIJ,CAwFJ,EAAE,AAsBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE;MAhIxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAmBH,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACV,OAAO,EAAE,KAAK,GACjB;MAnIb,AAsIgB,QAtIR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAAC;QACC,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QAlJjB,AA0IoB,QA1IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAIE,CAAC,CAAC;UACE,KAAK,E7F7Hd,KAA+B;U6F8HtB,WAAW,EAAE,MAAM,GACtB;QA7IrB,AA+IoB,QA/IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CASE,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MAjJrB,AAoJgB,QApJR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,EApJxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,CAAC;QACL,UAAU,ENmPlB,OAA2B;QMlPnB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAOtB;QAjKjB,AA6JwB,QA7JhB,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,GAQH,CAAC,CACE,CAAC,EA7JzB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,GAOH,CAAC,CACE,CAAC,CAAC;UACE,KAAK,E7FhJlB,KAA+B,G6FiJrB;IA/JzB,AAqKQ,QArKA,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAAC;MACR,UAAU,EjB3KR,IAAI;MiB4KN,KAAK,E7FzKJ,OAAO,C6FyKU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAKtB;MAhLT,AA6KY,QA7KJ,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAQP,CAAC,CAAC;QACE,KAAK,E7FhLR,OAAO,C6FgLc,UAAU,GAC/B;IA/Kb,AAkLQ,QAlLA,CAwFJ,EAAE,CA0FE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GA0Db;MA7OT,AAqLY,QArLJ,CAwFJ,EAAE,CA0FE,EAAE,AAGG,QAAQ,GAAC,IAAI,CAAC;QACX,YAAY,EAAE,IAAI,GACrB;MAvLb,AAyLY,QAzLJ,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAQjB;QAnMb,AA6LgB,QA7LR,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAIG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAlMjB,AAqMY,QArMJ,CAwFJ,EAAE,CA0FE,EAAE,GAmBG,CAAC,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAvMb,AAyMY,QAzMJ,CAwFJ,EAAE,CA0FE,EAAE,CAuBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MAhNb,AAmNgB,QAnNR,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FvMV,KAA+B,G6F4M7B;QA1NjB,AAuNoB,QAvNZ,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENoLjB,OAA4B,GMnLnB;MAzNrB,AA+NgB,QA/NR,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,EA/N7B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FnOZ,OAAO,G6FwOH;QAtOjB,AAmOoB,QAnOZ,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,AAIR,MAAM,EAnO3B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENqKjB,KAA2B,GMpKlB;MArOrB,AAyOY,QAzOJ,CAwFJ,EAAE,CA0FE,EAAE,CAuDE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAMb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENmJT,OAA2B,GM3I1B;EAZb,AAMgB,KANX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,OAAO,EANxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,MAAM,EARvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAME,QAAQ,CAAC;IACN,KAAK,EPxJL,OAAkB,GOyJrB;;ACtQjB,AAAA,KAAK,CAAC;EACF,UAAU,ElBiCC,OAAO;EkBhClB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GACnD;;AAED,AAAA,KAAK,CAAC;EAmBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FNC,KAAK;E8FOjB,UAAU,EAAE,IAAI,GAoFnB;EAzGD,AAEI,KAFC,CAED,GAAG;EAFP,KAAK,CAGD,gBAAgB,CAAC;IACb,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAhBL,AAQQ,KARH,CAED,GAAG,AAME,OAAO;IARhB,KAAK,CAGD,gBAAgB,AAKX,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IAXT,AAaQ,KAbH,CAED,GAAG,AAWE,OAAO;IAbhB,KAAK,CAGD,gBAAgB,AAUX,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAfT,AAuBI,KAvBC,CAuBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAzBL,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZpBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EAvCL,AAyCI,KAzCC,CAyCD,EAAE,GAAC,EAAE,CAAC;IACF,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZzBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA8CI,KA9CC,CA8CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EAtDL,AAwDI,KAxDC,CAwDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9F9BC,IAAI;I8F+BV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAsCrB;IAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAAC;MACC,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZrDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAwDI,KAxDC,CAwDD,IAAI,CAAC;QAmBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAsBrB;QAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAgBK;UACC,OAAO,EAAE,YAAY,GACxB;IAtFb,AAyFQ,KAzFH,CAwDD,IAAI,CAiCA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IA3FT,AA6FQ,KA7FH,CAwDD,IAAI,AAqCC,MAAM,CAAC;MACJ,UAAU,EPwTV,OAA2B,GOvT9B;IA/FT,AAiGQ,KAjGH,CAwDD,IAAI,AAyCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IAnGT,AAqGQ,KArGH,CAwDD,IAAI,AA6CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJhIa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;IIiIpF,WAAW,E9FnGG,GAAG;I8FoGjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClB1Fd,OAAO,GkB2FpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;AL1JL,AAAA,IAAI,COeC;EACJ,WAAW,ENboB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMc3F,cAAc,EAAE,QAAQ;EACxB,WAAW,EAAE,GAAG;EAChB,SAAS,EvBlBO,OAAO;EuBmBvB,WAAW,EvBlBO,GAAG,GuBmBrB;;AAGD,AAAA,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE,CAAC;EACF,WAAW,EN1BoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EM2BjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,aAAa,EAAE,UAAU;EACzB,QAAQ,EAAE,OAAO;EACjB,UAAU,EAAE,UAAU;EACtB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,QAAqB,CAAC,CAAC,CAAC,OAAqB,CAAC,CAAC,GACvD;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI;EACpB,SAAS,EvBzCK,OAAoB,GuB0ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvB7CK,OAAoB,GuB8ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvBjDK,MAAoB,GuBkDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBpDK,MAAoB,GuBqDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBvDK,MAAsB,GuBwDpC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvB1DK,MAAsB,GuB2DpC;;AAGD,AAAA,CAAC,CAAC;EACD,MAAM,EvB5DU,MAAwB,CuB4DhB,CAAC,GACzB;;AAGD,AAAA,EAAE;AACF,EAAE,CAAC;EACF,UAAU,EvBlEM,MAAwB;EuBmExC,aAAa,EvBnEG,MAAwB,GuB0ExC;EAVD,AAKC,EALC,CAKD,EAAE;EALH,EAAE,CAMD,EAAE;EALH,EAAE,CAID,EAAE;EAJH,EAAE,CAKD,EAAE,CAAC;IACF,UAAU,EAAE,CAAC;IACb,aAAa,EAAE,CAAC,GAChB;;AAIF,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpB1CN,OAAO;EoB2CxB,MAAM,EvB/EU,MAAwB,CuB+EhB,CAAC;EACzB,YAAY,EAAE,OAAmB,GAiBjC;EApBD,AAKC,UALS,CAKT,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EVaa,OAAkB,GUZpC;EARF,AAUC,UAVS,CAUT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVOa,OAAkB;IUNpC,SAAS,EAAE,QAAuB,GAKlC;IAnBF,AAgBE,UAhBQ,CAUT,IAAI,AAMF,OAAO,CAAC;MACR,OAAO,EAAE,aAAa,GACtB;;AP3EH,AAAA,GAAG,COgFC;EACH,MAAM,EvBrGU,MAAwB,CuBqGhB,CAAC;EACzB,OAAO,ExBhHa,QAAQ,GwBiH5B;;AAED,AAAA,IAAI,CAAC;EACJ,cAAc,EAAE,MAAM,GACtB;;AAGD,AAAA,KAAK,CAAC;EACL,SAAS,EAAE,QAAuB,GAClC;;AAED,AAAA,EAAE,CAAC;EACF,WAAW,EAAE,IAAI;EACjB,YAAY,EAAE,IAAI;EAClB,UAAU,EAAE,IAAI;EAChB,MAAM,EvBtHU,MAAwB,CuBsHhB,CAAC,GACzB;;AA1CD,AAAA,UAAU,CA6CC;EACV,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EACrB,QAAQ,EAAE,MAAM,GAChB;;AAED,AAAA,UAAU,GAAC,UAAU,GAAC,UAAU,CAAC;EAEhC,MAAM,EAAE,CAAC,GAuET;EAzED,AAIC,UAJS,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACnB,KAAK,EAAE,IAAI,GAqBX;IA/BF,AAaG,UAbO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AACX,OAAO,CAAC;MACR,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpB1JK,IAAI;MoB2Jd,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACV;IApBJ,AAsBG,UAtBO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AAUX,MAAM,CAAC;MACP,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBnKK,IAAI;MoBoKd,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GACf;EA7BJ,AAiCC,UAjCS,GAAC,UAAU,GAAC,UAAU,GAiC9B,CAAC,CAAC;IAEF,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EAtCF,AAwCC,UAxCS,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,CAAC;IAEb,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAjDF,AA8CE,UA9CQ,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,AAMX,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,SAAS,GAClB;EAhDH,AAmDC,UAnDS,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAExB,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAKnB;IA5DF,AAyDE,UAzDQ,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,AAMtB,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,MAAM,GACf;EA3DH,AA8DC,UA9DS,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAEnC,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAvEF,AAoEE,UApEQ,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,AAMjC,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,KAAK,GACd;;AAMH,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,ENzNoB,aAAa,EAAE,SAAS,GM0NvD;;AA1GD,AAAA,IAAI,CA4GC;EACJ,UAAU,EpBhLI,OAAO;EoBiLrB,KAAK,ET+KM,OAA2B;ES9KtC,OAAO,EAAE,WAAW;EACpB,aAAa,EAAE,GAAG,GAClB;;APtMD,AAAA,GAAG,COwMC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpBvLG,OAAO;EoBwLpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBtOD,IAAI;EoBuOpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpB/LS,OAAO;IoBgMrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAvHF,AAAA,EAAE,CA2HC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB1MP,OAAO,GoB2MxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhG/PO,OAAO;EgGgQxB,KAAK,EpBhQQ,IAAI,GoBiQjB;;AAGD,AACC,KADI,CACJ,CAAC,AAAA,YAAY,CAAC;EACb,KAAK,EAAE,IAAI,GACX;;AAHF,AAKC,KALI,CAKJ,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EACnB,KAAK,EhG1QW,OAAO,GgG2QvB;;AAIF,AAAA,gBAAgB,GAAC,eAAe,CAAC,qBAAqB,CAAC;EACtD,gBAAgB,EpBhRH,wBAAI,GoBiRjB;;AAED,AAAA,gBAAgB,GAAC,eAAe,CAAC,WAAW,CAAC;EAC5C,gBAAgB,ET6HL,OAA4B,GS5HvC;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAClD,gBAAgB,EAAE,IAAI,GACtB;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAC7D,gBAAgB,EAAE,IAAI,GACtB;;ACjSD,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFLO,OAAO,CqFKL,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,OAA2B,CFvYE,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEqYA,OAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,OAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,OAA2B,CFhYI,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFMM,OAAyB,CqFNtB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,KAA2B,CFvYE,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEqYA,KAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,KAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,KAA2B,CFhYI,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BWU,MAAwB,C0BXhB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtG7GK,OAAO;EsG8GtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,KAAK,EfkSG,OAA4B,GezRvC;EAhBD,AASI,QATI,CASJ,CAAC,CAAC;IACE,KAAK,Ef+RD,OAA4B,Ge1RnC;IAfL,AAYQ,QAZA,CASJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,Ef4RL,OAA4B,Ge3R/B;;AAKT,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtGlJW,KAAK,GsGmJxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtGtJK,KAAK,GsGuJxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtG5JW,KAAK;IsG6JrB,IAAI,EtG7JY,MAAK,GsG8JxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtG7KC,KAAK;MsG+KjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,OAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/spitsbergen.css b/themes/learn2-git-sync/css/styles/spitsbergen.css
deleted file mode 100644
index aa7ce10..0000000
--- a/themes/learn2-git-sync/css/styles/spitsbergen.css
+++ /dev/null
@@ -1,1188 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "16"; }
-
-.balance::after {
- content: "24"; }
-
-.color-block .color1 {
- background: #97cbfc;
- color: #000; }
- .color-block .color1::after {
- content: "#97cbfc"; }
-
-.color-block .color2 {
- background: #ffc1ff;
- color: #000; }
- .color-block .color2::after {
- content: "#ffc1ff"; }
-
-.fix-color .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-color .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-color .color:nth-child(3)::after {
- content: "#fff"; }
-
-.fix-contrast .color:nth-child(2) {
- background: #444548;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "#444548"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#fff"; }
-
-.best-contrast .color:nth-child(2) {
- background: #6c32b8;
- color: #fff; }
- .best-contrast .color:nth-child(2)::after {
- content: "#6c32b8"; }
-
-.best-contrast .color:nth-child(3) {
- background: black;
- color: #fff; }
- .best-contrast .color:nth-child(3)::after {
- content: "black"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #a4dcff;
- color: #000; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#a4dcff"; }
-
-.check-contrast .result::after {
- content: "false"; }
-
-.luminance .result::after {
- content: "0.56375, 0.66406"; }
-
-body {
- background: #FAFAFA;
- color: #383F45;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: #0a0c0d; }
- a:link, a:visited {
- color: #0a0c0d; }
- a:hover, a:active {
- color: black; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b,
-strong,
-label,
-th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #8d9297;
- color: #e8e9ea;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #80858b;
- background: #9a9fa3;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #ebeced;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #e6e7e8; }
- .searchbox input::-moz-placeholder {
- color: #e6e7e8; }
- .searchbox input:-moz-placeholder {
- color: #e6e7e8; }
- .searchbox input:-ms-input-placeholder {
- color: #e6e7e8; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #2d3237;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #3d444b; }
- #sidebar a,
- #sidebar i {
- color: #FAFAFA; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #d4d4d4; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(250, 250, 250, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #262a2e; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(250, 250, 250, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #1d2023;
- color: white !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #383F45 !important; }
- #sidebar h5.active i {
- color: #383F45 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics,
- #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: white; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul,
- #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: #FAFAFA;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #212529;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: #FAFAFA; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #383F45 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #383F45 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- display: inline;
- color: #FAFAFA; }
- #sidebar ul li.visited > a .read-icon:hover {
- color: #d4d4d4; }
- #sidebar ul li.active > a .read-icon, #sidebar ul li.active.visited > a .read-icon {
- display: inline;
- color: #383F45; }
- #sidebar ul li.active > a .read-icon:hover, #sidebar ul li.active.visited > a .read-icon:hover {
- color: black; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: black; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: black; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img,
- #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border,
- #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow,
- #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #e1e1e1; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #e1e1e1;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400;
- font-size: 1.05rem;
- line-height: 1.7; }
-
-h1,
-h2,
-h3,
-h4,
-h5,
-h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px;
- overflow-wrap: break-word;
- overflow: visible;
- word-break: break-word;
- white-space: normal;
- margin: 0.425rem 0 0.85rem 0; }
-
-p {
- margin: 1.7rem 0; }
-
-ul,
-ol {
- margin-top: 1.7rem;
- margin-bottom: 1.7rem; }
- ul ul,
- ul ol,
- ol ul,
- ol ol {
- margin-top: 0;
- margin-bottom: 0; }
-
-blockquote {
- border-left: 10px solid #F0F2F4;
- margin: 1.7rem 0;
- padding-left: 0.85rem; }
- blockquote p {
- font-size: 1.1rem;
- color: #666666; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #333333;
- font-size: 0.925rem; }
- blockquote cite:before {
- content: "\2014 \0020"; }
-
-pre {
- margin: 1.7rem 0;
- padding: 0.938rem; }
-
-code {
- vertical-align: bottom; }
-
-small {
- font-size: 0.925rem; }
-
-hr {
- border-left: none;
- border-right: none;
- border-top: none;
- margin: 1.7rem 0; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #383F45;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #383F45; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #383F45 !important;
- color: white !important;
- box-shadow: 0 3px 0 #2a3034 !important; }
- .button:hover {
- background: #2a3034 !important;
- box-shadow: 0 3px 0 #1d2023 !important;
- color: white !important; }
- .button:active {
- box-shadow: 0 1px 0 #1d2023 !important; }
- .button i {
- color: white !important; }
-
-.button-secondary {
- background: #16181b !important;
- color: white !important;
- box-shadow: 0 3px 0 #08090a !important; }
- .button-secondary:hover {
- background: #08090a !important;
- box-shadow: 0 3px 0 black !important;
- color: white !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 black !important; }
- .button-secondary i {
- color: white !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #2d3237;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem;
- color: #cccccc; }
- #top-bar a {
- color: #e6e6e6; }
- #top-bar a:hover {
- color: #f2f2f2; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: #212529;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-.version-chooser select {
- display: inline-block;
- color: #000000;
- background-color: #FFFFFF!;
- border: 1px solid #666666;
- font-size: 15px;
- font-weight: regular;
- margin: 0;
-}
-
-.videoWrapper {
- position: relative;
- padding-bottom: 56.25%; /* 16:9 */
- padding-top: 25px;
- height: 0;
-}
-.videoWrapper iframe {
- position: absolute;
- top: 0;
- left: 0;
- width: 100%;
- height: 100%;
-}
-
-/*# sourceMappingURL=spitsbergen.css.map */
diff --git a/themes/learn2-git-sync/css/styles/spitsbergen.css.map b/themes/learn2-git-sync/css/styles/spitsbergen.css.map
deleted file mode 100644
index 8be46a1..0000000
--- a/themes/learn2-git-sync/css/styles/spitsbergen.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "spitsbergen.css",
- "sources": [
- "../../scss/styles/spitsbergen.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AYuIZ,AnB9HA,gBmB8HgB,EAWhB,KAAK,CAAC,YAAY,CnBzIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAA+B,IAAI,GAmBa;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAoBa;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GAwB0C;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EApIqD,OAAqB;EAqIpF,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GA6B8D;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAiCQ;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EAjJF,KAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAkCyD;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,OAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAsCmD;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,OAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,kBAA2C,GAAI;;AE1Y3E,AAAA,IAAI,CAAC;EACJ,UAAU,EzFQQ,OAAO;EyFPzB,KAAK,EzFKO,OAAO;EyFJnB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GAClC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EHqGc,OAAkB,GG1FrC;EAZD,AAGC,CAHA,AAGC,KAAK,EAHP,CAAC,AAIC,QAAQ,CAAC;IACT,KAAK,EHiGa,OAAkB,GGhGpC;EANF,AAQC,CARA,AAQC,MAAM,EARR,CAAC,AASC,OAAO,CAAC;IACR,KAAK,EFiYK,KAA2B,GEhYrC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAK7B;EARF,AAKE,WALS,CACV,CAAC,AAIC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbHA,OAAoB,GaIrC;;AAED,AAAA,CAAC;AACD,MAAM;AEvBN,KAAK;AMdL,EAAE,CRqCK;EACN,WAAW,EzFdO,GAAG,GyFerB;;AAED,AAAA,kBAAkB,EG9ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAwFJ,EAAE,EC5FN,KAAK,EAAL,KAAK,CA2BD,QAAQ,EA3BZ,KAAK,CAwDD,IAAI,CLfW;E3BrCX,kBAAoB,E2BsCP,GAAG,CAAC,IAAI,CAAC,IAAI;E3BjC1B,eAAiB,E2BiCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3BlB1B,UAAY,E2BkBC,GAAG,CAAC,IAAI,CAAC,IAAI,GACjC;;AEhDD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FiBF,OAA6D;E4FhBrE,KAAK,EN+EW,OAA4B;EM9E5C,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5FwBA,IAAI;I4FvBT,MAAM,E5FwBA,IAAI,G4FlBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,OAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN6BO,OAA4B;IM5BxC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5F5BG,GAAG,G4FiCpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIR,OAAO,EAAE,YAAY,GAG5B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FLE,OAA4B;E6FM9C,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FKO,KAAK;E6FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FaM,GAAG;E6FZpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CN2Yf,OAA4B,GMrKvC;EAhPD,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FdM,OAAO,G6FuBrB;IAvBL,AAgBQ,QAhBA,CAYJ,CAAC,AAII,MAAM;IAhBf,QAAQ,CAaJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EN2XL,OAA4B,GM1X/B;IAlBT,AAoBQ,QApBA,CAYJ,CAAC,AAQI,SAAS;IApBlB,QAAQ,CAaJ,CAAC,AAOI,SAAS,CAAC;MACP,KAAK,E7FrBE,wBAAO,G6FsBjB;EAtBT,AAyBI,QAzBI,CAyBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CN+WpB,OAA2B,GM9WlC;EA3BL,AA6BI,QA7BI,CA6BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA/BL,AAiCI,QAjCI,CAiCJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAzEL,AAsCQ,QAtCA,CAiCJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA5CT,AA8CQ,QA9CA,CAiCJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7F/CE,wBAAO;M6FgDd,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IApDT,AAuDY,QAvDJ,CAiCJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENiVd,OAA2B;MMhVvB,KAAK,ENmVT,KAA4B,CMnVU,UAAU,GAC/C;IA1Db,AA8DY,QA9DJ,CAiCJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBpEZ,IAAI;MiBqEF,KAAK,E7FlER,OAAO,C6FkEc,UAAU,GAC/B;IAjEb,AAmEY,QAnEJ,CAiCJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7FtER,OAAO,C6FsEc,UAAU,GAC/B;EArEb,AA2EI,QA3EI,CA2EJ,EAAE,GAAC,EAAE,AAAA,OAAO,CAAC;IACT,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA9EL,AAkFQ,QAlFA,CAgFJ,EAAE,AAAA,OAAO,GAEJ,EAAE,AAAA,OAAO;EAlFlB,QAAQ,CAiFJ,EAAE,AAAA,OAAO,GACJ,EAAE,AAAA,OAAO,CAAC;IACP,OAAO,EAAE,KAAK,GACjB;EApFT,AAwFI,QAxFI,CAwFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAmJZ;IA/OL,AA+FY,QA/FJ,CAwFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EN4ST,KAA4B,GM3S3B;IAjGb,AAoGgB,QApGR,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,ENuSb,KAA4B,GMlSvB;MA1GjB,AAuGoB,QAvGZ,CAwFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAGI,MAAM,CAAC;QACJ,KAAK,ENoSjB,KAA4B,GMnSnB;IAzGrB,AA8GQ,QA9GA,CAwFJ,EAAE,AAsBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAoDjB;MAnKT,AAkHgB,QAlHR,CAwFJ,EAAE,AAsBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MApHjB,AAuHY,QAvHJ,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA9Hb,AA2HgB,QA3HR,CAwFJ,EAAE,AAsBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MA7HjB,AAgIY,QAhIJ,CAwFJ,EAAE,AAsBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE;MAhIxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAmBH,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACV,OAAO,EAAE,KAAK,GACjB;MAnIb,AAsIgB,QAtIR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAAC;QACC,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QAlJjB,AA0IoB,QA1IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CAIE,CAAC,CAAC;UACE,KAAK,E7F3IV,OAAO;U6F4IF,WAAW,EAAE,MAAM,GACtB;QA7IrB,AA+IoB,QA/IZ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,GACE,CAAC,CASE,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MAjJrB,AAoJgB,QApJR,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,EApJxB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,CAAC;QACL,UAAU,ENmPlB,OAA2B;QMlPnB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAOtB;QAjKjB,AA6JwB,QA7JhB,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAeE,OAAO,GAQH,CAAC,CACE,CAAC,EA7JzB,QAAQ,CAwFJ,EAAE,AAsBG,OAAO,GAuBH,EAAE,AAgBE,OAAO,GAOH,CAAC,CACE,CAAC,CAAC;UACE,KAAK,E7F9Jd,OAAO,G6F+JD;IA/JzB,AAqKQ,QArKA,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAAC;MACR,UAAU,EjB3KR,IAAI;MiB4KN,KAAK,E7FzKJ,OAAO,C6FyKU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAKtB;MAhLT,AA6KY,QA7KJ,CAwFJ,EAAE,CA6EE,EAAE,AAAA,OAAO,GAAC,CAAC,CAQP,CAAC,CAAC;QACE,KAAK,E7FhLR,OAAO,C6FgLc,UAAU,GAC/B;IA/Kb,AAkLQ,QAlLA,CAwFJ,EAAE,CA0FE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GA0Db;MA7OT,AAqLY,QArLJ,CAwFJ,EAAE,CA0FE,EAAE,AAGG,QAAQ,GAAC,IAAI,CAAC;QACX,YAAY,EAAE,IAAI,GACrB;MAvLb,AAyLY,QAzLJ,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAQjB;QAnMb,AA6LgB,QA7LR,CAwFJ,EAAE,CA0FE,EAAE,CAOE,CAAC,CAIG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAlMjB,AAqMY,QArMJ,CAwFJ,EAAE,CA0FE,EAAE,GAmBG,CAAC,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAvMb,AAyMY,QAzMJ,CAwFJ,EAAE,CA0FE,EAAE,CAuBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MAhNb,AAmNgB,QAnNR,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FrNN,OAAO,G6F0NT;QA1NjB,AAuNoB,QAvNZ,CAwFJ,EAAE,CA0FE,EAAE,AAgCG,QAAQ,GACJ,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENoLjB,OAA4B,GMnLnB;MAzNrB,AA+NgB,QA/NR,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,EA/N7B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,CAAC;QACV,OAAO,EAAE,MAAM;QACf,KAAK,E7FnOZ,OAAO,G6FwOH;QAtOjB,AAmOoB,QAnOZ,CAwFJ,EAAE,CA0FE,EAAE,AA2CG,OAAO,GAEH,CAAC,CAAC,UAAU,AAIR,MAAM,EAnO3B,QAAQ,CAwFJ,EAAE,CA0FE,EAAE,AA4CG,OAAO,AAAA,QAAQ,GACX,CAAC,CAAC,UAAU,AAIR,MAAM,CAAC;UACJ,KAAK,ENqKjB,KAA2B,GMpKlB;MArOrB,AAyOY,QAzOJ,CAwFJ,EAAE,CA0FE,EAAE,CAuDE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAMb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENmJT,KAA2B,GM3I1B;EAZb,AAMgB,KANX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,OAAO,EANxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,MAAM,EARvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAME,QAAQ,CAAC;IACN,KAAK,EPxJL,KAAkB,GOyJrB;;ACtQjB,AAAA,KAAK,CAAC;EACF,UAAU,ElBiCC,OAAO;EkBhClB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GACnD;;AAED,AAAA,KAAK,CAAC;EAmBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FNC,KAAK;E8FOjB,UAAU,EAAE,IAAI,GAoFnB;EAzGD,AAEI,KAFC,CAED,GAAG;EAFP,KAAK,CAGD,gBAAgB,CAAC;IACb,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAhBL,AAQQ,KARH,CAED,GAAG,AAME,OAAO;IARhB,KAAK,CAGD,gBAAgB,AAKX,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IAXT,AAaQ,KAbH,CAED,GAAG,AAWE,OAAO;IAbhB,KAAK,CAGD,gBAAgB,AAUX,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAfT,AAuBI,KAvBC,CAuBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAzBL,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZpBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AA2BI,KA3BC,CA2BD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EAvCL,AAyCI,KAzCC,CAyCD,EAAE,GAAC,EAAE,CAAC;IACF,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZzBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA8CI,KA9CC,CA8CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EAtDL,AAwDI,KAxDC,CAwDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9FhCC,IAAI;I8FiCV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAsCrB;IAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAAC;MACC,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZrDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAwDI,KAxDC,CAwDD,IAAI,CAAC;QAmBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAsBrB;QAxGL,AAoEQ,KApEH,CAwDD,IAAI,GAYC,CAAC,CAgBK;UACC,OAAO,EAAE,YAAY,GACxB;IAtFb,AAyFQ,KAzFH,CAwDD,IAAI,CAiCA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IA3FT,AA6FQ,KA7FH,CAwDD,IAAI,AAqCC,MAAM,CAAC;MACJ,UAAU,EPwTV,OAA2B,GOvT9B;IA/FT,AAiGQ,KAjGH,CAwDD,IAAI,AAyCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IAnGT,AAqGQ,KArGH,CAwDD,IAAI,AA6CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJhIa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;IIiIpF,WAAW,E9FrGG,GAAG;I8FsGjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClB1Fd,OAAO,GkB2FpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;AL1JL,AAAA,IAAI,COeC;EACJ,WAAW,ENboB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMc3F,cAAc,EAAE,QAAQ;EACxB,WAAW,EAAE,GAAG;EAChB,SAAS,EvBlBO,OAAO;EuBmBvB,WAAW,EvBlBO,GAAG,GuBmBrB;;AAGD,AAAA,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE;AACF,EAAE,CAAC;EACF,WAAW,EN1BoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EM2BjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,aAAa,EAAE,UAAU;EACzB,QAAQ,EAAE,OAAO;EACjB,UAAU,EAAE,UAAU;EACtB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,QAAqB,CAAC,CAAC,CAAC,OAAqB,CAAC,CAAC,GACvD;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI;EACpB,SAAS,EvBzCK,OAAoB,GuB0ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvB7CK,OAAoB,GuB8ClC;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI;EACpB,SAAS,EvBjDK,MAAoB,GuBkDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBpDK,MAAoB,GuBqDlC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvBvDK,MAAsB,GuBwDpC;;AAED,AAAA,EAAE,CAAC;EACF,SAAS,EvB1DK,MAAsB,GuB2DpC;;AAGD,AAAA,CAAC,CAAC;EACD,MAAM,EvB5DU,MAAwB,CuB4DhB,CAAC,GACzB;;AAGD,AAAA,EAAE;AACF,EAAE,CAAC;EACF,UAAU,EvBlEM,MAAwB;EuBmExC,aAAa,EvBnEG,MAAwB,GuB0ExC;EAVD,AAKC,EALC,CAKD,EAAE;EALH,EAAE,CAMD,EAAE;EALH,EAAE,CAID,EAAE;EAJH,EAAE,CAKD,EAAE,CAAC;IACF,UAAU,EAAE,CAAC;IACb,aAAa,EAAE,CAAC,GAChB;;AAIF,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpB1CN,OAAO;EoB2CxB,MAAM,EvB/EU,MAAwB,CuB+EhB,CAAC;EACzB,YAAY,EAAE,OAAmB,GAiBjC;EApBD,AAKC,UALS,CAKT,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EVaa,OAAkB,GUZpC;EARF,AAUC,UAVS,CAUT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVOa,OAAkB;IUNpC,SAAS,EAAE,QAAuB,GAKlC;IAnBF,AAgBE,UAhBQ,CAUT,IAAI,AAMF,OAAO,CAAC;MACR,OAAO,EAAE,aAAa,GACtB;;AP3EH,AAAA,GAAG,COgFC;EACH,MAAM,EvBrGU,MAAwB,CuBqGhB,CAAC;EACzB,OAAO,ExBhHa,QAAQ,GwBiH5B;;AAED,AAAA,IAAI,CAAC;EACJ,cAAc,EAAE,MAAM,GACtB;;AAGD,AAAA,KAAK,CAAC;EACL,SAAS,EAAE,QAAuB,GAClC;;AAED,AAAA,EAAE,CAAC;EACF,WAAW,EAAE,IAAI;EACjB,YAAY,EAAE,IAAI;EAClB,UAAU,EAAE,IAAI;EAChB,MAAM,EvBtHU,MAAwB,CuBsHhB,CAAC,GACzB;;AA1CD,AAAA,UAAU,CA6CC;EACV,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EACrB,QAAQ,EAAE,MAAM,GAChB;;AAED,AAAA,UAAU,GAAC,UAAU,GAAC,UAAU,CAAC;EAEhC,MAAM,EAAE,CAAC,GAuET;EAzED,AAIC,UAJS,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACnB,KAAK,EAAE,IAAI,GAqBX;IA/BF,AAaG,UAbO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AACX,OAAO,CAAC;MACR,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpB1JK,IAAI;MoB2Jd,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACV;IApBJ,AAsBG,UAtBO,GAAC,UAAU,GAAC,UAAU,CAI/B,CAAC,AAQC,YAAY,AAUX,MAAM,CAAC;MACP,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBnKK,IAAI;MoBoKd,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GACf;EA7BJ,AAiCC,UAjCS,GAAC,UAAU,GAAC,UAAU,GAiC9B,CAAC,CAAC;IAEF,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EAtCF,AAwCC,UAxCS,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,CAAC;IAEb,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAjDF,AA8CE,UA9CQ,GAAC,UAAU,GAAC,UAAU,GAwC9B,UAAU,GAAC,CAAC,AAMX,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,SAAS,GAClB;EAhDH,AAmDC,UAnDS,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAExB,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAKnB;IA5DF,AAyDE,UAzDQ,GAAC,UAAU,GAAC,UAAU,GAmD9B,UAAU,GAAC,UAAU,GAAC,CAAC,AAMtB,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,MAAM,GACf;EA3DH,AA8DC,UA9DS,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,CAAC;IAEnC,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAKnB;IAvEF,AAoEE,UApEQ,GAAC,UAAU,GAAC,UAAU,GA8D9B,UAAU,GAAC,UAAU,GAAC,UAAU,GAAC,CAAC,AAMjC,YAAY,AAAA,MAAM,CAAC;MACnB,OAAO,EAAE,KAAK,GACd;;AAMH,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,ENzNoB,aAAa,EAAE,SAAS,GM0NvD;;AA1GD,AAAA,IAAI,CA4GC;EACJ,UAAU,EpBhLI,OAAO;EoBiLrB,KAAK,ET+KM,OAA2B;ES9KtC,OAAO,EAAE,WAAW;EACpB,aAAa,EAAE,GAAG,GAClB;;APtMD,AAAA,GAAG,COwMC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpBvLG,OAAO;EoBwLpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBtOD,IAAI;EoBuOpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpB/LS,OAAO;IoBgMrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAvHF,AAAA,EAAE,CA2HC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB1MP,OAAO,GoB2MxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhG/PO,OAAO;EgGgQxB,KAAK,EpBhQQ,IAAI,GoBiQjB;;AAGD,AACC,KADI,CACJ,CAAC,AAAA,YAAY,CAAC;EACb,KAAK,EAAE,IAAI,GACX;;AAHF,AAKC,KALI,CAKJ,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EACnB,KAAK,EhG1QW,OAAO,GgG2QvB;;AAIF,AAAA,gBAAgB,GAAC,eAAe,CAAC,qBAAqB,CAAC;EACtD,gBAAgB,EpBhRH,wBAAI,GoBiRjB;;AAED,AAAA,gBAAgB,GAAC,eAAe,CAAC,WAAW,CAAC;EAC5C,gBAAgB,ET6HL,OAA4B,GS5HvC;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAClD,gBAAgB,EAAE,IAAI,GACtB;;AAED,AAAA,gBAAgB,GAAC,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAC7D,gBAAgB,EAAE,IAAI,GACtB;;ACjSD,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFLO,OAAO,CqFKL,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,OAA2B,CFvYE,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEqYA,OAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,OAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,OAA2B,CFhYI,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFMM,OAAyB,CqFNtB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,OAA2B,CFvYE,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEqYA,OAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,KAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,KAA2B,CFhYI,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BWU,MAAwB,C0BXhB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtGhHQ,OAA4B;EsGiH9C,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,KAAK,Ef+RG,OAA2B,GetRtC;EAhBD,AASI,QATI,CASJ,CAAC,CAAC;IACE,KAAK,Ef4RD,OAA2B,GevRlC;IAfL,AAYQ,QAZA,CASJ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EfyRL,OAA2B,GexR9B;;AAKT,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtGlJW,KAAK,GsGmJxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtGtJK,KAAK,GsGuJxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtG5JW,KAAK;IsG6JrB,IAAI,EtG7JY,MAAK,GsG8JxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtG7KC,KAAK;MsG+KjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,OAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/sunrise.css b/themes/learn2-git-sync/css/styles/sunrise.css
deleted file mode 100644
index a53a48d..0000000
--- a/themes/learn2-git-sync/css/styles/sunrise.css
+++ /dev/null
@@ -1,1116 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-.ratio::after {
- content: "19"; }
-
-.balance::after {
- content: "5"; }
-
-.color-block .color1 {
- background: #624e28;
- color: #fff; }
- .color-block .color1::after {
- content: "#624e28"; }
-
-.color-block .color2 {
- background: #4f894e;
- color: #fff; }
- .color-block .color2::after {
- content: "#4f894e"; }
-
-.fix-color .color:nth-child(2) {
- background: #000;
- color: #fff; }
- .fix-color .color:nth-child(2)::after {
- content: "#000"; }
-
-.fix-color .color:nth-child(3) {
- background: #fff;
- color: #000; }
- .fix-color .color:nth-child(3)::after {
- content: "#fff"; }
-
-.fix-contrast .color:nth-child(2) {
- background: #0a0602;
- color: #fff; }
- .fix-contrast .color:nth-child(2)::after {
- content: "#0a0602"; }
-
-.fix-contrast .color:nth-child(3) {
- background: #f6f9f6;
- color: #000; }
- .fix-contrast .color:nth-child(3)::after {
- content: "#f6f9f6"; }
-
-.best-contrast .color:nth-child(2) {
- background: #1d1302;
- color: #fff; }
- .best-contrast .color:nth-child(2)::after {
- content: "#1d1302"; }
-
-.best-contrast .color:nth-child(3) {
- background: #ffff55;
- color: #000; }
- .best-contrast .color:nth-child(3)::after {
- content: "#ffff55"; }
-
-.scale-luminance .color:nth-child(2) {
- background: #947b56;
- color: #fff; }
- .scale-luminance .color:nth-child(2)::after {
- content: "#947b56"; }
-
-.check-contrast .result::after {
- content: "false"; }
-
-.luminance .result::after {
- content: "0.08387, 0.20127"; }
-
-body #sidebar ul li.active > a {
- color: #000000 !important; }
-
-body {
- background: #ffff8f;
- color: #000000;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: black; }
- a:link, a:visited {
- color: black; }
- a:hover, a:active {
- color: black; }
-
-#body-inner a {
- text-decoration: underline;
- text-decoration-style: dotted; }
- #body-inner a:hover {
- text-decoration-style: solid; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b, strong, label, th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #ec9e69;
- color: #fbece1;
- text-align: center;
- padding: 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #e98f52;
- background: #efad80;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #fcefe6;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: #fbe9dc; }
- .searchbox input::-moz-placeholder {
- color: #fbe9dc; }
- .searchbox input:-moz-placeholder {
- color: #fbe9dc; }
- .searchbox input:-ms-input-placeholder {
- color: #fbe9dc; }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #a75015;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px;
- border-right: 1px solid #c75f19; }
- #sidebar a,
- #sidebar i {
- color: white; }
- #sidebar a:hover,
- #sidebar i:hover {
- color: #f4eae2; }
- #sidebar a.subtitle,
- #sidebar i.subtitle {
- color: rgba(255, 255, 255, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #994a13; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #874111;
- color: white !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #000000 !important; }
- #sidebar h5.active i {
- color: #000000 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics, #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: white; }
- #sidebar ul.searched .search-match a {
- color: white; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul, #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- color: white;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #904512;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul.topics > li.parent > a b, #sidebar ul.topics > li.active > a b {
- color: white; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #000000 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a b {
- color: #000000 !important; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- color: #ca384f;
- display: inline; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#body #navigation .nav .fa {
- color: #080401; }
- #body #navigation .nav .fa:active, #body #navigation .nav .fa:focus, #body #navigation .nav .fa:hover, #body #navigation .nav .fa:visited {
- color: #4c250a; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img, #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border, #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow, #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav i {
- font-size: 50px; }
- #body .nav:hover {
- background: #ffff8f; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #e6e6e6;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400; }
-
-h1, h2, h3, h4, h5, h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px; }
-
-h2 {
- letter-spacing: -2px; }
-
-h3 {
- letter-spacing: -1px; }
-
-blockquote {
- border-left: 10px solid #F0F2F4; }
- blockquote p {
- font-size: 1.1rem;
- color: #666666; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #333333;
- font-size: 1.2rem; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #ca384f;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #ca384f; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #ca384f !important;
- color: white !important;
- box-shadow: 0 3px 0 #d15065 !important; }
- .button:hover {
- background: #d15065 !important;
- box-shadow: 0 3px 0 #d7687a !important;
- color: white !important; }
- .button:active {
- box-shadow: 0 1px 0 #d7687a !important; }
- .button i {
- color: white !important; }
-
-.button-secondary {
- background: #902637 !important;
- color: white !important;
- box-shadow: 0 3px 0 #77202e !important; }
- .button-secondary:hover {
- background: #77202e !important;
- box-shadow: 0 3px 0 #5f1925 !important;
- color: white !important; }
- .button-secondary:active {
- box-shadow: 0 1px 0 #5f1925 !important; }
- .button-secondary i {
- color: white !important; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #ffff8f;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
- #top-github-link a {
- color: #0d0d0d; }
- #top-github-link a:hover {
- color: black; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4;
- color: black; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: black;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-/*# sourceMappingURL=sunrise.css.map */
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/styles/sunrise.css.map b/themes/learn2-git-sync/css/styles/sunrise.css.map
deleted file mode 100644
index 5ab97b0..0000000
--- a/themes/learn2-git-sync/css/styles/sunrise.css.map
+++ /dev/null
@@ -1,111 +0,0 @@
-{
- "version": 3,
- "file": "sunrise.css",
- "sources": [
- "../../scss/styles/sunrise.scss",
- "../../scss/_prefix.scss",
- "../../scss/vendor/bourbon/_bourbon.scss",
- "../../scss/vendor/bourbon/settings/_prefixer.scss",
- "../../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_is-num.scss",
- "../../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../../scss/vendor/bourbon/functions/_assign.scss",
- "../../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../../scss/vendor/bourbon/functions/_grid-width.scss",
- "../../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../../scss/vendor/bourbon/functions/_strip-units.scss",
- "../../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../../scss/vendor/bourbon/functions/_unpack.scss",
- "../../scss/vendor/bourbon/css3/_animation.scss",
- "../../scss/vendor/bourbon/css3/_appearance.scss",
- "../../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../../scss/vendor/bourbon/css3/_background.scss",
- "../../scss/vendor/bourbon/css3/_background-image.scss",
- "../../scss/vendor/bourbon/css3/_border-image.scss",
- "../../scss/vendor/bourbon/css3/_border-radius.scss",
- "../../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../../scss/vendor/bourbon/css3/_calc.scss",
- "../../scss/vendor/bourbon/css3/_columns.scss",
- "../../scss/vendor/bourbon/css3/_filter.scss",
- "../../scss/vendor/bourbon/css3/_flex-box.scss",
- "../../scss/vendor/bourbon/css3/_font-face.scss",
- "../../scss/vendor/bourbon/css3/_hyphens.scss",
- "../../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../../scss/vendor/bourbon/css3/_keyframes.scss",
- "../../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../../scss/vendor/bourbon/css3/_perspective.scss",
- "../../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../../scss/vendor/bourbon/css3/_transform.scss",
- "../../scss/vendor/bourbon/css3/_transition.scss",
- "../../scss/vendor/bourbon/css3/_user-select.scss",
- "../../scss/vendor/bourbon/css3/_placeholder.scss",
- "../../scss/vendor/bourbon/addons/_button.scss",
- "../../scss/vendor/bourbon/addons/_clearfix.scss",
- "../../scss/vendor/bourbon/addons/_directional-values.scss",
- "../../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../../scss/vendor/bourbon/addons/_font-family.scss",
- "../../scss/vendor/bourbon/addons/_hide-text.scss",
- "../../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../../scss/vendor/bourbon/addons/_position.scss",
- "../../scss/vendor/bourbon/addons/_prefixer.scss",
- "../../scss/vendor/bourbon/addons/_retina-image.scss",
- "../../scss/vendor/bourbon/addons/_size.scss",
- "../../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../../scss/vendor/bourbon/addons/_triangle.scss",
- "../../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../../scss/configuration/nucleus/_base.scss",
- "../../scss/configuration/nucleus/_core.scss",
- "../../scss/configuration/nucleus/_breakpoints.scss",
- "../../scss/configuration/nucleus/_layout.scss",
- "../../scss/configuration/nucleus/_typography.scss",
- "../../scss/configuration/nucleus/_nav.scss",
- "../../scss/configuration/theme/_base.scss",
- "../../scss/configuration/theme/_colors.scss",
- "../../scss/configuration/theme/_bullets.scss",
- "../../scss/nucleus/functions/_base.scss",
- "../../scss/nucleus/functions/_direction.scss",
- "../../scss/nucleus/functions/_range.scss",
- "../../scss/nucleus/mixins/_base.scss",
- "../../scss/nucleus/mixins/_breakpoints.scss",
- "../../scss/nucleus/mixins/_utilities.scss",
- "../../scss/theme/modules/_base.scss",
- "../../scss/theme/modules/_buttons.scss",
- "../../scss/_hover-color.scss",
- "../../scss/_contrast.scss",
- "../../scss/_affix.scss",
- "../../scss/theme/_core.scss",
- "../../scss/theme/_fonts.scss",
- "../../scss/theme/_forms.scss",
- "../../scss/theme/_header.scss",
- "../../scss/theme/_nav.scss",
- "../../scss/theme/_main.scss",
- "../../scss/_standard_colors.scss",
- "../../scss/theme/_typography.scss",
- "../../scss/theme/_tables.scss",
- "../../scss/theme/_buttons.scss",
- "../../scss/theme/_bullets.scss",
- "../../scss/theme/_tooltips.scss",
- "../../scss/theme/_scrollbar.scss",
- "../../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";A0FCA,OAAO,CAAC,8EAAI;AY8HZ,AnBrHA,gBmBqHgB,EAmBhB,KAAK,CAAC,YAAY,CnBxIF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AelBD,AbAA,OaAO,EAKP,iBAAiB,CbLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EaND,AbGC,OaHM,AbGL,OAAO,EaET,iBAAiB,AbFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;ACLF;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;EAuCE;AAoXF;;;GAGG;AASH;;;GAGG;AAQH;;;GAGG;ACrbH;;;;;;;;;;;EAWE;AAiWF,AAAA,MAAM,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,IAAW,GAAI;;AACxC,AAAA,QAAQ,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,GAAa,GAAI;;AAE5C,AAAA,YAAY,CAAC,OAAO,CAAC;EApBnB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAmBO;EAAvD,AAjBE,YAiBU,CAAC,OAAO,AAjBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAgBH,AAAA,YAAY,CAAC,OAAO,CAAC;EArBnB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAoBO;EAAvD,AAlBE,YAkBU,CAAC,OAAO,AAlBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAkBH,AACE,UADQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAxBpB,UAAU,EAlFmC,IAAI;EAmFjD,KAAK,EAAqC,IAAI,GAuBoC;EADpF,AApBE,UAoBQ,CACR,MAAM,AAAA,UAAW,CAAA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAkBH,AAEE,UAFQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,EAAE;EAzBpB,UAAU,EAnF8B,IAAI;EAoF5C,KAAK,EAA+B,IAAI,GAwB0C;EAFpF,AApBE,UAoBQ,CAER,MAAM,AAAA,UAAW,CAAA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,MAAW,GACrB;;AAuBH,AACE,aADW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA7BpB,UAAU,EApIqD,OAAqB;EAqIpF,KAAK,EAAqC,IAAI,GA4BwD;EADxG,AAzBE,aAyBW,CACX,MAAM,AAAA,UAAW,CALA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAuBH,AAEE,aAFW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,EAKE;EA9BpB,UAAU,EAxDC,OAAsC;EAyDjD,KAAK,EAA+B,IAAI,GA6B8D;EAFxG,AAzBE,aAyBW,CAEX,MAAM,AAAA,UAAW,CALA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AACE,cADY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAlCpB,UAAU,EALF,OAA0C;EAMlD,KAAK,EAAqC,IAAI,GAiCQ;EADxD,AA9BE,cA8BY,CACZ,MAAM,AAAA,UAAW,CAVA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AA4BH,AAEE,cAFY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,EAUE;EAnCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAA+B,IAAI,GAkC+D;EAFzG,AA9BE,cA8BY,CAEZ,MAAM,AAAA,UAAW,CAVA,CAAC,CAtBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAiCH,AACE,gBADc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,EAeE;EAvCpB,UAAU,EAjJF,OAA0C;EAkJlD,KAAK,EAAqC,IAAI,GAsC6C;EAD7F,AAnCE,gBAmCc,CACd,MAAM,AAAA,UAAW,CAfA,CAAC,CArBjB,OAAO,CAAC;IACP,OAAO,EAAE,SAAW,GACrB;;AAqCH,AACE,eADa,CACb,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,OAA6C,GAAI;;AAG7E,AACE,UADQ,CACR,OAAO,AAAA,OAAO,CAAC;EAAE,OAAO,EAAE,kBAA2C,GAAI;;AvFhW3E,AACI,IADA,CACA,QAAQ,CAAC,EAAE,CAAC,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;EACtB,KAAK,EArCA,OAAO,CAqCO,UAAU,GAChC;;AyF7CL,AAAA,IAAI,CAAC;EACJ,UAAU,EzFQQ,OAAO;EyFPzB,KAAK,EzFKO,OAAO;EyFJhB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GACrC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EF0YM,KAA2B,GEjYtC;EAVD,AAEC,CAFA,AAEC,KAAK,EAFP,CAAC,AAGC,QAAQ,CAAC;IACT,KAAK,EFuYK,KAA2B,GEtYrC;EALF,AAMC,CANA,AAMC,MAAM,EANR,CAAC,AAOC,OAAO,CAAC;IACR,KAAK,EFmYK,KAA2B,GElYrC;;AAGF,AACC,WADU,CACV,CAAC,CAAC;EACD,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAI7B;EAPF,AAIE,WAJS,CACV,CAAC,AAGC,MAAM,CAAC;IACP,qBAAqB,EAAE,KAAK,GAC5B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CbAA,OAAoB,GaCrC;;AAED,AAAA,CAAC,EAAE,MAAM,EEnBT,KAAK,EMdL,EAAE,CRiCQ;EACN,WAAW,EzFRI,GAAG,GyFSrB;;AAED,AAAA,kBAAkB,EG1ClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAqFJ,EAAE,ECzFN,KAAK,EAAL,KAAK,CAyBD,QAAQ,EAzBZ,KAAK,CAsDD,IAAI,CLjBW;E3BjCX,kBAAoB,E2BkCJ,GAAG,CAAC,IAAI,CAAC,IAAI;E3B7B7B,eAAiB,E2B6BD,GAAG,CAAC,IAAI,CAAC,IAAI;E3Bd7B,UAAY,E2BcI,GAAG,CAAC,IAAI,CAAC,IAAI,GACpC;;AE5CD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CfKD,IAAI,GeJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CfAD,IAAI;EeCpB,UAAU,EfOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GeG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EfAc,OAA8B,GeCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EfZI,OAAO;IeavB,UAAU,EfDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GeC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,E5FIG,OAAO;E4FHpB,KAAK,EN+EW,OAA4B;EM9E5C,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,E5F0BA,IAAI;I4FzBT,MAAM,E5F0BA,IAAI,G4FpBb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EhBdF,IAAI,GgBeT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CLgYT,OAA2B;EK/XnC,UAAU,ELyXF,OAA4B;EKxXpC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,EhB5BC,wBAAI;IgB6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EhBnCC,wBAAI;IgBoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,EhB1CH,wBAAI,GgB2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EN6BO,OAA4B;IM5BxC,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,E5F1BG,GAAG,G4F+BpB;IAzCL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,2BAA2B,CAAE;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,kBAAkB,CAAW;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,iBAAiB,CAAY;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;IuCkBL,AvCpBI,UuCoBM,CA2BN,KAAK,AvC/CJ,sBAAsB,CAAO;MuC2DtB,KAAK,ENmBG,OAA4B,GjC5E3C;;AwCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;EXcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IWrB1C,AAAA,eAAe,CAAC;MAIP,OAAO,EAAE,YAAY,GAG7B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,E7FUP,OAAiC;E6FT1C,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,E7FKO,KAAK;E6FJjB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,E7FeM,GAAG;E6FdpB,SAAS,EAAE,IAAI;EACf,YAAY,EAAE,GAAG,CAAC,KAAK,CN2Yf,OAA4B,GMjMvC;EApND,AAYI,QAZI,CAYJ,CAAC;EAZL,QAAQ,CAaJ,CAAC,CAAC;IACE,KAAK,E7FAE,KAA+B,G6FOzC;IArBL,AAeQ,QAfA,CAYJ,CAAC,AAGI,MAAM;IAff,QAAQ,CAaJ,CAAC,AAEI,MAAM,CAAC;MACJ,KAAK,EN4XL,OAA4B,GM3X/B;IAjBT,AAkBQ,QAlBA,CAYJ,CAAC,AAMI,SAAS;IAlBlB,QAAQ,CAaJ,CAAC,AAKI,SAAS,CAAC;MACP,KAAK,E7FLF,wBAA+B,G6FMrC;EApBT,AAuBI,QAvBI,CAuBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CNiXpB,OAA2B,GMhXlC;EAzBL,AA2BI,QA3BI,CA2BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA7BL,AA+BI,QA/BI,CA+BJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAvEL,AAoCQ,QApCA,CA+BJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA1CT,AA4CQ,QA5CA,CA+BJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,E7F/BF,wBAA+B;M6FgClC,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IAlDT,AAqDY,QArDJ,CA+BJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,ENmVd,OAA2B;MMlVvB,KAAK,ENqVT,KAA4B,CMrVU,UAAU,GAC/C;IAxDb,AA4DY,QA5DJ,CA+BJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EjBlEZ,IAAI;MiBmEF,KAAK,E7FhER,OAAO,C6FgEc,UAAU,GAC/B;IA/Db,AAiEY,QAjEJ,CA+BJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,E7FpER,OAAO,C6FoEc,UAAU,GAC/B;EAnEb,AAyEI,QAzEI,CAyEJ,EAAE,GAAG,EAAE,AAAA,OAAO,CAAC;IACX,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA5EL,AA+EQ,QA/EA,CA8EJ,EAAE,AAAA,OAAO,GACH,EAAE,AAAA,OAAO,EA/EnB,QAAQ,CA8EO,EAAE,AAAA,OAAO,GACd,EAAE,AAAA,OAAO,CAAC;IACR,OAAO,EAAE,KAAK,GACjB;EAjFT,AAqFI,QArFI,CAqFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GA0HZ;IAnNL,AA4FY,QA5FJ,CAqFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EN+ST,KAA4B,GM9S3B;IA9Fb,AAiGgB,QAjGR,CAqFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,EN0Sb,KAA4B,GMtSvB;MAtGjB,AAmGoB,QAnGZ,CAqFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAEI,MAAM,CAAC;QACJ,KAAK,ENwSjB,KAA4B,GMvSnB;IArGrB,AA0GQ,QA1GA,CAqFJ,EAAE,AAqBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GAiDjB;MA5JT,AA8GgB,QA9GR,CAqFJ,EAAE,AAqBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MAhHjB,AAmHY,QAnHJ,CAqFJ,EAAE,AAqBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QA1Hb,AAuHgB,QAvHR,CAqFJ,EAAE,AAqBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MAzHjB,AA4HY,QA5HJ,CAqFJ,EAAE,AAqBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE,EA5HxB,QAAQ,CAqFJ,EAAE,AAqBG,OAAO,GAkBY,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACzB,OAAO,EAAE,KAAK,GACjB;MA9Hb,AAiIgB,QAjIR,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CAAC;QACA,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QA7IjB,AAqIoB,QArIZ,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CAIC,CAAC,CAAC;UACE,KAAK,E7FxHd,KAA+B;U6FyHtB,WAAW,EAAE,MAAM,GACtB;QAxIrB,AA0IoB,QA1IZ,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CASC,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MA5IrB,AA+IgB,QA/IR,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeC,OAAO,EA/IxB,QAAQ,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeW,OAAO,CAAC;QACf,UAAU,ENyPlB,OAA2B;QMxPnB,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GAMtB;QA1JjB,AAsJwB,QAtJhB,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeC,OAAO,GAMF,CAAC,CACC,CAAC,EAtJzB,QAAQ,CAqFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeW,OAAO,GAMZ,CAAC,CACC,CAAC,CAAC;UACE,KAAK,E7FzIlB,KAA+B,G6F0IrB;IAxJzB,AA8JQ,QA9JA,CAqFJ,EAAE,CAyEE,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;MACV,UAAU,EjBpKR,IAAI;MiBqKN,KAAK,E7FlKJ,OAAO,C6FkKU,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GAItB;MAxKT,AAqKY,QArKJ,CAqFJ,EAAE,CAyEE,EAAE,AAAA,OAAO,GAAG,CAAC,CAOT,CAAC,CAAC;QACE,KAAK,E7FxKR,OAAO,C6FwKc,UAAU,GAC/B;IAvKb,AA0KQ,QA1KA,CAqFJ,EAAE,CAqFE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GAsCb;MAjNT,AA4KY,QA5KJ,CAqFJ,EAAE,CAqFE,EAAE,AAEG,QAAQ,GAAG,IAAI,CAAC;QACb,YAAY,EAAE,IAAI,GACrB;MA9Kb,AA+KY,QA/KJ,CAqFJ,EAAE,CAqFE,EAAE,CAKE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAOjB;QAxLb,AAkLgB,QAlLR,CAqFJ,EAAE,CAqFE,EAAE,CAKE,CAAC,CAGG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MAvLjB,AAyLY,QAzLJ,CAqFJ,EAAE,CAqFE,EAAE,GAeI,CAAC,CAAC;QACA,OAAO,EAAE,KAAK,GACjB;MA3Lb,AA6LY,QA7LJ,CAqFJ,EAAE,CAqFE,EAAE,CAmBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MApMb,AAuMgB,QAvMR,CAqFJ,EAAE,CAqFE,EAAE,AA4BG,QAAQ,GACH,CAAC,CAAC,UAAU,CAAC;QACX,KAAK,E7FnMX,OAA6B;Q6FoMvB,OAAO,EAAE,MAAM,GAClB;MA1MjB,AA6MY,QA7MJ,CAqFJ,EAAE,CAqFE,EAAE,CAmCE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AAKb,AAGY,KAHP,CACD,WAAW,CACP,IAAI,CACA,GAAG,CAAC;EACA,KAAK,ENgLT,OAA2B,GMzK1B;EAXb,AAKgB,KALX,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAEE,OAAO,EALxB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAGE,MAAM,EANvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAIE,MAAM,EAPvB,KAAK,CACD,WAAW,CACP,IAAI,CACA,GAAG,AAKE,QAAQ,CAAC;IACN,KAAK,EP1HL,OAAkB,GO2HrB;;ACxOjB,AAAA,KAAK,CAAC;EACL,UAAU,ElBiCI,OAAO;EkBhCrB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GAChD;;AAED,AAAA,KAAK,CAAC;EAiBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,E9FJC,KAAK;E8FKjB,UAAU,EAAE,IAAI,GAkFnB;EArGD,AACI,KADC,CACD,GAAG,EADP,KAAK,CACI,gBAAgB,CAAC;IAClB,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAdL,AAMQ,KANH,CACD,GAAG,AAKE,OAAO,EANhB,KAAK,CACI,gBAAgB,AAKhB,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IATT,AAWQ,KAXH,CACD,GAAG,AAUE,OAAO,EAXhB,KAAK,CACI,gBAAgB,AAUhB,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAbT,AAqBI,KArBC,CAqBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAvBL,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IZlBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IZrBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYhB1C,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EArCL,AAuCI,KAvCC,CAuCD,EAAE,GAAG,EAAE,CAAC;IACJ,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EZvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IYnB1C,AA4CI,KA5CC,CA4CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EApDL,AAsDI,KAtDC,CAsDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,E9F5BC,IAAI;I8F6BV,SAAS,EAAE,CAAC;IACZ,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAoCrB;IApGL,AAiEQ,KAjEH,CAsDD,IAAI,GAWE,CAAC,CAAC;MACA,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IZlDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MYnB1C,AAsDI,KAtDC,CAsDD,IAAI,CAAC;QAkBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAqBrB;QApGL,AAiEQ,KAjEH,CAsDD,IAAI,GAWE,CAAC,CAeK;UACA,OAAO,EAAE,YAAY,GACxB;IAlFb,AAqFQ,KArFH,CAsDD,IAAI,CA+BA,CAAC,CAAC;MACE,SAAS,EAAE,IAAI,GAClB;IAvFT,AAyFQ,KAzFH,CAsDD,IAAI,AAmCC,MAAM,CAAC;MACJ,UAAU,E9FtFH,OAAO,G8FuFjB;IA3FT,AA6FQ,KA7FH,CAsDD,IAAI,AAuCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IA/FT,AAiGQ,KAjGH,CAsDD,IAAI,AA2CC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJ5Ha,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;II6HpF,WAAW,E9F/FG,GAAG;I8FgGjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ClBtFd,OAAO,GkBuFpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;ALtJL,AAAA,IAAI,COCC;EACJ,WAAW,ENCoB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMAxF,cAAc,EAAE,QAAQ;EAC3B,WAAW,EAAE,GAAG,GAChB;;AAGD,AAAA,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC;EACtB,WAAW,ENLoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EMMjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI,GACpB;;AAGD,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CpBiBN,OAAO,GoBNxB;EAZD,AAEC,UAFS,CAET,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EV2Ea,OAAkB,GU1EpC;EALF,AAMC,UANS,CAMT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EVsEa,OAAkB;IUrEpC,SAAS,EAAE,MAAM,GACjB;;AAXF,AAAA,UAAU,CAeC;EACP,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EAClB,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,UAAU,GAAG,UAAU,GAAG,UAAU,CAAC;EAEpC,MAAM,EAAE,CAAC,GAmET;EArED,AAIC,UAJS,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACb,KAAK,EAAE,IAAI,GAoBjB;IA9BF,AAaY,UAbF,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,AAQO,YAAY,AACR,OAAO,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBjEP,IAAI;MoBkEF,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACb;IApBb,AAqBY,UArBF,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,AAQO,YAAY,AASR,MAAM,CAAC;MACJ,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EpBzEP,IAAI;MoB0EF,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GAClB;EA5Bb,AAgCC,UAhCS,GAAG,UAAU,GAAG,UAAU,GAgCjC,CAAC,CAAC;IAEH,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EArCF,AAuCC,UAvCS,GAAG,UAAU,GAAG,UAAU,GAuCjC,UAAU,GAAG,CAAC,CAAC;IAEhB,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAInB;IA/CF,AA4CQ,UA5CE,GAAG,UAAU,GAAG,UAAU,GAuCjC,UAAU,GAAG,CAAC,AAKR,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,SAAS,GACrB;EA9CT,AAiDC,UAjDS,GAAG,UAAU,GAAG,UAAU,GAiDjC,UAAU,GAAG,UAAU,GAAG,CAAC,CAAC;IAE7B,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAInB;IAzDF,AAsDQ,UAtDE,GAAG,UAAU,GAAG,UAAU,GAiDjC,UAAU,GAAG,UAAU,GAAG,CAAC,AAKrB,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,MAAM,GAClB;EAxDT,AA2DC,UA3DS,GAAG,UAAU,GAAG,UAAU,GA2DjC,UAAU,GAAG,UAAU,GAAG,UAAU,GAAG,CAAC,CAAC;IAE1C,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAInB;IAnEF,AAgEQ,UAhEE,GAAG,UAAU,GAAG,UAAU,GA2DjC,UAAU,GAAG,UAAU,GAAG,UAAU,GAAG,CAAC,AAKlC,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,KAAK,GACjB;;AAMT,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,EN5HoB,aAAa,EAAE,SAAS,GM6HvD;;AAED,AAAA,IAAI,CAAC;EACJ,UAAU,EpBnFI,OAAO;EoBoFrB,KAAK,ET4QM,OAA2B;ES3QtC,OAAO,EAAE,WAAW;EACnB,aAAa,EAAE,GAAG,GACnB;;AP5GD,AAAA,GAAG,CO8GC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EpB1FG,OAAO;EoB2FpB,MAAM,EAAE,GAAG,CAAC,KAAK,CpBzID,IAAI;EoB0IpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EpBlGS,OAAO;IoBmGrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAIF,AAAA,EAAE,CAAC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CpB7GP,OAAO,GoB8GxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EhGxJG,OAA6B;EgGyJ1C,KAAK,EpBnKQ,IAAI,GoBoKjB;;AAGD,AACI,KADC,CACD,CAAC,AAAA,YAAY,CAAC;EAAE,KAAK,EAAE,IAAI,GAAI;;AADnC,AAEI,KAFC,CAED,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EAAE,KAAK,EhG/JjB,OAA6B,GgG+JM;;AAIjD,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EAAE,gBAAgB,EpB7K7D,wBAAI,GoB6KgF;;AAClG,AAAA,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAAE,gBAAgB,ETmOrD,OAA4B,GSnOmD;;AAC3F,AAAA,gBAAgB,GAAG,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,IAAI,GAAI;;AACjF,AAAA,gBAAgB,GAAG,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,IAAI,GAAI;;ACpL5F,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EbSP,UAAU,ErFKG,OAA6B,CqFLvB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CE0YR,OAA4B,CF1YC,UAAU,GaRlD;EAHD,AbYC,OaZM,AbYL,MAAM,CAAC;IACP,UAAU,EEwYA,OAA4B,CFxYN,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYT,OAA4B,CFvYG,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EahBF,AbiBC,OajBM,AbiBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEmYT,OAA4B,CFnYG,UAAU,GACnD;EanBF,AboBC,OapBM,CboBN,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;AajBF,AAAA,iBAAiB,CAAC;EbIjB,UAAU,ErFMM,OAAyB,CqFNtB,UAAU;EAC7B,KAAK,ECibM,KAAK,CDjbuB,UAAU;EACjD,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEuYR,OAA2B,CFvYE,UAAU,GaHlD;EAHD,AbOC,iBaPgB,AbOf,MAAM,CAAC;IACP,UAAU,EEqYA,OAA2B,CFrYL,UAAU;IAC1C,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEoYT,OAA2B,CFpYI,UAAU;IACnD,KAAK,EC4aK,KAAK,CD5awB,UAAU,GACjD;EaXF,AbYC,iBaZgB,AbYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CEgYT,OAA2B,CFhYI,UAAU,GACnD;EadF,AbeC,iBafgB,CbehB,CAAC,CAAC;IACD,KAAK,ECsaK,KAAK,CDtawB,UAAU,GACjD;;ActBF,AAAA,QAAQ,CAAC;EACR,MAAM,E1BYa,MAAwB,C0BZnB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EhBUlB,KAAK,EAAE,GAAsB,GgBL7B;EjBIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBT1C,AAAA,kBAAkB,CAAC;MhBUlB,KAAK,EAAE,IAAsB,GgBL7B;;AAED,AAAA,oBAAoB,CAAC;EhBGpB,KAAK,EAAE,SAAsB,GgBE7B;EjBHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBF1C,AAAA,oBAAoB,CAAC;MhBGpB,KAAK,EAAE,IAAsB,GgBE7B;;AAED,AAAA,mBAAmB,CAAC;EhBJnB,KAAK,EAAE,GAAsB,GgBS7B;EjBVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IiBK1C,AAAA,mBAAmB,CAAC;MhBJnB,KAAK,EAAE,IAAsB,GgBS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EvBjCO,OAAO;EuBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EtBrCgB,MAAM;EsBsC3B,MAAM,EtBtCe,MAAM;EsBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EvBpCQ,IAAI;EuBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EvB5CO,OAAO,GuB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtB/Ca,OAA6B,GsBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EtBlDa,OAA8B,GsBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CVXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EUY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACI,gBADY,CACZ,KAAK,CAAC;EACF,QAAQ,EAAE,OAAO,GAKpB;EAPL,AAIQ,gBAJQ,CACZ,KAAK,CAGD,QAAQ,CAAC;IACL,QAAQ,EAAE,OAAO,GACpB;;AAKT,AACI,eADW,CACX,CAAC,CAAC;EACE,cAAc,EAAE,MAAM,GACzB;;AAIL,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIQ,UAJE,CAEN,WAAW,CAEP,EAAE,CAAC;EACC,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GACrB;;AATT,AAWQ,UAXE,CAEN,WAAW,CASP,EAAE,CAAC;EACC,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAcrB;EA5BT,AAgBY,UAhBF,CAEN,WAAW,CASP,EAAE,CAKE,EAAE,CAAC;IACC,WAAW,EAAE,IAAI,GACpB;EAlBb,AAoBY,UApBF,CAEN,WAAW,CASP,EAAE,CASE,MAAM,EApBlB,UAAU,CAEN,WAAW,CASP,EAAE,CXpBV,KAAK,EWSL,UAAU,CAEN,WAAW,CASP,EAAE,CLlCV,EAAE,CK2CiB;IACH,eAAe,EAAE,SAAS,GAC7B;EAtBb,AAwBY,UAxBF,CAEN,WAAW,CASP,EAAE,CAaE,EAAE,CAAC;IACC,WAAW,EAAE,KAAK,GACrB;;AA1Bb,AA8BQ,UA9BE,CAEN,WAAW,CA4BP,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EACf,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EPjEV,OAAO,GOkEV;;AAvCT,AAyCQ,UAzCE,CAEN,WAAW,CAuCP,EAAE,CAAC,EAAE,CAAC;EACF,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACzB;;AA5CT,AA8CQ,UA9CE,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAwB;EACpC,MAAM,EAAE,GAAG,CAAC,KAAK,CP3EjB,OAAO;EO4EP,KAAK,EP3EL,OAAO,GOiFV;EAvDT,AAmDY,UAnDF,CAEN,WAAW,CA4CP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP7EhB,OAAO,GO+EJ;;AAtDb,AAyDQ,UAzDE,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAAsB;EAClC,MAAM,EAAE,GAAG,CAAC,KAAK,CPpFnB,OAAO;EOqFL,KAAK,EPpFL,OAAO,GOyFV;EAjET,AA8DY,UA9DF,CAEN,WAAW,CAuDP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtFZ,OAAO,GOuFR;;AAhEb,AAmEQ,UAnEE,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CP5Ff,OAAO;EO6FT,KAAK,EP5FH,OAAO,GOiGZ;EA3ET,AAwEY,UAxEF,CAEN,WAAW,CAiEP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EP5FZ,OAAO,GO6FR;;AA1Eb,AA6EQ,UA7EE,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAAC;EACR,UAAU,EAAE,OAA0B;EACtC,MAAM,EAAE,GAAG,CAAC,KAAK,CPlGf,OAAO;EOmGT,KAAK,EPlGD,OAAO,GOuGd;EArFT,AAkFY,UAlFF,CAEN,WAAW,CA2EP,QAAQ,GAAC,EAAE,CAKP,EAAE,CAAC;IACC,UAAU,EPtGZ,OAAO,GOuGR;;AAKb,AAAA,QAAQ,CAAC;EACL,UAAU,EtG7GK,OAAO;EsG8GtB,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI,GACnB;;AAGD,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GASjB;EAhBD,AASI,gBATY,CASZ,CAAC,CAAC;IACE,KAAK,EfqRD,OAA4B,GehRnC;IAfL,AAYQ,gBAZQ,CASZ,CAAC,AAGI,MAAM,CAAC;MACJ,KAAK,EhBkTL,KAAK,GgBjTR;;AAKT,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG;EAChB,KAAK,EhBkSG,KAAK,GgB7RhB;EAfD,AAYI,KAZC,CAAC,YAAY,CAYd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;ApBxIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWf1C,AAAA,QAAQ,CS4JK;IACL,KAAK,EtGlJW,KAAK,GsGmJxB;ERlKL,AAAA,KAAK,CQoKK;IACF,WAAW,EtGtJK,KAAK,GsGuJxB;;ApBtJH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;EWZ1C,AAAA,QAAQ,CSsKK;IACL,KAAK,EtG5JW,KAAK;IsG6JrB,IAAI,EtG7JY,MAAK,GsG8JxB;ER7KL,AAAA,KAAK,CQ+KK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAsBnB;IAvBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAOI,eAPW,CAOX,KAAK,CAAC;MACF,WAAW,EtG7KC,KAAK;MsG+KjB,QAAQ,EAAE,MAAM,GACnB;IAXL,AAaI,eAbW,CAaX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAE,CAAC;MACP,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAE,EAAE;MACX,UAAU,EAAE,wBAAuB;MACnC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACf,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACrC,GAAG,EAAE,IAAI;EACT,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,KAAuB;EAC9B,gBAAgB,E1B9KL,OAAO;E0B+KlB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB7B;EAjCD,AAiBI,kBAjBc,AAiBb,MAAM,CAAC;IACJ,gBAAgB,EAAE,OAAoB,GACzC;EAED,AAAA,GAAG,CArBP,kBAAkB,CAqBR;IACF,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKrB;IAXD,AAQI,GARD,CArBP,kBAAkB,AA6BT,MAAM,CAAC;MACJ,gBAAgB,EAAE,OAAO,GAC5B;;AAKT,AAAA,eAAe,CAAC;EACZ,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC/B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/css/theme.css b/themes/learn2-git-sync/css/theme.css
deleted file mode 100644
index 2263ddd..0000000
--- a/themes/learn2-git-sync/css/theme.css
+++ /dev/null
@@ -1,979 +0,0 @@
-@charset "UTF-8";
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-#top-github-link, #body #breadcrumbs {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%); }
-
-.button, .button-secondary {
- display: inline-block;
- padding: 7px 12px; }
- .button:active, .button-secondary:active {
- margin: 2px 0 -2px 0; }
-
-body {
- background: #fff;
- color: #555;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale; }
-
-a {
- color: #1694CA; }
- a:hover {
- color: #0e6185; }
-
-#body-inner a:hover {
- text-decoration: underline;
- text-decoration-style: dotted; }
-
-pre {
- position: relative; }
-
-.bg {
- background: #fff;
- border: 1px solid #eaeaea; }
-
-b, strong, label, th {
- font-weight: 600; }
-
-.default-animation, #header #logo-svg, #header #logo-svg path, #sidebar, #sidebar ul, #body, #body .padding, #body .nav {
- -webkit-transition: all 0.5s ease;
- -moz-transition: all 0.5s ease;
- transition: all 0.5s ease; }
-
-fieldset {
- border: 1px solid #ddd; }
-
-textarea, input[type="email"], input[type="number"], input[type="password"], input[type="search"], input[type="tel"], input[type="text"], input[type="url"], input[type="color"], input[type="date"], input[type="datetime"], input[type="datetime-local"], input[type="month"], input[type="time"], input[type="week"], select[multiple=multiple] {
- background-color: white;
- border: 1px solid #ddd;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06); }
- textarea:hover, input[type="email"]:hover, input[type="number"]:hover, input[type="password"]:hover, input[type="search"]:hover, input[type="tel"]:hover, input[type="text"]:hover, input[type="url"]:hover, input[type="color"]:hover, input[type="date"]:hover, input[type="datetime"]:hover, input[type="datetime-local"]:hover, input[type="month"]:hover, input[type="time"]:hover, input[type="week"]:hover, select[multiple=multiple]:hover {
- border-color: #c4c4c4; }
- textarea:focus, input[type="email"]:focus, input[type="number"]:focus, input[type="password"]:focus, input[type="search"]:focus, input[type="tel"]:focus, input[type="text"]:focus, input[type="url"]:focus, input[type="color"]:focus, input[type="date"]:focus, input[type="datetime"]:focus, input[type="datetime-local"]:focus, input[type="month"]:focus, input[type="time"]:focus, input[type="week"]:focus, select[multiple=multiple]:focus {
- border-color: #1694CA;
- box-shadow: inset 0 1px 3px rgba(0, 0, 0, 0.06), 0 0 5px rgba(19, 131, 179, 0.7); }
-
-#header {
- background: #1694CA;
- color: #fff;
- text-align: center;
- padding: 0rem 1rem 2rem 1rem; }
- #header a {
- display: inline-block; }
- #header #logo-svg {
- width: 8rem;
- height: 2rem; }
- #header #logo-svg path {
- fill: #fff; }
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
- border: 1px solid #19a5e1;
- background: #1383b3;
- border-radius: 4px; }
- .searchbox label {
- color: rgba(255, 255, 255, 0.8);
- position: absolute;
- left: 10px;
- top: 3px; }
- .searchbox span {
- color: rgba(255, 255, 255, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer; }
- .searchbox span:hover {
- color: rgba(255, 255, 255, 0.9); }
- .searchbox input {
- display: inline-block;
- color: #fff;
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: 400; }
- .searchbox input::-webkit-input-placeholder {
- color: rgba(255, 255, 255, 0.6); }
- .searchbox input::-moz-placeholder {
- color: rgba(255, 255, 255, 0.6); }
- .searchbox input:-moz-placeholder {
- color: rgba(255, 255, 255, 0.6); }
- .searchbox input:-ms-input-placeholder {
- color: rgba(255, 255, 255, 0.6); }
-
-#sidebar-toggle {
- display: none; }
- @media only all and (max-width: 47.938em) {
- #sidebar-toggle {
- display: inline-block; } }
-
-#sidebar {
- background-color: #38424D;
- position: fixed;
- top: 0;
- width: 300px;
- bottom: 0;
- left: 0;
- font-weight: 500;
- font-size: 15px; }
- #sidebar a {
- color: #ffffff; }
- #sidebar a:hover, #sidebar a.button {
- color: white; }
- #sidebar a.subtitle {
- color: rgba(204, 204, 204, 0.6); }
- #sidebar hr {
- border-bottom: 1px solid #323a44; }
- #sidebar a.padding {
- padding: 0 1rem; }
- #sidebar h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2; }
- #sidebar h5 a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar h5 i {
- color: rgba(204, 204, 204, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%; }
- #sidebar h5.parent a {
- background: #293038;
- color: #d9d9d9 !important; }
- #sidebar h5.active a {
- background: #fff;
- color: #555 !important; }
- #sidebar h5.active i {
- color: #555 !important; }
- #sidebar h5 + ul.topics {
- display: none;
- margin-top: 0; }
- #sidebar h5.parent + ul.topics, #sidebar h5.active + ul.topics {
- display: block; }
- #sidebar ul {
- list-style: none;
- padding: 0;
- margin: 0; }
- #sidebar ul.searched a {
- color: #999999; }
- #sidebar ul.searched .search-match a {
- color: #e6e6e6; }
- #sidebar ul.searched .search-match a:hover {
- color: white; }
- #sidebar ul.topics {
- margin: 0 1rem; }
- #sidebar ul.topics.searched ul {
- display: block; }
- #sidebar ul.topics ul {
- display: none;
- padding-bottom: 1rem; }
- #sidebar ul.topics ul ul {
- padding-bottom: 0; }
- #sidebar ul.topics li.parent ul, #sidebar ul.topics > li.active ul {
- display: block; }
- #sidebar ul.topics > li > a {
- line-height: 2rem;
- font-size: 1.1rem; }
- #sidebar ul.topics > li > a b {
- opacity: 0.5;
- font-weight: normal; }
- #sidebar ul.topics > li > a .fa {
- margin-top: 9px; }
- #sidebar ul.topics > li.parent, #sidebar ul.topics > li.active {
- background: #2d353e;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li.active > a {
- background: #fff;
- color: #555 !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem; }
- #sidebar ul li {
- padding: 0; }
- #sidebar ul li.visited + span {
- margin-right: 16px; }
- #sidebar ul li a {
- display: block;
- padding: 2px 0; }
- #sidebar ul li a span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block; }
- #sidebar ul li > a {
- padding: 4px 0; }
- #sidebar ul li .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right; }
- #sidebar ul li.visited > a .read-icon {
- color: #1694CA;
- display: inline; }
- #sidebar ul li li {
- padding-left: 1rem;
- text-indent: 0.2rem; }
-
-#main {
- background: #f7f7f7;
- margin: 0 0 1.563rem 0; }
-
-#body {
- position: relative;
- margin-left: 300px;
- min-height: 100%; }
- #body img, #body .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center; }
- #body img.border, #body .video-container.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px; }
- #body img.shadow, #body .video-container.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1); }
- #body .bordered {
- border: 1px solid #ccc; }
- #body .padding {
- padding: 3rem 6rem; }
- @media only all and (max-width: 59.938em) {
- #body .padding {
- position: static;
- padding: 15px 3rem; } }
- @media only all and (max-width: 47.938em) {
- #body .padding {
- padding: 5px 1rem; } }
- #body h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem; }
- @media only all and (max-width: 59.938em) {
- #body #navigation {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table; } }
- #body .nav {
- position: fixed;
- top: 0;
- bottom: 0;
- width: 4rem;
- font-size: 50px;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center; }
- #body .nav > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center; }
- @media only all and (max-width: 59.938em) {
- #body .nav {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0; }
- #body .nav > i {
- display: inline-block; } }
- #body .nav:hover {
- background: #F6F6F6; }
- #body .nav.nav-pref {
- left: 0; }
- #body .nav.nav-next {
- right: 0; }
-
-#body-inner {
- margin-bottom: 5rem; }
-
-#chapter {
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0; }
- #chapter #body-inner {
- padding-bottom: 3rem;
- max-width: 80%; }
- #chapter h3 {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-align: center; }
- #chapter h1 {
- font-size: 5rem;
- border-bottom: 4px solid #F0F2F4; }
- #chapter p {
- text-align: center;
- font-size: 1.2rem; }
-
-#footer {
- padding: 3rem 1rem;
- color: #b3b3b3;
- font-size: 13px; }
- #footer p {
- margin: 0; }
-
-body {
- font-family: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- letter-spacing: -0.03rem;
- font-weight: 400; }
-
-h1, h2, h3, h4, h5, h6 {
- font-family: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px; }
-
-h1 {
- text-align: center;
- letter-spacing: -3px; }
-
-h2 {
- letter-spacing: -2px; }
-
-h3 {
- letter-spacing: -1px; }
-
-blockquote {
- border-left: 10px solid #F0F2F4; }
- blockquote p {
- font-size: 1.1rem;
- color: #999; }
- blockquote cite {
- display: block;
- text-align: right;
- color: #666;
- font-size: 1.2rem; }
-
-blockquote {
- position: relative; }
-
-blockquote blockquote {
- position: static; }
-
-blockquote > blockquote > blockquote {
- margin: 0; }
- blockquote > blockquote > blockquote p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666; }
- blockquote > blockquote > blockquote p:first-child:before {
- position: absolute;
- top: 2px;
- color: #fff;
- font-family: FontAwesome;
- content: '';
- left: 10px; }
- blockquote > blockquote > blockquote p:first-child:after {
- position: absolute;
- top: 2px;
- color: #fff;
- left: 2rem;
- font-weight: bold;
- content: 'Info'; }
- blockquote > blockquote > blockquote > p {
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB; }
- blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -94px;
- border-top: 30px solid rgba(217, 83, 79, 0.8);
- background: #FAE2E2; }
- blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Warning'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA; }
- blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Note'; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p {
- margin-left: -142px;
- border-top: 30px solid rgba(92, 184, 92, 0.8);
- background: #E6F9E6; }
- blockquote > blockquote > blockquote > blockquote > blockquote > blockquote > p:first-child:after {
- content: 'Tip'; }
-
-code,
-kbd,
-pre,
-samp {
- font-family: "Inconsolata", monospace; }
-
-code {
- background: #f9f2f4;
- color: #9c1d3d;
- padding: .2rem .4rem;
- border-radius: 3px; }
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: #f6f6f6;
- border: 1px solid #ddd;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem; }
- pre code {
- color: #237794;
- background: inherit;
- font-size: 1rem; }
-
-hr {
- border-bottom: 4px solid #F0F2F4; }
-
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: #1694CA;
- color: #fff; }
-
-#body a.anchor-link {
- color: #ccc; }
-
-#body a.anchor-link:hover {
- color: #1694CA; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track {
- background-color: rgba(255, 255, 255, 0.3); }
-
-.scrollbar-inner > .scroll-element .scroll-bar {
- background-color: #b5d1eb; }
-
-.scrollbar-inner > .scroll-element:hover .scroll-bar {
- background-color: #ccc; }
-
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar {
- background-color: #ccc; }
-
-table {
- border: 1px solid #eaeaea;
- table-layout: auto; }
-
-th {
- background: #f7f7f7;
- padding: 0.5rem; }
-
-td {
- padding: 0.5rem;
- border: 1px solid #eaeaea; }
-
-.button {
- background: #1694CA;
- color: #fff;
- box-shadow: 0 3px 0 #1380ae; }
- .button:hover {
- background: #1380ae;
- box-shadow: 0 3px 0 #106c93;
- color: #fff; }
- .button:active {
- box-shadow: 0 1px 0 #106c93; }
-
-.button-secondary {
- background: #F8B450;
- color: #fff;
- box-shadow: 0 3px 0 #f7a733; }
- .button-secondary:hover {
- background: #f7a733;
- box-shadow: 0 3px 0 #f69b15;
- color: #fff; }
- .button-secondary:active {
- box-shadow: 0 1px 0 #f69b15; }
-
-.bullets {
- margin: 1.7rem 0;
- margin-left: -0.85rem;
- margin-right: -0.85rem;
- overflow: auto; }
-
-.bullet {
- float: left;
- padding: 0 0.85rem; }
-
-.two-column-bullet {
- width: 50%; }
- @media only all and (max-width: 47.938em) {
- .two-column-bullet {
- width: 100%; } }
-
-.three-column-bullet {
- width: 33.33333%; }
- @media only all and (max-width: 47.938em) {
- .three-column-bullet {
- width: 100%; } }
-
-.four-column-bullet {
- width: 25%; }
- @media only all and (max-width: 47.938em) {
- .four-column-bullet {
- width: 100%; } }
-
-.bullet-icon {
- float: left;
- background: #1694CA;
- padding: 0.875rem;
- width: 3.5rem;
- height: 3.5rem;
- border-radius: 50%;
- color: #fff;
- font-size: 1.75rem;
- text-align: center; }
-
-.bullet-icon-1 {
- background: #1694CA; }
-
-.bullet-icon-2 {
- background: #16cac4; }
-
-.bullet-icon-3 {
- background: #b2ca16; }
-
-.bullet-content {
- margin-left: 4.55rem; }
-
-.tooltipped {
- position: relative; }
-
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
- color: #fff;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: rgba(0, 0, 0, 0.8);
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased; }
-
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: rgba(0, 0, 0, 0.8);
- pointer-events: none;
- content: "";
- border: 5px solid transparent; }
-
-.tooltipped:hover:before, .tooltipped:hover:after,
-.tooltipped:active:before,
-.tooltipped:active:after,
-.tooltipped:focus:before,
-.tooltipped:focus:after {
- display: inline-block;
- text-decoration: none; }
-
-.tooltipped-s:after,
-.tooltipped-se:after,
-.tooltipped-sw:after {
- top: 100%;
- right: 50%;
- margin-top: 5px; }
-
-.tooltipped-s:before,
-.tooltipped-se:before,
-.tooltipped-sw:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-se:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-sw:after {
- margin-right: -15px; }
-
-.tooltipped-n:after,
-.tooltipped-ne:after,
-.tooltipped-nw:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px; }
-
-.tooltipped-n:before,
-.tooltipped-ne:before,
-.tooltipped-nw:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-ne:after {
- right: auto;
- left: 50%;
- margin-left: -15px; }
-
-.tooltipped-nw:after {
- margin-right: -15px; }
-
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%); }
-
-.tooltipped-w:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%); }
-
-.tooltipped-w:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: rgba(0, 0, 0, 0.8); }
-
-.tooltipped-e:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%); }
-
-.tooltipped-e:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: rgba(0, 0, 0, 0.8); }
-
-/*************** SCROLLBAR BASE CSS ***************/
-.highlightable {
- padding: 25px 0 15px; }
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative; }
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important; }
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-.scroll-element {
- display: none; }
-
-.scroll-element, .scroll-element div {
- box-sizing: content-box; }
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block; }
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default; }
-
-.scroll-textarea > .scroll-content {
- overflow: hidden !important; }
-
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important; }
-
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0; }
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div {
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10; }
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%; }
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden; }
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px; }
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3; }
-
-/* update scrollbar offset if both scrolls are visible */
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track {
- top: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size {
- left: -12px; }
-
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size {
- top: -12px; }
-
-.lightbox-active #body {
- overflow: visible; }
- .lightbox-active #body .padding {
- overflow: visible; }
-
-#github-contrib i {
- vertical-align: middle; }
-
-.featherlight img {
- margin: 0 !important; }
-
-.lifecycle #body-inner ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative; }
-
-.lifecycle #body-inner ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative; }
- .lifecycle #body-inner ol li {
- margin-left: 1rem; }
- .lifecycle #body-inner ol strong, .lifecycle #body-inner ol label, .lifecycle #body-inner ol th {
- text-decoration: underline; }
- .lifecycle #body-inner ol ol {
- margin-left: -1rem; }
-
-.lifecycle #body-inner h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: #1ABC9C; }
-
-.lifecycle #body-inner ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important; }
-
-.lifecycle #body-inner .level-1 + ol {
- background: #f6fefc;
- border: 4px solid #1ABC9C;
- color: #16A085; }
- .lifecycle #body-inner .level-1 + ol h3 {
- background: #2ECC71; }
-
-.lifecycle #body-inner .level-2 + ol {
- background: #f7fdf9;
- border: 4px solid #2ECC71;
- color: #27AE60; }
- .lifecycle #body-inner .level-2 + ol h3 {
- background: #3498DB; }
-
-.lifecycle #body-inner .level-3 + ol {
- background: #f3f9fd;
- border: 4px solid #3498DB;
- color: #2980B9; }
- .lifecycle #body-inner .level-3 + ol h3 {
- background: #34495E; }
-
-.lifecycle #body-inner .level-4 + ol {
- background: #e4eaf0;
- border: 4px solid #34495E;
- color: #2C3E50; }
- .lifecycle #body-inner .level-4 + ol h3 {
- background: #34495E; }
-
-#top-bar {
- background: #F6F6F6;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem; }
-
-#top-github-link {
- position: relative;
- z-index: 1;
- float: right;
- display: block; }
-
-#body #breadcrumbs {
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
- line-height: 1.4; }
- #body #breadcrumbs span {
- padding: 0 0.1rem; }
-
-@media only all and (max-width: 59.938em) {
- #sidebar {
- width: 230px; }
- #body {
- margin-left: 230px; } }
-
-@media only all and (max-width: 47.938em) {
- #sidebar {
- width: 230px;
- left: -230px; }
- #body {
- margin-left: 0;
- width: 100%; }
- .sidebar-hidden {
- overflow: hidden; }
- .sidebar-hidden #sidebar {
- left: 0; }
- .sidebar-hidden #body {
- margin-left: 230px;
- overflow: hidden; }
- .sidebar-hidden #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, 0.5);
- cursor: pointer; } }
-
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: 1.45rem;
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: #3c3c3c;
- background-color: #f9f2f4;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0; }
- .copy-to-clipboard:hover {
- background-color: #f1e1e5; }
- pre .copy-to-clipboard {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px; }
- pre .copy-to-clipboard:hover {
- background-color: #d9d9d9; }
-
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d; }
-
-.version-chooser select {
- display: inline-block;
- color: #000000;
- background-color: #FFFFFF;
- border: 1px solid #666666;
- font-size: 15px;
- font-weight: regular;
- margin: 0;
-}
-
-.videoWrapper {
- position: relative;
- padding-bottom: 56.25%; /* 16:9 */
- padding-top: 25px;
- height: 0;
-}
-.videoWrapper iframe {
- position: absolute;
- top: 0;
- left: 0;
- width: 100%;
- height: 100%;
-}
-
-/*# sourceMappingURL=theme.css.map */
diff --git a/themes/learn2-git-sync/css/theme.css.map b/themes/learn2-git-sync/css/theme.css.map
deleted file mode 100644
index e4218d4..0000000
--- a/themes/learn2-git-sync/css/theme.css.map
+++ /dev/null
@@ -1,107 +0,0 @@
-{
- "version": 3,
- "file": "theme.css",
- "sources": [
- "../scss/theme.scss",
- "../scss/vendor/bourbon/_bourbon.scss",
- "../scss/vendor/bourbon/settings/_prefixer.scss",
- "../scss/vendor/bourbon/settings/_px-to-em.scss",
- "../scss/vendor/bourbon/helpers/_convert-units.scss",
- "../scss/vendor/bourbon/helpers/_gradient-positions-parser.scss",
- "../scss/vendor/bourbon/helpers/_is-num.scss",
- "../scss/vendor/bourbon/helpers/_linear-angle-parser.scss",
- "../scss/vendor/bourbon/helpers/_linear-gradient-parser.scss",
- "../scss/vendor/bourbon/helpers/_linear-positions-parser.scss",
- "../scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss",
- "../scss/vendor/bourbon/helpers/_radial-arg-parser.scss",
- "../scss/vendor/bourbon/helpers/_radial-positions-parser.scss",
- "../scss/vendor/bourbon/helpers/_radial-gradient-parser.scss",
- "../scss/vendor/bourbon/helpers/_render-gradients.scss",
- "../scss/vendor/bourbon/helpers/_shape-size-stripper.scss",
- "../scss/vendor/bourbon/helpers/_str-to-num.scss",
- "../scss/vendor/bourbon/functions/_assign.scss",
- "../scss/vendor/bourbon/functions/_color-lightness.scss",
- "../scss/vendor/bourbon/functions/_flex-grid.scss",
- "../scss/vendor/bourbon/functions/_golden-ratio.scss",
- "../scss/vendor/bourbon/functions/_grid-width.scss",
- "../scss/vendor/bourbon/functions/_modular-scale.scss",
- "../scss/vendor/bourbon/functions/_px-to-em.scss",
- "../scss/vendor/bourbon/functions/_px-to-rem.scss",
- "../scss/vendor/bourbon/functions/_strip-units.scss",
- "../scss/vendor/bourbon/functions/_tint-shade.scss",
- "../scss/vendor/bourbon/functions/_transition-property-name.scss",
- "../scss/vendor/bourbon/functions/_unpack.scss",
- "../scss/vendor/bourbon/css3/_animation.scss",
- "../scss/vendor/bourbon/css3/_appearance.scss",
- "../scss/vendor/bourbon/css3/_backface-visibility.scss",
- "../scss/vendor/bourbon/css3/_background.scss",
- "../scss/vendor/bourbon/css3/_background-image.scss",
- "../scss/vendor/bourbon/css3/_border-image.scss",
- "../scss/vendor/bourbon/css3/_border-radius.scss",
- "../scss/vendor/bourbon/css3/_box-sizing.scss",
- "../scss/vendor/bourbon/css3/_calc.scss",
- "../scss/vendor/bourbon/css3/_columns.scss",
- "../scss/vendor/bourbon/css3/_filter.scss",
- "../scss/vendor/bourbon/css3/_flex-box.scss",
- "../scss/vendor/bourbon/css3/_font-face.scss",
- "../scss/vendor/bourbon/css3/_hyphens.scss",
- "../scss/vendor/bourbon/css3/_hidpi-media-query.scss",
- "../scss/vendor/bourbon/css3/_image-rendering.scss",
- "../scss/vendor/bourbon/css3/_keyframes.scss",
- "../scss/vendor/bourbon/css3/_linear-gradient.scss",
- "../scss/vendor/bourbon/css3/_perspective.scss",
- "../scss/vendor/bourbon/css3/_radial-gradient.scss",
- "../scss/vendor/bourbon/css3/_transform.scss",
- "../scss/vendor/bourbon/css3/_transition.scss",
- "../scss/vendor/bourbon/css3/_user-select.scss",
- "../scss/vendor/bourbon/css3/_placeholder.scss",
- "../scss/vendor/bourbon/addons/_button.scss",
- "../scss/vendor/bourbon/addons/_clearfix.scss",
- "../scss/vendor/bourbon/addons/_directional-values.scss",
- "../scss/vendor/bourbon/addons/_ellipsis.scss",
- "../scss/vendor/bourbon/addons/_font-family.scss",
- "../scss/vendor/bourbon/addons/_hide-text.scss",
- "../scss/vendor/bourbon/addons/_html5-input-types.scss",
- "../scss/vendor/bourbon/addons/_position.scss",
- "../scss/vendor/bourbon/addons/_prefixer.scss",
- "../scss/vendor/bourbon/addons/_retina-image.scss",
- "../scss/vendor/bourbon/addons/_size.scss",
- "../scss/vendor/bourbon/addons/_timing-functions.scss",
- "../scss/vendor/bourbon/addons/_triangle.scss",
- "../scss/vendor/bourbon/addons/_word-wrap.scss",
- "../scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss",
- "../scss/configuration/nucleus/_base.scss",
- "../scss/configuration/nucleus/_core.scss",
- "../scss/configuration/nucleus/_breakpoints.scss",
- "../scss/configuration/nucleus/_layout.scss",
- "../scss/configuration/nucleus/_typography.scss",
- "../scss/configuration/nucleus/_nav.scss",
- "../scss/configuration/theme/_base.scss",
- "../scss/configuration/theme/_colors.scss",
- "../scss/configuration/theme/_bullets.scss",
- "../scss/nucleus/functions/_base.scss",
- "../scss/nucleus/functions/_direction.scss",
- "../scss/nucleus/functions/_range.scss",
- "../scss/nucleus/mixins/_base.scss",
- "../scss/nucleus/mixins/_breakpoints.scss",
- "../scss/nucleus/mixins/_utilities.scss",
- "../scss/theme/modules/_base.scss",
- "../scss/theme/modules/_buttons.scss",
- "../scss/theme/_configuration.scss",
- "../scss/theme/_core.scss",
- "../scss/theme/_fonts.scss",
- "../scss/theme/_forms.scss",
- "../scss/theme/_header.scss",
- "../scss/theme/_nav.scss",
- "../scss/theme/_main.scss",
- "../scss/theme/_typography.scss",
- "../scss/theme/_tables.scss",
- "../scss/theme/_buttons.scss",
- "../scss/theme/_bullets.scss",
- "../scss/theme/_tooltips.scss",
- "../scss/theme/_scrollbar.scss",
- "../scss/theme/_custom.scss"
- ],
- "names": [],
- "mappings": ";AuFCA,OAAO,CAAC,8EAAI;AWuHZ,AhB9GA,gBgB8GgB,EAWhB,KAAK,CAAC,YAAY,ChBzHF;EACf,QAAQ,EAAE,QAAQ;EAClB,GAAG,EAAE,GAAG;EACR,iBAAiB,EAAE,gBAAgB;EACnC,cAAc,EAAE,gBAAgB;EAChC,YAAY,EAAE,gBAAgB;EAC9B,aAAa,EAAE,gBAAgB;EAC/B,SAAS,EAAE,gBAAgB,GAC3B;;AYlBD,AVAA,OUAO,EAKP,iBAAiB,CVLT;EACP,OAAO,EAAE,YAAY;EACrB,OAAO,EAAE,QAAQ,GAIjB;EUND,AVGC,OUHM,AVGL,OAAO,EUET,iBAAiB,AVFf,OAAO,CAAC;IACR,MAAM,EAAE,YAAY,GACpB;;AELF,AAAA,IAAI,CAAC;EACJ,UAAU,EXiBI,IAAI;EWhBlB,KAAK,EXDW,IAAI;EWEjB,sBAAsB,EAAE,WAAW;EACnC,uBAAuB,EAAE,SAAS,GACrC;;AAED,AAAA,CAAC,CAAC;EACD,KAAK,EXNY,OAAO,GWUxB;EALD,AAEC,CAFA,AAEC,MAAM,CAAC;IACP,KAAK,EAAE,OAAyB,GAChC;;AAGF,AAEE,WAFS,CACV,CAAC,AACC,MAAM,CAAC;EACP,eAAe,EAAE,SAAS;EAC1B,qBAAqB,EAAE,MAAM,GAC7B;;AAIH,AAAA,GAAG,CAAC;EACH,QAAQ,EAAE,QAAQ,GAClB;;AAED,AAAA,GAAG,CAAC;EACH,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,GAAG,CAAC,KAAK,CXMA,OAAoB,GWLrC;;AAED,AAAA,CAAC,EAAE,MAAM,EEbT,KAAK,EKdL,EAAE,CP2BQ;EACN,WAAW,EDnBI,GAAG,GCoBrB;;AAED,AAAA,kBAAkB,EGpClB,OAAO,CAWH,SAAS,EAXb,OAAO,CAWH,SAAS,CAKL,IAAI,ECPZ,QAAQ,EAAR,QAAQ,CAoFJ,EAAE,ECxFN,KAAK,EAAL,KAAK,CAyBD,QAAQ,EAzBZ,KAAK,CAsDD,IAAI,CLvBW;EzB3BX,kBAAoB,EyB4BJ,GAAG,CAAC,IAAI,CAAC,IAAI;EzBvB7B,eAAiB,EyBuBD,GAAG,CAAC,IAAI,CAAC,IAAI;EzBR7B,UAAY,EyBQI,GAAG,CAAC,IAAI,CAAC,IAAI,GACpC;;AEtCD,AAAA,QAAQ,CAAC;EACR,MAAM,EAAE,GAAG,CAAC,KAAK,CbKD,IAAI,GaJpB;;AAED,AAAA,QAAQ,EAAE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,GAAgB,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,GAAa,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,GAAkB,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,GAAwB,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,GAAe,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,GAAc,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,EAAvQ;EACxD,gBAAgB,EAAE,KAAK;EACvB,MAAM,EAAE,GAAG,CAAC,KAAK,CbAD,IAAI;EaCpB,UAAU,EbOW,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,GaG1D;EAbD,AAKC,QALO,AAKN,MAAM,EALE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKd,MAAM,EALuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKnC,MAAM,EAL6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAKzD,MAAM,EALqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CAKjF,MAAM,EAL2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAKvG,MAAM,EAL8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAK1H,MAAM,EALkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAK9I,MAAM,EALqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKjK,MAAM,EAL0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKtL,MAAM,EAL8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAK1M,MAAM,EALsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CAKlO,MAAM,EALoP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAKhQ,MAAM,EALyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKrR,MAAM,EAL6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAKzS,MAAM,EALiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAK9T,MAAM,CAAC;IACP,YAAY,EbAc,OAA8B,GaCxD;EAPF,AASC,QATO,AASN,MAAM,EATE,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASd,MAAM,EATuB,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASnC,MAAM,EAT6C,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CASzD,MAAM,EATqE,KAAK,CAAA,AAAA,IAAC,CAAK,QAAQ,AAAb,CASjF,MAAM,EAT2F,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CASvG,MAAM,EAT8G,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAS1H,MAAM,EATkI,KAAK,CAAA,AAAA,IAAC,CAAK,KAAK,AAAV,CAS9I,MAAM,EATqJ,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CASjK,MAAM,EAT0K,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CAStL,MAAM,EAT8L,KAAK,CAAA,AAAA,IAAC,CAAK,UAAU,AAAf,CAS1M,MAAM,EATsN,KAAK,CAAA,AAAA,IAAC,CAAK,gBAAgB,AAArB,CASlO,MAAM,EAToP,KAAK,CAAA,AAAA,IAAC,CAAK,OAAO,AAAZ,CAShQ,MAAM,EATyQ,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASrR,MAAM,EAT6R,KAAK,CAAA,AAAA,IAAC,CAAK,MAAM,AAAX,CASzS,MAAM,EATiT,MAAM,CAAA,AAAA,QAAC,CAAD,QAAC,AAAA,CAS9T,MAAM,CAAC;IACP,YAAY,EbZI,OAAO;IaavB,UAAU,EbDU,KAAK,CAAC,CAAC,CAAC,GAAG,CAAC,GAAG,CAAC,mBAAqB,EACf,CAAC,CAAC,CAAC,CAAC,GAAG,CAAM,uBAAmC,GaC1F;;AChBF,AAAA,OAAO,CAAC;EACJ,UAAU,EdCI,OAAO;EcArB,KAAK,EdEK,IAAI;EcDd,UAAU,EAAE,MAAM;EAElB,OAAO,EAAE,IAAI,GAgBhB;EArBD,AAOI,OAPG,CAOH,CAAC,CAAC;IACE,OAAO,EAAE,YAAY,GACxB;EATL,AAWI,OAXG,CAWH,SAAS,CAAC;IAEN,KAAK,EJQA,IAAI;IIPT,MAAM,EJQA,IAAI,GIFb;IApBL,AAgBQ,OAhBD,CAWH,SAAS,CAKL,IAAI,CAAC;MAED,IAAI,EddF,IAAI,GceT;;AAIT,AAAA,UAAU,CAAC;EACP,UAAU,EAAE,MAAM;EAClB,QAAQ,EAAE,QAAQ;EAElB,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAAuB;EACzC,UAAU,EAAE,OAAqB;EACjC,aAAa,EAAE,GAAG,GAoCrB;EA1CD,AAQI,UARM,CAQN,KAAK,CAAC;IACF,KAAK,Ed5BC,wBAAI;Ic6BV,QAAQ,EAAE,QAAQ;IAClB,IAAI,EAAE,IAAI;IACV,GAAG,EAAE,GAAG,GACX;EAbL,AAeI,UAfM,CAeN,IAAI,CAAC;IACD,KAAK,EdnCC,wBAAI;IcoCV,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,IAAI;IACX,GAAG,EAAE,GAAG;IACR,MAAM,EAAE,OAAO,GAKlB;IAzBL,AAsBQ,UAtBE,CAeN,IAAI,AAOC,MAAM,CAAC;MACJ,KAAK,Ed1CH,wBAAI,Gc2CT;EAxBT,AA2BI,UA3BM,CA2BN,KAAK,CAAC;IACF,OAAO,EAAE,YAAY;IACrB,KAAK,EdhDC,IAAI;IciDV,KAAK,EAAE,IAAI;IACX,MAAM,EAAE,IAAI;IACZ,UAAU,EAAE,WAAW;IACvB,MAAM,EAAE,CAAC;IACT,OAAO,EAAE,aAAa;IACtB,MAAM,EAAE,CAAC;IACT,WAAW,EJ3CG,GAAG,GIgDpB;IAzCL,ArCpBI,UqCoBM,CA2BN,KAAK,ArC/CJ,2BAA2B,CAAE;MqC2DtB,KAAK,Ed1DH,wBAAI,GvBCb;IqCkBL,ArCpBI,UqCoBM,CA2BN,KAAK,ArC/CJ,kBAAkB,CAAW;MqC2DtB,KAAK,Ed1DH,wBAAI,GvBCb;IqCkBL,ArCpBI,UqCoBM,CA2BN,KAAK,ArC/CJ,iBAAiB,CAAY;MqC2DtB,KAAK,Ed1DH,wBAAI,GvBCb;IqCkBL,ArCpBI,UqCoBM,CA2BN,KAAK,ArC/CJ,sBAAsB,CAAO;MqC2DtB,KAAK,Ed1DH,wBAAI,GvBCb;;AsCLL,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAMhB;ETcC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;ISrB1C,AAAA,eAAe,CAAC;MAIP,OAAO,EAAE,YAAY,GAG7B;;AAED,AAAA,QAAQ,CAAC;EAEL,gBAAgB,ELPP,OAAO;EKQhB,QAAQ,EAAE,KAAK;EACf,GAAG,EAAE,CAAC;EACN,KAAK,ELZO,KAAK;EKajB,MAAM,EAAE,CAAC;EACT,IAAI,EAAE,CAAC;EACP,WAAW,ELFM,GAAG;EKGpB,SAAS,EAAE,IAAI,GAkMlB;EA3MD,AAWI,QAXI,CAWJ,CAAC,CAAC;IACE,KAAK,ELfE,OAAO,GKuBjB;IApBL,AAaQ,QAbA,CAWJ,CAAC,AAEI,MAAM,EAbf,QAAQ,CAWJ,CAAC,AAGI,OAAO,CAAC;MACL,KAAK,EAAE,KAA4B,GACtC;IAhBT,AAiBQ,QAjBA,CAWJ,CAAC,AAMI,SAAS,CAAC;MACP,KAAK,ELrBF,wBAAO,GKsBb;EAnBT,AAsBI,QAtBI,CAsBJ,EAAE,CAAC;IACC,aAAa,EAAE,GAAG,CAAC,KAAK,CAAC,OAAuB,GACnD;EAxBL,AA0BI,QA1BI,CA0BJ,CAAC,AAAA,QAAQ,CAAC;IACN,OAAO,EAAE,MAAM,GAClB;EA5BL,AA8BI,QA9BI,CA8BJ,EAAE,CAAC;IACC,MAAM,EAAE,QAAQ;IAChB,QAAQ,EAAE,QAAQ;IAClB,WAAW,EAAE,CAAC,GAqCjB;IAtEL,AAmCQ,QAnCA,CA8BJ,EAAE,CAKE,CAAC,CAAC;MACE,OAAO,EAAE,KAAK;MACd,WAAW,EAAE,CAAC;MACd,YAAY,EAAE,CAAC;MACf,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IAzCT,AA2CQ,QA3CA,CA8BJ,EAAE,CAaE,CAAC,CAAC;MACE,KAAK,EL/CF,wBAAO;MKgDV,QAAQ,EAAE,QAAQ;MAClB,KAAK,EAAE,MAAM;MACb,GAAG,EAAE,MAAM;MACX,SAAS,EAAE,GAAG,GACjB;IAjDT,AAoDY,QApDJ,CA8BJ,EAAE,AAqBG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EAAE,OAAuB;MACnC,KAAK,EAAE,OAA0B,CAAC,UAAU,GAC/C;IAvDb,AA2DY,QA3DJ,CA8BJ,EAAE,AA4BG,OAAO,CACJ,CAAC,CAAC;MACE,UAAU,EfjEZ,IAAI;MekEF,KAAK,EfrEJ,IAAI,CeqEa,UAAU,GAC/B;IA9Db,AAgEY,QAhEJ,CA8BJ,EAAE,AA4BG,OAAO,CAMJ,CAAC,CAAC;MACE,KAAK,EfzEJ,IAAI,CeyEa,UAAU,GAC/B;EAlEb,AAwEI,QAxEI,CAwEJ,EAAE,GAAG,EAAE,AAAA,OAAO,CAAC;IACX,OAAO,EAAE,IAAI;IACb,UAAU,EAAE,CAAC,GAChB;EA3EL,AA8EQ,QA9EA,CA6EJ,EAAE,AAAA,OAAO,GACH,EAAE,AAAA,OAAO,EA9EnB,QAAQ,CA6EO,EAAE,AAAA,OAAO,GACd,EAAE,AAAA,OAAO,CAAC;IACR,OAAO,EAAE,KAAK,GACjB;EAhFT,AAoFI,QApFI,CAoFJ,EAAE,CAAC;IAEC,UAAU,EAAE,IAAI;IAChB,OAAO,EAAE,CAAC;IACV,MAAM,EAAE,CAAC,GAkHZ;IA1ML,AA2FY,QA3FJ,CAoFJ,EAAE,AAMG,SAAS,CACN,CAAC,CAAC;MACE,KAAK,EAAE,OAA0B,GACpC;IA7Fb,AAgGgB,QAhGR,CAoFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,CAAC;MACE,KAAK,EAAE,OAA2B,GAIrC;MArGjB,AAkGoB,QAlGZ,CAoFJ,EAAE,AAMG,SAAS,CAKN,aAAa,CACT,CAAC,AAEI,MAAM,CAAC;QACJ,KAAK,EAAE,KAA2B,GACrC;IApGrB,AAyGQ,QAzGA,CAoFJ,EAAE,AAqBG,OAAO,CAAC;MACL,MAAM,EAAE,MAAM,GA4CjB;MAtJT,AA6GgB,QA7GR,CAoFJ,EAAE,AAqBG,OAAO,AAGH,SAAS,CACN,EAAE,CAAC;QACC,OAAO,EAAE,KAAK,GACjB;MA/GjB,AAkHY,QAlHJ,CAoFJ,EAAE,AAqBG,OAAO,CASJ,EAAE,CAAC;QACC,OAAO,EAAE,IAAI;QACb,cAAc,EAAE,IAAI,GAKvB;QAzHb,AAsHgB,QAtHR,CAoFJ,EAAE,AAqBG,OAAO,CASJ,EAAE,CAIE,EAAE,CAAC;UACC,cAAc,EAAE,CAAC,GACpB;MAxHjB,AA2HY,QA3HJ,CAoFJ,EAAE,AAqBG,OAAO,CAkBJ,EAAE,AAAA,OAAO,CAAC,EAAE,EA3HxB,QAAQ,CAoFJ,EAAE,AAqBG,OAAO,GAkBY,EAAE,AAAA,OAAO,CAAC,EAAE,CAAC;QACzB,OAAO,EAAE,KAAK,GACjB;MA7Hb,AAgIgB,QAhIR,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CAAC;QACA,WAAW,EAAE,IAAI;QACjB,SAAS,EAAE,MAAM,GAUpB;QA5IjB,AAoIoB,QApIZ,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CAIC,CAAC,CAAC;UACE,OAAO,EAAE,GAAG;UACZ,WAAW,EAAE,MAAM,GACtB;QAvIrB,AAyIoB,QAzIZ,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,GACE,CAAC,CASC,GAAG,CAAC;UACA,UAAU,EAAE,GAAG,GAClB;MA3IrB,AA8IgB,QA9IR,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeC,OAAO,EA9IxB,QAAQ,CAoFJ,EAAE,AAqBG,OAAO,GAsBF,EAAE,AAeW,OAAO,CAAC;QACf,UAAU,EAAE,OAAuB;QACnC,WAAW,EAAE,KAAK;QAClB,YAAY,EAAE,KAAK;QACnB,YAAY,EAAE,IAAI;QAClB,aAAa,EAAE,IAAI,GACtB;IApJjB,AAwJQ,QAxJA,CAoFJ,EAAE,CAoEE,EAAE,AAAA,OAAO,GAAG,CAAC,CAAC;MACV,UAAU,Ef9JR,IAAI;Me+JN,KAAK,EflKA,IAAI,CekKS,UAAU;MAC5B,WAAW,EAAE,KAAK;MAClB,YAAY,EAAE,KAAK;MACnB,YAAY,EAAE,IAAI;MAClB,aAAa,EAAE,IAAI,GACtB;IA/JT,AAiKQ,QAjKA,CAoFJ,EAAE,CA6EE,EAAE,CAAC;MACC,OAAO,EAAE,CAAC,GAsCb;MAxMT,AAmKY,QAnKJ,CAoFJ,EAAE,CA6EE,EAAE,AAEG,QAAQ,GAAG,IAAI,CAAC;QACb,YAAY,EAAE,IAAI,GACrB;MArKb,AAsKY,QAtKJ,CAoFJ,EAAE,CA6EE,EAAE,CAKE,CAAC,CAAC;QACE,OAAO,EAAE,KAAK;QACd,OAAO,EAAE,KAAK,GAOjB;QA/Kb,AAyKgB,QAzKR,CAoFJ,EAAE,CA6EE,EAAE,CAKE,CAAC,CAGG,IAAI,CAAC;UACD,aAAa,EAAE,QAAQ;UACvB,QAAQ,EAAE,MAAM;UAChB,WAAW,EAAE,MAAM;UACnB,OAAO,EAAE,KAAK,GACjB;MA9KjB,AAgLY,QAhLJ,CAoFJ,EAAE,CA6EE,EAAE,GAeI,CAAC,CAAC;QACA,OAAO,EAAE,KAAK,GACjB;MAlLb,AAoLY,QApLJ,CAoFJ,EAAE,CA6EE,EAAE,CAmBE,GAAG,CAAC;QACA,OAAO,EAAE,IAAI;QACb,KAAK,EAAE,KAAK;QACZ,SAAS,EAAE,IAAI;QACf,SAAS,EAAE,IAAI;QACf,MAAM,EAAE,SAAS;QACjB,UAAU,EAAE,KAAK,GACpB;MA3Lb,AA8LgB,QA9LR,CAoFJ,EAAE,CA6EE,EAAE,AA4BG,QAAQ,GACH,CAAC,CAAC,UAAU,CAAC;QACX,KAAK,EftMP,OAAO;QeuML,OAAO,EAAE,MAAM,GAClB;MAjMjB,AAoMY,QApMJ,CAoFJ,EAAE,CA6EE,EAAE,CAmCE,EAAE,CAAC;QACC,YAAY,EAAE,IAAI;QAClB,WAAW,EAAE,MAAM,GACtB;;AChNb,AAAA,KAAK,CAAC;EACL,UAAU,EhBiCI,OAAO;EgBhCrB,MAAM,EAAE,CAAC,CAAC,CAAC,CAAC,QAAkC,CAAC,CAAC,GAChD;;AAED,AAAA,KAAK,CAAC;EAiBF,QAAQ,EAAE,QAAQ;EAClB,WAAW,ENrBC,KAAK;EMsBjB,UAAU,EAAE,IAAI,GA+EnB;EAlGD,AACI,KADC,CACD,GAAG,EADP,KAAK,CACI,gBAAgB,CAAC;IAClB,MAAM,EAAE,SAAS;IACjB,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAUrB;IAdL,AAMQ,KANH,CACD,GAAG,AAKE,OAAO,EANhB,KAAK,CACI,gBAAgB,AAKhB,OAAO,CAAC;MACL,MAAM,EAAE,4BAA4B;MACpC,OAAO,EAAE,GAAG,GACf;IATT,AAWQ,KAXH,CACD,GAAG,AAUE,OAAO,EAXhB,KAAK,CACI,gBAAgB,AAUhB,OAAO,CAAC;MACL,UAAU,EAAE,CAAC,CAAC,IAAI,CAAC,IAAI,CAAC,kBAAkB,GAC7C;EAbT,AAqBI,KArBC,CAqBD,SAAS,CAAC;IACN,MAAM,EAAE,cAAc,GACzB;EAvBL,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;IAEL,OAAO,EAAE,IAAI,CAAC,IAAqB,GAUtC;IVlBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MUnB1C,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;QAKD,QAAQ,EAAE,MAAM;QAChB,OAAO,EAAE,IAAI,CAAC,IAAqB,GAM1C;IVrBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MUhB1C,AAyBI,KAzBC,CAyBD,QAAQ,CAAC;QAUD,OAAO,EAAE,QAAQ,GAExB;EArCL,AAuCI,KAvCC,CAuCD,EAAE,GAAG,EAAE,CAAC;IACJ,UAAU,EAAE,OAAO;IACnB,aAAa,EAAE,IAAI,GACtB;EVvBH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IUnB1C,AA4CI,KA5CC,CA4CD,WAAW,CAAC;MAGJ,QAAQ,EAAE,MAAM;MAChB,YAAY,EAAE,YAAY;MAC1B,KAAK,EAAE,IAAI;MACX,OAAO,EAAE,KAAK,GAErB;EApDL,AAsDI,KAtDC,CAsDD,IAAI,CAAC;IAED,QAAQ,EAAE,KAAK;IACf,GAAG,EAAE,CAAC;IACN,MAAM,EAAE,CAAC;IACT,KAAK,EN9CC,IAAI;IM+CV,SAAS,EAAE,IAAI;IACf,MAAM,EAAE,IAAI;IACZ,MAAM,EAAE,OAAO;IACf,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,MAAM,GAiCrB;IAjGL,AAiEQ,KAjEH,CAsDD,IAAI,GAWE,CAAC,CAAC;MACA,OAAO,EAAE,UAAU;MACnB,cAAc,EAAE,MAAM;MACtB,UAAU,EAAE,MAAM,GACrB;IVlDP,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;MUnB1C,AAsDI,KAtDC,CAsDD,IAAI,CAAC;QAkBG,OAAO,EAAE,UAAU;QACnB,QAAQ,EAAE,MAAM;QAChB,GAAG,EAAE,IAAI;QACT,KAAK,EAAE,GAAG;QACV,UAAU,EAAE,MAAM;QAClB,MAAM,EAAE,KAAK;QACb,WAAW,EAAE,KAAK;QAClB,WAAW,EAAE,CAAC,GAkBrB;QAjGL,AAiEQ,KAjEH,CAsDD,IAAI,GAWE,CAAC,CAeK;UACA,OAAO,EAAE,YAAY,GACxB;IAlFb,AAsFQ,KAtFH,CAsDD,IAAI,AAgCC,MAAM,CAAC;MACJ,UAAU,ENpFV,OAAO,GMqFV;IAxFT,AA0FQ,KA1FH,CAsDD,IAAI,AAoCC,SAAS,CAAC;MACP,IAAI,EAAE,CAAC,GACV;IA5FT,AA8FQ,KA9FH,CAsDD,IAAI,AAwCC,SAAS,CAAC;MACP,KAAK,EAAE,CAAC,GACX;;AAIT,AAAA,WAAW,CAAC;EACR,aAAa,EAAE,IAAI,GACtB;;AAGD,AAAA,QAAQ,CAAC;EAEL,OAAO,EAAE,IAAI;EACb,WAAW,EAAE,MAAM;EACnB,eAAe,EAAE,MAAM;EACvB,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,MAAM,GAsBlB;EA5BD,AAQI,QARI,CAQJ,WAAW,CAAC;IACR,cAAc,EAAE,IAAI;IACpB,SAAS,EAAE,GAAG,GACjB;EAXL,AAaI,QAbI,CAaJ,EAAE,CAAC;IACC,WAAW,EJzHa,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;II0HpF,WAAW,EN7GG,GAAG;IM8GjB,UAAU,EAAE,MAAM,GACrB;EAjBL,AAmBI,QAnBI,CAmBJ,EAAE,CAAC;IACC,SAAS,EAAE,IAAI;IACf,aAAa,EAAE,GAAG,CAAC,KAAK,ChBnFd,OAAO,GgBoFpB;EAtBL,AAwBI,QAxBI,CAwBJ,CAAC,CAAC;IACE,UAAU,EAAE,MAAM;IAClB,SAAS,EAAE,MAAM,GACpB;;AAGL,AAAA,OAAO,CAAC;EACJ,OAAO,EAAE,SAAS;EAClB,KAAK,EAAE,OAA0B;EACjC,SAAS,EAAE,IAAI,GAKlB;EARD,AAKI,OALG,CAKH,CAAC,CAAC;IACE,MAAM,EAAE,CAAC,GACZ;;ALnJL,AAAA,IAAI,CMCC;EACJ,WAAW,ELCoB,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EKAxF,cAAc,EAAE,QAAQ;EAC3B,WAAW,EAAE,GAAG,GAChB;;AAGD,AAAA,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,EAAE,CAAC;EACtB,WAAW,ELLoB,YAAY,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;EKMjG,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,kBAAkB;EAClC,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,UAAU,EAAE,MAAM;EAClB,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI,GACpB;;AAED,AAAA,EAAE,CAAC;EACF,cAAc,EAAE,IAAI,GACpB;;AAGD,AAAA,UAAU,CAAC;EACV,WAAW,EAAE,IAAI,CAAC,KAAK,CjBiBN,OAAO,GiBNxB;EAZD,AAEC,UAFS,CAET,CAAC,CAAC;IACD,SAAS,EAAE,MAAM;IACjB,KAAK,EAAE,IAAI,GACX;EALF,AAMC,UANS,CAMT,IAAI,CAAC;IACJ,OAAO,EAAE,KAAK;IACd,UAAU,EAAE,KAAK;IACjB,KAAK,EAAE,IAAI;IACX,SAAS,EAAE,MAAM,GACjB;;AAXF,AAAA,UAAU,CAeC;EACP,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,UAAU,CAAC,UAAU,CAAC;EAClB,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,UAAU,GAAG,UAAU,GAAG,UAAU,CAAC;EAEpC,MAAM,EAAE,CAAC,GAmET;EArED,AAIC,UAJS,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,CAAC;IACD,OAAO,EAAE,IAAI;IACb,OAAO,EAAE,KAAK;IACd,SAAS,EAAE,IAAI;IACf,UAAU,EAAE,IAAI;IAChB,aAAa,EAAE,IAAI;IACb,KAAK,EAAE,IAAI,GAoBjB;IA9BF,AAaY,UAbF,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,AAQO,YAAY,AACR,OAAO,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EjBjEP,IAAI;MiBkEF,WAAW,EAAE,WAAW;MACxB,OAAO,EAAE,IAAI;MACb,IAAI,EAAE,IAAI,GACb;IApBb,AAqBY,UArBF,GAAG,UAAU,GAAG,UAAU,CAInC,CAAC,AAQO,YAAY,AASR,MAAM,CAAC;MACJ,QAAQ,EAAE,QAAQ;MAClB,GAAG,EAAE,GAAG;MACR,KAAK,EjBzEP,IAAI;MiB0EF,IAAI,EAAE,IAAI;MACV,WAAW,EAAE,IAAI;MACjB,OAAO,EAAE,MAAM,GAClB;EA5Bb,AAgCC,UAhCS,GAAG,UAAU,GAAG,UAAU,GAgCjC,CAAC,CAAC;IAEH,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GACnB;EArCF,AAuCC,UAvCS,GAAG,UAAU,GAAG,UAAU,GAuCjC,UAAU,GAAG,CAAC,CAAC;IAEhB,WAAW,EAAE,KAAK;IAClB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAInB;IA/CF,AA4CQ,UA5CE,GAAG,UAAU,GAAG,UAAU,GAuCjC,UAAU,GAAG,CAAC,AAKR,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,SAAS,GACrB;EA9CT,AAiDC,UAjDS,GAAG,UAAU,GAAG,UAAU,GAiDjC,UAAU,GAAG,UAAU,GAAG,CAAC,CAAC;IAE7B,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,kBAAkB;IAC9B,UAAU,EAAE,OAAO,GAInB;IAzDF,AAsDQ,UAtDE,GAAG,UAAU,GAAG,UAAU,GAiDjC,UAAU,GAAG,UAAU,GAAG,CAAC,AAKrB,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,MAAM,GAClB;EAxDT,AA2DC,UA3DS,GAAG,UAAU,GAAG,UAAU,GA2DjC,UAAU,GAAG,UAAU,GAAG,UAAU,GAAG,CAAC,CAAC;IAE1C,WAAW,EAAE,MAAM;IACnB,UAAU,EAAE,IAAI,CAAC,KAAK,CAAM,sBAAO;IACnC,UAAU,EAAE,OAAO,GAInB;IAnEF,AAgEQ,UAhEE,GAAG,UAAU,GAAG,UAAU,GA2DjC,UAAU,GAAG,UAAU,GAAG,UAAU,GAAG,CAAC,AAKlC,YAAY,AAAA,MAAM,CAAC;MAChB,OAAO,EAAE,KAAK,GACjB;;AAMT,AAAA,IAAI;AACJ,GAAG;AACH,GAAG;AACH,IAAI,CAAC;EACJ,WAAW,EL5HoB,aAAa,EAAE,SAAS,GK6HvD;;AAED,AAAA,IAAI,CAAC;EACJ,UAAU,EjBnFI,OAAO;EiBoFrB,KAAK,EAAE,OAAsB;EAC7B,OAAO,EAAE,WAAW;EACnB,aAAa,EAAE,GAAG,GACnB;;ANlHD,AAAA,GAAG,CMoHC;EACH,OAAO,EAAE,IAAI;EACb,MAAM,EAAE,MAAM;EACd,UAAU,EjB1FG,OAAO;EiB2FpB,MAAM,EAAE,GAAG,CAAC,KAAK,CjBzID,IAAI;EiB0IpB,aAAa,EAAE,GAAG;EAClB,WAAW,EAAE,IAAI;EACjB,SAAS,EAAE,IAAI,GAOf;EAdD,AASC,GATE,CASF,IAAI,CAAC;IACJ,KAAK,EjBlGS,OAAO;IiBmGrB,UAAU,EAAE,OAAO;IACnB,SAAS,EAAE,IAAI,GACf;;AAIF,AAAA,EAAE,CAAC;EACF,aAAa,EAAE,GAAG,CAAC,KAAK,CjB7GP,OAAO,GiB8GxB;;AAGD,AAAA,WAAW,CAAC;EACX,UAAU,EAAE,KAAK;EACjB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,KAAK,EAAE,IAAI;EACX,UAAU,EjBpKO,OAAO;EiBqKxB,KAAK,EjBnKQ,IAAI,GiBoKjB;;AAGD,AACI,KADC,CACD,CAAC,AAAA,YAAY,CAAC;EAAE,KAAK,EAAE,IAAI,GAAI;;AADnC,AAEI,KAFC,CAED,CAAC,AAAA,YAAY,AAAA,MAAM,CAAC;EAAE,KAAK,EjB3Kb,OAAO,GiB2KwB;;AAIjD,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EAAE,gBAAgB,EjB7K7D,wBAAI,GiB6KgF;;AAClG,AAAA,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,OAAoB,GAAI;;AAC3F,AAAA,gBAAgB,GAAG,eAAe,AAAA,MAAM,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,IAAI,GAAI;;AACjF,AAAA,gBAAgB,GAAG,eAAe,AAAA,iBAAiB,CAAC,WAAW,CAAC;EAAE,gBAAgB,EAAE,IAAI,GAAI;;ACpL5F,AAAA,KAAK,CAAC;EACL,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B;EAC7C,YAAY,EAAE,IAAI,GACrB;;AAED,AAAA,EAAE,CAAC;EAEF,UAAU,EAAE,OAA+B;EAC3C,OAAO,EAAE,MAAM,GACf;;AAED,AAAA,EAAE,CAAC;EACF,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,GAAG,CAAC,KAAK,CAAC,OAA8B,GAChD;;ACdD,AAAA,OAAO,CAAC;EVSP,UAAU,ETPO,OAAO;ESQxB,KAAK,ETNQ,IAAI;ESOjB,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAkB,GURtC;EAHD,AVYC,OUZM,AVYL,MAAM,CAAC;IACP,UAAU,EAAE,OAAkB;IAC9B,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAmB;IACvC,KAAK,ETXO,IAAI,GSYhB;EUhBF,AViBC,OUjBM,AViBL,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAmB,GACvC;;AUdF,AAAA,iBAAiB,CAAC;EVIjB,UAAU,ETNS,OAAO;ESO1B,KAAK,ETNQ,IAAI;ESOjB,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAkB,GUHtC;EAHD,AVOC,iBUPgB,AVOf,MAAM,CAAC;IACP,UAAU,EAAE,OAAkB;IAC9B,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAmB;IACvC,KAAK,ETXO,IAAI,GSYhB;EUXF,AVYC,iBUZgB,AVYf,OAAO,CAAC;IACR,UAAU,EAAE,CAAC,CAAC,GAAG,CAAC,CAAC,CAAC,OAAmB,GACvC;;AWnBF,AAAA,QAAQ,CAAC;EACR,MAAM,EvBYa,MAAwB,CuBZnB,CAAC;EACzB,WAAW,EAAE,QAAoB;EACjC,YAAY,EAAE,QAAoB;EAClC,QAAQ,EAAE,IAAI,GACd;;AAED,AAAA,OAAO,CAAC;EACP,KAAK,EAAE,IAAI;EACX,OAAO,EAAE,CAAC,CAAC,OAAmB,GAC9B;;AAED,AAAA,kBAAkB,CAAC;EbUlB,KAAK,EAAE,GAAsB,GaL7B;EdIC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IcT1C,AAAA,kBAAkB,CAAC;MbUlB,KAAK,EAAE,IAAsB,GaL7B;;AAED,AAAA,oBAAoB,CAAC;EbGpB,KAAK,EAAE,SAAsB,GaE7B;EdHC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IcF1C,AAAA,oBAAoB,CAAC;MbGpB,KAAK,EAAE,IAAsB,GaE7B;;AAED,AAAA,mBAAmB,CAAC;EbJnB,KAAK,EAAE,GAAsB,GaS7B;EdVC,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;IcK1C,AAAA,mBAAmB,CAAC;MbJnB,KAAK,EAAE,IAAsB,GaS7B;;AAED,AAAA,YAAY,CAAC;EACZ,KAAK,EAAE,IAAI;EACX,UAAU,EpBjCO,OAAO;EoBkCxB,OAAO,EAAE,QAAqB;EAC9B,KAAK,EnBrCgB,MAAM;EmBsC3B,MAAM,EnBtCe,MAAM;EmBuC3B,aAAa,EAAE,GAAG;EAClB,KAAK,EpBpCQ,IAAI;EoBqCjB,SAAS,EAAE,OAAqB;EAChC,UAAU,EAAE,MAAM,GAClB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EpB5CO,OAAO,GoB6CxB;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EnB/Ca,OAA6B,GmBgDpD;;AAED,AAAA,cAAc,CAAC;EACd,UAAU,EnBlDa,OAA8B,GmBmDrD;;AAED,AAAA,eAAe,CAAC;EACf,WAAW,EAAE,OAAuB,GACpC;;ACvDD,AAAA,WAAW,CAAC;EACV,QAAQ,EAAE,QAAQ,GACnB;;AAGD,AAAA,WAAW,AAAA,MAAM,CAAC;EAChB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,OAAO;EAChB,IAAI,EAAE,MAAM,CAAC,MAAM,CAAC,IAAI,CAAC,GAAG,CTXE,MAAM,EAAE,WAAW,EAAE,QAAQ,EAAE,QAAQ,EAAE,OAAO,EAAE,UAAU;ESY1F,KAAK,EAbc,IAAI;EAcvB,UAAU,EAAE,MAAM;EAClB,eAAe,EAAE,IAAI;EACrB,WAAW,EAAE,IAAI;EACjB,cAAc,EAAE,IAAI;EACpB,cAAc,EAAE,MAAM;EACtB,SAAS,EAAE,UAAU;EACrB,WAAW,EAAE,GAAG;EAChB,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,gBAAgB;EACzB,UAAU,EAxBe,kBAAkB;EAyB3C,aAAa,EAAE,GAAG;EAClB,sBAAsB,EAAE,oBAAoB,GAC7C;;AAGD,AAAA,WAAW,AAAA,OAAO,CAAC;EACjB,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,OAAO;EAChB,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,CAAC;EACR,MAAM,EAAE,CAAC;EACT,KAAK,EApCoB,kBAAkB;EAqC3C,cAAc,EAAE,IAAI;EACpB,OAAO,EAAE,EAAE;EACX,MAAM,EAAE,qBAAqB,GAC9B;;AAGD,AAGE,WAHS,AAAA,MAAM,AAGd,OAAO,EAHV,WAAW,AAAA,MAAM,AAId,MAAM;AAHT,WAAW,AAAA,OAAO,AAEf,OAAO;AAFV,WAAW,AAAA,OAAO,AAGf,MAAM;AAFT,WAAW,AAAA,MAAM,AACd,OAAO;AADV,WAAW,AAAA,MAAM,AAEd,MAAM,CAAC;EACN,OAAO,EAAE,YAAY;EACrB,eAAe,EAAE,IAAI,GACtB;;AAIH,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,UAAU,EAAE,GAAG,GAChB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,mBAAmB,EApEI,kBAAkB,GAqE1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAGE,aAHW,AAGV,MAAM;AAFT,cAAc,AAEX,MAAM;AADT,cAAc,AACX,MAAM,CAAC;EACN,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,aAAa,EAAE,GAAG,GACnB;;AAPH,AASE,aATW,AASV,OAAO;AARV,cAAc,AAQX,OAAO;AAPV,cAAc,AAOX,OAAO,CAAC;EACP,GAAG,EAAE,IAAI;EACT,KAAK,EAAE,GAAG;EACV,MAAM,EAAE,IAAI;EACZ,YAAY,EAAE,IAAI;EAClB,gBAAgB,EAnGO,kBAAkB,GAoG1C;;AAGH,AACE,cADY,AACX,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,IAAI,EAAE,GAAG;EACT,WAAW,EAAE,KAAK,GACnB;;AAGH,AAAA,cAAc,AAAA,MAAM,CAAC;EACnB,YAAY,EAAE,KAAK,GACpB;;AAGD,AAAA,aAAa,AAAA,MAAM;AACnB,aAAa,AAAA,MAAM,CAAC;EAClB,SAAS,EAAE,eAAe,GAC3B;;AAGD,AACE,aADW,AACV,MAAM,CAAC;EACN,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,YAAY,EAAE,GAAG;EACjB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,UAAU,EAAE,IAAI;EAChB,iBAAiB,EAvIM,kBAAkB,GAwI1C;;AAIH,AACE,aADW,AACV,MAAM,CAAC;EACN,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,IAAI;EACV,WAAW,EAAE,GAAG;EAChB,SAAS,EAAE,eAAe,GAC3B;;AANH,AAQE,aARW,AAQV,OAAO,CAAC;EACP,GAAG,EAAE,GAAG;EACR,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,GAAG;EACX,UAAU,EAAE,IAAI;EAChB,kBAAkB,EAzJK,kBAAkB,GA0J1C;;AC3JH,oDAAoD;AAEpD,AAAA,cAAc,CAAC;EACX,OAAO,EAAE,WAAW,GACvB;;AAED,AAAA,eAAe,CAAC;EACZ,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,YAAY;EACrB,QAAQ,EAAE,QAAQ,GACrB;;AAED,AAAA,eAAe,GAAG,eAAe,CAAC;EAC9B,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,sBAAsB;EAClC,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI;EAChB,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AAED,AAAA,eAAe,GAAG,eAAe,AAAA,mBAAmB,CAAC;EACjD,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAED,AAAA,eAAe,CAAC;EACZ,OAAO,EAAE,IAAI,GAChB;;AACD,AAAA,eAAe,EAAE,eAAe,CAAC,GAAG,CAAC;EACjC,UAAU,EAAE,WAAW,GAC1B;;AAED,AAAA,eAAe,AAAA,SAAS,AAAA,uBAAuB;AAC/C,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC;EAC5C,OAAO,EAAE,KAAK,GACjB;;AAED,AAAA,eAAe,CAAC,WAAW;AAC3B,eAAe,CAAC,aAAa,CAAC;EAC1B,MAAM,EAAE,OAAO,GAClB;;AAKD,AAAA,gBAAgB,GAAG,eAAe,CAAC;EAC/B,QAAQ,EAAE,iBAAiB,GAC9B;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,CAAC;EAC1C,MAAM,EAAE,eAAe;EACvB,UAAU,EAAE,UAAU;EACtB,MAAM,EAAE,eAAe;EACvB,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,eAAe;EAC3B,SAAS,EAAE,eAAe;EAC1B,QAAQ,EAAE,iBAAiB;EAC3B,OAAO,EAAE,IAAI;EACb,OAAO,EAAE,GAAG;EACZ,QAAQ,EAAE,mBAAmB;EAC7B,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,eAAe,GACzB;;AACD,AAAA,gBAAgB,GAAG,eAAe,GAAG,QAAQ,AAAA,mBAAmB,CAAC;EAC7D,MAAM,EAAE,CAAC;EACT,KAAK,EAAE,CAAC,GACX;;AAKD,wDAAwD;AAExD,AAAA,gBAAgB,GAAG,eAAe;AAClC,gBAAgB,GAAG,eAAe,CAAC,GAAG,CACtC;EACI,MAAM,EAAE,IAAI;EACZ,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,CAAC;EACV,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,EAAE,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,GAAG,CAAC;EACnC,OAAO,EAAE,KAAK;EACd,MAAM,EAAE,IAAI;EACZ,IAAI,EAAE,CAAC;EACP,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,GAAG;EACX,MAAM,EAAE,GAAG;EACX,IAAI,EAAE,CAAC;EACP,KAAK,EAAE,IAAI,GACd;;AAED,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,CAAC;EACxC,MAAM,EAAE,IAAI;EACZ,KAAK,EAAE,GAAG;EACV,GAAG,EAAE,CAAC;EACN,KAAK,EAAE,GAAG,GACb;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB,CAAC;EACrD,QAAQ,EAAE,MAAM,GACnB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,qBAAqB,EAAE,GAAG;EAC1B,kBAAkB,EAAE,GAAG;EACvB,aAAa,EAAE,GAAG,GACrB;;AAED,AAAA,gBAAgB,GAAG,eAAe,CAAC,qBAAqB;AACxD,gBAAgB,GAAG,eAAe,CAAC,WAAW,CAAC;EAC3C,UAAU,EAAC,qDAAqD;EAChE,MAAM,EAAE,iBAAiB;EACzB,OAAO,EAAE,GAAG,GACf;;AAGD,yDAAyD;AAEzD,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AAC1G,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,qBAAqB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;AAGzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,IAAI,EAAE,KAAK,GAAI;;AACzG,AAAA,gBAAgB,GAAG,eAAe,AAAA,SAAS,AAAA,uBAAuB,CAAC,oBAAoB,CAAC;EAAE,GAAG,EAAE,KAAK,GAAI;;ACrIxG,AACC,gBADe,CACf,KAAK,CAAC;EACL,QAAQ,EAAE,OAAO,GAIjB;EANF,AAGE,gBAHc,CACf,KAAK,CAEJ,QAAQ,CAAC;IACR,QAAQ,EAAE,OAAO,GACjB;;AAKH,AACC,eADc,CACd,CAAC,CAAC;EACD,cAAc,EAAE,MAAM,GACtB;;AAIF,AAAA,aAAa,CAAC,GAAG,CAAC;EACd,MAAM,EAAE,YAAY,GACvB;;AAGD,AAIE,UAJQ,CAET,WAAW,CAEV,EAAE,CAAC;EACF,UAAU,EAAE,IAAI;EAChB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,QAAQ,EAAE,QAAQ,GAClB;;AATH,AAWE,UAXQ,CAET,WAAW,CASV,EAAE,CAAC;EACF,MAAM,EAAE,aAAa;EACrB,OAAO,EAAE,IAAI;EACb,QAAQ,EAAE,QAAQ,GAYlB;EA1BH,AAgBG,UAhBO,CAET,WAAW,CASV,EAAE,CAKD,EAAE,CAAC;IAAC,WAAW,EAAE,IAAI,GAAG;EAhB3B,AAkBG,UAlBO,CAET,WAAW,CASV,EAAE,CAOD,MAAM,EAlBT,UAAU,CAET,WAAW,CASV,EAAE,CVnBJ,KAAK,EUQL,UAAU,CAET,WAAW,CASV,EAAE,CLjCJ,EAAE,CKwCQ;IACN,eAAe,EAAE,SAAS,GAC1B;EApBJ,AAsBG,UAtBO,CAET,WAAW,CASV,EAAE,CAWD,EAAE,CAAC;IACF,WAAW,EAAE,KAAK,GAClB;;AAxBJ,AA4BE,UA5BQ,CAET,WAAW,CA0BV,EAAE,CAAA,AAAA,KAAC,EAAO,OAAO,AAAd,EAAgB;EAClB,SAAS,EAAE,IAAI;EACf,QAAQ,EAAE,QAAQ;EAClB,MAAM,EAAE,CAAC;EACT,OAAO,EAAE,QAAQ;EACjB,KAAK,EAAE,CAAC;EACR,OAAO,EAAE,IAAI;EACb,KAAK,EAAE,IAAI;EACX,UAAU,EbtCD,OAAO,GauChB;;AArCH,AAuCE,UAvCQ,CAET,WAAW,CAqCV,EAAE,CAAC,EAAE,CAAC;EACL,UAAU,EAAE,eAAe;EAC3B,KAAK,EAAE,eAAe,GACtB;;AA1CH,AA4CE,UA5CQ,CAET,WAAW,CA0CV,QAAQ,GAAG,EAAE,CAAC;EACb,UAAU,EAAE,OAAuB;EACnC,MAAM,EAAE,GAAG,CAAC,KAAK,CbhDR,OAAO;EaiDhB,KAAK,EbhDI,OAAO,GaqDhB;EApDH,AAgDG,UAhDO,CAET,WAAW,CA0CV,QAAQ,GAAG,EAAE,CAIZ,EAAE,CAAC;IACF,UAAU,EbjDJ,OAAO,GamDb;;AAnDJ,AAsDE,UAtDQ,CAET,WAAW,CAoDV,QAAQ,GAAG,EAAE,CAAE;EACd,UAAU,EAAE,OAAqB;EACjC,MAAM,EAAE,GAAG,CAAC,KAAK,CbxDV,OAAO;EayDd,KAAK,EbxDI,OAAO,Ga4DhB;EA7DH,AA0DG,UA1DO,CAET,WAAW,CAoDV,QAAQ,GAAG,EAAE,CAIZ,EAAE,CAAC;IACF,UAAU,EbzDA,OAAO,Ga0DjB;;AA5DJ,AA+DE,UA/DQ,CAET,WAAW,CA6DV,QAAQ,GAAG,EAAE,CAAC;EACb,UAAU,EAAE,OAAyB;EACrC,MAAM,EAAE,GAAG,CAAC,KAAK,Cb/DN,OAAO;EagElB,KAAK,Eb/DM,OAAO,GamElB;EAtEH,AAmEG,UAnEO,CAET,WAAW,CA6DV,QAAQ,GAAG,EAAE,CAIZ,EAAE,CAAC;IACF,UAAU,Eb9DA,OAAO,Ga+DjB;;AArEJ,AAwEE,UAxEQ,CAET,WAAW,CAsEV,QAAQ,GAAG,EAAE,CAAA;EACZ,UAAU,EAAE,OAAyB;EACrC,MAAM,EAAE,GAAG,CAAC,KAAK,CbpEN,OAAO;EaqElB,KAAK,EbpEQ,OAAO,GawEpB;EA/EH,AA4EG,UA5EO,CAET,WAAW,CAsEV,QAAQ,GAAG,EAAE,CAIZ,EAAE,CAAC;IACF,UAAU,EbvEA,OAAO,GawEjB;;AAKJ,AAAA,QAAQ,CAAC;EACL,UAAU,EbvGF,OAAO;EawGf,aAAa,EAAE,GAAG;EAClB,MAAM,EAAE,eAAe;EACvB,OAAO,EAAE,MAAM;EACf,MAAM,EAAE,CAAC;EACT,UAAU,EAAE,IAAI,GACnB;;AAGD,AAAA,gBAAgB,CAAC;EAGb,QAAQ,EAAE,QAAQ;EAClB,OAAO,EAAE,CAAC;EAEV,KAAK,EAAE,KAAK;EACZ,OAAO,EAAE,KAAK,GACjB;;AAGD,AAAA,KAAK,CAAC,YAAY,CAAC;EAIf,MAAM,EAAE,IAAI;EACZ,OAAO,EAAE,KAAK;EACd,aAAa,EAAE,CAAC;EAChB,YAAY,EAAE,CAAC;EAEf,WAAW,EAAE,GAAG,GAKnB;EAdD,AAWI,KAXC,CAAC,YAAY,CAWd,IAAI,CAAC;IACD,OAAO,EAAE,QAAQ,GACpB;;AjBxHH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;ESf1C,AAAA,QAAQ,CQ4IK;IACL,KAAK,EbnJW,KAAK,GaoJxB;EPlJL,AAAA,KAAK,COmJK;IACF,WAAW,EbtJK,KAAK,GauJxB;;AjBrIH,MAAM,MAAM,GAAG,MAAM,SAAS,EAAE,QAAQ;ESZ1C,AAAA,QAAQ,CQoJK;IACL,KAAK,Eb3JW,KAAK;Ia4JrB,IAAI,Eb5JY,MAAK,Ga6JxB;EP3JL,AAAA,KAAK,CO4JK;IACF,WAAW,EAAE,CAAC;IACd,KAAK,EAAE,IAAI,GACd;EAED,AAAA,eAAe,CAAC;IACZ,QAAQ,EAAE,MAAM,GAoBnB;IArBD,AAGI,eAHW,CAGX,QAAQ,CAAC;MACL,IAAI,EAAE,CAAC,GACV;IALL,AAMI,eANW,CAMX,KAAK,CAAC;MACF,WAAW,Eb1KC,KAAK;Ma4KjB,QAAQ,EAAE,MAAM,GACnB;IAVL,AAWI,eAXW,CAWX,QAAQ,CAAC;MACL,QAAQ,EAAE,QAAQ;MAClB,IAAI,EAAC,CAAC;MACN,KAAK,EAAE,CAAC;MACR,GAAG,EAAE,CAAC;MACN,MAAM,EAAE,CAAC;MACT,OAAO,EAAC,EAAE;MACV,UAAU,EAAE,wBAAoB;MAChC,MAAM,EAAE,OAAO,GAClB;;AAKT,AAAA,kBAAkB,CAAC;EACjB,gBAAgB,EAAE,yBAAyB;EAC3C,mBAAmB,EAAE,OAAO;EAC5B,eAAe,EAAE,SAAS;EAC1B,iBAAiB,EAAE,SAAS;EAC5B,KAAK,EAAE,IAAI;EACX,MAAM,EAAE,OAA6B;EACtC,GAAG,EAAE,IAAI;EACR,OAAO,EAAE,YAAY;EACrB,cAAc,EAAE,MAAM;EACtB,QAAQ,EAAE,QAAQ;EAClB,KAAK,EAAE,OAAsB;EAC7B,gBAAgB,EvBzJH,OAAO;EuB0JpB,WAAW,EAAE,MAAM;EACnB,MAAM,EAAE,OAAO;EACf,aAAa,EAAE,WAAW,GAkB3B;EAjCD,AAiBE,kBAjBgB,AAiBf,MAAM,CAAC;IACN,gBAAgB,EAAE,OAAoB,GACvC;EAED,AAAA,GAAG,CArBL,kBAAkB,CAqBV;IACJ,QAAQ,EAAE,QAAQ;IAClB,KAAK,EAAE,GAAG;IACV,GAAG,EAAE,GAAG;IACR,gBAAgB,EAAE,IAAI;IACtB,YAAY,EAAE,IAAI;IAClB,aAAa,EAAE,GAAG,GAKnB;IAXD,AAQE,GARC,CArBL,kBAAkB,AA6Bb,MAAM,CAAC;MACN,gBAAgB,EAAE,OAAO,GAC1B;;AAKL,AAAA,eAAe,CAAC;EACd,uBAAuB,EAAE,WAAW;EACpC,oBAAoB,EAAE,WAAW;EACjC,eAAe,EAAE,WAAW,GAC7B"
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/editor-buttons/admin/js/shortcode-presentation.js b/themes/learn2-git-sync/editor-buttons/admin/js/shortcode-presentation.js
deleted file mode 100644
index 611def0..0000000
--- a/themes/learn2-git-sync/editor-buttons/admin/js/shortcode-presentation.js
+++ /dev/null
@@ -1,22 +0,0 @@
-(function($){
- $(function(){
- $('body').on('grav-editor-ready', function() {
- var Instance = Grav.default.Forms.Fields.EditorField.Instance;
- Instance.addButton({
- 'shortcodes-presentation': {
- identifier: 'shortcodes-presentation',
- title: 'Presentation',
- label: ' ',
- modes: ['gfm', 'markdown'],
- action: function(_ref) {
- var codemirror = _ref.codemirror, button = _ref.button;
- button.on('click.editor.shortcodes-presentation', function() {
- Instance.buttonStrategies.replaceSelections({ token: '$1', template: '[presentation="presentations/"]$1', codemirror: codemirror});
- codemirror.setCursor(codemirror.getCursor().line,codemirror.getCursor().ch-2);
- });
- }
- }
- });
- });
- });
-})(jQuery);
diff --git a/themes/learn2-git-sync/images/clippy.svg b/themes/learn2-git-sync/images/clippy.svg
deleted file mode 100644
index e1b1703..0000000
--- a/themes/learn2-git-sync/images/clippy.svg
+++ /dev/null
@@ -1,3 +0,0 @@
-
-
-
diff --git a/themes/learn2-git-sync/images/favicon.png b/themes/learn2-git-sync/images/favicon.png
deleted file mode 100644
index ec645f1..0000000
Binary files a/themes/learn2-git-sync/images/favicon.png and /dev/null differ
diff --git a/themes/learn2-git-sync/images/logo.png b/themes/learn2-git-sync/images/logo.png
deleted file mode 100644
index 287a4e7..0000000
Binary files a/themes/learn2-git-sync/images/logo.png and /dev/null differ
diff --git a/themes/learn2-git-sync/languages.yaml b/themes/learn2-git-sync/languages.yaml
deleted file mode 100644
index 58d0c31..0000000
--- a/themes/learn2-git-sync/languages.yaml
+++ /dev/null
@@ -1,20 +0,0 @@
-en:
- THEME_LEARN2_GIT_EDIT_THIS_PAGE: Edit this Page
- THEME_LEARN2_GIT_NOTE: Notice an error? Think you can improve this documentation?
- THEME_LEARN2_CLEAR_HISTORY: Clear History
- THEME_LEARN2_BUILT_WITH_GRAV: Built with Grav - The Modern Flat File CMS
- THEME_LEARN2_SEARCH_DOCUMENTATION: Search Documentation
- THEME_LEARN2_GIT_ADD_SETUP_GIT_SYNC_PLUGIN: Add/Setup Git Sync Plugin
- THEME_LEARN2_GIT_SETUP_GIT_SYNC_PLUGIN: Setup Git Sync
- THEME_LEARN2_ADVANCED_SEARCH: Advanced Full-text Search
- THEME_LEARN2_ADVANCED_SEARCH_PLACEHOLDER: Search the documentation...
-fr:
- THEME_LEARN2_GIT_EDIT_THIS_PAGE: Modifier cette page
- THEME_LEARN2_GIT_NOTE: Vous avez découvert des erreurs ? Vous pensez pouvoir améliorer cette documentation ?
- THEME_LEARN2_CLEAR_HISTORY: Effacer l'historique
- THEME_LEARN2_BUILT_WITH_GRAV: Créé avec Grav - Le CMS moderne sans base de données
- THEME_LEARN2_SEARCH_DOCUMENTATION: Rechercher dans la documentation
- THEME_LEARN2_GIT_ADD_SETUP_GIT_SYNC_PLUGIN: Configuration Git
- THEME_LEARN2_GIT_SETUP_GIT_SYNC_PLUGIN: Configuration Git
- THEME_LEARN2_ADVANCED_SEARCH: Recherche avancée en texte intégral
- THEME_LEARN2_ADVANCED_SEARCH_PLACEHOLDER: Rechercher la documentation ...
diff --git a/themes/learn2-git-sync/learn2-git-sync.php b/themes/learn2-git-sync/learn2-git-sync.php
deleted file mode 100644
index 9deea7f..0000000
--- a/themes/learn2-git-sync/learn2-git-sync.php
+++ /dev/null
@@ -1,139 +0,0 @@
- ['onTwigInitialized', 0],
- 'onThemeInitialized' => ['onThemeInitialized', 0],
- 'onShortcodeHandlers' => ['onShortcodeHandlers', 0],
- 'onTwigSiteVariables' => ['onTwigSiteVariables', 0],
- 'onTNTSearchIndex' => ['onTNTSearchIndex', 0],
- 'registerNextGenEditorPlugin' => ['registerNextGenEditorPluginShortcodes', 0]
- ];
- }
-
- public function onShortcodeHandlers()
- {
- $this->grav['shortcode']->registerAllShortcodes('user://themes/learn2-git-sync/shortcodes');
- }
-
- public function registerNextGenEditorPluginShortcodes($event) {
- $plugins = $event['plugins'];
-
- $plugins['js'][] = 'user://themes/learn2-git-sync/nextgen-editor/shortcodes/googleslides.js';
- $plugins['js'][] = 'user://themes/learn2-git-sync/nextgen-editor/shortcodes/h5p.js';
- $plugins['js'][] = 'user://themes/learn2-git-sync/nextgen-editor/shortcodes/pdf.js';
-
- $event['plugins'] = $plugins;
- return $event;
- }
-
- public function onTwigSiteVariables()
- {
- if ($this->isAdmin() && ($this->grav['config']->get('plugins.shortcode-core.enabled'))) {
- $this->grav['assets']->add('theme://editor-buttons/admin/js/shortcode-presentation.js');
- }
- }
-
- public function onTNTSearchIndex(Event $e)
- {
- $fields = $e['fields'];
- $page = $e['page'];
- $taxonomy = $page->taxonomy();
-
- if (isset($taxonomy['tag'])) {
- $fields->tag = implode(",", $taxonomy['tag']);
- }
- }
-
- public function onTwigInitialized() {
- $sc = $this->grav['shortcode'];
- $sc->getHandlers()->addAlias('version', 'lang');
- }
-
- /**
- * Register events and route with Grav
- *
- * @return void
- */
- public function onThemeInitialized()
- {
- /* Check if Admin-interface */
- if (!$this->isAdmin()) {
- $this->enable(
- [
- 'onPageInitialized' => ['onPageInitialized', 0]
- ]
- );
- }
- }
-
- /**
- * Get default category setting
- *
- * @return string
- */
- public static function getdefaulttaxonomycategory()
- {
- $config = Grav::instance()['config'];
- return $config->get('themes.' . $config->get('system.pages.theme'). '.default_taxonomy_category');
- }
-
- /**
- * Handle CSS
- *
- * @return void
- */
- public function onPageInitialized()
- {
- $assets = $this->grav['assets'];
- $config = $this->config();
- if (isset($config['style'])) {
- $style = $config['style'];
- if ($style == 'default') {
- $style = 'theme';
- }
- $current = self::fileFinder(
- $style,
- '.css',
- 'theme://css/styles',
- 'theme://css'
- );
- $assets->addCss($current, 101);
- }
- }
-
- /**
- * Search for a file in multiple locations
- *
- * @param string $file Filename.
- * @param string $ext File extension.
- * @param array ...$locations List of paths.
- *
- * @return string
- */
- public static function fileFinder($file, $ext, ...$locations)
- {
- $return = false;
- foreach ($locations as $location) {
- if (file_exists($location . '/' . $file . $ext)) {
- $return = $location . '/' . $file . $ext;
- break;
- }
- }
- return $return;
- }
-}
-?>
diff --git a/themes/learn2-git-sync/learn2-git-sync.yaml b/themes/learn2-git-sync/learn2-git-sync.yaml
deleted file mode 100644
index 4d98813..0000000
--- a/themes/learn2-git-sync/learn2-git-sync.yaml
+++ /dev/null
@@ -1,20 +0,0 @@
-enabled: true
-root_page: # optional: set root page of documentation
-top_level_version: false # Use versions for top level navigation
-google_analytics_code: # Enter your `UA-XXXXXXXX-X` code here
-home_url: # http://getgrav.org
-github:
- position: top # top | bottom | off
- icon: # FontAwesome icon
- tree:
-default_taxonomy_category: docs
-style: default
-
-streams:
- schemes:
- theme:
- type: ReadOnlyStream
- prefixes:
- '':
- - themes://learn2-git-sync
- - themes://learn2
diff --git a/themes/learn2-git-sync/nextgen-editor/shortcodes/googleslides.js b/themes/learn2-git-sync/nextgen-editor/shortcodes/googleslides.js
deleted file mode 100644
index a94b50d..0000000
--- a/themes/learn2-git-sync/nextgen-editor/shortcodes/googleslides.js
+++ /dev/null
@@ -1,37 +0,0 @@
-window.nextgenEditor.addHook('hookInit', () => {
- window.nextgenEditor.addButtonGroup('learn2-with-git-sync', {
- label: 'Learn2 with Git Sync',
- });
-});
-
-window.nextgenEditor.addShortcode('googleslides', {
- type: 'block',
- plugin: 'learn2-with-git-sync',
- title: 'Google Slides',
- button: {
- label: 'Google Slides',
- group: 'learn2-with-git-sync',
- },
- attributes: {
- url: {
- type: String,
- innerHTML: true,
- title: 'URL',
- widget: 'input-text',
- default: '',
- },
- },
- titlebar({ attributes }) {
- return `URL: ${attributes.url || 'No URL provided '} `;
- },
- content({ attributes }) {
- return attributes.url
- ? `
`
- : 'No URL provided
';
- },
- preserve: {
- block: [
- 'iframe',
- ],
- },
-});
diff --git a/themes/learn2-git-sync/nextgen-editor/shortcodes/h5p.js b/themes/learn2-git-sync/nextgen-editor/shortcodes/h5p.js
deleted file mode 100644
index eb0e984..0000000
--- a/themes/learn2-git-sync/nextgen-editor/shortcodes/h5p.js
+++ /dev/null
@@ -1,39 +0,0 @@
-window.nextgenEditor.addHook('hookInit', () => {
- window.nextgenEditor.addButtonGroup('learn2-with-git-sync', {
- label: 'Learn2 with Git Sync',
- });
-});
-
-window.nextgenEditor.addShortcode('h5p', {
- type: 'block',
- plugin: 'learn2-with-git-sync',
- title: 'H5P',
- button: {
- label: 'H5P',
- group: 'learn2-with-git-sync',
- },
- attributes: {
- url: {
- type: String,
- innerHTML: true,
- title: 'URL',
- widget: 'input-text',
- default: '',
- },
- },
- titlebar({ attributes }) {
- return `URL: ${attributes.url || 'No URL provided '} `;
- },
- content({ attributes }) {
- return attributes.url
-
- ? `
`
- : 'No URL provided
';
- },
- preserve: {
- block: [
- 'iframe',
- 'script',
- ],
- },
-});
diff --git a/themes/learn2-git-sync/nextgen-editor/shortcodes/pdf.js b/themes/learn2-git-sync/nextgen-editor/shortcodes/pdf.js
deleted file mode 100644
index 8d8acb3..0000000
--- a/themes/learn2-git-sync/nextgen-editor/shortcodes/pdf.js
+++ /dev/null
@@ -1,38 +0,0 @@
-window.nextgenEditor.addHook('hookInit', () => {
- window.nextgenEditor.addButtonGroup('learn2-with-git-sync', {
- label: 'Learn2 with Git Sync',
- });
-});
-
-window.nextgenEditor.addShortcode('pdf', {
- type: 'block',
- plugin: 'learn2-with-git-sync',
- title: 'PDF',
- button: {
- label: 'PDF',
- group: 'learn2-with-git-sync',
- },
- attributes: {
- url: {
- type: String,
- innerHTML: true,
- title: 'URL',
- widget: 'input-text',
- default: '',
- },
- },
- titlebar({ attributes }) {
- return `URL: ${attributes.url || 'No URL provided '} `;
- },
- content({ attributes }) {
- return attributes.url
-
- ? `
`
- : 'No URL provided
';
- },
- preserve: {
- block: [
- 'iframe',
- ],
- },
-});
diff --git a/themes/learn2-git-sync/screenshot.jpg b/themes/learn2-git-sync/screenshot.jpg
deleted file mode 100644
index 00d7516..0000000
Binary files a/themes/learn2-git-sync/screenshot.jpg and /dev/null differ
diff --git a/themes/learn2-git-sync/scss/_affix.scss b/themes/learn2-git-sync/scss/_affix.scss
deleted file mode 100644
index dc2faf7..0000000
--- a/themes/learn2-git-sync/scss/_affix.scss
+++ /dev/null
@@ -1,37 +0,0 @@
-// Core
-@import "theme/core";
-@import "theme/fonts";
-
-// Forms
-@import "theme/forms";
-
-// Header
-@import "theme/header";
-
-// Nav
-@import "theme/nav";
-
-// Main
-@import "theme/main";
-
-// Typography
-@import "standard_colors";
-@import "theme/typography";
-
-// Tables
-@import "theme/tables";
-
-// Buttons
-@import "theme/buttons";
-
-// Bullets
-@import "theme/bullets";
-
-// Custom
-@import "theme/tooltips";
-
-// Scrollbar
-@import "theme/scrollbar";
-
-// Custom
-@import "theme/custom";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/_contrast.scss b/themes/learn2-git-sync/scss/_contrast.scss
deleted file mode 100644
index d2a36a9..0000000
--- a/themes/learn2-git-sync/scss/_contrast.scss
+++ /dev/null
@@ -1,417 +0,0 @@
-/*
- WCAG color contrast formula
- https://www.w3.org/TR/2016/NOTE-WCAG20-TECHS-20161007/G18#G18-procedure
-
- @see https://codepen.io/giana/project/full/ZWbGzD
-
- This pen uses the non-standard Sass pow() function
- https://css-tricks.com/snippets/sass/power-function/
- Using it outside of CodePen requires you provide your own pow() function with support for decimals
-
- To generate random colors, we're also using a two-variable random() function includded with compass.
-*/
-
-@function pow($number, $exponent) {
- @if (round($exponent) != $exponent) {
- @return exp($exponent * ln($number));
- }
-
- $value: 1;
-
- @if $exponent > 0 {
- @for $i from 1 through $exponent {
- $value: $value * $number;
- }
- } @else if $exponent < 0 {
- @for $i from 1 through -$exponent {
- $value: $value / $number;
- }
- }
-
- @return $value;
-}
-
-@function factorial($value) {
- $result: 1;
-
- @if $value == 0 {
- @return $result;
- }
-
- @for $index from 1 through $value {
- $result: $result * $index;
- }
-
- @return $result;
-}
-
-@function summation($iteratee, $input, $initial: 0, $limit: 100) {
- $sum: 0;
-
- @for $index from $initial to $limit {
- $sum: $sum + call($iteratee, $input, $index);
- }
-
- @return $sum;
-}
-
-@function exp-maclaurin($x, $n) {
- @return (pow($x, $n) / factorial($n));
-}
-
-@function exp($value) {
- @return summation(exp-maclaurin, $value, 0, 100);
-}
-
-@function ln-maclaurin($x, $n) {
- @return (pow(-1, $n + 1) / $n) * (pow($x - 1, $n));
-}
-
-@function ln($value) {
- $ten-exp: 1;
- $ln-ten: 2.30258509;
-
- @while ($value > pow(10, $ten-exp)) {
- $ten-exp: $ten-exp + 1;
- }
-
- @return summation(ln-maclaurin, $value / pow(10, $ten-exp), 1, 100) + $ten-exp * $ln-ten;
-}
-
-
-
-// Check if value is not a number, eg, NaN or Infinity
-@function is-nan($value) {
- @return $value / $value != 1;
-}
-
-// Constrain number between two values
-@function clip($value, $min : 0.0001, $max : 0.9999) {
- @return if($value > $max, $max, if($value < $min, $min, $value));
-}
-
-// Checks if value is within specified bounds, inclusive
-@function in-bounds($value, $min : 0, $max : 1) {
- @return if($value >= $min and $value <= $max, true, false);
-}
-
-//== Step one: Convert
-
-// Returns an RGB channel processed as XYZ... or partly at least
-// See w3.org link for formula
-@function xyz($channel) {
- $channel: $channel / 255;
-
- @return if($channel <= 0.03928, $channel / 12.92, pow((($channel + 0.055) / 1.055), 2.4));
-}
-
-// Reverse of xyz(). Returns XYZ value to RGB channel
-// https://en.wikipedia.org/wiki/SRGB
-@function srgb($channel) {
- @return 255 * if($channel <= 0.0031308, $channel * 12.92, 1.055 * pow($channel, 1/2.4) - 0.055);
-}
-
-//== Step two: Measure brightness
-
-// Returns relative luminance of color
-// See w3.org link for formula
-@function luminance($color) {
- $red: xyz(red($color));
- $green: xyz(green($color));
- $blue: xyz(blue($color));
-
- @return $red * 0.2126 + $green * 0.7152 + $blue * 0.0722;
-}
-
-//== Step three: Check contrast
-
-// Checks if two colors pass minimum contrast requirements, option to return ratio instead of true/false
-// See w3.org link for formula
-@function check-contrast($color1, $color2 : #fff, $min-ratio : 'AA', $return-ratio : false) {
- // Accept keywords for ratio
- @if($min-ratio == 'AA' or $min-ratio == 'AAALG') { $min-ratio: 4.5; }
- @elseif($min-ratio == 'AALG') { $min-ratio: 3; }
- @elseif($min-ratio == 'AAA') { $min-ratio: 7; }
-
- // Check brightness of each color
- $lum1: luminance($color1);
- $lum2: luminance($color2);
-
- // Measure contrast ratio
- $ratio: (max($lum1, $lum2) + 0.05) / (min($lum1, $lum2) + 0.05);
-
- // Return ratio if option set
- @if($return-ratio) { @return $ratio; }
-
- // Else return boolean
- @return if($ratio >= $min-ratio, true, false);
-}
-
-//== Step four: Scale luminance and lightness
-
-// Takes color, scales luminance, spits out new color
-@function scale-luminance($color, $target-luminance) {
- // First, scale the channels by the required amount
- $scale: $target-luminance / luminance($color);
-
- // And clip them, so we don't end up dividing by zero... among other things I forget
- $red: clip(xyz(red($color))) * $scale;
- $green: clip(xyz(green($color))) * $scale;
- $blue: clip(xyz(blue($color))) * $scale;
-
- // Sometimes, that's not enough and one channel hits #ff or #00. We'll need to scale the other channels to compensate
- $red-passes: in-bounds($red);
- $green-passes: in-bounds($green);
- $blue-passes: in-bounds($blue);
-
- @if(not $red-passes or not $green-passes or not $blue-passes) {
- // First, pick a channel to be a baseline, so the rest can be expressed as ratios
- $baseline: min($red, $green, $blue);
-
- // Then set up the variables expressed in terms of the baseline
- $r: $red / $baseline;
- $g: $green / $baseline;
- $b: $blue / $baseline;
-
- // Subtract any channel no longer in bounds
- //-- TODO This needs to DRY. how to dry. help
- @if(not $red-passes) {
- $target-luminance: $target-luminance - 0.2126;
- $r: 0;
- }
-
- @if(not $green-passes) {
- $target-luminance: $target-luminance - 0.7152;
- $g: 0;
- }
-
- @if (not $blue-passes) {
- $target-luminance: $target-luminance - 0.0722;
- $b: 0;
- }
-
- // Now get the required difference by using the luminance() formula
- $x: $target-luminance / ($r * 0.2126 + $g * 0.7152 + $b * 0.0722);
-
- // And multiply the channels by this new per-channel luminance
- @if($red-passes) { $red: $r * $x; }
- @if($green-passes) { $green: $g * $x; }
- @if($blue-passes) { $blue: $b * $x; }
- }
-
- // Return the new color
- @return rgb(srgb($red), srgb($green), srgb($blue));
-}
-
-// Scales lightness by 0.1% while checking contrast ratio. This is just a last-ditch effort to correct rounding errors
-@function scale-light($color1, $color2, $min-ratio, $operation, $iterations) {
- // Loop this function for however many iterations are passed
- @for $n from 1 through $iterations {
- // Return color unchanged if it passes contrast check
- @if(check-contrast($color1, $color2, $min-ratio)) {
- @return $color1;
- } @else {
- // Otherwise use the built-in lighten() and darken() functions, which change the lightness channel (ie, the L in HSL)
- // Our previous scale-luminance() function changes both saturation and lightness
- $color1: if($operation == lighten, lighten($color1, 0.1%), darken($color1, 0.1%));
- }
- }
-
- // Return the best color we've got
- @return $color1;
-}
-
-//== Step six: Fix colors
-
-// Tries to fix contrast by adjusting $color1
-@function fix-color($color1, $color2 : #fff, $min-ratio : 'AA', $iterations : 5) {
- // Accept keywords for ratio
- @if($min-ratio == 'AA' or $min-ratio == 'AAALG') { $min-ratio: 4.5; }
- @elseif($min-ratio == 'AALG') { $min-ratio: 3; }
- @elseif($min-ratio == 'AAA') { $min-ratio: 7; }
-
- // If check fails, begin conversion
- @if(not check-contrast($color1, $color2, $min-ratio)) {
- // First get both luminances and clip so #fff and #000 don't break anything
- $lum1: clip(luminance($color1));
- $lum2: clip(luminance($color2));
-
- // Defaults we'll set later
- $target-luminance: $lum1;
- $operation: '';
-
- // If the same luminance is passed, lighten/darken one to make conversion possible
- @if($lum1 == $lum2) {
- // Darken light colors and lighten dark colors, so we have more room to scale them (eg, we won't hit #fff or #000 before we can fix them)
- @if($lum1 > 0.5) {
- $color1: darken($color1, 1%);
- $lum1: luminance($color1);
- } @else {
- $color1: lighten($color1, 1%);
- $lum1: luminance($color1);
- }
- }
-
- // Now let's get the target luminance. This basically reverses check-contrast(), so we know what luminance to aim for
- @if(max($lum1, $lum2) == $lum1) {
- $target-luminance: (($lum2 + 0.05) * $min-ratio - 0.05);
- $operation: lighten;
- } @else {
- $target-luminance: (($lum2 + 0.05) / $min-ratio - 0.05);
- $operation: darken;
- }
-
- // Skip the whole conversion if we just need #fff or #000
- @if($target-luminance >= 1) { @return #fff; }
- @elseif ($target-luminance <= 0) { @return #000; }
- @else {
- // Scale color by calculated difference to arrive at target luminance
- $color1: scale-luminance($color1, $target-luminance);
-
- // Try to fix any rounding errors by lightening or darkening
- $color1: scale-light($color1, $color2, $min-ratio, $operation, $iterations);
- }
-
- }
-
- // Tada
- @return $color1;
-}
-
-// Tries to fix contrast of both colors by weighted balance (0–100)
-// 0 = don't change first color, change second color;
-// 100 = change first color, don't change second color
-@function fix-contrast($color1, $color2, $min-ratio : 'AA', $balance : 50) {
- @if(not check-contrast($color1, $color2, $min-ratio)) {
- // Fix colors
- $color-fixed-1: fix-color($color1, $color2, $min-ratio);
- $color-fixed-2: fix-color($color2, $color1, $min-ratio);
-
- // We're just fixing both colors, then mixing back the original color using the native Sass function. Easy-peasy
- $color1: mix($color-fixed-1, $color1, $balance);
- $color2: mix($color2, $color-fixed-2, $balance);
-
- // If the current configuration doesn't work, try to fix it
- @if (not check-contrast($color1, $color2, $min-ratio)) {
- // This happens if, again, we reach #fff or #000 before we want to
- @if(not in-bounds(luminance($color-fixed-2), 0.00002, 0.99936)) {
- // So we scale the opposite color to compensate
- $color1: fix-color($color1, $color2, $min-ratio);
- @warn "Your settings didn't work. Modifying first color in an attempt to fix."
- }
- @if(not in-bounds(luminance($color-fixed-1), 0.00002, 0.99936)) {
- $color2: fix-color($color2, $color1, $min-ratio);
- @warn "Your settings didn't work. Modifying second color in an attempt to fix."
- }
- }
- }
-
- // Returns a list with both colors, use nth($result, 1) and nth($result, 2) to get colors. See below for example
- @return $color1, $color2;
-}
-
-// Get the best contrast when given three colors
-@function best-contrast($color, $color1, $color2, $ratio1 : 'AA', $ratio2 : $ratio1) {
- @if(not check-contrast($color, $color1, $ratio1) or not check-contrast($color, $color2, $ratio2)) {
- // First get the luminance of the two static colors
- $lum1: luminance(fix-color($color1, $color1, $ratio1));
- $lum2: luminance(fix-color($color2, $color2, $ratio2));
-
- // Average the luminance together to get the maximum difference
- $average-lum: ($lum1 + $lum2) / 2;
-
- // Then set changing color to this luminance
- $color: scale-luminance($color, $average-lum);
-
- // Warn if it fails contrast check
- @if(not check-contrast($color, $color1, $ratio1)) {
- @warn 'Your color fails to contrast with #{$color1}';
- }
-
- @if(not check-contrast($color, $color2, $ratio2)) {
- @warn 'Your color fails to contrast with #{$color2}';
- }
- }
-
- @return $color;
-}
-
-
-//====== Helper functions
-
-@function randomColor() {
- $color: hsl(random(360), random(100), random(100));
- @return $color;
-}
-
-@mixin show-color($color) {
- background: $color;
- color: if(luminance($color) > 0.55, #000, #fff);
-
- &::after {
- content: '#{$color}';
- }
-}
-
-//====== Put in your own settings here
-$ratio: random(21); // A number between 1 and 21
-$balance: random(100); // A number between 0 and 100
-
-// Any valid color
-$color1: randomColor();
-$color2: scale-luminance(randomColor(), luminance($color1) + 0.1);
-$color3: randomColor();
-
-.ratio::after { content: '#{$ratio}'; }
-.balance::after { content: '#{$balance}'; }
-
-.color-block .color1 { @include show-color($color1); }
-.color-block .color2 { @include show-color($color2); }
-
-.fix-color {
- .color:nth-child(2) { @include show-color(fix-color($color1, $color2, $ratio)); }
- .color:nth-child(3) { @include show-color(fix-color($color2, $color1, $ratio)); }
-}
-
-.fix-contrast {
- .color:nth-child(2) { @include show-color(nth(fix-contrast($color1, $color2, $ratio, $balance),1)); }
- .color:nth-child(3) { @include show-color(nth(fix-contrast($color1, $color2, $ratio, $balance),2)); }
-}
-
-.best-contrast {
- .color:nth-child(2) { @include show-color($color3); }
- .color:nth-child(3) { @include show-color(best-contrast($color3, $color1, $color2, $ratio, $ratio)); }
-}
-
-.scale-luminance {
- .color:nth-child(2) { @include show-color(scale-luminance($color1, luminance($color2))); }
-}
-
-.check-contrast {
- .result::after { content: '#{check-contrast($color1, $color2, $ratio)}' ;}
-}
-
-.luminance {
- .result::after { content: '#{luminance($color1), luminance($color2)}' ;}
-}
-
-
-// Amplify (strengthen) color by percentage
-// @see https://www.scrivito.com/blog/sass-magic
-@function amplify($color, $percentage) {
- @if (lightness( $color ) <= 50) {
- @return darken($color, $percentage);
- }
- @else {
- @return lighten($color, $percentage);
- }
-}
-// Diminish (weaken) color by percentage
-@function diminish($color, $percentage) {
- @if (lightness( $color ) >= 50) {
- @return darken($color, $percentage);
- }
- @else {
- @return lighten($color, $percentage);
- }
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/_hover-color.scss b/themes/learn2-git-sync/scss/_hover-color.scss
deleted file mode 100644
index 8f11dd2..0000000
--- a/themes/learn2-git-sync/scss/_hover-color.scss
+++ /dev/null
@@ -1,449 +0,0 @@
-/*
- The Ultimate Hover Color Function
- @author Gray Gilmore - http://code.graygilmore.com
-
- The goal of this Sass function is provide a hover color that works
- with any color on any background. No longer do we need to bundle
- hover color options with our themes, let Sassy McSasserson take care
- of that for you.
-
- The hover color, seen in this demo as "After" text, must be visible in
- all situations and, hopefully, pass the WCAG 2.0 contrast ratio [1]
- formula (4.5:1).
-
- [1] http://www.w3.org/TR/2008/REC-WCAG20-20081211/#visual-audio-contrast-contrast
-
- contrast-ratio() help from @davidkaneda
-
-
- ## Usage ##
-
- a {
- color: $link-color;
-
- &:hover {
- color: hover($background-color, $link-color);
- }
- }
-
- button {
- background: $button-background;
- color: $button-color;
-
- &:hover {
- background: hover($background-color, $button-background);
- }
- }
-
- ## End Usage ##
-
-*/
-
-@function color-luminance($value) {
- @if $value <= 0.03928 {
- @return $value / 12.92;
- } @else {
- @return ($value + 0.055)/1.055 * ($value + 0.055)/1.055;
- }
-}
-
-@function luminosity($color) {
- $r: color-luminance(red($color) / 255);
- $g: color-luminance(green($color) / 255);
- $b: color-luminance(blue($color) / 255);
-
- @return 0.2126 * $r + 0.7152 * $g + 0.0722 * $b;
-}
-
-@function contrast-ratio($c1, $c2) {
- $l1: luminosity($c1);
- $l2: luminosity($c2);
-
- @if $l2 > $l1 {
- @return $l2 / $l1;
- } @else {
- @return $l1 / $l2;
- }
-}
-
-@function contrast-color($background, $color) {
-
- $style: '';
- $hover-color: '';
- $color-lightness: abs(lightness($color));
- $background-lightness: abs(lightness($background));
- $contrast: abs(contrast-ratio($background, $color));
-
- @if $color-lightness == 100 {
- /* White */
- @if $background-lightness >= 90 {
- $hover-color: darken($background, 15);
- } @else {
- $hover-color: mix($color, $background, 80);
- }
- } @else if $color-lightness == 0 {
- /* Black */
- @if $background-lightness < 10 {
- $hover-color: lighten($background, 20);
- } @else {
- $hover-color: mix($color, $background, 60);
- }
- } @else if $background-lightness < $color-lightness {
- /* Light text on dark background */
- $style: 'lighten';
- @if $color-lightness > 90 {
- /* Color too light to lighten */
- $hover-color: darken($color, 20);
- $style: 'darken';
- } @else {
- $hover-color: lighten($color, 20);
- }
-
- } @else {
- /* Dark text on light background */
- $style: 'darken';
- @if $color-lightness < 15 {
- /* Color is too dark to further darken */
- $hover-color: lighten($color, 20);
- $style: 'lighten';
- } @else {
- $hover-color: darken($color, 20);
- }
- }
-
- /*
- Sometimes the $hover-color won't have enough contrast
- with the background. We'll try to fix this below by
- increasing our darken/lighten range by +/- 5
- */
-
- /* Only test if the $color isn't white or black */
- /*@if $color-lightness != 0 and $color-lightness != 100 {
- $new-contrast: contrast-ratio($background, $hover-color);
- @if $new-contrast < 8 {
- @for $i from 15 to 25 {
- @if $style == 'darken' {
- $test-color: darken($color, $i);
- } @else {
- $test-color: lighten($color, $i);
- }
- $test-contrast: contrast-ratio($background, $test-color);
-
- @if $test-contrast > $new-contrast {
- $new-contrast: $test-contrast;
- $hover-color: $test-color;
- }
- }
- }
- }*/
-
- @return $hover-color;
-}
-
-
-// Precomputed linear color channel values, for use in contrast calculations.
-// See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
-//
-// Algorithm, for c in 0 to 255:
-// f(c) {
-// c = c / 255;
-// return c < 0.03928 ? c / 12.92 : Math.pow((c + 0.055) / 1.055, 2.4);
-// }
-//
-// This lookup table is needed since there is no `pow` in SASS.
-$linear-channel-values:
- 0
- .0003035269835488375
- .000607053967097675
- .0009105809506465125
- .00121410793419535
- .0015176349177441874
- .001821161901293025
- .0021246888848418626
- .0024282158683907
- .0027317428519395373
- .003035269835488375
- .003346535763899161
- .003676507324047436
- .004024717018496307
- .004391442037410293
- .004776953480693729
- .005181516702338386
- .005605391624202723
- .006048833022857054
- .006512090792594475
- .006995410187265387
- .007499032043226175
- .008023192985384994
- .008568125618069307
- .009134058702220787
- .00972121732023785
- .010329823029626936
- .010960094006488246
- .011612245179743885
- .012286488356915872
- .012983032342173012
- .013702083047289686
- .014443843596092545
- .01520851442291271
- .01599629336550963
- .016807375752887384
- .017641954488384078
- .018500220128379697
- .019382360956935723
- .0202885630566524
- .021219010376003555
- .022173884793387385
- .02315336617811041
- .024157632448504756
- .02518685962736163
- .026241221894849898
- .027320891639074894
- .028426039504420793
- .0295568344378088
- .030713443732993635
- .03189603307301153
- .033104766570885055
- .03433980680868217
- .03560131487502034
- .03688945040110004
- .0382043715953465
- .03954623527673284
- .04091519690685319
- .042311410620809675
- .043735029256973465
- .04518620438567554
- .046665086336880095
- .04817182422688942
- .04970656598412723
- .05126945837404324
- .052860647023180246
- .05448027644244237
- .05612849004960009
- .05780543019106723
- .0595112381629812
- .06124605423161761
- .06301001765316767
- .06480326669290577
- .06662593864377289
- .06847816984440017
- .07036009569659588
- .07227185068231748
- .07421356838014963
- .07618538148130785
- .07818742180518633
- .08021982031446832
- .0822827071298148
- .08437621154414882
- .08650046203654976
- .08865558628577294
- .09084171118340768
- .09305896284668745
- .0953074666309647
- .09758734714186246
- .09989872824711389
- .10224173308810132
- .10461648409110419
- .10702310297826761
- .10946171077829933
- .1119324278369056
- .11443537382697373
- .11697066775851084
- .11953842798834562
- .12213877222960187
- .12477181756095049
- .12743768043564743
- .1301364766903643
- .13286832155381798
- .13563332965520566
- .13843161503245183
- .14126329114027164
- .14412847085805777
- .14702726649759498
- .14995978981060856
- .15292615199615017
- .1559264637078274
- .1589608350608804
- .162029375639111
- .1651321945016676
- .16826940018969075
- .1714411007328226
- .17464740365558504
- .17788841598362912
- .18116424424986022
- .184474994500441
- .18782077230067787
- .19120168274079138
- .1946178304415758
- .19806931955994886
- .20155625379439707
- .20507873639031693
- .20863687014525575
- .21223075741405523
- .21586050011389926
- .2195261997292692
- .2232279573168085
- .22696587351009836
- .23074004852434915
- .23455058216100522
- .238397573812271
- .24228112246555486
- .24620132670783548
- .25015828472995344
- .25415209433082675
- .2581828529215958
- .26225065752969623
- .26635560480286247
- .2704977910130658
- .27467731206038465
- .2788942634768104
- .2831487404299921
- .2874408377269175
- .29177064981753587
- .2961382707983211
- .3005437944157765
- .3049873140698863
- .30946892281750854
- .31398871337571754
- .31854677812509186
- .32314320911295075
- .3277780980565422
- .33245153634617935
- .33716361504833037
- .3419144249086609
- .3467040563550296
- .35153259950043936
- .3564001441459435
- .3613067797835095
- .3662525955988395
- .3712376804741491
- .3762621229909065
- .38132601143253014
- .386429433787049
- .39157247774972326
- .39675523072562685
- .4019777798321958
- .4072402119017367
- .41254261348390375
- .4178850708481375
- .4232676699860717
- .4286904966139066
- .43415363617474895
- .4396571738409188
- .44520119451622786
- .45078578283822346
- .45641102318040466
- .4620769996544071
- .467783796112159
- .47353149614800955
- .4793201831008268
- .4851499400560704
- .4910208498478356
- .4969329950608704
- .5028864580325687
- .5088813208549338
- .5149176653765214
- .5209955732043543
- .5271151257058131
- .5332764040105052
- .5394794890121072
- .5457244613701866
- .5520114015120001
- .5583403896342679
- .5647115057049292
- .5711248294648731
- .5775804404296506
- .5840784178911641
- .5906188409193369
- .5972017883637634
- .6038273388553378
- .6104955708078648
- .6172065624196511
- .6239603916750761
- .6307571363461468
- .6375968739940326
- .6444796819705821
- .6514056374198242
- .6583748172794485
- .665387298282272
- .6724431569576875
- .6795424696330938
- .6866853124353135
- .6938717612919899
- .7011018919329731
- .7083757798916868
- .7156935005064807
- .7230551289219693
- .7304607400903537
- .7379104087727308
- .7454042095403874
- .7529422167760779
- .7605245046752924
- .768151147247507
- .7758222183174236
- .7835377915261935
- .7912979403326302
- .799102738014409
- .8069522576692516
- .8148465722161012
- .8227857543962835
- .8307698767746546
- .83879901174074
- .846873231509858
- .8549926081242338
- .8631572134541023
- .8713671191987972
- .8796223968878317
- .8879231178819663
- .8962693533742664
- .9046611743911496
- .9130986517934192
- .9215818562772946
- .9301108583754237
- .938685728457888
- .9473065367331999
- .9559733532492861
- .9646862478944651
- .9734452903984125
- .9822505503331171
- .9911020971138298
- 1;
-
-/**
- * Calculate the luminance for a color.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-@function luminance($color) {
- $red: nth($linear-channel-values, red($color) + 1);
- $green: nth($linear-channel-values, green($color) + 1);
- $blue: nth($linear-channel-values, blue($color) + 1);
-
- @return .2126 * $red + .7152 * $green + .0722 * $blue;
-}
-
-/**
- * Calculate the contrast ratio between two colors.
- * See https://www.w3.org/TR/WCAG20-TECHS/G17.html#G17-tests
- */
-@function contrast($back, $front) {
- $backLum: luminance($back) + .05;
- $foreLum: luminance($front) + .05;
-
- @return max($backLum, $foreLum) / min($backLum, $foreLum);
-}
-
-/**
- * Determine whether to use dark or light text on top of given color.
- * Returns black for dark text and white for light text.
- */
-@function maximize-color-contrast($color) {
- $lightContrast: contrast($color, white);
- $darkContrast: contrast($color, black);
-
- @if ($lightContrast > $darkContrast) {
- @return white;
- }
- @else {
- @return black;
- }
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/_prefix.scss b/themes/learn2-git-sync/scss/_prefix.scss
deleted file mode 100644
index 6e5e22f..0000000
--- a/themes/learn2-git-sync/scss/_prefix.scss
+++ /dev/null
@@ -1,21 +0,0 @@
-// REQUIRED DEPENDENCIES - DO NOT CHANGE
-
-// Load Third Party Libraries
-@import "vendor/bourbon/bourbon";
-
-// Load Nucleus Configuration
-@import "configuration/nucleus/base";
-
-// Load Template Configuration
-@import "configuration/theme/base";
-
-// Load Nucleus Mixins and Functions
-@import "nucleus/functions/base";
-@import "nucleus/mixins/base";
-
-// Load Template Library
-@import "theme/modules/base";
-
-// Utils
-@import "hover-color";
-@import "contrast";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/_standard_colors.scss b/themes/learn2-git-sync/scss/_standard_colors.scss
deleted file mode 100644
index fcadea0..0000000
--- a/themes/learn2-git-sync/scss/_standard_colors.scss
+++ /dev/null
@@ -1,24 +0,0 @@
-// Standard Colors
-$turquoise: #1ABC9C;
-$green_sea: #16A085;
-$emerald: #2ECC71;
-$nephritis: #27AE60;
-$peter_river: #3498DB;
-$belize_hole: #2980B9;
-$amethyst: #A66BBE;
-$wisteria: #8E44AD;
-$wet_asphalt: #34495E;
-$midnight_blue: #2C3E50;
-$sunflower: #F2CA27;
-$orange: #F39C12;
-$carrot: #E67E22;
-$pumpkin: #D35400;
-$alizarin: #E74C3C;
-$pomegranate: #C0392B;
-$clouds: #ECF0F1;
-$silver: #BDC3C7;
-$concrete: #95A5A6;
-$asbestos: #7F8C8D;
-
-$progress-bar: #50D681;
-$star-color: #F8C74A;
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/configuration/nucleus/_base.scss b/themes/learn2-git-sync/scss/configuration/nucleus/_base.scss
deleted file mode 100644
index 0b7e898..0000000
--- a/themes/learn2-git-sync/scss/configuration/nucleus/_base.scss
+++ /dev/null
@@ -1,14 +0,0 @@
-// Core
-@import "core";
-
-// Breakpoints
-@import "breakpoints";
-
-// Layout
-@import "layout";
-
-// Typography
-@import "typography";
-
-// Nav
-@import "nav";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/configuration/nucleus/_breakpoints.scss b/themes/learn2-git-sync/scss/configuration/nucleus/_breakpoints.scss
deleted file mode 100644
index 55dbbd0..0000000
--- a/themes/learn2-git-sync/scss/configuration/nucleus/_breakpoints.scss
+++ /dev/null
@@ -1,17 +0,0 @@
-// Media Device Breakpoints
-$large-desktop-container: 75.000em !default;
-$desktop-container: 60.000em !default;
-$tablet-container: 48.000em !default;
-$large-mobile-container: 30.000em !default;
-$mobile-container: 100% !default;
-
-// Breakpoint Variables For Particles
-$media: "all" !default;
-$desktop-max: "#{$media} and (max-width:#{$desktop-container - 0.062})" !default;
-$mobile-only: "#{$media} and (max-width:#{$tablet-container - 0.062})" !default;
-$no-mobile: "#{$media} and (min-width:#{$tablet-container})" !default;
-$small-mobile-range: "#{$media} and (max-width:#{$large-mobile-container})" !default;
-$large-mobile-range: "#{$media} and (min-width:#{$large-mobile-container + 0.063}) and (max-width:#{$tablet-container - 0.062})" !default;
-$tablet-range: "#{$media} and (min-width:#{$tablet-container}) and (max-width:#{$desktop-container - 0.062})" !default;
-$desktop-range: "#{$media} and (min-width:#{$desktop-container}) and (max-width:#{$large-desktop-container - 0.062})" !default;
-$large-desktop-range: "#{$media} and (min-width:#{$large-desktop-container})" !default;
diff --git a/themes/learn2-git-sync/scss/configuration/nucleus/_core.scss b/themes/learn2-git-sync/scss/configuration/nucleus/_core.scss
deleted file mode 100644
index 49c79ff..0000000
--- a/themes/learn2-git-sync/scss/configuration/nucleus/_core.scss
+++ /dev/null
@@ -1,2 +0,0 @@
-// Border Radius
-$core-border-radius: rem(3) !default;
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/configuration/nucleus/_layout.scss b/themes/learn2-git-sync/scss/configuration/nucleus/_layout.scss
deleted file mode 100644
index 98b2803..0000000
--- a/themes/learn2-git-sync/scss/configuration/nucleus/_layout.scss
+++ /dev/null
@@ -1,8 +0,0 @@
-// Content Block Spacing Variables
-$content-margin: 0.625rem !default;
-$content-padding: 0.938rem !default;
-
-// Fixed Block Variables
-$fixed-block-full: percentage(1/4) !default;
-$fixed-block-desktop: percentage(1/3) !default;
-$fixed-block-tablet: percentage(1/2) !default;
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/configuration/nucleus/_nav.scss b/themes/learn2-git-sync/scss/configuration/nucleus/_nav.scss
deleted file mode 100644
index 5a63a55..0000000
--- a/themes/learn2-git-sync/scss/configuration/nucleus/_nav.scss
+++ /dev/null
@@ -1,3 +0,0 @@
-// Dropdowns
-$dropdown-width: 140px !default;
-$flyout-width: 140px !default;
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/configuration/nucleus/_typography.scss b/themes/learn2-git-sync/scss/configuration/nucleus/_typography.scss
deleted file mode 100644
index 2a66536..0000000
--- a/themes/learn2-git-sync/scss/configuration/nucleus/_typography.scss
+++ /dev/null
@@ -1,14 +0,0 @@
-// Base Sizes
-$core-font-size: 1.05rem !default;
-$core-line-height: 1.7 !default;
-
-// Heading Sizes
-$h1-font-size: $core-font-size + 2.20 !default;
-$h2-font-size: $core-font-size + 1.50 !default;
-$h3-font-size: $core-font-size + 1.10 !default;
-$h4-font-size: $core-font-size + 0.75 !default;
-$h5-font-size: $core-font-size + 0.35 !default;
-$h6-font-size: $core-font-size - 0.15 !default;
-
-// Spacing
-$leading-margin: $core-line-height * 1rem !default;
diff --git a/themes/learn2-git-sync/scss/configuration/theme/_base.scss b/themes/learn2-git-sync/scss/configuration/theme/_base.scss
deleted file mode 100644
index b97612e..0000000
--- a/themes/learn2-git-sync/scss/configuration/theme/_base.scss
+++ /dev/null
@@ -1,5 +0,0 @@
-// Colors
-@import "colors";
-
-// Typography
-@import "bullets";
diff --git a/themes/learn2-git-sync/scss/configuration/theme/_bullets.scss b/themes/learn2-git-sync/scss/configuration/theme/_bullets.scss
deleted file mode 100644
index 004a88a..0000000
--- a/themes/learn2-git-sync/scss/configuration/theme/_bullets.scss
+++ /dev/null
@@ -1,5 +0,0 @@
-$bullet-icon-size: 3.5rem;
-
-$bullet-icon-color-1: $core-accent;
-$bullet-icon-color-2: adjust-hue($core-accent, -20);
-$bullet-icon-color-3: adjust-hue($core-accent, -130);
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/configuration/theme/_colors.scss b/themes/learn2-git-sync/scss/configuration/theme/_colors.scss
deleted file mode 100644
index f4be185..0000000
--- a/themes/learn2-git-sync/scss/configuration/theme/_colors.scss
+++ /dev/null
@@ -1,60 +0,0 @@
-// Core
-$core-text: #555;
-$core-accent: #1694CA;
-$secondary-link: #F8B450;
-$white: #fff;
-$black: #000;
-$light-gray: #ddd;
-
-// Borders
-$core-border-color: $light-gray;
-$core-border-color-hover: darken($core-border-color, 10);
-$core-border-color-focus: $core-accent;
-
-// Shadows
-$core-box-shadow: inset 0 1px 3px hsla(0, 0%, 0%, 0.06);
-$core-box-shadow-focus: $core-box-shadow, 0 0 5px rgba(darken($core-border-color-focus, 5), 0.7);
-
-// Background
-$page-bg: #fff;
-
-// Header
-$header-text: #aaa;
-
-// Nav
-$nav-link: #aaa;
-
-// Showcase
-$showcase-bg: lighten($core-accent, 6%);
-$showcase-text: #fff;
-
-// Feature
-$feature-bg: #fff;
-
-// Main Body
-$main-bg: #f7f7f7;
-$body-border: darken($main-bg, 5%);
-
-// Sidebar
-$sidebar-text: #aaa;
-
-// Bottom
-$bottom-bg: #f7f7f7;
-$bottom-text: $core-text;
-
-// Footer
-$footer-bg: #404040;
-$footer-text: #ccc;
-
-$rule-color: #F0F2F4;
-$code-text: #c7254e;
-$code-bg: #f9f2f4;
-$pre-text: #237794;
-$pre-bg: #f6f6f6;
-
-// Dark Contrast variation
-$dark-navbar-text: #999;
-$dark-sidebar: #222;
-$dark-sidebar-text: #999;
-$dark-main-bg: #333;
-$dark-body-border: #666;
diff --git a/themes/learn2-git-sync/scss/nucleus.scss b/themes/learn2-git-sync/scss/nucleus.scss
deleted file mode 100644
index 9cb9575..0000000
--- a/themes/learn2-git-sync/scss/nucleus.scss
+++ /dev/null
@@ -1,27 +0,0 @@
-// REQUIRED DEPENDENCIES - DO NOT CHANGE
-
-// Load Third Party Libraries
-@import "vendor/bourbon/bourbon";
-
-// Load Nucleus Configuration
-@import "configuration/nucleus/base";
-
-// Load Nucleus Mixins and Functions
-@import "nucleus/functions/base";
-@import "nucleus/mixins/base";
-
-//-------------------------------------------
-
-// LOAD NUCLEUS COMPONENTS
-
-// Core
-@import "nucleus/core";
-
-// Flex
-@import "nucleus/flex";
-
-// Typography
-@import "nucleus/typography";
-
-// Forms
-@import "nucleus/forms";
diff --git a/themes/learn2-git-sync/scss/nucleus/_core.scss b/themes/learn2-git-sync/scss/nucleus/_core.scss
deleted file mode 100644
index 0cd0e8d..0000000
--- a/themes/learn2-git-sync/scss/nucleus/_core.scss
+++ /dev/null
@@ -1,217 +0,0 @@
-*, *::before, *::after {
- @include box-sizing(border-box);
-}
-
-@-webkit-viewport{width:device-width}
-@-moz-viewport{width:device-width}
-@-ms-viewport{width:device-width}
-@-o-viewport{width:device-width}
-@viewport{width:device-width}
-
-html {
- font-size: 100%;
- -ms-text-size-adjust: 100%;
- -webkit-text-size-adjust: 100%;
-}
-
-body {
- margin: 0;
-}
-
-article,
-aside,
-details,
-figcaption,
-figure,
-footer,
-header,
-hgroup,
-main,
-nav,
-section,
-summary {
- display: block;
-}
-
-audio,
-canvas,
-progress,
-video {
- display: inline-block;
- vertical-align: baseline;
-}
-
-audio:not([controls]) {
- display: none;
- height: 0;
-}
-
-[hidden],
-template {
- display: none;
-}
-
-a {
- background: transparent;
- text-decoration: none;
-}
-
-a:active,
-a:hover {
- outline: 0;
-}
-
-abbr[title] {
- border-bottom: 1px dotted;
-}
-
-b,
-strong {
- font-weight: bold;
-}
-
-dfn {
- font-style: italic;
-}
-
-mark {
- background: #FFFF27;
- color: #333;
-}
-
-sub,
-sup {
- font-size: $core-font-size - 0.250;
- line-height: 0;
- position: relative;
- vertical-align: baseline;
-}
-
-sup {
- top: -0.5em;
-}
-
-sub {
- bottom: -0.25em;
-}
-
-img {
- border: 0;
- max-width: 100%;
-}
-
-svg:not(:root) {
- overflow: hidden;
-}
-
-figure {
- margin: 1em 40px;
-}
-
-hr {
- height: 0;
-}
-
-pre {
- overflow: auto;
-}
-
-code,
-kbd,
-pre,
-samp {
- // font-size: $core-font-size;
-}
-
-button,
-input,
-optgroup,
-select,
-textarea {
- color: inherit;
- font: inherit;
- margin: 0;
-}
-
-button {
- overflow: visible;
-}
-
-button,
-select {
- text-transform: none;
-}
-
-button,
-html input[type="button"],
-input[type="reset"],
-input[type="submit"] {
- -webkit-appearance: button;
- cursor: pointer;
-}
-
-button[disabled],
-html input[disabled] {
- cursor: default;
-}
-
-button::-moz-focus-inner,
-input::-moz-focus-inner {
- border: 0;
- padding: 0;
-}
-
-input {
- line-height: normal;
-}
-
-input[type="checkbox"],
-input[type="radio"] {
- padding: 0;
-}
-
-input[type="number"]::-webkit-inner-spin-button,
-input[type="number"]::-webkit-outer-spin-button {
- height: auto;
-}
-
-input[type="search"] {
- -webkit-appearance: textfield;
-}
-
-input[type="search"]::-webkit-search-cancel-button,
-input[type="search"]::-webkit-search-decoration {
- -webkit-appearance: none;
-}
-
-legend {
- border: 0;
- padding: 0;
-}
-
-textarea {
- overflow: auto;
-}
-
-optgroup {
- font-weight: bold;
-}
-
-table {
- border-collapse: collapse;
- border-spacing: 0;
- table-layout: fixed;
- width: 100%;
-}
-
-tr, td, th {
- vertical-align: middle;
-}
-
-th, td {
- padding: ($leading-margin / 4) 0;
-}
-
-th {
- text-align: left;
-}
diff --git a/themes/learn2-git-sync/scss/nucleus/_flex.scss b/themes/learn2-git-sync/scss/nucleus/_flex.scss
deleted file mode 100644
index 4e9a42e..0000000
--- a/themes/learn2-git-sync/scss/nucleus/_flex.scss
+++ /dev/null
@@ -1,189 +0,0 @@
-// Page Container
-.container {
- width: $large-desktop-container;
- margin: 0 auto;
- padding: 0;
- @include breakpoint(desktop-range) {
- width: $desktop-container;
- }
- @include breakpoint(tablet-range) {
- width: $tablet-container;
- }
- @include breakpoint(large-mobile-range) {
- width: $large-mobile-container;
- }
- @include breakpoint(small-mobile-range) {
- width: $mobile-container;
- }
-}
-
-// Grid Row and Column Setup
-.grid {
- @include display(flex);
- @include flex-flow(row);
- list-style: none;
- margin: 0;
- padding: 0;
- @include breakpoint(mobile-only) {
- @include flex-flow(row wrap);
- }
-}
-
-.block {
- @include flex(1);
- min-width: 0;
- min-height: 0;
- @include breakpoint(mobile-only) {
- @include flex(0 100%);
- }
-}
-
-// Content Block Spacing
-.content {
- margin: $content-margin;
- padding: $content-padding;
-}
-
-body [class*="size-"] {
- @include breakpoint(mobile-only) {
- @include flex(0 100%);
- }
-}
-
-// Custom Size Modifiers
-.size-1-2 {
- @include flex(0 percentage(1/2));
-}
-
-.size-1-3 {
- @include flex(0 percentage(1/3));
-}
-
-.size-1-4 {
- @include flex(0 percentage(1/4));
-}
-
-.size-1-5 {
- @include flex(0 percentage(1/5));
-}
-
-.size-1-6 {
- @include flex(0 percentage(1/6));
-}
-
-.size-1-7 {
- @include flex(0 percentage(1/7));
-}
-
-.size-1-8 {
- @include flex(0 percentage(1/8));
-}
-
-.size-1-9 {
- @include flex(0 percentage(1/9));
-}
-
-.size-1-10 {
- @include flex(0 percentage(1/10));
-}
-
-.size-1-11 {
- @include flex(0 percentage(1/11));
-}
-
-.size-1-12 {
- @include flex(0 percentage(1/12));
-}
-
-@include breakpoint(tablet-range) {
- .size-tablet-1-2 {
- @include flex(0 percentage(1/2));
- }
-
- .size-tablet-1-3 {
- @include flex(0 percentage(1/3));
- }
-
- .size-tablet-1-4 {
- @include flex(0 percentage(1/4));
- }
-
- .size-tablet-1-5 {
- @include flex(0 percentage(1/5));
- }
-
- .size-tablet-1-6 {
- @include flex(0 percentage(1/6));
- }
-
- .size-tablet-1-7 {
- @include flex(0 percentage(1/7));
- }
-
- .size-tablet-1-8 {
- @include flex(0 percentage(1/8));
- }
-
- .size-tablet-1-9 {
- @include flex(0 percentage(1/9));
- }
-
- .size-tablet-1-10 {
- @include flex(0 percentage(1/10));
- }
-
- .size-tablet-1-11 {
- @include flex(0 percentage(1/11));
- }
-
- .size-tablet-1-12 {
- @include flex(0 percentage(1/12));
- }
-}
-
-// Fix for Firefox versions 27 and below
-@include breakpoint(mobile-only) {
- @supports not (flex-wrap: wrap) {
- .grid {
- display: block;
- @include flex-wrap(inherit);
- }
- .block {
- display: block;
- @include flex(inherit);
- }
- }
-}
-
-// Reordering
-.first-block {
- -webkit-box-ordinal-group: 0;
- -webkit-order: -1;
- -ms-flex-order: -1;
- order: -1;
-}
-
-.last-block {
- -webkit-box-ordinal-group: 2;
- -webkit-order: 1;
- -ms-flex-order: 1;
- order: 1;
-}
-
-// Fixed Grid Style
-.fixed-blocks {
- @include flex-flow(row wrap);
- .block {
- @include flex(inherit);
- width: $fixed-block-full;
- @include breakpoint(desktop-range) {
- width: $fixed-block-desktop;
- }
- @include breakpoint(tablet-range) {
- width: $fixed-block-tablet;
- }
- @include breakpoint(mobile-only) {
- width: 100%;
- }
- }
-}
diff --git a/themes/learn2-git-sync/scss/nucleus/_forms.scss b/themes/learn2-git-sync/scss/nucleus/_forms.scss
deleted file mode 100644
index 0c3e91f..0000000
--- a/themes/learn2-git-sync/scss/nucleus/_forms.scss
+++ /dev/null
@@ -1,63 +0,0 @@
-fieldset {
- border: 0;
- padding: $content-padding;
- margin: 0 0 $leading-margin 0;
-}
-
-input,
-label,
-select {
- display: block;
-}
-
-label {
- margin-bottom: $leading-margin / 4;
-
- &.required:after {
- content: "*";
- }
-
- abbr {
- display: none;
- }
-}
-
-textarea, #{$all-text-inputs}, select[multiple=multiple] {
- @include transition(border-color);
- border-radius: $core-border-radius;
- margin-bottom: $leading-margin / 2;
- padding: ($leading-margin / 4) ($leading-margin / 4);
- width: 100%;
-
- &:focus {
- outline: none;
- }
-}
-
-textarea {
- resize: vertical;
-}
-
-input[type="checkbox"], input[type="radio"] {
- display: inline;
- margin-right: $leading-margin / 4;
-}
-
-input[type="file"] {
- width: 100%;
-}
-
-select {
- width: auto;
- max-width: 100%;
- margin-bottom: $leading-margin;
-}
-
-button,
-input[type="submit"] {
- cursor: pointer;
- user-select: none;
- vertical-align: middle;
- white-space: nowrap;
- border: inherit;
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/nucleus/_typography.scss b/themes/learn2-git-sync/scss/nucleus/_typography.scss
deleted file mode 100644
index 5e74101..0000000
--- a/themes/learn2-git-sync/scss/nucleus/_typography.scss
+++ /dev/null
@@ -1,86 +0,0 @@
-// Body Base
-body {
- font-size: $core-font-size;
- line-height: $core-line-height;
-}
-
-// Headings
-h1, h2, h3, h4, h5, h6 {
- margin: $leading-margin / 2 0 $leading-margin 0;
- text-rendering: optimizeLegibility;
-}
-
-h1 {
- font-size: $h1-font-size;
-}
-
-h2 {
- font-size: $h2-font-size;
-}
-
-h3 {
- font-size: $h3-font-size;
-}
-
-h4 {
- font-size: $h4-font-size;
-}
-
-h5 {
- font-size: $h5-font-size;
-}
-
-h6 {
- font-size: $h6-font-size;
-}
-
-// Paragraph
-p {
- margin: $leading-margin 0;
-}
-
-// Lists
-ul, ol {
- margin-top: $leading-margin;
- margin-bottom: $leading-margin;
- ul, ol {
- margin-top: 0;
- margin-bottom: 0;
- }
-}
-
-// Blockquote
-blockquote {
- margin: $leading-margin 0;
- padding-left: $leading-margin / 2;
-}
-
-cite {
- display: block;
- font-size: $core-font-size - 0.125;
- &:before {
- content: "\2014 \0020";
- }
-}
-
-// Inline and Code
-pre {
- margin: $leading-margin 0;
- padding: $content-padding;
-}
-
-code {
- vertical-align: bottom;
-}
-
-// Extras
-small {
- font-size: $core-font-size - 0.125;
-}
-
-hr {
- border-left: none;
- border-right: none;
- border-top: none;
- margin: $leading-margin 0;
-}
diff --git a/themes/learn2-git-sync/scss/nucleus/functions/_base.scss b/themes/learn2-git-sync/scss/nucleus/functions/_base.scss
deleted file mode 100644
index ffef250..0000000
--- a/themes/learn2-git-sync/scss/nucleus/functions/_base.scss
+++ /dev/null
@@ -1,2 +0,0 @@
-@import "direction";
-@import "range";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/nucleus/functions/_direction.scss b/themes/learn2-git-sync/scss/nucleus/functions/_direction.scss
deleted file mode 100644
index e336bb5..0000000
--- a/themes/learn2-git-sync/scss/nucleus/functions/_direction.scss
+++ /dev/null
@@ -1,37 +0,0 @@
-@function opposite-direction($dir) {
- @if $dir == 'left' {
- @return right;
- }
- @else if $dir == 'right' {
- @return left;
- }
- @else if $dir == 'ltr' {
- @return rtl;
- }
- @else if $dir == 'rtl' {
- @return ltr;
- }
- @else if $dir == 'top' {
- @return bottom;
- }
- @else if $dir == 'bottom' {
- @return top;
- }
- @else {
- @warn "#{$dir} is not a direction! Make sure your direction is all lowercase!";
- @return false;
- }
-}
-
-@function named-direction($dir) {
- @if $dir == 'ltr' {
- @return left;
- }
- @else if $dir == 'rtl' {
- @return right;
- }
- @else {
- @warn "#{$dir} is not a valid HTML direction! Make sure you are using a valid HTML direction";
- @return false;
- }
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/nucleus/functions/_range.scss b/themes/learn2-git-sync/scss/nucleus/functions/_range.scss
deleted file mode 100644
index 0e9dec9..0000000
--- a/themes/learn2-git-sync/scss/nucleus/functions/_range.scss
+++ /dev/null
@@ -1,13 +0,0 @@
-@function lower-bound($range){
- @if length($range) <= 0 {
- @return 0;
- }
- @return nth($range,1);
-}
-
-@function upper-bound($range) {
- @if length($range) < 2 {
- @return 999999999999;
- }
- @return nth($range, 2);
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/nucleus/mixins/_base.scss b/themes/learn2-git-sync/scss/nucleus/mixins/_base.scss
deleted file mode 100644
index 5e4a709..0000000
--- a/themes/learn2-git-sync/scss/nucleus/mixins/_base.scss
+++ /dev/null
@@ -1,2 +0,0 @@
-@import "breakpoints";
-@import "utilities";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/nucleus/mixins/_breakpoints.scss b/themes/learn2-git-sync/scss/nucleus/mixins/_breakpoints.scss
deleted file mode 100644
index 67e1e46..0000000
--- a/themes/learn2-git-sync/scss/nucleus/mixins/_breakpoints.scss
+++ /dev/null
@@ -1,27 +0,0 @@
-// Breakpoints
-@mixin breakpoint($breakpoint, $media: all) {
- @if $breakpoint == large-desktop-range {
- @media only #{$media} and (min-width: $large-desktop-container) { @content; }
- }
- @else if $breakpoint == desktop-range {
- @media only #{$media} and (min-width: $desktop-container) and (max-width: $large-desktop-container - 0.062) { @content; }
- }
- @else if $breakpoint == tablet-range {
- @media only #{$media} and (min-width: $tablet-container) and (max-width: $desktop-container - 0.062) { @content; }
- }
- @else if $breakpoint == large-mobile-range {
- @media only #{$media} and (min-width: $large-mobile-container + 0.063) and (max-width: $tablet-container - 0.062) { @content; }
- }
- @else if $breakpoint == small-mobile-range {
- @media only #{$media} and (max-width: $large-mobile-container) { @content; }
- }
- @else if $breakpoint == no-mobile {
- @media only #{$media} and (min-width: $tablet-container) { @content; }
- }
- @else if $breakpoint == mobile-only {
- @media only #{$media} and (max-width: $tablet-container - 0.062) { @content; }
- }
- @else if $breakpoint == desktop-only {
- @media only #{$media} and (max-width: $desktop-container - 0.062) { @content; }
- }
-}
diff --git a/themes/learn2-git-sync/scss/nucleus/mixins/_utilities.scss b/themes/learn2-git-sync/scss/nucleus/mixins/_utilities.scss
deleted file mode 100644
index adda82f..0000000
--- a/themes/learn2-git-sync/scss/nucleus/mixins/_utilities.scss
+++ /dev/null
@@ -1,31 +0,0 @@
-
-
-// List Reset
-%list-reset {
- margin: 0;
- padding: 0;
- list-style: none;
-}
-
-// Vertical Centering
-%vertical-align {
- position: relative;
- top: 50%;
- -webkit-transform: translateY(-50%);
- -moz-transform: translateY(-50%);
- -o-transform: translateY(-50%);
- -ms-transform: translateY(-50%);
- transform: translateY(-50%);
-}
-
-// Columns
-@mixin columns($columns) {
- width: percentage(1/$columns);
-}
-
-// Float with margin variable
-@mixin float($direction, $margin: 0) {
- float: $direction;
- margin-#{opposite-direction($direction)}: $margin;
-}
-
diff --git a/themes/learn2-git-sync/scss/nucleus/particles/_align-text.scss b/themes/learn2-git-sync/scss/nucleus/particles/_align-text.scss
deleted file mode 100644
index 94ef815..0000000
--- a/themes/learn2-git-sync/scss/nucleus/particles/_align-text.scss
+++ /dev/null
@@ -1,46 +0,0 @@
-// Alignment Classes
-$align-class-names:
- large-desktop,
- desktop,
- tablet,
- large-mobile,
- small-mobile,
- no-mobile,
- mobile-only;
-
-// Breakpoints
-$align-class-breakpoints:
- $large-desktop-range,
- $desktop-range,
- $tablet-range,
- $large-mobile-range,
- $small-mobile-range,
- $no-mobile,
- $mobile-only;
-
-// Create Responsive Alignment Classes
-@mixin align-classes{
- .text-left {
- text-align: left !important;
- }
- .text-right {
- text-align: right !important;
- }
- .text-center {
- text-align: center !important;
- }
- .text-justify {
- text-align: justify !important;
- }
-
- @for $i from 1 through length($align-class-names) {
- @media #{(nth($align-class-breakpoints, $i))} {
- .#{(nth($align-class-names, $i))}-text-left { text-align: left !important; }
- .#{(nth($align-class-names, $i))}-text-right { text-align: right !important; }
- .#{(nth($align-class-names, $i))}-text-center { text-align: center !important; }
- .#{(nth($align-class-names, $i))}-text-justify { text-align: justify !important; }
- }
- }
-}
-
-@include align-classes;
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/nucleus/particles/_visibility.scss b/themes/learn2-git-sync/scss/nucleus/particles/_visibility.scss
deleted file mode 100644
index e69de29..0000000
diff --git a/themes/learn2-git-sync/scss/styles/arctic.scss b/themes/learn2-git-sync/scss/styles/arctic.scss
deleted file mode 100644
index 6b14ea6..0000000
--- a/themes/learn2-git-sync/scss/styles/arctic.scss
+++ /dev/null
@@ -1,49 +0,0 @@
-@import "../prefix";
-
-// Theme
-$theme-main: #CDCDCD;
-$theme-secondary: #49494b;
-$theme-main-alt: #99D3DF;
-$theme-secondary-alt: #88B8D6;
-$theme-text: #333333;
-$theme-text-alt: #ffffff;
-$theme-background: #E9E9E9;
-
-// Implementation and customization
-$page-bg: $theme-background;
-$core-text: $theme-text;
-$core-accent: darken($theme-secondary, 25%);
-$secondary-link: darken($core-accent, 15%);
-
-$header-height: 0;
-$header-bg: $theme-main-alt;
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: darken($theme-secondary-alt, 15%);
-$sidebar-text: darken($theme-background, 5%);
-$sidebar-link: lighten($theme-background, 75%);
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: $theme-background;
-$progress-bar: #50D681;
-$check-color: lighten($sidebar-bg, 30%);
-$star-color: #F8C74A;
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-// Body
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-body {
- #sidebar ul li.active > a {
- color: $theme-text !important;
- }
-}
-
-@import "../affix";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/styles/dark_ocean.scss b/themes/learn2-git-sync/scss/styles/dark_ocean.scss
deleted file mode 100644
index 4f7739c..0000000
--- a/themes/learn2-git-sync/scss/styles/dark_ocean.scss
+++ /dev/null
@@ -1,49 +0,0 @@
-@import "../prefix";
-
-// Theme
-$theme-main: #65737E;
-$theme-secondary: #96B5B4;
-$theme-main-alt: #65737E;
-$theme-secondary-alt: #2B303B;
-$theme-text: #2B303B;
-$theme-text-alt: #C0C5CE;
-$theme-background: #2B303B;
-
-// Implementation and customization
-$page-bg: $theme-background;
-$core-text: lighten($theme-text, 75%);
-$core-accent: $theme-text-alt;
-$secondary-link: darken($core-accent, 15%);
-
-$header-height: 0;
-$header-bg: $theme-secondary;
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: $theme-secondary-alt;
-$sidebar-text: darken($theme-background, 5%);
-$sidebar-link: lighten($theme-background, 75%);
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: $theme-background;
-$progress-bar: #50D681;
-$check-color: lighten($sidebar-bg, 30%);
-$star-color: #F8C74A;
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-// Body
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-body {
- #sidebar ul li.active > a {
- color: $theme-text !important;
- }
-}
-
-@import "../affix";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/styles/gold.scss b/themes/learn2-git-sync/scss/styles/gold.scss
deleted file mode 100644
index 8c3668e..0000000
--- a/themes/learn2-git-sync/scss/styles/gold.scss
+++ /dev/null
@@ -1,49 +0,0 @@
-@import "../prefix";
-
-// Theme
-$theme-main: #373737;
-$theme-secondary: #333333;
-$theme-main-alt: #C0B283;
-$theme-secondary-alt: #DCD0C0;
-$theme-text: #333333;
-$theme-text-alt: #ffffff;
-$theme-background: #F4F4F4;
-
-// Implementation and customization
-$page-bg: $theme-background;
-$core-text: $theme-text;
-$core-accent: darken($theme-secondary, 25%);
-$secondary-link: darken($core-accent, 15%);
-
-$header-height: 0;
-$header-bg: $theme-main-alt;
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: darken($theme-secondary-alt, 15%);
-$sidebar-text: darken($theme-background, 5%);
-$sidebar-link: lighten($theme-background, 75%);
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: $theme-background;
-$progress-bar: #50D681;
-$check-color: lighten($sidebar-bg, 30%);
-$star-color: #F8C74A;
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-// Body
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-body {
- #sidebar ul li.active > a {
- color: $theme-text !important;
- }
-}
-
-@import "../affix";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/styles/grey.scss b/themes/learn2-git-sync/scss/styles/grey.scss
deleted file mode 100644
index 88f90bc..0000000
--- a/themes/learn2-git-sync/scss/styles/grey.scss
+++ /dev/null
@@ -1,49 +0,0 @@
-@import "../prefix";
-
-// Theme
-$theme-main: #D8D8D8;
-$theme-secondary: #D1D1D1;
-$theme-main-alt: #848484;
-$theme-secondary-alt: #A5A5A5;
-$theme-text: #080000;
-$theme-text-alt: #ffffff;
-$theme-background: #f9f9f9;
-
-// Implementation and customization
-$page-bg: $theme-background;
-$core-text: $theme-text;
-$core-accent: darken($theme-secondary, 25%);
-$secondary-link: darken($core-accent, 15%);
-
-$header-height: 0;
-$header-bg: $theme-main-alt;
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: $theme-secondary-alt;
-$sidebar-text: darken($theme-background, 5%);
-$sidebar-link: lighten($theme-background, 75%);
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: $theme-background;
-$progress-bar: #50D681;
-$check-color: lighten($sidebar-bg, 30%);
-$star-color: #F8C74A;
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-// Body
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-body {
- #sidebar ul li.active > a {
- color: $theme-text !important;
- }
-}
-
-@import "../affix";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/styles/hoth.scss b/themes/learn2-git-sync/scss/styles/hoth.scss
deleted file mode 100644
index b80035f..0000000
--- a/themes/learn2-git-sync/scss/styles/hoth.scss
+++ /dev/null
@@ -1,41 +0,0 @@
-@import "../prefix";
-
-// Theme
-$theme-main: #CAD1D9;
-$theme-secondary: #383F45;
-$theme-main-alt: #FAFAFA;
-$theme-secondary-alt: darken($theme-secondary, 5%);
-$theme-text: #383F45;
-$theme-text-alt: #2c2d30;
-$theme-background: #FAFAFA;
-
-// Implementation and customization
-$page-bg: $theme-background;
-$core-text: $theme-text;
-$core-accent: $theme-secondary;
-$secondary-link: darken($core-accent, 15%);
-
-$header-height: 0;
-$header-bg: darken(desaturate(adjust-hue($theme-main, -2), 11.90), 24.90);
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: $theme-secondary-alt;
-$sidebar-text: darken($theme-background, 5%);
-$sidebar-link: $theme-background;
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: $theme-secondary-alt;
-$check-color: lighten($sidebar-bg, 30%);
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-// Body
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-@import "../affix";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/styles/metal.scss b/themes/learn2-git-sync/scss/styles/metal.scss
deleted file mode 100644
index 1c48713..0000000
--- a/themes/learn2-git-sync/scss/styles/metal.scss
+++ /dev/null
@@ -1,43 +0,0 @@
-@import "../prefix";
-
-// Theme
-$theme-main: #bd8c7d;
-$theme-secondary: #d1bfa7;
-$theme-main-alt: #8e8e90;
-$theme-secondary-alt: #49494b;
-$theme-text: #000000;
-$theme-text-alt: #ffffff;
-$theme-background: #e8e8e6;
-
-// Implementation and customization
-$page-bg: $theme-text-alt;
-$core-text: $theme-text;
-$core-accent: darken(desaturate($theme-main, 0.22), 5.10);
-$secondary-link: darken($core-accent, 15%);
-
-$header-height: 0;
-$header-bg: $theme-main;
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: $theme-secondary-alt;
-$sidebar-text: darken($theme-background, 5%);
-$sidebar-link: $theme-background;
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: $theme-background;
-$progress-bar: #50D681;
-$check-color: lighten($sidebar-bg, 30%);
-$star-color: #F8C74A;
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-// Body
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-@import "../affix";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/styles/navy_sunrise.scss b/themes/learn2-git-sync/scss/styles/navy_sunrise.scss
deleted file mode 100644
index 8f431b3..0000000
--- a/themes/learn2-git-sync/scss/styles/navy_sunrise.scss
+++ /dev/null
@@ -1,49 +0,0 @@
-@import "../prefix";
-
-// Theme
-$theme-main: #18121E;
-$theme-secondary: #233237;
-$theme-main-alt: #984B43;
-$theme-secondary-alt: #EAC67A;
-$theme-text: #333333;
-$theme-text-alt: #ffffff;
-$theme-background: #F4F4F4;
-
-// Implementation and customization
-$page-bg: $theme-background;
-$core-text: $theme-text;
-$core-accent: $theme-secondary;
-$secondary-link: darken($core-accent, 15%);
-
-$header-height: 0;
-$header-bg: $theme-secondary-alt;
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: $theme-main-alt;
-$sidebar-text: darken($theme-background, 5%);
-$sidebar-link: lighten($theme-background, 75%);
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: $theme-background;
-$progress-bar: #50D681;
-$check-color: lighten($sidebar-bg, 30%);
-$star-color: #F8C74A;
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-// Body
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-body {
- #sidebar ul li.active > a {
- color: $theme-text !important;
- }
-}
-
-@import "../affix";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/styles/sunrise.scss b/themes/learn2-git-sync/scss/styles/sunrise.scss
deleted file mode 100644
index e0c1d04..0000000
--- a/themes/learn2-git-sync/scss/styles/sunrise.scss
+++ /dev/null
@@ -1,49 +0,0 @@
-@import "../prefix";
-
-// Theme
-$theme-main: #d56073;
-$theme-secondary: lighten($theme-main, 15%);
-$theme-main-alt: #ec9e69;
-$theme-secondary-alt: darken($theme-main-alt, 15%);
-$theme-text: #000000;
-$theme-text-alt: #ffffff;
-$theme-background: #ffff8f;
-
-// Implementation and customization
-$page-bg: $theme-background;
-$core-text: $theme-text;
-$core-accent: darken($theme-secondary, 25%);
-$secondary-link: darken($core-accent, 15%);
-
-$header-height: 0;
-$header-bg: $theme-main-alt;
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: darken($theme-secondary-alt, 15%);
-$sidebar-text: darken($theme-background, 5%);
-$sidebar-link: lighten($theme-background, 75%);
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: $theme-background;
-$progress-bar: #50D681;
-$check-color: lighten($sidebar-bg, 30%);
-$star-color: #F8C74A;
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-// Body
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-body {
- #sidebar ul li.active > a {
- color: $theme-text !important;
- }
-}
-
-@import "../affix";
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/theme.scss b/themes/learn2-git-sync/scss/theme.scss
deleted file mode 100644
index 06105f3..0000000
--- a/themes/learn2-git-sync/scss/theme.scss
+++ /dev/null
@@ -1,61 +0,0 @@
-// REQUIRED DEPENDENCIES - DO NOT CHANGE
-
-// Load Third Party Libraries
-@import "vendor/bourbon/bourbon";
-
-// Load Nucleus Configuration
-@import "configuration/nucleus/base";
-
-// Load Template Configuration
-@import "configuration/theme/base";
-
-// Load Nucleus Mixins and Functions
-@import "nucleus/functions/base";
-@import "nucleus/mixins/base";
-
-// Load Template Library
-@import "theme/modules/base";
-
-//-------------------------------------------
-
-// TEMPLATE COMPONENTS
-
-// Configuration
-@import "theme/configuration";
-
-// Core
-@import "theme/core";
-@import "theme/fonts";
-
-// Forms
-@import "theme/forms";
-
-// Header
-@import "theme/header";
-
-// Nav
-@import "theme/nav";
-
-// Main
-@import "theme/main";
-
-// Typography
-@import "theme/typography";
-
-// Tables
-@import "theme/tables";
-
-// Buttons
-@import "theme/buttons";
-
-// Bullets
-@import "theme/bullets";
-
-// Custom
-@import "theme/tooltips";
-
-// Scrollbar
-@import "theme/scrollbar";
-
-// Custom
-@import "theme/custom";
diff --git a/themes/learn2-git-sync/scss/theme/_bullets.scss b/themes/learn2-git-sync/scss/theme/_bullets.scss
deleted file mode 100644
index c1762df..0000000
--- a/themes/learn2-git-sync/scss/theme/_bullets.scss
+++ /dev/null
@@ -1,60 +0,0 @@
-.bullets {
- margin: $leading-margin 0;
- margin-left: -$leading-margin / 2;
- margin-right: -$leading-margin / 2;
- overflow: auto;
-}
-
-.bullet {
- float: left;
- padding: 0 $leading-margin / 2;
-}
-
-.two-column-bullet {
- @include columns(2);
- @include breakpoint(mobile-only) {
- @include columns(1);
- }
-}
-
-.three-column-bullet {
- @include columns(3);
- @include breakpoint(mobile-only) {
- @include columns(1);
- }
-}
-
-.four-column-bullet {
- @include columns(4);
- @include breakpoint(mobile-only) {
- @include columns(1);
- }
-}
-
-.bullet-icon {
- float: left;
- background: $bullet-icon-color-1;
- padding: $bullet-icon-size / 4;
- width: $bullet-icon-size;
- height: $bullet-icon-size;
- border-radius: 50%;
- color: $white;
- font-size: $bullet-icon-size / 2;
- text-align: center;
-}
-
-.bullet-icon-1 {
- background: $bullet-icon-color-1;
-}
-
-.bullet-icon-2 {
- background: $bullet-icon-color-2;
-}
-
-.bullet-icon-3 {
- background: $bullet-icon-color-3;
-}
-
-.bullet-content {
- margin-left: $bullet-icon-size * 1.3;
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/theme/_buttons.scss b/themes/learn2-git-sync/scss/theme/_buttons.scss
deleted file mode 100644
index 2402bde..0000000
--- a/themes/learn2-git-sync/scss/theme/_buttons.scss
+++ /dev/null
@@ -1,9 +0,0 @@
-.button {
- @extend %button;
- @include button-color($core-accent);
-}
-
-.button-secondary {
- @extend %button;
- @include button-color($secondary-link);
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/theme/_configuration.scss b/themes/learn2-git-sync/scss/theme/_configuration.scss
deleted file mode 100644
index 1e9d777..0000000
--- a/themes/learn2-git-sync/scss/theme/_configuration.scss
+++ /dev/null
@@ -1,45 +0,0 @@
-$header-height: 0;
-$header-bg: $core-accent;
-$sidebar-width: 300px;
-$sidebar-width-smaller: 230px;
-$sidebar-bg: #38424D;
-$sidebar-text: #bbbbbb;
-$sidebar-link: #cccccc;
-$navbar-text: desaturate($core-accent,50%);
-$navbar-bg: #F6F6F6;
-$progress-bar: #50D681;
-$check-color: lighten($sidebar-bg, 30%);
-$star-color: #F8C74A;
-
-// Font Weights
-$font-weight-bold: 600;
-$font-weight-medium: 500;
-$font-weight-regular: 400;
-
-$body-margin: 4rem;
-
-// Logo
-$logo-width: 8rem;
-$logo-height: 2rem;
-
-// Standard Colors
-$turquoise: #1ABC9C;
-$green_sea: #16A085;
-$emerald: #2ECC71;
-$nephritis: #27AE60;
-$peter_river: #3498DB;
-$belize_hole: #2980B9;
-$amethyst: #A66BBE;
-$wisteria: #8E44AD;
-$wet_asphalt: #34495E;
-$midnight_blue: #2C3E50;
-$sunflower: #F2CA27;
-$orange: #F39C12;
-$carrot: #E67E22;
-$pumpkin: #D35400;
-$alizarin: #E74C3C;
-$pomegranate: #C0392B;
-$clouds: #ECF0F1;
-$silver: #BDC3C7;
-$concrete: #95A5A6;
-$asbestos: #7F8C8D;
diff --git a/themes/learn2-git-sync/scss/theme/_core.scss b/themes/learn2-git-sync/scss/theme/_core.scss
deleted file mode 100644
index ce23939..0000000
--- a/themes/learn2-git-sync/scss/theme/_core.scss
+++ /dev/null
@@ -1,49 +0,0 @@
-body {
- background: $page-bg;
- color: $core-text;
- -webkit-font-smoothing: antialiased;
- -moz-osx-font-smoothing: grayscale;
-}
-
-a {
- color: contrast-color($page-bg, $core-text);
-
- &:link,
- &:visited {
- color: contrast-color($page-bg, $core-text);
- }
-
- &:hover,
- &:active {
- color: amplify(contrast-color($page-bg, $core-text), 5%);
- }
-}
-
-#body-inner {
- a {
- text-decoration: underline;
- text-decoration-style: dotted;
-
- &:hover {
- text-decoration-style: solid;
- }
- }
-}
-
-pre {
- position: relative;
-}
-
-.bg {
- background: #fff;
- border: 1px solid $body-border;
-}
-
-b,
-strong {
- font-weight: $font-weight-bold
-}
-
-.default-animation {
- @include transition(all 0.5s ease);
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/theme/_custom.scss b/themes/learn2-git-sync/scss/theme/_custom.scss
deleted file mode 100644
index 438c0ad..0000000
--- a/themes/learn2-git-sync/scss/theme/_custom.scss
+++ /dev/null
@@ -1,253 +0,0 @@
-@charset "UTF-8";
-
-// Your custom SCSS should be written here...
-
-//Fluidbox fixes
-.lightbox-active {
- #body {
- overflow: visible;
-
- .padding {
- overflow: visible;
- }
- }
-}
-
-//Github
-#github-contrib {
- i {
- vertical-align: middle;
- }
-}
-
-//Featherlight fixes
-.featherlight img {
- margin: 0 !important;
-}
-
-//Lifecycle
-.lifecycle {
-
- #body-inner {
-
- ul {
- list-style: none;
- margin: 0;
- padding: 2rem 0 0;
- position: relative;
- }
-
- ol {
- margin: 1rem 0 1rem 0;
- padding: 2rem;
- position: relative;
-
- li {
- margin-left: 1rem;
- }
-
- strong {
- text-decoration: underline;
- }
-
- ol {
- margin-left: -1rem;
- }
-
- }
-
- h3[class*='level'] {
- font-size: 20px;
- position: absolute;
- margin: 0;
- padding: 4px 10px;
- right: 0;
- z-index: 1000;
- color: #fff;
- background: $turquoise;
- }
-
- ol h3 {
- margin-top: 1rem !important;
- right: 2rem !important;
- }
-
- .level-1+ol {
- background: lighten($turquoise, 56%);
- border: 4px solid $turquoise;
- color: $green_sea;
-
- h3 {
- background: $emerald;
-
- }
- }
-
- .level-2+ol {
- background: lighten($emerald, 49%);
- border: 4px solid $emerald;
- color: $nephritis;
-
- h3 {
- background: $peter_river;
- }
- }
-
- .level-3+ol {
- background: lighten($peter_river, 44%);
- border: 4px solid $peter_river;
- color: $belize_hole;
-
- h3 {
- background: $wet_asphalt;
- }
- }
-
- .level-4+ol {
- background: lighten($wet_asphalt, 63%);
- border: 4px solid $wet_asphalt;
- color: $midnight_blue;
-
- h3 {
- background: $wet_asphalt;
- }
- }
- }
-}
-
-#top-bar {
- background: $navbar-bg;
- border-radius: 2px;
- margin: 0rem -1rem 2rem;
- padding: 0 1rem;
- height: 0;
- min-height: 3rem;
- color: diminish(maximize-color-contrast($navbar-bg), 20%);
-
- a {
- color: diminish(maximize-color-contrast($navbar-bg), 10%);
-
- &:hover {
- color: diminish(maximize-color-contrast($navbar-bg), 5%);
- }
- }
-}
-
-// Github link
-#top-github-link {
-
- @extend %vertical-align;
- position: relative;
- z-index: 1;
-
- float: right;
- display: block;
-}
-
-// Breadcrumbs
-#body #breadcrumbs {
-
- @extend %vertical-align;
-
- height: auto;
- display: block;
- margin-bottom: 0;
- padding-left: 0;
-
- line-height: 1.4;
-
- span {
- padding: 0 0.1rem;
- }
-}
-
-// Media Query stuff
-@include breakpoint(desktop-only) {
- #sidebar {
- width: $sidebar-width-smaller;
- }
-
- #body {
- margin-left: $sidebar-width-smaller;
- }
-}
-
-@include breakpoint(mobile-only) {
- #sidebar {
- width: $sidebar-width-smaller;
- left: - $sidebar-width-smaller;
- }
-
- #body {
- margin-left: 0;
- width: 100%;
- }
-
- .sidebar-hidden {
- overflow: hidden;
-
- #sidebar {
- left: 0;
- }
-
- #body {
- margin-left: $sidebar-width-smaller;
-
- overflow: hidden;
- }
-
- #overlay {
- position: absolute;
- left: 0;
- right: 0;
- top: 0;
- bottom: 0;
- z-index: 10;
- background: rgba(255, 255, 255, .5);
- cursor: pointer;
- }
- }
-}
-
-// clipboard
-.copy-to-clipboard {
- background-image: url(../images/clippy.svg);
- background-position: 50% 50%;
- background-size: 16px 16px;
- background-repeat: no-repeat;
- width: 27px;
- height: $core-font-size + (.2rem * 2);
- top: -1px;
- display: inline-block;
- vertical-align: middle;
- position: relative;
- color: darken($core-text, 10%);
- background-color: $code-bg;
- margin-left: -.2rem;
- cursor: pointer;
- border-radius: 0 2px 2px 0;
-
- &:hover {
- background-color: darken($code-bg, 5%);
- }
-
- pre & {
- position: absolute;
- right: 4px;
- top: 4px;
- background-color: #eee;
- border-color: #ddd;
- border-radius: 2px;
-
- &:hover {
- background-color: #d9d9d9;
- }
- }
-}
-
-// Utils
-.parent-element {
- -webkit-transform-style: preserve-3d;
- -moz-transform-style: preserve-3d;
- transform-style: preserve-3d;
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/theme/_fonts.scss b/themes/learn2-git-sync/scss/theme/_fonts.scss
deleted file mode 100644
index ad04c23..0000000
--- a/themes/learn2-git-sync/scss/theme/_fonts.scss
+++ /dev/null
@@ -1,8 +0,0 @@
-// Import Google Web Fonts
-@import url(//fonts.googleapis.com/css?family=Montserrat:400|Muli:300,400|Inconsolata);
-
-$font-family-default: "Muli", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
-$font-family-header: "Montserrat", "Helvetica", "Tahoma", "Geneva", "Arial", sans-serif;
-$font-family-mono: "Inconsolata", monospace;
-$font-family-serif: "Georgia", "Times", "Times New Roman", serif;
-
diff --git a/themes/learn2-git-sync/scss/theme/_forms.scss b/themes/learn2-git-sync/scss/theme/_forms.scss
deleted file mode 100644
index 8339109..0000000
--- a/themes/learn2-git-sync/scss/theme/_forms.scss
+++ /dev/null
@@ -1,22 +0,0 @@
-fieldset {
- border: 1px solid $core-border-color;
-}
-
-textarea, #{$all-text-inputs}, select[multiple=multiple] {
- background-color: white;
- border: 1px solid $core-border-color;
- box-shadow: $core-box-shadow;
-
- &:hover {
- border-color: $core-border-color-hover;
- }
-
- &:focus {
- border-color: $core-border-color-focus;
- box-shadow: $core-box-shadow-focus;
- }
-}
-
-label {
- @extend strong;
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/theme/_header.scss b/themes/learn2-git-sync/scss/theme/_header.scss
deleted file mode 100644
index 8a1cc19..0000000
--- a/themes/learn2-git-sync/scss/theme/_header.scss
+++ /dev/null
@@ -1,66 +0,0 @@
-#header {
- background: $header-bg;
- color: contrast-color($header-bg, $white);
- text-align: center;
-
- padding: 1rem;
-
- a {
- display: inline-block;
- }
-
- #logo-svg {
- @extend .default-animation;
- width: $logo-width;
- height: $logo-height;
-
- path {
- @extend .default-animation;
- fill: $white;
- }
- }
-}
-
-.searchbox {
- margin-top: 0.5rem;
- position: relative;
-
- border: 1px solid diminish($header-bg, 5%);
- background: amplify($header-bg, 5%);
- border-radius: 4px;
-
- label {
- color: rgba($white, 0.8);
- position: absolute;
- left: 10px;
- top: 3px;
- }
-
- span {
- color: rgba($white, 0.6);
- position: absolute;
- right: 10px;
- top: 3px;
- cursor: pointer;
-
- &:hover {
- color: rgba($white, 0.9);
- }
- }
-
- input {
- display: inline-block;
- color: contrast-color(amplify($header-bg, 5%), $white);
- width: 100%;
- height: 30px;
- background: transparent;
- border: 0;
- padding: 0 25px 0 30px;
- margin: 0;
- font-weight: $font-weight-regular;
-
- @include placeholder {
- color: contrast-color(diminish($header-bg, 5%), $white);
- }
- }
-}
diff --git a/themes/learn2-git-sync/scss/theme/_main.scss b/themes/learn2-git-sync/scss/theme/_main.scss
deleted file mode 100644
index b6d189a..0000000
--- a/themes/learn2-git-sync/scss/theme/_main.scss
+++ /dev/null
@@ -1,152 +0,0 @@
-#main {
- background: $main-bg;
- margin: 0 0 $content-margin + $content-padding 0;
-}
-
-#body {
- img, .video-container {
- margin: 3rem auto;
- display: block;
- text-align: center;
-
- &.border {
- border: 2px solid #e6e6e6 !important;
- padding: 2px;
- }
-
- &.shadow {
- box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1);
- }
- }
-
- @extend .default-animation;
- position: relative;
- margin-left: $sidebar-width;
- min-height: 100%;
-
- .bordered {
- border: 1px solid #ccc;
- }
-
- .padding {
- @extend .default-animation;
- padding: 3rem ($body-margin + 2rem);
-
- @include breakpoint(desktop-only) {
- position: static;
- padding: 15px ($body-margin - 1rem);
- }
-
- @include breakpoint(mobile-only) {
- padding: 5px 1rem;
- }
- }
-
- h1 + hr {
- margin-top: -1.7rem;
- margin-bottom: 3rem;
- }
-
- #navigation {
-
- @include breakpoint(desktop-only) {
- position: static;
- margin-right: 0 !important;
- width: 100%;
- display: table;
- }
- }
-
- .nav {
- @extend .default-animation;
- position: fixed;
- top: 0;
- bottom: 0;
- width: $body-margin;
- font-size: 0;
- height: 100%;
- cursor: pointer;
- display: table;
- text-align: center;
- > i {
- display: table-cell;
- vertical-align: middle;
- text-align: center;
- }
-
- @include breakpoint(desktop-only) {
- display: table-cell;
- position: static;
- top: auto;
- width: 50%;
- text-align: center;
- height: 100px;
- line-height: 100px;
- padding-top: 0;
- > i {
- display: inline-block;
- }
- }
-
- i {
- font-size: 50px;
- }
-
- &:hover {
- background: $navbar-bg;
- }
-
- &.nav-pref {
- left: 0;
- }
-
- &.nav-next {
- right: 0;
- }
- }
-}
-
-#body-inner {
- margin-bottom: 5rem;
-}
-
-// Chapter title
-#chapter {
-
- display: flex;
- align-items: center;
- justify-content: center;
- height: 100%;
- padding: 2rem 0;
-
- #body-inner {
- padding-bottom: 3rem;
- max-width: 80%;
- }
-
- h3 {
- font-family: $font-family-default;
- font-weight: $font-weight-regular;
- text-align: center;
- }
-
- h1 {
- font-size: 5rem;
- border-bottom: 4px solid $rule-color;
- }
-
- p {
- text-align: center;
- font-size: 1.2rem;
- }
-}
-
-#footer {
- padding: 3rem 1rem;
- color: darken($sidebar-link, 10%);
- font-size: 13px;
-
- p {
- margin: 0;
- }
-}
diff --git a/themes/learn2-git-sync/scss/theme/_nav.scss b/themes/learn2-git-sync/scss/theme/_nav.scss
deleted file mode 100644
index 6a85ab3..0000000
--- a/themes/learn2-git-sync/scss/theme/_nav.scss
+++ /dev/null
@@ -1,237 +0,0 @@
-#sidebar-toggle {
- display: none;
-
- @include breakpoint(mobile-only) {
- display: inline-block;
- }
-
-}
-
-#sidebar {
- @extend .default-animation;
- background-color: $sidebar-bg;
- position: fixed;
- top: 0;
- width: $sidebar-width;
- bottom: 0;
- left: 0;
- font-weight: $font-weight-medium;
- font-size: 15px;
- border-right: 1px solid diminish($sidebar-bg, 7%);
-
- a,
- i {
- color: $sidebar-link;
- &:hover {
- color: amplify(contrast-color($sidebar-bg, $sidebar-link), 5%);
- }
- &.subtitle {
- color: rgba($sidebar-link, 0.6);
- }
- }
-
- hr {
- border-bottom: 1px solid amplify($sidebar-bg, 3%);
- }
-
- a.padding {
- padding: 0 1rem;
- }
-
- h5 {
- margin: 2rem 0 0;
- position: relative;
- line-height: 2;
-
- a {
- display: block;
- margin-left: 0;
- margin-right: 0;
- padding-left: 1rem;
- padding-right: 1rem;
- }
-
- i {
- color: rgba($sidebar-link, 0.6);
- position: absolute;
- right: 0.6rem;
- top: 0.7rem;
- font-size: 80%;
- }
-
- &.parent {
- a {
- background: amplify($sidebar-bg, 7%);
- color: amplify($sidebar-link, 5%) !important;
- }
- }
-
- &.active {
- a {
- background: $white;
- color: $core-text !important;
- }
-
- i {
- color: $core-text !important;
- }
- }
-
-
- }
-
- h5 + ul.topics {
- display: none;
- margin-top: 0;
- }
-
- h5.parent, h5.active {
- + ul.topics {
- display: block;
- }
- }
-
-
- ul {
- @extend .default-animation;
- list-style: none;
- padding: 0;
- margin: 0;
-
- &.searched {
- a {
- color: amplify($sidebar-link, 20%);
- }
-
- .search-match {
- a {
- color: amplify($sidebar-link, 10%);
- &:hover {
- color: amplify($sidebar-link, 20%);
- }
- }
- }
- }
-
- &.topics {
- margin: 0 1rem;
-
- &.searched {
- ul {
- display: block;
- }
- }
-
- ul {
- display: none;
- padding-bottom: 1rem;
-
- ul {
- padding-bottom: 0;
- }
- }
-
- li.parent ul, > li.active ul {
- display: block;
- }
-
- > li {
- > a {
- line-height: 2rem;
- font-size: 1.1rem;
-
- b {
- color: $sidebar-link;
- font-weight: normal;
- }
-
- .fa {
- margin-top: 9px;
- }
- }
-
- &.parent, &.active {
- background: amplify($sidebar-bg, 5%);
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem;
- > a {
- b {
- color: $sidebar-link;
- }
- }
- }
- }
- }
-
- li.active > a {
- background: $white;
- color: $core-text !important;
- margin-left: -1rem;
- margin-right: -1rem;
- padding-left: 1rem;
- padding-right: 1rem;
- b {
- color: $core-text !important;
- }
- }
-
- li {
- padding: 0;
- &.visited + span {
- margin-right: 16px;
- }
- a {
- display: block;
- padding: 2px 0;
- span {
- text-overflow: ellipsis;
- overflow: hidden;
- white-space: nowrap;
- display: block;
- }
- }
- > a {
- padding: 4px 0;
- }
-
- .fa {
- display: none;
- float: right;
- font-size: 13px;
- min-width: 16px;
- margin: 4px 0 0 0;
- text-align: right;
- }
-
- &.visited {
- > a .read-icon {
- color: $core-accent;
- display: inline;
- }
- }
-
- li {
- padding-left: 1rem;
- text-indent: 0.2rem;
- }
- }
-
- }
-}
-#body {
- #navigation {
- .nav {
- .fa {
- color: amplify(contrast-color($page-bg, $sidebar-bg), 15%);
- &:active,
- &:focus,
- &:hover,
- &:visited {
- color: contrast-color($page-bg, $sidebar-bg);
- }
- }
- }
- }
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/theme/_scrollbar.scss b/themes/learn2-git-sync/scss/theme/_scrollbar.scss
deleted file mode 100644
index 00c9cfc..0000000
--- a/themes/learn2-git-sync/scss/theme/_scrollbar.scss
+++ /dev/null
@@ -1,139 +0,0 @@
-/*************** SCROLLBAR BASE CSS ***************/
-
-.highlightable {
- padding: 25px 0 15px;
-}
-
-.scroll-wrapper {
- overflow: hidden !important;
- padding: 0 !important;
- position: relative;
-}
-
-.scroll-wrapper > .scroll-content {
- border: none !important;
- box-sizing: content-box !important;
- height: auto;
- left: 0;
- margin: 0;
- max-height: none;
- max-width: none !important;
- overflow: scroll !important;
- padding: 0;
- position: relative !important;
- top: 0;
- width: auto !important;
-}
-
-.scroll-wrapper > .scroll-content::-webkit-scrollbar {
- height: 0;
- width: 0;
-}
-
-.scroll-element {
- display: none;
-}
-.scroll-element, .scroll-element div {
- box-sizing: content-box;
-}
-
-.scroll-element.scroll-x.scroll-scrollx_visible,
-.scroll-element.scroll-y.scroll-scrolly_visible {
- display: block;
-}
-
-.scroll-element .scroll-bar,
-.scroll-element .scroll-arrow {
- cursor: default;
-}
-
-.scroll-textarea {
-
-}
-.scroll-textarea > .scroll-content {
- overflow: hidden !important;
-}
-.scroll-textarea > .scroll-content > textarea {
- border: none !important;
- box-sizing: border-box;
- height: 100% !important;
- margin: 0;
- max-height: none !important;
- max-width: none !important;
- overflow: scroll !important;
- outline: none;
- padding: 2px;
- position: relative !important;
- top: 0;
- width: 100% !important;
-}
-.scroll-textarea > .scroll-content > textarea::-webkit-scrollbar {
- height: 0;
- width: 0;
-}
-
-
-
-
-/*************** SIMPLE INNER SCROLLBAR ***************/
-
-.scrollbar-inner > .scroll-element,
-.scrollbar-inner > .scroll-element div
-{
- border: none;
- margin: 0;
- padding: 0;
- position: absolute;
- z-index: 10;
-}
-
-.scrollbar-inner > .scroll-element div {
- display: block;
- height: 100%;
- left: 0;
- top: 0;
- width: 100%;
-}
-
-.scrollbar-inner > .scroll-element.scroll-x {
- bottom: 2px;
- height: 8px;
- left: 0;
- width: 100%;
-}
-
-.scrollbar-inner > .scroll-element.scroll-y {
- height: 100%;
- right: 2px;
- top: 0;
- width: 8px;
-}
-
-.scrollbar-inner > .scroll-element .scroll-element_outer {
- overflow: hidden;
-}
-
-.scrollbar-inner > .scroll-element .scroll-element_outer,
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -webkit-border-radius: 8px;
- -moz-border-radius: 8px;
- border-radius: 8px;
-}
-
-.scrollbar-inner > .scroll-element .scroll-element_track,
-.scrollbar-inner > .scroll-element .scroll-bar {
- -ms-filter:"progid:DXImageTransform.Microsoft.Alpha(Opacity=30)";
- filter: alpha(opacity=30);
- opacity: 0.3;
-}
-
-
-/* update scrollbar offset if both scrolls are visible */
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_track { left: -12px; }
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_track { top: -12px; }
-
-
-.scrollbar-inner > .scroll-element.scroll-x.scroll-scrolly_visible .scroll-element_size { left: -12px; }
-.scrollbar-inner > .scroll-element.scroll-y.scroll-scrollx_visible .scroll-element_size { top: -12px; }
diff --git a/themes/learn2-git-sync/scss/theme/_tables.scss b/themes/learn2-git-sync/scss/theme/_tables.scss
deleted file mode 100644
index 923eb7f..0000000
--- a/themes/learn2-git-sync/scss/theme/_tables.scss
+++ /dev/null
@@ -1,15 +0,0 @@
-table {
- border: 1px solid lighten($core-border-color,5%);
- table-layout: auto;
-}
-
-th {
- @extend strong;
- background: lighten($core-border-color,10%);
- padding: 0.5rem;
-}
-
-td {
- padding: 0.5rem;
- border: 1px solid lighten($core-border-color,5%);
-}
diff --git a/themes/learn2-git-sync/scss/theme/_tooltips.scss b/themes/learn2-git-sync/scss/theme/_tooltips.scss
deleted file mode 100644
index 9ec8ef8..0000000
--- a/themes/learn2-git-sync/scss/theme/_tooltips.scss
+++ /dev/null
@@ -1,157 +0,0 @@
-$multiline-max-width: 250px;
-$tooltip-background-color: rgba(0, 0, 0, 0.8);
-$tooltip-text-color: #fff;
-
-.tooltipped {
- position: relative;
-}
-
-// This is the tooltip bubble
-.tooltipped:after {
- position: absolute;
- z-index: 1000000;
- display: none;
- padding: 5px 8px;
- font: normal normal 11px/1.5 $font-family-default;
- color: $tooltip-text-color;
- text-align: center;
- text-decoration: none;
- text-shadow: none;
- text-transform: none;
- letter-spacing: normal;
- word-wrap: break-word;
- white-space: pre;
- pointer-events: none;
- content: attr(aria-label);
- background: $tooltip-background-color;
- border-radius: 3px;
- -webkit-font-smoothing: subpixel-antialiased;
-}
-
-// This is the tooltip arrow
-.tooltipped:before {
- position: absolute;
- z-index: 1000001;
- display: none;
- width: 0;
- height: 0;
- color: $tooltip-background-color;
- pointer-events: none;
- content: "";
- border: 5px solid transparent;
-}
-
-// This will indicate when we'll activate the tooltip
-.tooltipped:hover,
-.tooltipped:active,
-.tooltipped:focus {
- &:before,
- &:after {
- display: inline-block;
- text-decoration: none;
- }
-}
-
-// Tooltipped south
-.tooltipped-s,
-.tooltipped-se,
-.tooltipped-sw {
- &:after {
- top: 100%;
- right: 50%;
- margin-top: 5px;
- }
-
- &:before {
- top: auto;
- right: 50%;
- bottom: -5px;
- margin-right: -5px;
- border-bottom-color: $tooltip-background-color;
- }
-}
-
-.tooltipped-se {
- &:after {
- right: auto;
- left: 50%;
- margin-left: -15px;
- }
-}
-
-.tooltipped-sw:after {
- margin-right: -15px;
-}
-
-// Tooltips above the object
-.tooltipped-n,
-.tooltipped-ne,
-.tooltipped-nw {
- &:after {
- right: 50%;
- bottom: 100%;
- margin-bottom: 5px;
- }
-
- &:before {
- top: -5px;
- right: 50%;
- bottom: auto;
- margin-right: -5px;
- border-top-color: $tooltip-background-color;
- }
-}
-
-.tooltipped-ne {
- &:after {
- right: auto;
- left: 50%;
- margin-left: -15px;
- }
-}
-
-.tooltipped-nw:after {
- margin-right: -15px;
-}
-
-// Move the tooltip body to the center of the object.
-.tooltipped-s:after,
-.tooltipped-n:after {
- transform: translateX(50%);
-}
-
-// Tooltipped to the left
-.tooltipped-w {
- &:after {
- right: 100%;
- bottom: 50%;
- margin-right: 5px;
- transform: translateY(50%);
- }
-
- &:before {
- top: 50%;
- bottom: 50%;
- left: -5px;
- margin-top: -5px;
- border-left-color: $tooltip-background-color;
- }
-}
-
-// tooltipped to the right
-.tooltipped-e {
- &:after {
- bottom: 50%;
- left: 100%;
- margin-left: 5px;
- transform: translateY(50%);
- }
-
- &:before {
- top: 50%;
- right: -5px;
- bottom: 50%;
- margin-top: -5px;
- border-right-color: $tooltip-background-color;
- }
-}
diff --git a/themes/learn2-git-sync/scss/theme/_typography.scss b/themes/learn2-git-sync/scss/theme/_typography.scss
deleted file mode 100644
index 0fdc478..0000000
--- a/themes/learn2-git-sync/scss/theme/_typography.scss
+++ /dev/null
@@ -1,181 +0,0 @@
-// Body Base
-body {
- font-family: $font-family-default;
- letter-spacing: -0.03rem;
- font-weight: 400;
-}
-
-// Headings
-h1, h2, h3, h4, h5, h6 {
- font-family: $font-family-header;
- font-weight: 400;
- text-rendering: optimizeLegibility;
- line-height: 150%;
- letter-spacing: -0px;
-}
-
-h1 {
- text-align: center;
- letter-spacing: -3px;
-}
-
-h2 {
- letter-spacing: -2px;
-}
-
-h3 {
- letter-spacing: -1px;
-}
-
-// Blockquote
-blockquote {
- border-left: 10px solid $rule-color;
- p {
- font-size: 1.1rem;
- color: contrast-color($page-bg, #999999);
- }
- cite {
- display: block;
- text-align: right;
- color: contrast-color($page-bg, #666666);
- font-size: 1.2rem;
- }
-}
-
-// NOTES!!!!
-blockquote {
- position: relative;
-}
-
-blockquote blockquote {
- position: static;
-}
-
-blockquote > blockquote > blockquote {
-
- margin: 0;
-
- p {
- padding: 15px;
- display: block;
- font-size: 1rem;
- margin-top: 0rem;
- margin-bottom: 0rem;
- color: #666;
-
- &:first-child {
- &:before {
- position: absolute;
- top: 2px;
- color: $white;
- font-family: FontAwesome;
- content: '';
- left: 10px;
- }
- &:after {
- position: absolute;
- top: 2px;
- color: $white;
- left: 2rem;
- font-weight: bold;
- content: 'Info';
- }
- }
- }
-
- > p {
- // Yellow
- margin-left: -71px;
- border-top: 30px solid #F0B37E;
- background: #FFF2DB;
- }
-
- > blockquote > p {
- // Red
- margin-left: -94px;
- border-top: 30px solid rgba(#D9534F, 0.8);
- background: #FAE2E2;
- &:first-child:after {
- content: 'Warning';
- }
- }
-
- > blockquote > blockquote > p {
- // Blue
- margin-left: -118px;
- border-top: 30px solid #6AB0DE;
- background: #E7F2FA;
- &:first-child:after {
- content: 'Note';
- }
- }
-
- > blockquote > blockquote > blockquote > p {
- // Green
- margin-left: -142px;
- border-top: 30px solid rgba(#5CB85C, 0.8);
- background: #E6F9E6;
- &:first-child:after {
- content: 'Tip';
- }
- }
-
-}
-
-// Inline and Code
-code,
-kbd,
-pre,
-samp {
- font-family: $font-family-mono;
-}
-
-code {
- background: $code-bg;
- color: amplify($code-text, 10%);
- padding: .2rem .4rem;
- border-radius: 3px;
-}
-
-pre {
- padding: 1rem;
- margin: 2rem 0;
- background: $pre-bg;
- border: 1px solid $core-border-color;
- border-radius: 2px;
- line-height: 1.15;
- font-size: 1rem;
-
- code {
- color: $pre-text;
- background: inherit;
- font-size: 1rem;
- }
-}
-
-// Extras
-hr {
- border-bottom: 4px solid $rule-color;
-}
-
-// Page Title
-.page-title {
- margin-top: -25px;
- padding: 25px;
- float: left;
- clear: both;
- background: $core-accent;
- color: $white;
-}
-
-// Anchor links
-#body {
- a.anchor-link { color: #ccc; }
- a.anchor-link:hover { color: $core-accent; }
-}
-
-// Scrollbar
-.scrollbar-inner > .scroll-element .scroll-element_track { background-color: rgba($white, 0.3); }
-.scrollbar-inner > .scroll-element .scroll-bar { background-color: amplify(#A1C4E5, 5%); }
-.scrollbar-inner > .scroll-element:hover .scroll-bar { background-color: #ccc; }
-.scrollbar-inner > .scroll-element.scroll-draggable .scroll-bar { background-color: #ccc; }
diff --git a/themes/learn2-git-sync/scss/theme/modules/_base.scss b/themes/learn2-git-sync/scss/theme/modules/_base.scss
deleted file mode 100644
index 1de274b..0000000
--- a/themes/learn2-git-sync/scss/theme/modules/_base.scss
+++ /dev/null
@@ -1,2 +0,0 @@
-// Buttons
-@import "buttons";
diff --git a/themes/learn2-git-sync/scss/theme/modules/_buttons.scss b/themes/learn2-git-sync/scss/theme/modules/_buttons.scss
deleted file mode 100644
index 84b3db7..0000000
--- a/themes/learn2-git-sync/scss/theme/modules/_buttons.scss
+++ /dev/null
@@ -1,24 +0,0 @@
-%button {
- display: inline-block;
- padding: 7px 12px;
- &:active {
- margin: 2px 0 -2px 0;
- }
-}
-
-@mixin button-color($color) {
- background: $color !important;
- color: maximize-color-contrast($color) !important;
- box-shadow: 0 3px 0 amplify($color, 6%) !important;
- &:hover {
- background: amplify($color, 6%) !important;
- box-shadow: 0 3px 0 amplify($color, 12%) !important;
- color: maximize-color-contrast($color) !important;
- }
- &:active {
- box-shadow: 0 1px 0 amplify($color, 12%) !important;
- }
- i {
- color: maximize-color-contrast($color) !important;
- }
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss b/themes/learn2-git-sync/scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss
deleted file mode 100644
index f946b3b..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/_bourbon-deprecated-upcoming.scss
+++ /dev/null
@@ -1,8 +0,0 @@
-//************************************************************************//
-// These mixins/functions are deprecated
-// They will be removed in the next MAJOR version release
-//************************************************************************//
-@mixin inline-block {
- display: inline-block;
- @warn "inline-block mixin is deprecated and will be removed in the next major version release";
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/_bourbon.scss b/themes/learn2-git-sync/scss/vendor/bourbon/_bourbon.scss
deleted file mode 100644
index 64cb6ea..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/_bourbon.scss
+++ /dev/null
@@ -1,77 +0,0 @@
-// Settings
-@import "settings/prefixer";
-@import "settings/px-to-em";
-
-// Custom Helpers
-@import "helpers/convert-units";
-@import "helpers/gradient-positions-parser";
-@import "helpers/is-num";
-@import "helpers/linear-angle-parser";
-@import "helpers/linear-gradient-parser";
-@import "helpers/linear-positions-parser";
-@import "helpers/linear-side-corner-parser";
-@import "helpers/radial-arg-parser";
-@import "helpers/radial-positions-parser";
-@import "helpers/radial-gradient-parser";
-@import "helpers/render-gradients";
-@import "helpers/shape-size-stripper";
-@import "helpers/str-to-num";
-
-// Custom Functions
-@import "functions/assign";
-@import "functions/color-lightness";
-@import "functions/flex-grid";
-@import "functions/golden-ratio";
-@import "functions/grid-width";
-@import "functions/modular-scale";
-@import "functions/px-to-em";
-@import "functions/px-to-rem";
-@import "functions/strip-units";
-@import "functions/tint-shade";
-@import "functions/transition-property-name";
-@import "functions/unpack";
-
-// CSS3 Mixins
-@import "css3/animation";
-@import "css3/appearance";
-@import "css3/backface-visibility";
-@import "css3/background";
-@import "css3/background-image";
-@import "css3/border-image";
-@import "css3/border-radius";
-@import "css3/box-sizing";
-@import "css3/calc";
-@import "css3/columns";
-@import "css3/filter";
-@import "css3/flex-box";
-@import "css3/font-face";
-@import "css3/hyphens";
-@import "css3/hidpi-media-query";
-@import "css3/image-rendering";
-@import "css3/keyframes";
-@import "css3/linear-gradient";
-@import "css3/perspective";
-@import "css3/radial-gradient";
-@import "css3/transform";
-@import "css3/transition";
-@import "css3/user-select";
-@import "css3/placeholder";
-
-// Addons & other mixins
-@import "addons/button";
-@import "addons/clearfix";
-@import "addons/directional-values";
-@import "addons/ellipsis";
-@import "addons/font-family";
-@import "addons/hide-text";
-@import "addons/html5-input-types";
-@import "addons/position";
-@import "addons/prefixer";
-@import "addons/retina-image";
-@import "addons/size";
-@import "addons/timing-functions";
-@import "addons/triangle";
-@import "addons/word-wrap";
-
-// Soon to be deprecated Mixins
-@import "bourbon-deprecated-upcoming";
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_button.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_button.scss
deleted file mode 100644
index 14a89e4..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_button.scss
+++ /dev/null
@@ -1,374 +0,0 @@
-@mixin button ($style: simple, $base-color: #4294f0, $text-size: inherit, $padding: 7px 18px) {
-
- @if type-of($style) == string and type-of($base-color) == color {
- @include buttonstyle($style, $base-color, $text-size, $padding);
- }
-
- @if type-of($style) == string and type-of($base-color) == number {
- $padding: $text-size;
- $text-size: $base-color;
- $base-color: #4294f0;
-
- @if $padding == inherit {
- $padding: 7px 18px;
- }
-
- @include buttonstyle($style, $base-color, $text-size, $padding);
- }
-
- @if type-of($style) == color and type-of($base-color) == color {
- $base-color: $style;
- $style: simple;
- @include buttonstyle($style, $base-color, $text-size, $padding);
- }
-
- @if type-of($style) == color and type-of($base-color) == number {
- $padding: $text-size;
- $text-size: $base-color;
- $base-color: $style;
- $style: simple;
-
- @if $padding == inherit {
- $padding: 7px 18px;
- }
-
- @include buttonstyle($style, $base-color, $text-size, $padding);
- }
-
- @if type-of($style) == number {
- $padding: $base-color;
- $text-size: $style;
- $base-color: #4294f0;
- $style: simple;
-
- @if $padding == #4294f0 {
- $padding: 7px 18px;
- }
-
- @include buttonstyle($style, $base-color, $text-size, $padding);
- }
-
- &:disabled {
- opacity: 0.5;
- cursor: not-allowed;
- }
-}
-
-
-// Selector Style Button
-//************************************************************************//
-@mixin buttonstyle($type, $b-color, $t-size, $pad) {
- // Grayscale button
- @if $type == simple and $b-color == grayscale($b-color) {
- @include simple($b-color, true, $t-size, $pad);
- }
-
- @if $type == shiny and $b-color == grayscale($b-color) {
- @include shiny($b-color, true, $t-size, $pad);
- }
-
- @if $type == pill and $b-color == grayscale($b-color) {
- @include pill($b-color, true, $t-size, $pad);
- }
-
- @if $type == flat and $b-color == grayscale($b-color) {
- @include flat($b-color, true, $t-size, $pad);
- }
-
- // Colored button
- @if $type == simple {
- @include simple($b-color, false, $t-size, $pad);
- }
-
- @else if $type == shiny {
- @include shiny($b-color, false, $t-size, $pad);
- }
-
- @else if $type == pill {
- @include pill($b-color, false, $t-size, $pad);
- }
-
- @else if $type == flat {
- @include flat($b-color, false, $t-size, $pad);
- }
-}
-
-
-// Simple Button
-//************************************************************************//
-@mixin simple($base-color, $grayscale: false, $textsize: inherit, $padding: 7px 18px) {
- $color: hsl(0, 0, 100%);
- $border: adjust-color($base-color, $saturation: 9%, $lightness: -14%);
- $inset-shadow: adjust-color($base-color, $saturation: -8%, $lightness: 15%);
- $stop-gradient: adjust-color($base-color, $saturation: 9%, $lightness: -11%);
- $text-shadow: adjust-color($base-color, $saturation: 15%, $lightness: -18%);
-
- @if is-light($base-color) {
- $color: hsl(0, 0, 20%);
- $text-shadow: adjust-color($base-color, $saturation: 10%, $lightness: 4%);
- }
-
- @if $grayscale == true {
- $border: grayscale($border);
- $inset-shadow: grayscale($inset-shadow);
- $stop-gradient: grayscale($stop-gradient);
- $text-shadow: grayscale($text-shadow);
- }
-
- border: 1px solid $border;
- border-radius: 3px;
- box-shadow: inset 0 1px 0 0 $inset-shadow;
- color: $color;
- display: inline-block;
- font-size: $textsize;
- font-weight: bold;
- @include linear-gradient ($base-color, $stop-gradient);
- padding: $padding;
- text-decoration: none;
- text-shadow: 0 1px 0 $text-shadow;
- background-clip: padding-box;
-
- &:hover:not(:disabled) {
- $base-color-hover: adjust-color($base-color, $saturation: -4%, $lightness: -5%);
- $inset-shadow-hover: adjust-color($base-color, $saturation: -7%, $lightness: 5%);
- $stop-gradient-hover: adjust-color($base-color, $saturation: 8%, $lightness: -14%);
-
- @if $grayscale == true {
- $base-color-hover: grayscale($base-color-hover);
- $inset-shadow-hover: grayscale($inset-shadow-hover);
- $stop-gradient-hover: grayscale($stop-gradient-hover);
- }
-
- box-shadow: inset 0 1px 0 0 $inset-shadow-hover;
- cursor: pointer;
- @include linear-gradient ($base-color-hover, $stop-gradient-hover);
- }
-
- &:active:not(:disabled),
- &:focus:not(:disabled) {
- $border-active: adjust-color($base-color, $saturation: 9%, $lightness: -14%);
- $inset-shadow-active: adjust-color($base-color, $saturation: 7%, $lightness: -17%);
-
- @if $grayscale == true {
- $border-active: grayscale($border-active);
- $inset-shadow-active: grayscale($inset-shadow-active);
- }
-
- border: 1px solid $border-active;
- box-shadow: inset 0 0 8px 4px $inset-shadow-active, inset 0 0 8px 4px $inset-shadow-active;
- }
-}
-
-
-// Shiny Button
-//************************************************************************//
-@mixin shiny($base-color, $grayscale: false, $textsize: inherit, $padding: 7px 18px) {
- $color: hsl(0, 0, 100%);
- $border: adjust-color($base-color, $red: -117, $green: -111, $blue: -81);
- $border-bottom: adjust-color($base-color, $red: -126, $green: -127, $blue: -122);
- $fourth-stop: adjust-color($base-color, $red: -79, $green: -70, $blue: -46);
- $inset-shadow: adjust-color($base-color, $red: 37, $green: 29, $blue: 12);
- $second-stop: adjust-color($base-color, $red: -56, $green: -50, $blue: -33);
- $text-shadow: adjust-color($base-color, $red: -140, $green: -141, $blue: -114);
- $third-stop: adjust-color($base-color, $red: -86, $green: -75, $blue: -48);
-
- @if is-light($base-color) {
- $color: hsl(0, 0, 20%);
- $text-shadow: adjust-color($base-color, $saturation: 10%, $lightness: 4%);
- }
-
- @if $grayscale == true {
- $border: grayscale($border);
- $border-bottom: grayscale($border-bottom);
- $fourth-stop: grayscale($fourth-stop);
- $inset-shadow: grayscale($inset-shadow);
- $second-stop: grayscale($second-stop);
- $text-shadow: grayscale($text-shadow);
- $third-stop: grayscale($third-stop);
- }
-
- border: 1px solid $border;
- border-bottom: 1px solid $border-bottom;
- border-radius: 5px;
- box-shadow: inset 0 1px 0 0 $inset-shadow;
- color: $color;
- display: inline-block;
- font-size: $textsize;
- font-weight: bold;
- @include linear-gradient(top, $base-color 0%, $second-stop 50%, $third-stop 50%, $fourth-stop 100%);
- padding: $padding;
- text-align: center;
- text-decoration: none;
- text-shadow: 0 -1px 1px $text-shadow;
-
- &:hover:not(:disabled) {
- $first-stop-hover: adjust-color($base-color, $red: -13, $green: -15, $blue: -18);
- $second-stop-hover: adjust-color($base-color, $red: -66, $green: -62, $blue: -51);
- $third-stop-hover: adjust-color($base-color, $red: -93, $green: -85, $blue: -66);
- $fourth-stop-hover: adjust-color($base-color, $red: -86, $green: -80, $blue: -63);
-
- @if $grayscale == true {
- $first-stop-hover: grayscale($first-stop-hover);
- $second-stop-hover: grayscale($second-stop-hover);
- $third-stop-hover: grayscale($third-stop-hover);
- $fourth-stop-hover: grayscale($fourth-stop-hover);
- }
-
- cursor: pointer;
- @include linear-gradient(top, $first-stop-hover 0%,
- $second-stop-hover 50%,
- $third-stop-hover 50%,
- $fourth-stop-hover 100%);
- }
-
- &:active:not(:disabled),
- &:focus:not(:disabled) {
- $inset-shadow-active: adjust-color($base-color, $red: -111, $green: -116, $blue: -122);
-
- @if $grayscale == true {
- $inset-shadow-active: grayscale($inset-shadow-active);
- }
-
- box-shadow: inset 0 0 20px 0 $inset-shadow-active;
- }
-}
-
-
-// Pill Button
-//************************************************************************//
-@mixin pill($base-color, $grayscale: false, $textsize: inherit, $padding: 7px 18px) {
- $color: hsl(0, 0, 100%);
- $border-bottom: adjust-color($base-color, $hue: 8, $saturation: -11%, $lightness: -26%);
- $border-sides: adjust-color($base-color, $hue: 4, $saturation: -21%, $lightness: -21%);
- $border-top: adjust-color($base-color, $hue: -1, $saturation: -30%, $lightness: -15%);
- $inset-shadow: adjust-color($base-color, $hue: -1, $saturation: -1%, $lightness: 7%);
- $stop-gradient: adjust-color($base-color, $hue: 8, $saturation: 14%, $lightness: -10%);
- $text-shadow: adjust-color($base-color, $hue: 5, $saturation: -19%, $lightness: -15%);
-
- @if is-light($base-color) {
- $color: hsl(0, 0, 20%);
- $text-shadow: adjust-color($base-color, $saturation: 10%, $lightness: 4%);
- }
-
- @if $grayscale == true {
- $border-bottom: grayscale($border-bottom);
- $border-sides: grayscale($border-sides);
- $border-top: grayscale($border-top);
- $inset-shadow: grayscale($inset-shadow);
- $stop-gradient: grayscale($stop-gradient);
- $text-shadow: grayscale($text-shadow);
- }
-
- border: 1px solid $border-top;
- border-color: $border-top $border-sides $border-bottom;
- border-radius: 16px;
- box-shadow: inset 0 1px 0 0 $inset-shadow;
- color: $color;
- display: inline-block;
- font-size: $textsize;
- font-weight: normal;
- line-height: 1;
- @include linear-gradient ($base-color, $stop-gradient);
- padding: $padding;
- text-align: center;
- text-decoration: none;
- text-shadow: 0 -1px 1px $text-shadow;
- background-clip: padding-box;
-
- &:hover:not(:disabled) {
- $base-color-hover: adjust-color($base-color, $lightness: -4.5%);
- $border-bottom: adjust-color($base-color, $hue: 8, $saturation: 13.5%, $lightness: -32%);
- $border-sides: adjust-color($base-color, $hue: 4, $saturation: -2%, $lightness: -27%);
- $border-top: adjust-color($base-color, $hue: -1, $saturation: -17%, $lightness: -21%);
- $inset-shadow-hover: adjust-color($base-color, $saturation: -1%, $lightness: 3%);
- $stop-gradient-hover: adjust-color($base-color, $hue: 8, $saturation: -4%, $lightness: -15.5%);
- $text-shadow-hover: adjust-color($base-color, $hue: 5, $saturation: -5%, $lightness: -22%);
-
- @if $grayscale == true {
- $base-color-hover: grayscale($base-color-hover);
- $border-bottom: grayscale($border-bottom);
- $border-sides: grayscale($border-sides);
- $border-top: grayscale($border-top);
- $inset-shadow-hover: grayscale($inset-shadow-hover);
- $stop-gradient-hover: grayscale($stop-gradient-hover);
- $text-shadow-hover: grayscale($text-shadow-hover);
- }
-
- border: 1px solid $border-top;
- border-color: $border-top $border-sides $border-bottom;
- box-shadow: inset 0 1px 0 0 $inset-shadow-hover;
- cursor: pointer;
- @include linear-gradient ($base-color-hover, $stop-gradient-hover);
- text-shadow: 0 -1px 1px $text-shadow-hover;
- background-clip: padding-box;
- }
-
- &:active:not(:disabled),
- &:focus:not(:disabled) {
- $active-color: adjust-color($base-color, $hue: 4, $saturation: -12%, $lightness: -10%);
- $border-active: adjust-color($base-color, $hue: 6, $saturation: -2.5%, $lightness: -30%);
- $border-bottom-active: adjust-color($base-color, $hue: 11, $saturation: 6%, $lightness: -31%);
- $inset-shadow-active: adjust-color($base-color, $hue: 9, $saturation: 2%, $lightness: -21.5%);
- $text-shadow-active: adjust-color($base-color, $hue: 5, $saturation: -12%, $lightness: -21.5%);
-
- @if $grayscale == true {
- $active-color: grayscale($active-color);
- $border-active: grayscale($border-active);
- $border-bottom-active: grayscale($border-bottom-active);
- $inset-shadow-active: grayscale($inset-shadow-active);
- $text-shadow-active: grayscale($text-shadow-active);
- }
-
- background: $active-color;
- border: 1px solid $border-active;
- border-bottom: 1px solid $border-bottom-active;
- box-shadow: inset 0 0 6px 3px $inset-shadow-active;
- text-shadow: 0 -1px 1px $text-shadow-active;
- }
-}
-
-
-
-// Flat Button
-//************************************************************************//
-@mixin flat($base-color, $grayscale: false, $textsize: inherit, $padding: 7px 18px) {
- $color: hsl(0, 0, 100%);
-
- @if is-light($base-color) {
- $color: hsl(0, 0, 20%);
- }
-
- background-color: $base-color;
- border-radius: 3px;
- border: none;
- color: $color;
- display: inline-block;
- font-size: inherit;
- font-weight: bold;
- padding: 7px 18px;
- text-decoration: none;
- background-clip: padding-box;
-
- &:hover:not(:disabled){
- $base-color-hover: adjust-color($base-color, $saturation: 4%, $lightness: 5%);
-
- @if $grayscale == true {
- $base-color-hover: grayscale($base-color-hover);
- }
-
- background-color: $base-color-hover;
- cursor: pointer;
- }
-
- &:active:not(:disabled),
- &:focus:not(:disabled) {
- $base-color-active: adjust-color($base-color, $saturation: -4%, $lightness: -5%);
-
- @if $grayscale == true {
- $base-color-active: grayscale($base-color-active);
- }
-
- background-color: $base-color-active;
- cursor: pointer;
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_clearfix.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_clearfix.scss
deleted file mode 100644
index 783cfbc..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_clearfix.scss
+++ /dev/null
@@ -1,23 +0,0 @@
-// Modern micro clearfix provides an easy way to contain floats without adding additional markup.
-//
-// Example usage:
-//
-// // Contain all floats within .wrapper
-// .wrapper {
-// @include clearfix;
-// .content,
-// .sidebar {
-// float : left;
-// }
-// }
-
-@mixin clearfix {
- &:after {
- content:"";
- display:table;
- clear:both;
- }
-}
-
-// Acknowledgements
-// Beat *that* clearfix: [Thierry Koblentz](http://www.css-101.org/articles/clearfix/latest-new-clearfix-so-far.php)
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_directional-values.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_directional-values.scss
deleted file mode 100644
index 742f103..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_directional-values.scss
+++ /dev/null
@@ -1,111 +0,0 @@
-// directional-property mixins are shorthands
-// for writing properties like the following
-//
-// @include margin(null 0 10px);
-// ------
-// margin-right: 0;
-// margin-bottom: 10px;
-// margin-left: 0;
-//
-// - or -
-//
-// @include border-style(dotted null);
-// ------
-// border-top-style: dotted;
-// border-bottom-style: dotted;
-//
-// ------
-//
-// Note: You can also use false instead of null
-
-@function collapse-directionals($vals) {
- $output: null;
-
- $A: nth( $vals, 1 );
- $B: if( length($vals) < 2, $A, nth($vals, 2));
- $C: if( length($vals) < 3, $A, nth($vals, 3));
- $D: if( length($vals) < 2, $A, nth($vals, if( length($vals) < 4, 2, 4) ));
-
- @if $A == 0 { $A: 0 }
- @if $B == 0 { $B: 0 }
- @if $C == 0 { $C: 0 }
- @if $D == 0 { $D: 0 }
-
- @if $A == $B and $A == $C and $A == $D { $output: $A }
- @else if $A == $C and $B == $D { $output: $A $B }
- @else if $B == $D { $output: $A $B $C }
- @else { $output: $A $B $C $D }
-
- @return $output;
-}
-
-@function contains-falsy($list) {
- @each $item in $list {
- @if not $item {
- @return true;
- }
- }
-
- @return false;
-}
-
-@mixin directional-property($pre, $suf, $vals) {
- // Property Names
- $top: $pre + "-top" + if($suf, "-#{$suf}", "");
- $bottom: $pre + "-bottom" + if($suf, "-#{$suf}", "");
- $left: $pre + "-left" + if($suf, "-#{$suf}", "");
- $right: $pre + "-right" + if($suf, "-#{$suf}", "");
- $all: $pre + if($suf, "-#{$suf}", "");
-
- $vals: collapse-directionals($vals);
-
- @if contains-falsy($vals) {
- @if nth($vals, 1) { #{$top}: nth($vals, 1); }
-
- @if length($vals) == 1 {
- @if nth($vals, 1) { #{$right}: nth($vals, 1); }
- } @else {
- @if nth($vals, 2) { #{$right}: nth($vals, 2); }
- }
-
- // prop: top/bottom right/left
- @if length($vals) == 2 {
- @if nth($vals, 1) { #{$bottom}: nth($vals, 1); }
- @if nth($vals, 2) { #{$left}: nth($vals, 2); }
-
- // prop: top right/left bottom
- } @else if length($vals) == 3 {
- @if nth($vals, 3) { #{$bottom}: nth($vals, 3); }
- @if nth($vals, 2) { #{$left}: nth($vals, 2); }
-
- // prop: top right bottom left
- } @else if length($vals) == 4 {
- @if nth($vals, 3) { #{$bottom}: nth($vals, 3); }
- @if nth($vals, 4) { #{$left}: nth($vals, 4); }
- }
-
- // prop: top/right/bottom/left
- } @else {
- #{$all}: $vals;
- }
-}
-
-@mixin margin($vals...) {
- @include directional-property(margin, false, $vals...);
-}
-
-@mixin padding($vals...) {
- @include directional-property(padding, false, $vals...);
-}
-
-@mixin border-style($vals...) {
- @include directional-property(border, style, $vals...);
-}
-
-@mixin border-color($vals...) {
- @include directional-property(border, color, $vals...);
-}
-
-@mixin border-width($vals...) {
- @include directional-property(border, width, $vals...);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_ellipsis.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_ellipsis.scss
deleted file mode 100644
index a8ea2a4..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_ellipsis.scss
+++ /dev/null
@@ -1,7 +0,0 @@
-@mixin ellipsis($width: 100%) {
- display: inline-block;
- max-width: $width;
- overflow: hidden;
- text-overflow: ellipsis;
- white-space: nowrap;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_font-family.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_font-family.scss
deleted file mode 100644
index 31f5d9c..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_font-family.scss
+++ /dev/null
@@ -1,5 +0,0 @@
-$georgia: Georgia, Cambria, "Times New Roman", Times, serif;
-$helvetica: "Helvetica Neue", Helvetica, Roboto, Arial, sans-serif;
-$lucida-grande: "Lucida Grande", Tahoma, Verdana, Arial, sans-serif;
-$monospace: "Bitstream Vera Sans Mono", Consolas, Courier, monospace;
-$verdana: Verdana, Geneva, sans-serif;
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_hide-text.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_hide-text.scss
deleted file mode 100644
index fc79438..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_hide-text.scss
+++ /dev/null
@@ -1,10 +0,0 @@
-@mixin hide-text {
- overflow: hidden;
-
- &:before {
- content: "";
- display: block;
- width: 0;
- height: 100%;
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_html5-input-types.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_html5-input-types.scss
deleted file mode 100644
index 9e9324a..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_html5-input-types.scss
+++ /dev/null
@@ -1,86 +0,0 @@
-//************************************************************************//
-// Generate a variable ($all-text-inputs) with a list of all html5
-// input types that have a text-based input, excluding textarea.
-// http://diveintohtml5.org/forms.html
-//************************************************************************//
-$inputs-list: 'input[type="email"]',
- 'input[type="number"]',
- 'input[type="password"]',
- 'input[type="search"]',
- 'input[type="tel"]',
- 'input[type="text"]',
- 'input[type="url"]',
-
- // Webkit & Gecko may change the display of these in the future
- 'input[type="color"]',
- 'input[type="date"]',
- 'input[type="datetime"]',
- 'input[type="datetime-local"]',
- 'input[type="month"]',
- 'input[type="time"]',
- 'input[type="week"]';
-
-// Bare inputs
-//************************************************************************//
-$all-text-inputs: assign-inputs($inputs-list);
-
-// Hover Pseudo-class
-//************************************************************************//
-$all-text-inputs-hover: assign-inputs($inputs-list, hover);
-
-// Focus Pseudo-class
-//************************************************************************//
-$all-text-inputs-focus: assign-inputs($inputs-list, focus);
-
-
-
-// You must use interpolation on the variable:
-// #{$all-text-inputs}
-// #{$all-text-inputs-hover}
-// #{$all-text-inputs-focus}
-
-// Example
-//************************************************************************//
-// #{$all-text-inputs}, textarea {
-// border: 1px solid red;
-// }
-
-
-
-//************************************************************************//
-// Generate a variable ($all-button-inputs) with a list of all html5
-// input types that have a button-based input, excluding button.
-//************************************************************************//
-$inputs-button-list: 'input[type="button"]',
- 'input[type="reset"]',
- 'input[type="submit"]';
-
-// Bare inputs
-//************************************************************************//
-$all-button-inputs: assign-inputs($inputs-button-list);
-
-// Hover Pseudo-class
-//************************************************************************//
-$all-button-inputs-hover: assign-inputs($inputs-button-list, hover);
-
-// Focus Pseudo-class
-//************************************************************************//
-$all-button-inputs-focus: assign-inputs($inputs-button-list, focus);
-
-// Active Pseudo-class
-//************************************************************************//
-$all-button-inputs-active: assign-inputs($inputs-button-list, active);
-
-
-
-// You must use interpolation on the variable:
-// #{$all-button-inputs}
-// #{$all-button-inputs-hover}
-// #{$all-button-inputs-focus}
-// #{$all-button-inputs-active}
-
-// Example
-//************************************************************************//
-// #{$all-button-inputs}, button {
-// border: 1px solid red;
-// }
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_position.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_position.scss
deleted file mode 100644
index 7de7518..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_position.scss
+++ /dev/null
@@ -1,32 +0,0 @@
-@mixin position ($position: relative, $coordinates: null null null null) {
-
- @if type-of($position) == list {
- $coordinates: $position;
- $position: relative;
- }
-
- $coordinates: unpack($coordinates);
-
- $top: nth($coordinates, 1);
- $right: nth($coordinates, 2);
- $bottom: nth($coordinates, 3);
- $left: nth($coordinates, 4);
-
- position: $position;
-
- @if ($top and $top == auto) or (type-of($top) == number) {
- top: $top;
- }
-
- @if ($right and $right == auto) or (type-of($right) == number) {
- right: $right;
- }
-
- @if ($bottom and $bottom == auto) or (type-of($bottom) == number) {
- bottom: $bottom;
- }
-
- @if ($left and $left == auto) or (type-of($left) == number) {
- left: $left;
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_prefixer.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_prefixer.scss
deleted file mode 100644
index c32f502..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_prefixer.scss
+++ /dev/null
@@ -1,45 +0,0 @@
-//************************************************************************//
-// Example: @include prefixer(border-radius, $radii, webkit ms spec);
-//************************************************************************//
-// Variables located in /settings/_prefixer.scss
-
-@mixin prefixer ($property, $value, $prefixes) {
- @each $prefix in $prefixes {
- @if $prefix == webkit {
- @if $prefix-for-webkit {
- -webkit-#{$property}: $value;
- }
- }
- @else if $prefix == moz {
- @if $prefix-for-mozilla {
- -moz-#{$property}: $value;
- }
- }
- @else if $prefix == ms {
- @if $prefix-for-microsoft {
- -ms-#{$property}: $value;
- }
- }
- @else if $prefix == o {
- @if $prefix-for-opera {
- -o-#{$property}: $value;
- }
- }
- @else if $prefix == spec {
- @if $prefix-for-spec {
- #{$property}: $value;
- }
- }
- @else {
- @warn "Unrecognized prefix: #{$prefix}";
- }
- }
-}
-
-@mixin disable-prefix-for-all() {
- $prefix-for-webkit: false !global;
- $prefix-for-mozilla: false !global;
- $prefix-for-microsoft: false !global;
- $prefix-for-opera: false !global;
- $prefix-for-spec: false !global;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_rem.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_rem.scss
deleted file mode 100644
index ddd7022..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_rem.scss
+++ /dev/null
@@ -1,33 +0,0 @@
-@mixin rem($property, $size, $base: $em-base) {
- @if not unitless($base) {
- $base: strip-units($base);
- }
-
- $unitless_values: ();
- @each $num in $size {
- @if not unitless($num) {
- @if unit($num) == "em" {
- $num: $num * $base;
- }
-
- $num: strip-units($num);
- }
-
- $unitless_values: append($unitless_values, $num);
- }
- $size: $unitless_values;
-
- $pixel_values: ();
- $rem_values: ();
- @each $value in $pxval {
- $pixel_value: $value * 1px;
- $pixel_values: append($pixel_values, $pixel_value);
-
- $rem_value: ($value / $base) * 1rem;
- $rem_values: append($rem_values, $rem_value);
- }
-
- #{$property}: $pixel_values;
- #{$property}: $rem_values;
-}
-
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_retina-image.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_retina-image.scss
deleted file mode 100644
index 7931bd1..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_retina-image.scss
+++ /dev/null
@@ -1,31 +0,0 @@
-@mixin retina-image($filename, $background-size, $extension: png, $retina-filename: null, $retina-suffix: _2x, $asset-pipeline: false) {
- @if $asset-pipeline {
- background-image: image-url("#{$filename}.#{$extension}");
- }
- @else {
- background-image: url("#{$filename}.#{$extension}");
- }
-
- @include hidpi {
- @if $asset-pipeline {
- @if $retina-filename {
- background-image: image-url("#{$retina-filename}.#{$extension}");
- }
- @else {
- background-image: image-url("#{$filename}#{$retina-suffix}.#{$extension}");
- }
- }
-
- @else {
- @if $retina-filename {
- background-image: url("#{$retina-filename}.#{$extension}");
- }
- @else {
- background-image: url("#{$filename}#{$retina-suffix}.#{$extension}");
- }
- }
-
- background-size: $background-size;
-
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_size.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_size.scss
deleted file mode 100644
index ac705e2..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_size.scss
+++ /dev/null
@@ -1,16 +0,0 @@
-@mixin size($size) {
- $height: nth($size, 1);
- $width: $height;
-
- @if length($size) > 1 {
- $height: nth($size, 2);
- }
-
- @if $height == auto or (type-of($height) == number and not unitless($height)) {
- height: $height;
- }
-
- @if $width == auto or (type-of($height) == number and not unitless($width)) {
- width: $width;
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_timing-functions.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_timing-functions.scss
deleted file mode 100644
index 51b2410..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_timing-functions.scss
+++ /dev/null
@@ -1,32 +0,0 @@
-// CSS cubic-bezier timing functions. Timing functions courtesy of jquery.easie (github.com/jaukia/easie)
-// Timing functions are the same as demo'ed here: http://jqueryui.com/demos/effect/easing.html
-
-// EASE IN
-$ease-in-quad: cubic-bezier(0.550, 0.085, 0.680, 0.530);
-$ease-in-cubic: cubic-bezier(0.550, 0.055, 0.675, 0.190);
-$ease-in-quart: cubic-bezier(0.895, 0.030, 0.685, 0.220);
-$ease-in-quint: cubic-bezier(0.755, 0.050, 0.855, 0.060);
-$ease-in-sine: cubic-bezier(0.470, 0.000, 0.745, 0.715);
-$ease-in-expo: cubic-bezier(0.950, 0.050, 0.795, 0.035);
-$ease-in-circ: cubic-bezier(0.600, 0.040, 0.980, 0.335);
-$ease-in-back: cubic-bezier(0.600, -0.280, 0.735, 0.045);
-
-// EASE OUT
-$ease-out-quad: cubic-bezier(0.250, 0.460, 0.450, 0.940);
-$ease-out-cubic: cubic-bezier(0.215, 0.610, 0.355, 1.000);
-$ease-out-quart: cubic-bezier(0.165, 0.840, 0.440, 1.000);
-$ease-out-quint: cubic-bezier(0.230, 1.000, 0.320, 1.000);
-$ease-out-sine: cubic-bezier(0.390, 0.575, 0.565, 1.000);
-$ease-out-expo: cubic-bezier(0.190, 1.000, 0.220, 1.000);
-$ease-out-circ: cubic-bezier(0.075, 0.820, 0.165, 1.000);
-$ease-out-back: cubic-bezier(0.175, 0.885, 0.320, 1.275);
-
-// EASE IN OUT
-$ease-in-out-quad: cubic-bezier(0.455, 0.030, 0.515, 0.955);
-$ease-in-out-cubic: cubic-bezier(0.645, 0.045, 0.355, 1.000);
-$ease-in-out-quart: cubic-bezier(0.770, 0.000, 0.175, 1.000);
-$ease-in-out-quint: cubic-bezier(0.860, 0.000, 0.070, 1.000);
-$ease-in-out-sine: cubic-bezier(0.445, 0.050, 0.550, 0.950);
-$ease-in-out-expo: cubic-bezier(1.000, 0.000, 0.000, 1.000);
-$ease-in-out-circ: cubic-bezier(0.785, 0.135, 0.150, 0.860);
-$ease-in-out-back: cubic-bezier(0.680, -0.550, 0.265, 1.550);
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_triangle.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_triangle.scss
deleted file mode 100644
index 573954e..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_triangle.scss
+++ /dev/null
@@ -1,83 +0,0 @@
-@mixin triangle ($size, $color, $direction) {
- height: 0;
- width: 0;
-
- $width: nth($size, 1);
- $height: nth($size, length($size));
-
- $foreground-color: nth($color, 1);
- $background-color: if(length($color) == 2, nth($color, 2), transparent);
-
- @if ($direction == up) or ($direction == down) or ($direction == right) or ($direction == left) {
-
- $width: $width / 2;
- $height: if(length($size) > 1, $height, $height/2);
-
- @if $direction == up {
- border-left: $width solid $background-color;
- border-right: $width solid $background-color;
- border-bottom: $height solid $foreground-color;
-
- } @else if $direction == right {
- border-top: $width solid $background-color;
- border-bottom: $width solid $background-color;
- border-left: $height solid $foreground-color;
-
- } @else if $direction == down {
- border-left: $width solid $background-color;
- border-right: $width solid $background-color;
- border-top: $height solid $foreground-color;
-
- } @else if $direction == left {
- border-top: $width solid $background-color;
- border-bottom: $width solid $background-color;
- border-right: $height solid $foreground-color;
- }
- }
-
- @else if ($direction == up-right) or ($direction == up-left) {
- border-top: $height solid $foreground-color;
-
- @if $direction == up-right {
- border-left: $width solid $background-color;
-
- } @else if $direction == up-left {
- border-right: $width solid $background-color;
- }
- }
-
- @else if ($direction == down-right) or ($direction == down-left) {
- border-bottom: $height solid $foreground-color;
-
- @if $direction == down-right {
- border-left: $width solid $background-color;
-
- } @else if $direction == down-left {
- border-right: $width solid $background-color;
- }
- }
-
- @else if ($direction == inset-up) {
- border-width: $height $width;
- border-style: solid;
- border-color: $background-color $background-color $foreground-color;
- }
-
- @else if ($direction == inset-down) {
- border-width: $height $width;
- border-style: solid;
- border-color: $foreground-color $background-color $background-color;
- }
-
- @else if ($direction == inset-right) {
- border-width: $width $height;
- border-style: solid;
- border-color: $background-color $background-color $background-color $foreground-color;
- }
-
- @else if ($direction == inset-left) {
- border-width: $width $height;
- border-style: solid;
- border-color: $background-color $foreground-color $background-color $background-color;
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_word-wrap.scss b/themes/learn2-git-sync/scss/vendor/bourbon/addons/_word-wrap.scss
deleted file mode 100644
index 9734a59..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/addons/_word-wrap.scss
+++ /dev/null
@@ -1,8 +0,0 @@
-@mixin word-wrap($wrap: break-word) {
- word-wrap: $wrap;
-
- @if $wrap == break-word {
- overflow-wrap: break-word;
- word-break: break-all;
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_animation.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_animation.scss
deleted file mode 100644
index 08c3dbf..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_animation.scss
+++ /dev/null
@@ -1,52 +0,0 @@
-// http://www.w3.org/TR/css3-animations/#the-animation-name-property-
-// Each of these mixins support comma separated lists of values, which allows different transitions for individual properties to be described in a single style rule. Each value in the list corresponds to the value at that same position in the other properties.
-
-// Official animation shorthand property.
-@mixin animation ($animations...) {
- @include prefixer(animation, $animations, webkit moz spec);
-}
-
-// Individual Animation Properties
-@mixin animation-name ($names...) {
- @include prefixer(animation-name, $names, webkit moz spec);
-}
-
-
-@mixin animation-duration ($times...) {
- @include prefixer(animation-duration, $times, webkit moz spec);
-}
-
-
-@mixin animation-timing-function ($motions...) {
-// ease | linear | ease-in | ease-out | ease-in-out
- @include prefixer(animation-timing-function, $motions, webkit moz spec);
-}
-
-
-@mixin animation-iteration-count ($values...) {
-// infinite |
- @include prefixer(animation-iteration-count, $values, webkit moz spec);
-}
-
-
-@mixin animation-direction ($directions...) {
-// normal | alternate
- @include prefixer(animation-direction, $directions, webkit moz spec);
-}
-
-
-@mixin animation-play-state ($states...) {
-// running | paused
- @include prefixer(animation-play-state, $states, webkit moz spec);
-}
-
-
-@mixin animation-delay ($times...) {
- @include prefixer(animation-delay, $times, webkit moz spec);
-}
-
-
-@mixin animation-fill-mode ($modes...) {
-// none | forwards | backwards | both
- @include prefixer(animation-fill-mode, $modes, webkit moz spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_appearance.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_appearance.scss
deleted file mode 100644
index 3eb16e4..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_appearance.scss
+++ /dev/null
@@ -1,3 +0,0 @@
-@mixin appearance ($value) {
- @include prefixer(appearance, $value, webkit moz ms o spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_backface-visibility.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_backface-visibility.scss
deleted file mode 100644
index 1161fe6..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_backface-visibility.scss
+++ /dev/null
@@ -1,6 +0,0 @@
-//************************************************************************//
-// Backface-visibility mixin
-//************************************************************************//
-@mixin backface-visibility($visibility) {
- @include prefixer(backface-visibility, $visibility, webkit spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_background-image.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_background-image.scss
deleted file mode 100644
index 6abe88b..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_background-image.scss
+++ /dev/null
@@ -1,42 +0,0 @@
-//************************************************************************//
-// Background-image property for adding multiple background images with
-// gradients, or for stringing multiple gradients together.
-//************************************************************************//
-
-@mixin background-image($images...) {
- $webkit-images: ();
- $spec-images: ();
-
- @each $image in $images {
- $webkit-image: ();
- $spec-image: ();
-
- @if (type-of($image) == string) {
- $url-str: str-slice($image, 0, 3);
- $gradient-type: str-slice($image, 0, 6);
-
- @if $url-str == "url" {
- $webkit-image: $image;
- $spec-image: $image;
- }
-
- @else if $gradient-type == "linear" {
- $gradients: _linear-gradient-parser($image);
- $webkit-image: map-get($gradients, webkit-image);
- $spec-image: map-get($gradients, spec-image);
- }
-
- @else if $gradient-type == "radial" {
- $gradients: _radial-gradient-parser($image);
- $webkit-image: map-get($gradients, webkit-image);
- $spec-image: map-get($gradients, spec-image);
- }
- }
-
- $webkit-images: append($webkit-images, $webkit-image, comma);
- $spec-images: append($spec-images, $spec-image, comma);
- }
-
- background-image: $webkit-images;
- background-image: $spec-images;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_background.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_background.scss
deleted file mode 100644
index 9bce930..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_background.scss
+++ /dev/null
@@ -1,55 +0,0 @@
-//************************************************************************//
-// Background property for adding multiple backgrounds using shorthand
-// notation.
-//************************************************************************//
-
-@mixin background($backgrounds...) {
- $webkit-backgrounds: ();
- $spec-backgrounds: ();
-
- @each $background in $backgrounds {
- $webkit-background: ();
- $spec-background: ();
- $background-type: type-of($background);
-
- @if $background-type == string or list {
- $background-str: if($background-type == list, nth($background, 1), $background);
-
- $url-str: str-slice($background-str, 0, 3);
- $gradient-type: str-slice($background-str, 0, 6);
-
- @if $url-str == "url" {
- $webkit-background: $background;
- $spec-background: $background;
- }
-
- @else if $gradient-type == "linear" {
- $gradients: _linear-gradient-parser("#{$background}");
- $webkit-background: map-get($gradients, webkit-image);
- $spec-background: map-get($gradients, spec-image);
- }
-
- @else if $gradient-type == "radial" {
- $gradients: _radial-gradient-parser("#{$background}");
- $webkit-background: map-get($gradients, webkit-image);
- $spec-background: map-get($gradients, spec-image);
- }
-
- @else {
- $webkit-background: $background;
- $spec-background: $background;
- }
- }
-
- @else {
- $webkit-background: $background;
- $spec-background: $background;
- }
-
- $webkit-backgrounds: append($webkit-backgrounds, $webkit-background, comma);
- $spec-backgrounds: append($spec-backgrounds, $spec-background, comma);
- }
-
- background: $webkit-backgrounds;
- background: $spec-backgrounds;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_border-image.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_border-image.scss
deleted file mode 100644
index e338c2d..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_border-image.scss
+++ /dev/null
@@ -1,59 +0,0 @@
-@mixin border-image($borders...) {
- $webkit-borders: ();
- $spec-borders: ();
-
- @each $border in $borders {
- $webkit-border: ();
- $spec-border: ();
- $border-type: type-of($border);
-
- @if $border-type == string or list {
- $border-str: if($border-type == list, nth($border, 1), $border);
-
- $url-str: str-slice($border-str, 0, 3);
- $gradient-type: str-slice($border-str, 0, 6);
-
- @if $url-str == "url" {
- $webkit-border: $border;
- $spec-border: $border;
- }
-
- @else if $gradient-type == "linear" {
- $gradients: _linear-gradient-parser("#{$border}");
- $webkit-border: map-get($gradients, webkit-image);
- $spec-border: map-get($gradients, spec-image);
- }
-
- @else if $gradient-type == "radial" {
- $gradients: _radial-gradient-parser("#{$border}");
- $webkit-border: map-get($gradients, webkit-image);
- $spec-border: map-get($gradients, spec-image);
- }
-
- @else {
- $webkit-border: $border;
- $spec-border: $border;
- }
- }
-
- @else {
- $webkit-border: $border;
- $spec-border: $border;
- }
-
- $webkit-borders: append($webkit-borders, $webkit-border, comma);
- $spec-borders: append($spec-borders, $spec-border, comma);
- }
-
- -webkit-border-image: $webkit-borders;
- border-image: $spec-borders;
- border-style: solid;
-}
-
-//Examples:
-// @include border-image(url("image.png"));
-// @include border-image(url("image.png") 20 stretch);
-// @include border-image(linear-gradient(45deg, orange, yellow));
-// @include border-image(linear-gradient(45deg, orange, yellow) stretch);
-// @include border-image(linear-gradient(45deg, orange, yellow) 20 30 40 50 stretch round);
-// @include border-image(radial-gradient(top, cover, orange, yellow, orange));
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_border-radius.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_border-radius.scss
deleted file mode 100644
index 7c17190..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_border-radius.scss
+++ /dev/null
@@ -1,22 +0,0 @@
-//************************************************************************//
-// Shorthand Border-radius mixins
-//************************************************************************//
-@mixin border-top-radius($radii) {
- @include prefixer(border-top-left-radius, $radii, spec);
- @include prefixer(border-top-right-radius, $radii, spec);
-}
-
-@mixin border-bottom-radius($radii) {
- @include prefixer(border-bottom-left-radius, $radii, spec);
- @include prefixer(border-bottom-right-radius, $radii, spec);
-}
-
-@mixin border-left-radius($radii) {
- @include prefixer(border-top-left-radius, $radii, spec);
- @include prefixer(border-bottom-left-radius, $radii, spec);
-}
-
-@mixin border-right-radius($radii) {
- @include prefixer(border-top-right-radius, $radii, spec);
- @include prefixer(border-bottom-right-radius, $radii, spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_box-sizing.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_box-sizing.scss
deleted file mode 100644
index f07e1d4..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_box-sizing.scss
+++ /dev/null
@@ -1,4 +0,0 @@
-@mixin box-sizing ($box) {
-// content-box | border-box | inherit
- @include prefixer(box-sizing, $box, webkit moz spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_calc.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_calc.scss
deleted file mode 100644
index 94d7e4c..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_calc.scss
+++ /dev/null
@@ -1,4 +0,0 @@
-@mixin calc($property, $value) {
- #{$property}: -webkit-calc(#{$value});
- #{$property}: calc(#{$value});
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_columns.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_columns.scss
deleted file mode 100644
index 96f601c..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_columns.scss
+++ /dev/null
@@ -1,47 +0,0 @@
-@mixin columns($arg: auto) {
-// ||
- @include prefixer(columns, $arg, webkit moz spec);
-}
-
-@mixin column-count($int: auto) {
-// auto || integer
- @include prefixer(column-count, $int, webkit moz spec);
-}
-
-@mixin column-gap($length: normal) {
-// normal || length
- @include prefixer(column-gap, $length, webkit moz spec);
-}
-
-@mixin column-fill($arg: auto) {
-// auto || length
- @include prefixer(column-fill, $arg, webkit moz spec);
-}
-
-@mixin column-rule($arg) {
-// || ||
- @include prefixer(column-rule, $arg, webkit moz spec);
-}
-
-@mixin column-rule-color($color) {
- @include prefixer(column-rule-color, $color, webkit moz spec);
-}
-
-@mixin column-rule-style($style: none) {
-// none | hidden | dashed | dotted | double | groove | inset | inset | outset | ridge | solid
- @include prefixer(column-rule-style, $style, webkit moz spec);
-}
-
-@mixin column-rule-width ($width: none) {
- @include prefixer(column-rule-width, $width, webkit moz spec);
-}
-
-@mixin column-span($arg: none) {
-// none || all
- @include prefixer(column-span, $arg, webkit moz spec);
-}
-
-@mixin column-width($length: auto) {
-// auto || length
- @include prefixer(column-width, $length, webkit moz spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_filter.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_filter.scss
deleted file mode 100644
index 8560d77..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_filter.scss
+++ /dev/null
@@ -1,5 +0,0 @@
-@mixin filter($function: none) {
- // [
- @include prefixer(perspective, $depth, webkit moz spec);
-}
-
-@mixin perspective-origin($value: 50% 50%) {
- @include prefixer(perspective-origin, $value, webkit moz spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_placeholder.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_placeholder.scss
deleted file mode 100644
index 5682fd0..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_placeholder.scss
+++ /dev/null
@@ -1,8 +0,0 @@
-@mixin placeholder {
- $placeholders: ":-webkit-input" ":-moz" "-moz" "-ms-input";
- @each $placeholder in $placeholders {
- &:#{$placeholder}-placeholder {
- @content;
- }
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_radial-gradient.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_radial-gradient.scss
deleted file mode 100644
index 7a8c376..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_radial-gradient.scss
+++ /dev/null
@@ -1,39 +0,0 @@
-// Requires Sass 3.1+
-@mixin radial-gradient($G1, $G2,
- $G3: null, $G4: null,
- $G5: null, $G6: null,
- $G7: null, $G8: null,
- $G9: null, $G10: null,
- $pos: null,
- $shape-size: null,
- $fallback: null) {
-
- $data: _radial-arg-parser($G1, $G2, $pos, $shape-size);
- $G1: nth($data, 1);
- $G2: nth($data, 2);
- $pos: nth($data, 3);
- $shape-size: nth($data, 4);
-
- $full: $G1, $G2, $G3, $G4, $G5, $G6, $G7, $G8, $G9, $G10;
-
- // Strip deprecated cover/contain for spec
- $shape-size-spec: _shape-size-stripper($shape-size);
-
- // Set $G1 as the default fallback color
- $first-color: nth($full, 1);
- $fallback-color: nth($first-color, 1);
-
- @if (type-of($fallback) == color) or ($fallback == "transparent") {
- $fallback-color: $fallback;
- }
-
- // Add Commas and spaces
- $shape-size: if($shape-size, '#{$shape-size}, ', null);
- $pos: if($pos, '#{$pos}, ', null);
- $pos-spec: if($pos, 'at #{$pos}', null);
- $shape-size-spec: if(($shape-size-spec != ' ') and ($pos == null), '#{$shape-size-spec}, ', '#{$shape-size-spec} ');
-
- background-color: $fallback-color;
- background-image: -webkit-radial-gradient(unquote(#{$pos}#{$shape-size}#{$full}));
- background-image: unquote("radial-gradient(#{$shape-size-spec}#{$pos-spec}#{$full})");
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_transform.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_transform.scss
deleted file mode 100644
index 8cc3596..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_transform.scss
+++ /dev/null
@@ -1,15 +0,0 @@
-@mixin transform($property: none) {
-// none |
- @include prefixer(transform, $property, webkit moz ms o spec);
-}
-
-@mixin transform-origin($axes: 50%) {
-// x-axis - left | center | right | length | %
-// y-axis - top | center | bottom | length | %
-// z-axis - length
- @include prefixer(transform-origin, $axes, webkit moz ms o spec);
-}
-
-@mixin transform-style ($style: flat) {
- @include prefixer(transform-style, $style, webkit moz ms o spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_transition.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_transition.scss
deleted file mode 100644
index 5ad4c0a..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_transition.scss
+++ /dev/null
@@ -1,77 +0,0 @@
-// Shorthand mixin. Supports multiple parentheses-deliminated values for each variable.
-// Example: @include transition (all 2s ease-in-out);
-// @include transition (opacity 1s ease-in 2s, width 2s ease-out);
-// @include transition-property (transform, opacity);
-
-@mixin transition ($properties...) {
- // Fix for vendor-prefix transform property
- $needs-prefixes: false;
- $webkit: ();
- $moz: ();
- $spec: ();
-
- // Create lists for vendor-prefixed transform
- @each $list in $properties {
- @if nth($list, 1) == "transform" {
- $needs-prefixes: true;
- $list1: -webkit-transform;
- $list2: -moz-transform;
- $list3: ();
-
- @each $var in $list {
- $list3: join($list3, $var);
-
- @if $var != "transform" {
- $list1: join($list1, $var);
- $list2: join($list2, $var);
- }
- }
-
- $webkit: append($webkit, $list1);
- $moz: append($moz, $list2);
- $spec: append($spec, $list3);
- }
-
- // Create lists for non-prefixed transition properties
- @else {
- $webkit: append($webkit, $list, comma);
- $moz: append($moz, $list, comma);
- $spec: append($spec, $list, comma);
- }
- }
-
- @if $needs-prefixes {
- -webkit-transition: $webkit;
- -moz-transition: $moz;
- transition: $spec;
- }
- @else {
- @if length($properties) >= 1 {
- @include prefixer(transition, $properties, webkit moz spec);
- }
-
- @else {
- $properties: all 0.15s ease-out 0s;
- @include prefixer(transition, $properties, webkit moz spec);
- }
- }
-}
-
-@mixin transition-property ($properties...) {
- -webkit-transition-property: transition-property-names($properties, 'webkit');
- -moz-transition-property: transition-property-names($properties, 'moz');
- transition-property: transition-property-names($properties, false);
-}
-
-@mixin transition-duration ($times...) {
- @include prefixer(transition-duration, $times, webkit moz spec);
-}
-
-@mixin transition-timing-function ($motions...) {
-// ease | linear | ease-in | ease-out | ease-in-out | cubic-bezier()
- @include prefixer(transition-timing-function, $motions, webkit moz spec);
-}
-
-@mixin transition-delay ($times...) {
- @include prefixer(transition-delay, $times, webkit moz spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_user-select.scss b/themes/learn2-git-sync/scss/vendor/bourbon/css3/_user-select.scss
deleted file mode 100644
index 1380aa8..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/css3/_user-select.scss
+++ /dev/null
@@ -1,3 +0,0 @@
-@mixin user-select($arg: none) {
- @include prefixer(user-select, $arg, webkit moz ms spec);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_assign.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_assign.scss
deleted file mode 100644
index 9a1db93..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_assign.scss
+++ /dev/null
@@ -1,11 +0,0 @@
-@function assign-inputs($inputs, $pseudo: null) {
- $list : ();
-
- @each $input in $inputs {
- $input: unquote($input);
- $input: if($pseudo, $input + ":" + $pseudo, $input);
- $list: append($list, $input, comma);
- }
-
- @return $list;
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_color-lightness.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_color-lightness.scss
deleted file mode 100644
index 8c6df4e..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_color-lightness.scss
+++ /dev/null
@@ -1,13 +0,0 @@
-// Programatically determines whether a color is light or dark
-// Returns a boolean
-// More details here http://robots.thoughtbot.com/closer-look-color-lightness
-
-@function is-light($hex-color) {
- $-local-red: red(rgba($hex-color, 1.0));
- $-local-green: green(rgba($hex-color, 1.0));
- $-local-blue: blue(rgba($hex-color, 1.0));
-
- $-local-lightness: ($-local-red * 0.2126 + $-local-green * 0.7152 + $-local-blue * 0.0722) / 255;
-
- @return $-local-lightness > .6;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_flex-grid.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_flex-grid.scss
deleted file mode 100644
index 3bbd866..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_flex-grid.scss
+++ /dev/null
@@ -1,39 +0,0 @@
-// Flexible grid
-@function flex-grid($columns, $container-columns: $fg-max-columns) {
- $width: $columns * $fg-column + ($columns - 1) * $fg-gutter;
- $container-width: $container-columns * $fg-column + ($container-columns - 1) * $fg-gutter;
- @return percentage($width / $container-width);
-}
-
-// Flexible gutter
-@function flex-gutter($container-columns: $fg-max-columns, $gutter: $fg-gutter) {
- $container-width: $container-columns * $fg-column + ($container-columns - 1) * $fg-gutter;
- @return percentage($gutter / $container-width);
-}
-
-// The $fg-column, $fg-gutter and $fg-max-columns variables must be defined in your base stylesheet to properly use the flex-grid function.
-// This function takes the fluid grid equation (target / context = result) and uses columns to help define each.
-//
-// The calculation presumes that your column structure will be missing the last gutter:
-//
-// -- column -- gutter -- column -- gutter -- column
-//
-// $fg-column: 60px; // Column Width
-// $fg-gutter: 25px; // Gutter Width
-// $fg-max-columns: 12; // Total Columns For Main Container
-//
-// div {
-// width: flex-grid(4); // returns (315px / 995px) = 31.65829%;
-// margin-left: flex-gutter(); // returns (25px / 995px) = 2.51256%;
-//
-// p {
-// width: flex-grid(2, 4); // returns (145px / 315px) = 46.031746%;
-// float: left;
-// margin: flex-gutter(4); // returns (25px / 315px) = 7.936508%;
-// }
-//
-// blockquote {
-// float: left;
-// width: flex-grid(2, 4); // returns (145px / 315px) = 46.031746%;
-// }
-// }
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_golden-ratio.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_golden-ratio.scss
deleted file mode 100644
index 463d14a..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_golden-ratio.scss
+++ /dev/null
@@ -1,3 +0,0 @@
-@function golden-ratio($value, $increment) {
- @return modular-scale($value, $increment, $golden)
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_grid-width.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_grid-width.scss
deleted file mode 100644
index 8e63d83..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_grid-width.scss
+++ /dev/null
@@ -1,13 +0,0 @@
-@function grid-width($n) {
- @return $n * $gw-column + ($n - 1) * $gw-gutter;
-}
-
-// The $gw-column and $gw-gutter variables must be defined in your base stylesheet to properly use the grid-width function.
-//
-// $gw-column: 100px; // Column Width
-// $gw-gutter: 40px; // Gutter Width
-//
-// div {
-// width: grid-width(4); // returns 520px;
-// margin-left: $gw-gutter; // returns 40px;
-// }
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_modular-scale.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_modular-scale.scss
deleted file mode 100644
index afc59eb..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_modular-scale.scss
+++ /dev/null
@@ -1,66 +0,0 @@
-// Scaling Variables
-$golden: 1.618;
-$minor-second: 1.067;
-$major-second: 1.125;
-$minor-third: 1.2;
-$major-third: 1.25;
-$perfect-fourth: 1.333;
-$augmented-fourth: 1.414;
-$perfect-fifth: 1.5;
-$minor-sixth: 1.6;
-$major-sixth: 1.667;
-$minor-seventh: 1.778;
-$major-seventh: 1.875;
-$octave: 2;
-$major-tenth: 2.5;
-$major-eleventh: 2.667;
-$major-twelfth: 3;
-$double-octave: 4;
-
-@function modular-scale($value, $increment, $ratio) {
- $v1: nth($value, 1);
- $v2: nth($value, length($value));
- $value: $v1;
-
- // scale $v2 to just above $v1
- @while $v2 > $v1 {
- $v2: ($v2 / $ratio); // will be off-by-1
- }
- @while $v2 < $v1 {
- $v2: ($v2 * $ratio); // will fix off-by-1
- }
-
- // check AFTER scaling $v2 to prevent double-counting corner-case
- $double-stranded: $v2 > $v1;
-
- @if $increment > 0 {
- @for $i from 1 through $increment {
- @if $double-stranded and ($v1 * $ratio) > $v2 {
- $value: $v2;
- $v2: ($v2 * $ratio);
- } @else {
- $v1: ($v1 * $ratio);
- $value: $v1;
- }
- }
- }
-
- @if $increment < 0 {
- // adjust $v2 to just below $v1
- @if $double-stranded {
- $v2: ($v2 / $ratio);
- }
-
- @for $i from $increment through -1 {
- @if $double-stranded and ($v1 / $ratio) < $v2 {
- $value: $v2;
- $v2: ($v2 / $ratio);
- } @else {
- $v1: ($v1 / $ratio);
- $value: $v1;
- }
- }
- }
-
- @return $value;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_px-to-em.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_px-to-em.scss
deleted file mode 100644
index 4832245..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_px-to-em.scss
+++ /dev/null
@@ -1,13 +0,0 @@
-// Convert pixels to ems
-// eg. for a relational value of 12px write em(12) when the parent is 16px
-// if the parent is another value say 24px write em(12, 24)
-
-@function em($pxval, $base: $em-base) {
- @if not unitless($pxval) {
- $pxval: strip-units($pxval);
- }
- @if not unitless($base) {
- $base: strip-units($base);
- }
- @return ($pxval / $base) * 1em;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_px-to-rem.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_px-to-rem.scss
deleted file mode 100644
index 96b244e..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_px-to-rem.scss
+++ /dev/null
@@ -1,15 +0,0 @@
-// Convert pixels to rems
-// eg. for a relational value of 12px write rem(12)
-// Assumes $em-base is the font-size of
-
-@function rem($pxval) {
- @if not unitless($pxval) {
- $pxval: strip-units($pxval);
- }
-
- $base: $em-base;
- @if not unitless($base) {
- $base: strip-units($base);
- }
- @return ($pxval / $base) * 1rem;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_strip-units.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_strip-units.scss
deleted file mode 100644
index 6afc6e6..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_strip-units.scss
+++ /dev/null
@@ -1,5 +0,0 @@
-// Srtips the units from a value. e.g. 12px -> 12
-
-@function strip-units($val) {
- @return ($val / ($val * 0 + 1));
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_tint-shade.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_tint-shade.scss
deleted file mode 100644
index f717200..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_tint-shade.scss
+++ /dev/null
@@ -1,9 +0,0 @@
-// Add percentage of white to a color
-@function tint($color, $percent){
- @return mix(white, $color, $percent);
-}
-
-// Add percentage of black to a color
-@function shade($color, $percent){
- @return mix(black, $color, $percent);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_transition-property-name.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_transition-property-name.scss
deleted file mode 100644
index 54cd422..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_transition-property-name.scss
+++ /dev/null
@@ -1,22 +0,0 @@
-// Return vendor-prefixed property names if appropriate
-// Example: transition-property-names((transform, color, background), moz) -> -moz-transform, color, background
-//************************************************************************//
-@function transition-property-names($props, $vendor: false) {
- $new-props: ();
-
- @each $prop in $props {
- $new-props: append($new-props, transition-property-name($prop, $vendor), comma);
- }
-
- @return $new-props;
-}
-
-@function transition-property-name($prop, $vendor: false) {
- // put other properties that need to be prefixed here aswell
- @if $vendor and $prop == transform {
- @return unquote('-'+$vendor+'-'+$prop);
- }
- @else {
- @return $prop;
- }
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_unpack.scss b/themes/learn2-git-sync/scss/vendor/bourbon/functions/_unpack.scss
deleted file mode 100644
index 3775963..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/functions/_unpack.scss
+++ /dev/null
@@ -1,17 +0,0 @@
-// Convert shorthand to the 4-value syntax
-
-@function unpack($shorthand) {
- @if length($shorthand) == 1 {
- @return nth($shorthand, 1) nth($shorthand, 1) nth($shorthand, 1) nth($shorthand, 1);
- }
- @else if length($shorthand) == 2 {
- @return nth($shorthand, 1) nth($shorthand, 2) nth($shorthand, 1) nth($shorthand, 2);
- }
- @else if length($shorthand) == 3 {
- @return nth($shorthand, 1) nth($shorthand, 2) nth($shorthand, 3) nth($shorthand, 2);
- }
- @else {
- @return $shorthand;
- }
-}
-
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_convert-units.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_convert-units.scss
deleted file mode 100644
index 3443db3..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_convert-units.scss
+++ /dev/null
@@ -1,15 +0,0 @@
-//************************************************************************//
-// Helper function for str-to-num fn.
-// Source: http://sassmeister.com/gist/9647408
-//************************************************************************//
-@function _convert-units($number, $unit) {
- $strings: 'px' 'cm' 'mm' '%' 'ch' 'pica' 'in' 'em' 'rem' 'pt' 'pc' 'ex' 'vw' 'vh' 'vmin' 'vmax', 'deg', 'rad', 'grad', 'turn';
- $units: 1px 1cm 1mm 1% 1ch 1pica 1in 1em 1rem 1pt 1pc 1ex 1vw 1vh 1vmin 1vmax, 1deg, 1rad, 1grad, 1turn;
- $index: index($strings, $unit);
-
- @if not $index {
- @warn "Unknown unit `#{$unit}`.";
- @return false;
- }
- @return $number * nth($units, $index);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_gradient-positions-parser.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_gradient-positions-parser.scss
deleted file mode 100644
index 07d30b6..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_gradient-positions-parser.scss
+++ /dev/null
@@ -1,13 +0,0 @@
-@function _gradient-positions-parser($gradient-type, $gradient-positions) {
- @if $gradient-positions
- and ($gradient-type == linear)
- and (type-of($gradient-positions) != color) {
- $gradient-positions: _linear-positions-parser($gradient-positions);
- }
- @else if $gradient-positions
- and ($gradient-type == radial)
- and (type-of($gradient-positions) != color) {
- $gradient-positions: _radial-positions-parser($gradient-positions);
- }
- @return $gradient-positions;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_is-num.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_is-num.scss
deleted file mode 100644
index 71459e1..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_is-num.scss
+++ /dev/null
@@ -1,8 +0,0 @@
-//************************************************************************//
-// Helper for linear-gradient-parser
-//************************************************************************//
-@function _is-num($char) {
- $values: '0' '1' '2' '3' '4' '5' '6' '7' '8' '9' 0 1 2 3 4 5 6 7 8 9;
- $index: index($values, $char);
- @return if($index, true, false);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-angle-parser.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-angle-parser.scss
deleted file mode 100644
index e0401ed..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-angle-parser.scss
+++ /dev/null
@@ -1,25 +0,0 @@
-// Private function for linear-gradient-parser
-@function _linear-angle-parser($image, $first-val, $prefix, $suffix) {
- $offset: null;
- $unit-short: str-slice($first-val, str-length($first-val) - 2, str-length($first-val));
- $unit-long: str-slice($first-val, str-length($first-val) - 3, str-length($first-val));
-
- @if ($unit-long == "grad") or
- ($unit-long == "turn") {
- $offset: if($unit-long == "grad", -100grad * 3, -0.75turn);
- }
-
- @else if ($unit-short == "deg") or
- ($unit-short == "rad") {
- $offset: if($unit-short == "deg", -90 * 3, 1.6rad);
- }
-
- @if $offset {
- $num: _str-to-num($first-val);
-
- @return (
- webkit-image: -webkit- + $prefix + ($offset - $num) + $suffix,
- spec-image: $image
- );
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-gradient-parser.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-gradient-parser.scss
deleted file mode 100644
index 12bcdcd..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-gradient-parser.scss
+++ /dev/null
@@ -1,41 +0,0 @@
-@function _linear-gradient-parser($image) {
- $image: unquote($image);
- $gradients: ();
- $start: str-index($image, "(");
- $end: str-index($image, ",");
- $first-val: str-slice($image, $start + 1, $end - 1);
-
- $prefix: str-slice($image, 0, $start);
- $suffix: str-slice($image, $end, str-length($image));
-
- $has-multiple-vals: str-index($first-val, " ");
- $has-single-position: unquote(_position-flipper($first-val) + "");
- $has-angle: _is-num(str-slice($first-val, 0, 0));
-
- @if $has-multiple-vals {
- $gradients: _linear-side-corner-parser($image, $first-val, $prefix, $suffix, $has-multiple-vals);
- }
-
- @else if $has-single-position != "" {
- $pos: unquote($has-single-position + "");
-
- $gradients: (
- webkit-image: -webkit- + $image,
- spec-image: $prefix + "to " + $pos + $suffix
- );
- }
-
- @else if $has-angle {
- // Rotate degree for webkit
- $gradients: _linear-angle-parser($image, $first-val, $prefix, $suffix);
- }
-
- @else {
- $gradients: (
- webkit-image: -webkit- + $image,
- spec-image: $image
- );
- }
-
- @return $gradients;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-positions-parser.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-positions-parser.scss
deleted file mode 100644
index d26383e..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-positions-parser.scss
+++ /dev/null
@@ -1,61 +0,0 @@
-@function _linear-positions-parser($pos) {
- $type: type-of(nth($pos, 1));
- $spec: null;
- $degree: null;
- $side: null;
- $corner: null;
- $length: length($pos);
- // Parse Side and corner positions
- @if ($length > 1) {
- @if nth($pos, 1) == "to" { // Newer syntax
- $side: nth($pos, 2);
-
- @if $length == 2 { // eg. to top
- // Swap for backwards compatability
- $degree: _position-flipper(nth($pos, 2));
- }
- @else if $length == 3 { // eg. to top left
- $corner: nth($pos, 3);
- }
- }
- @else if $length == 2 { // Older syntax ("top left")
- $side: _position-flipper(nth($pos, 1));
- $corner: _position-flipper(nth($pos, 2));
- }
-
- @if ("#{$side} #{$corner}" == "left top") or ("#{$side} #{$corner}" == "top left") {
- $degree: _position-flipper(#{$side}) _position-flipper(#{$corner});
- }
- @else if ("#{$side} #{$corner}" == "right top") or ("#{$side} #{$corner}" == "top right") {
- $degree: _position-flipper(#{$side}) _position-flipper(#{$corner});
- }
- @else if ("#{$side} #{$corner}" == "right bottom") or ("#{$side} #{$corner}" == "bottom right") {
- $degree: _position-flipper(#{$side}) _position-flipper(#{$corner});
- }
- @else if ("#{$side} #{$corner}" == "left bottom") or ("#{$side} #{$corner}" == "bottom left") {
- $degree: _position-flipper(#{$side}) _position-flipper(#{$corner});
- }
- $spec: to $side $corner;
- }
- @else if $length == 1 {
- // Swap for backwards compatability
- @if $type == string {
- $degree: $pos;
- $spec: to _position-flipper($pos);
- }
- @else {
- $degree: -270 - $pos; //rotate the gradient opposite from spec
- $spec: $pos;
- }
- }
- $degree: unquote($degree + ",");
- $spec: unquote($spec + ",");
- @return $degree $spec;
-}
-
-@function _position-flipper($pos) {
- @return if($pos == left, right, null)
- if($pos == right, left, null)
- if($pos == top, bottom, null)
- if($pos == bottom, top, null);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss
deleted file mode 100644
index 86ad88f..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_linear-side-corner-parser.scss
+++ /dev/null
@@ -1,31 +0,0 @@
-// Private function for linear-gradient-parser
-@function _linear-side-corner-parser($image, $first-val, $prefix, $suffix, $has-multiple-vals) {
- $val-1: str-slice($first-val, 0, $has-multiple-vals - 1 );
- $val-2: str-slice($first-val, $has-multiple-vals + 1, str-length($first-val));
- $val-3: null;
- $has-val-3: str-index($val-2, " ");
-
- @if $has-val-3 {
- $val-3: str-slice($val-2, $has-val-3 + 1, str-length($val-2));
- $val-2: str-slice($val-2, 0, $has-val-3 - 1);
- }
-
- $pos: _position-flipper($val-1) _position-flipper($val-2) _position-flipper($val-3);
- $pos: unquote($pos + "");
-
- // Use old spec for webkit
- @if $val-1 == "to" {
- @return (
- webkit-image: -webkit- + $prefix + $pos + $suffix,
- spec-image: $image
- );
- }
-
- // Bring the code up to spec
- @else {
- @return (
- webkit-image: -webkit- + $image,
- spec-image: $prefix + "to " + $pos + $suffix
- );
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-arg-parser.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-arg-parser.scss
deleted file mode 100644
index a3a3704..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-arg-parser.scss
+++ /dev/null
@@ -1,69 +0,0 @@
-@function _radial-arg-parser($G1, $G2, $pos, $shape-size) {
- @each $value in $G1, $G2 {
- $first-val: nth($value, 1);
- $pos-type: type-of($first-val);
- $spec-at-index: null;
-
- // Determine if spec was passed to mixin
- @if type-of($value) == list {
- $spec-at-index: if(index($value, at), index($value, at), false);
- }
- @if $spec-at-index {
- @if $spec-at-index > 1 {
- @for $i from 1 through ($spec-at-index - 1) {
- $shape-size: $shape-size nth($value, $i);
- }
- @for $i from ($spec-at-index + 1) through length($value) {
- $pos: $pos nth($value, $i);
- }
- }
- @else if $spec-at-index == 1 {
- @for $i from ($spec-at-index + 1) through length($value) {
- $pos: $pos nth($value, $i);
- }
- }
- $G1: null;
- }
-
- // If not spec calculate correct values
- @else {
- @if ($pos-type != color) or ($first-val != "transparent") {
- @if ($pos-type == number)
- or ($first-val == "center")
- or ($first-val == "top")
- or ($first-val == "right")
- or ($first-val == "bottom")
- or ($first-val == "left") {
-
- $pos: $value;
-
- @if $pos == $G1 {
- $G1: null;
- }
- }
-
- @else if
- ($first-val == "ellipse")
- or ($first-val == "circle")
- or ($first-val == "closest-side")
- or ($first-val == "closest-corner")
- or ($first-val == "farthest-side")
- or ($first-val == "farthest-corner")
- or ($first-val == "contain")
- or ($first-val == "cover") {
-
- $shape-size: $value;
-
- @if $value == $G1 {
- $G1: null;
- }
-
- @else if $value == $G2 {
- $G2: null;
- }
- }
- }
- }
- }
- @return $G1, $G2, $pos, $shape-size;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-gradient-parser.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-gradient-parser.scss
deleted file mode 100644
index 6dde50f..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-gradient-parser.scss
+++ /dev/null
@@ -1,50 +0,0 @@
-@function _radial-gradient-parser($image) {
- $image: unquote($image);
- $gradients: ();
- $start: str-index($image, "(");
- $end: str-index($image, ",");
- $first-val: str-slice($image, $start + 1, $end - 1);
-
- $prefix: str-slice($image, 0, $start);
- $suffix: str-slice($image, $end, str-length($image));
-
- $is-spec-syntax: str-index($first-val, "at");
-
- @if $is-spec-syntax and $is-spec-syntax > 1 {
- $keyword: str-slice($first-val, 1, $is-spec-syntax - 2);
- $pos: str-slice($first-val, $is-spec-syntax + 3, str-length($first-val));
- $pos: append($pos, $keyword, comma);
-
- $gradients: (
- webkit-image: -webkit- + $prefix + $pos + $suffix,
- spec-image: $image
- )
- }
-
- @else if $is-spec-syntax == 1 {
- $pos: str-slice($first-val, $is-spec-syntax + 3, str-length($first-val));
-
- $gradients: (
- webkit-image: -webkit- + $prefix + $pos + $suffix,
- spec-image: $image
- )
- }
-
- @else if str-index($image, "cover") or str-index($image, "contain") {
- @warn "Radial-gradient needs to be updated to conform to latest spec.";
-
- $gradients: (
- webkit-image: null,
- spec-image: $image
- )
- }
-
- @else {
- $gradients: (
- webkit-image: -webkit- + $image,
- spec-image: $image
- )
- }
-
- @return $gradients;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-positions-parser.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-positions-parser.scss
deleted file mode 100644
index 6a5b477..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_radial-positions-parser.scss
+++ /dev/null
@@ -1,18 +0,0 @@
-@function _radial-positions-parser($gradient-pos) {
- $shape-size: nth($gradient-pos, 1);
- $pos: nth($gradient-pos, 2);
- $shape-size-spec: _shape-size-stripper($shape-size);
-
- $pre-spec: unquote(if($pos, "#{$pos}, ", null))
- unquote(if($shape-size, "#{$shape-size},", null));
- $pos-spec: if($pos, "at #{$pos}", null);
-
- $spec: "#{$shape-size-spec} #{$pos-spec}";
-
- // Add comma
- @if ($spec != ' ') {
- $spec: "#{$spec},"
- }
-
- @return $pre-spec $spec;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_render-gradients.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_render-gradients.scss
deleted file mode 100644
index 5765676..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_render-gradients.scss
+++ /dev/null
@@ -1,26 +0,0 @@
-// User for linear and radial gradients within background-image or border-image properties
-
-@function _render-gradients($gradient-positions, $gradients, $gradient-type, $vendor: false) {
- $pre-spec: null;
- $spec: null;
- $vendor-gradients: null;
- @if $gradient-type == linear {
- @if $gradient-positions {
- $pre-spec: nth($gradient-positions, 1);
- $spec: nth($gradient-positions, 2);
- }
- }
- @else if $gradient-type == radial {
- $pre-spec: nth($gradient-positions, 1);
- $spec: nth($gradient-positions, 2);
- }
-
- @if $vendor {
- $vendor-gradients: -#{$vendor}-#{$gradient-type}-gradient(#{$pre-spec} $gradients);
- }
- @else if $vendor == false {
- $vendor-gradients: "#{$gradient-type}-gradient(#{$spec} #{$gradients})";
- $vendor-gradients: unquote($vendor-gradients);
- }
- @return $vendor-gradients;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_shape-size-stripper.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_shape-size-stripper.scss
deleted file mode 100644
index ee5eda4..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_shape-size-stripper.scss
+++ /dev/null
@@ -1,10 +0,0 @@
-@function _shape-size-stripper($shape-size) {
- $shape-size-spec: null;
- @each $value in $shape-size {
- @if ($value == "cover") or ($value == "contain") {
- $value: null;
- }
- $shape-size-spec: "#{$shape-size-spec} #{$value}";
- }
- @return $shape-size-spec;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_str-to-num.scss b/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_str-to-num.scss
deleted file mode 100644
index b3d6168..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/helpers/_str-to-num.scss
+++ /dev/null
@@ -1,50 +0,0 @@
-//************************************************************************//
-// Helper function for linear/radial-gradient-parsers.
-// Source: http://sassmeister.com/gist/9647408
-//************************************************************************//
-@function _str-to-num($string) {
- // Matrices
- $strings: '0' '1' '2' '3' '4' '5' '6' '7' '8' '9';
- $numbers: 0 1 2 3 4 5 6 7 8 9;
-
- // Result
- $result: 0;
- $divider: 0;
- $minus: false;
-
- // Looping through all characters
- @for $i from 1 through str-length($string) {
- $character: str-slice($string, $i, $i);
- $index: index($strings, $character);
-
- @if $character == '-' {
- $minus: true;
- }
-
- @else if $character == '.' {
- $divider: 1;
- }
-
- @else {
- @if not $index {
- $result: if($minus, $result * -1, $result);
- @return _convert-units($result, str-slice($string, $i));
- }
-
- $number: nth($numbers, $index);
-
- @if $divider == 0 {
- $result: $result * 10;
- }
-
- @else {
- // Move the decimal dot to the left
- $divider: $divider * 10;
- $number: $number / $divider;
- }
-
- $result: $result + $number;
- }
- }
- @return if($minus, $result * -1, $result);
-}
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/settings/_prefixer.scss b/themes/learn2-git-sync/scss/vendor/bourbon/settings/_prefixer.scss
deleted file mode 100644
index ecab49f..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/settings/_prefixer.scss
+++ /dev/null
@@ -1,6 +0,0 @@
-// Variable settings for /addons/prefixer.scss
-$prefix-for-webkit: true !default;
-$prefix-for-mozilla: true !default;
-$prefix-for-microsoft: true !default;
-$prefix-for-opera: true !default;
-$prefix-for-spec: true !default; // required for keyframe mixin
diff --git a/themes/learn2-git-sync/scss/vendor/bourbon/settings/_px-to-em.scss b/themes/learn2-git-sync/scss/vendor/bourbon/settings/_px-to-em.scss
deleted file mode 100644
index f2f9a3e..0000000
--- a/themes/learn2-git-sync/scss/vendor/bourbon/settings/_px-to-em.scss
+++ /dev/null
@@ -1 +0,0 @@
-$em-base: 16px !default;
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/_color-schemer.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/_color-schemer.scss
deleted file mode 100644
index 469c697..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/_color-schemer.scss
+++ /dev/null
@@ -1,31 +0,0 @@
-@import "blend-modes";
-
-// Defaults
-$cs-primary : #f00 !default;
-$cs-scheme : mono !default; // mono, complement, triad, tetrad, analogic, accented-analogic
-$cs-hue-offset : 30 !default;
-$cs-brightness-offset : false !default;
-$cs-color-model : rgb !default; // rgb, ryb
-$cs-colorblind : normal !default;
-$cs-harmonize-mode : null !default;
-$cs-harmonize-color : $cs-primary !default;
-$cs-harmonize-amount : 10% !default;
-
-// Partials
-@import "color-schemer/interpolation";
-@import "color-schemer/cmyk";
-@import "color-schemer/ryb";
-@import "color-schemer/colorblind";
-@import "color-schemer/equalize";
-@import "color-schemer/mix";
-@import "color-schemer/tint-shade";
-@import "color-schemer/color-adjustments";
-@import "color-schemer/harmonize";
-@import "color-schemer/color-schemer";
-
-@import "color-schemer/comparison";
-
-@import "color-schemer/mixins";
-
-// Tell other files that this is loaded.
-$color-schemer-loaded : true;
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_cmyk.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_cmyk.scss
deleted file mode 100644
index 847115e..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_cmyk.scss
+++ /dev/null
@@ -1,14 +0,0 @@
-@function cmyk($cyan, $magenta, $yellow, $black) {
-
- // Get the color values out of white
- $cyan : mix(cyan , white, $cyan );
- $magenta : mix(magenta, white, $magenta);
- $yellow : mix(yellow , white, $yellow );
- $black : mix(black , white, $black );
-
- // Subtract the colors from white
- $color: white - invert($cyan) - invert($magenta) - invert($yellow) - invert($black);
-
-
- @return $color;
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_color-adjustments.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_color-adjustments.scss
deleted file mode 100644
index 6028b49..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_color-adjustments.scss
+++ /dev/null
@@ -1,30 +0,0 @@
-// RGB functions
-@function set-red($color, $red) {
- @return rgba($red, green($color), blue($color), alpha($color));
-}
-
-@function set-green($color, $green) {
- @return rgba(red($color), $green, blue($color), alpha($color));
-}
-
-@function set-blue($color, $blue) {
- @return rgba(red($color), green($color), $blue, alpha($color));
-}
-
-
-// HSL Functions
-@function set-hue($color, $hue) {
- @return hsla($hue, saturation($color), lightness($color), alpha($color));
-}
-
-@function set-saturation($color, $saturation) {
- @return hsla(hue($color), $saturation, lightness($color), alpha($color));
-}
-
-@function set-lightness($color, $lightness) {
- @return hsla(hue($color), saturation($color), $lightness, alpha($color));
-}
-
-@function set-alpha($color, $alpha) {
- @return hsla(hue($color), saturation($color), lightness($color), $alpha);
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_color-schemer.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_color-schemer.scss
deleted file mode 100644
index c092734..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_color-schemer.scss
+++ /dev/null
@@ -1,208 +0,0 @@
-// brightness and hue offsets are based on the lightness and saturation of the color
-// unless defined otherwise.
-@function cs-brightness-offset($cs-brightness-offset) {
- @if $cs-brightness-offset == false {
- // find the difference between lightness
- @return lightness($cs-primary) - lightness(invert($cs-primary));
- }
- @else {
- @return $cs-brightness-offset;
- }
-}
-
-// Harmonized or Unaltered Color
-@function clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount) {
- @if $cs-harmonize-mode != null {
- @return cs-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- } @else {
- @return $color;
- }
-}
-
-// Primary color
-@function cs-primary($cs-primary:$cs-primary, $cs-scheme:$cs-scheme, $cs-hue-offset:$cs-hue-offset, $cs-brightness-offset:$cs-brightness-offset, $cs-harmonize-mode:$cs-harmonize-mode, $cs-harmonize-color:$cs-harmonize-color, $cs-harmonize-amount:$cs-harmonize-amount) {
- @return clean-or-harmonize($cs-primary, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
-}
-
-// Secondary color scheme
-@function cs-secondary($cs-primary:$cs-primary, $cs-scheme:$cs-scheme, $cs-hue-offset:$cs-hue-offset, $cs-brightness-offset:$cs-brightness-offset, $cs-harmonize-mode:$cs-harmonize-mode, $cs-harmonize-color:$cs-harmonize-color, $cs-harmonize-amount:$cs-harmonize-amount) {
- $cs-brightness-offset: cs-brightness-offset($cs-brightness-offset);
-
- // mono
- @if $cs-scheme == mono {
- @if $cs-brightness-offset < 0 {
- $color: lighten($cs-primary, abs($cs-brightness-offset));
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: darken($cs-primary, abs($cs-brightness-offset));
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-
- // complement
- @if $cs-scheme == complement {
- @if $cs-color-model == ryb {
- $color: ryb-complement($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: complement($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-
- // triad
- @if $cs-scheme == triad {
- @if $cs-color-model == ryb {
- $color: ryb-adjust-hue(ryb-complement($cs-primary), $cs-hue-offset);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: adjust-hue(complement($cs-primary), $cs-hue-offset);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-
- // tetrad
- @if $cs-scheme == tetrad {
- @if $cs-color-model == ryb {
- $color: ryb-adjust-hue($cs-primary, $cs-hue-offset);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: adjust-hue($cs-primary, $cs-hue-offset);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-
- // analogic
- @if $cs-scheme == analogic or $cs-scheme == accented-analogic {
- @if $cs-color-model == ryb {
- $color: ryb-adjust-hue($cs-primary, $cs-hue-offset);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: adjust-hue($cs-primary, $cs-hue-offset);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-}
-
-// Tertiary color scheme
-@function cs-tertiary($cs-primary:$cs-primary, $cs-scheme:$cs-scheme, $cs-hue-offset:$cs-hue-offset, $cs-brightness-offset:$cs-brightness-offset, $cs-harmonize-mode:$cs-harmonize-mode, $cs-harmonize-color:$cs-harmonize-color, $cs-harmonize-amount:$cs-harmonize-amount) {
- $cs-brightness-offset: cs-brightness-offset($cs-brightness-offset);
-
- // mono
- @if $cs-scheme == mono {
- $color: mix(cs-primary(), cs-secondary());
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
-
- // complement
- @if $cs-scheme == complement {
- $color: equalize($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
-
- // triad
- @if $cs-scheme == triad {
- @if $cs-color-model == ryb {
- $color: ryb-adjust-hue(ryb-complement($cs-primary), $cs-hue-offset * -1);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: adjust-hue(complement($cs-primary), $cs-hue-offset * -1);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-
- // tetrad
- @if $cs-scheme == tetrad {
- @if $cs-color-model == ryb {
- $color: ryb-complement($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: complement($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-
- // analogic
- @if $cs-scheme == analogic or $cs-scheme == accented-analogic {
- @if $cs-color-model == ryb {
- $color: ryb-adjust-hue($cs-primary, $cs-hue-offset * -1);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: adjust-hue($cs-primary, $cs-hue-offset * -1);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-
- // accented-analogic
- @if $cs-scheme == accented-analogic {
- @if $cs-color-model == ryb {
- $color: ryb-complement($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: complement($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-}
-
-// Quadrary color scheme
-@function cs-quadrary($cs-primary:$cs-primary, $cs-scheme:$cs-scheme, $cs-hue-offset:$cs-hue-offset, $cs-brightness-offset:$cs-brightness-offset, $cs-harmonize-mode:$cs-harmonize-mode, $cs-harmonize-color:$cs-harmonize-color, $cs-harmonize-amount:$cs-harmonize-amount) {
- $cs-brightness-offset: cs-brightness-offset($cs-brightness-offset);
-
- // mono
- @if $cs-scheme == mono {
- $color: equalize($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
-
- // complement
- @if $cs-scheme == complement {
- $color: equalize(ryb-complement($cs-primary));
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
-
- // triad
- @if $cs-scheme == triad {
- $color: equalize($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
-
- // tetrad
- @if $cs-scheme == tetrad {
- @if $cs-color-model == ryb {
- $color: ryb-adjust-hue(ryb-complement($cs-primary), $cs-hue-offset);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: adjust-hue(complement($cs-primary), $cs-hue-offset);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-
- // analogic
- @if $cs-scheme == analogic {
- $color: equalize($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
-
- // accented-analogic
- @if $cs-scheme == accented-analogic {
- @if $cs-color-model == ryb {
- $color: ryb-complement($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- @else {
- $color: complement($cs-primary);
- @return clean-or-harmonize($color, $cs-harmonize-color, $cs-harmonize-mode, $cs-harmonize-amount);
- }
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_colorblind.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_colorblind.scss
deleted file mode 100644
index b509183..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_colorblind.scss
+++ /dev/null
@@ -1,29 +0,0 @@
-@function cs-colorblind($color, $mode: $cs-colorblind) {
-
- // Refrence: http://www.w3.org/TR/AERT#color-contrast
-
- // Deuteranopia
- @if $mode == deuteranopia {
- @return $color;
- }
-
- // Protanopia
- @if $mode == protanopia {
- @return $color;
- }
-
- // Tritanopia
- @if $mode == tritanopia {
- @return $color;
- }
-
-
- // Return color if no color blind mode.
- @else {
- @return $color;
- }
-}
-
-@function cs-cb($color, $mode: $cs-colorblind) {
- @return cs-colorblind($color, $mode);
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_comparison.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_comparison.scss
deleted file mode 100644
index 84ed665..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_comparison.scss
+++ /dev/null
@@ -1,15 +0,0 @@
-//////////////////////////////
-// Color Is Dark
-//
-// Checks to see if the input color is a dark color taking into account both lightness and hue.
-// Suitable for determining, for instance, if a background should have a dark or light text color.
-// @return true/false (boolean)
-//////////////////////////////
-
-@function cs-is-dark($color) {
- @if (lightness($color) < 60% and (hue($color) >= 210 or hue($color) <= 27)) or (lightness($color) <= 32%) {
- @return true;
- } @else {
- @return false;
- }
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_equalize.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_equalize.scss
deleted file mode 100644
index d043bc8..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_equalize.scss
+++ /dev/null
@@ -1,5 +0,0 @@
-// Color equalize credit to Mason Wendell:
-// https://github.com/canarymason/The-Coding-Designers-Survival-Kit/blob/master/sass/partials/lib/variables/_color_schemes.sass
-@function equalize($color) {
- @return hsl(hue($color), 100%, 50%);
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_harmonize.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_harmonize.scss
deleted file mode 100644
index 719b43d..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_harmonize.scss
+++ /dev/null
@@ -1,59 +0,0 @@
-@function cs-harmonize($background, $foreground: $cs-harmonize-color, $mode: $cs-harmonize-mode, $amount: $cs-harmonize-amount) {
- $blend: null;
- @if ($mode == normal) {
- $blend: blend-normal($foreground, $background); }
- @else if ($mode == multiply) {
- $blend: blend-multiply($foreground, $background); }
- @else if ($mode == lighten) {
- $blend: blend-lighten($foreground, $background); }
- @else if ($mode == darken) {
- $blend: blend-darken($foreground, $background); }
- @else if ($mode == darkercolor) {
- $blend: blend-darkercolor($foreground, $background); }
- @else if ($mode == lightercolor) {
- $blend: blend-lightercolor($foreground, $background); }
- @else if ($mode == lineardodge) {
- $blend: blend-lineardodge($foreground, $background); }
- @else if ($mode == linearburn) {
- $blend: blend-linearburn($foreground, $background); }
- @else if ($mode == difference) {
- $blend: blend-difference($foreground, $background); }
- @else if ($mode == screen) {
- $blend: blend-screen($foreground, $background); }
- @else if ($mode == exclusion) {
- $blend: blend-exclusion($foreground, $background); }
- @else if ($mode == overlay) {
- $blend: blend-overlay($foreground, $background); }
- @else if ($mode == softlight) {
- $blend: blend-softlight($foreground, $background); }
- @else if ($mode == hardlight) {
- $blend: blend-hardlight($foreground, $background); }
- @else if ($mode == colordodge) {
- $blend: blend-colordodge($foreground, $background); }
- @else if ($mode == colorburn) {
- $blend: blend-colorburn($foreground, $background); }
- @else if ($mode == linearlight) {
- $blend: blend-linearlight($foreground, $background); }
- @else if ($mode == vividlight) {
- $blend: blend-vividlight($foreground, $background); }
- @else if ($mode == pinlight) {
- $blend: blend-pinlight($foreground, $background); }
- @else if ($mode == hardmix) {
- $blend: blend-hardmix($foreground, $background); }
- @else if ($mode == colorblend) {
- $blend: blend-colorblend($foreground, $background); }
- @else if ($mode == dissolve) {
- $blend: blend-dissolve($foreground, $background); }
- @else if ($mode == divide) {
- $blend: blend-divide($foreground, $background); }
- @else if ($mode == hue) {
- $blend: blend-hue($foreground, $background); }
- @else if ($mode == luminosity) {
- $blend: blend-luminosity($foreground, $background); }
- @else if ($mode == saturation) {
- $blend: blend-saturation($foreground, $background); }
- @else if ($mode == subtract) {
- $blend: blend-subtract($foreground, $background); }
- $mixed: mix($blend, $background, $amount);
- @return $mixed;
-}
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_interpolation.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_interpolation.scss
deleted file mode 100644
index 2ec182e..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_interpolation.scss
+++ /dev/null
@@ -1,34 +0,0 @@
-@function cs-interpolate($value, $units: 360, $stops: $ryb-interpolation) {
-
- // Loop numbers out of scale back into the scale.
- @while $value >= 360 {
- $value: $value - 360;
- }
- @while $value < 0 {
- $value: $value + 360;
- }
-
- // Find out how many units in each stop
- $cs-color-deg: $units / length($stops);
-
- // Count through stops
- $cs-deg-count: $cs-color-deg;
- $cs-stop-count: 1;
-
- // Add the first stop to the end so it will be
- // interpolated with the last stop.
- $stops: append($stops, nth($stops, 1));
-
- // Start interpolating
- @for $i from 0 through length($stops) {
- @if $value < $cs-deg-count {
- @return cs-mix(nth($stops, $cs-stop-count + 1), nth($stops, $cs-stop-count), abs(percentage(($cs-deg-count - $value) / $cs-color-deg) - 100 ), $model: rgb);
- }
-
- // If the value is not in this stop, loop up to another stop.
- @else {
- $cs-deg-count: $cs-deg-count + $cs-color-deg;
- $cs-stop-count: $cs-stop-count + 1
- }
- }
-}
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_mix.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_mix.scss
deleted file mode 100644
index 4d3a68f..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_mix.scss
+++ /dev/null
@@ -1,40 +0,0 @@
-@function cs-mix($color1, $color2, $percent: 50%, $model: $cs-color-model) {
-
- $decimal : abs($percent - 100%) / 100%;
- $hue-offset : ();
-
- @if $model == rgb {
- $hue-offset : (hue($color1) - hue($color2)) * $decimal;
- @if (hue($color1) - hue($color2)) * .5 < -90deg {
- $hue-offset : (hue($color1) + 360deg - hue($color2)) * $decimal;
- }
- @if (hue($color1) - hue($color2)) * .5 > 90deg {
- $hue-offset : (hue($color1) - 360deg - hue($color2)) * $decimal;
- }
- }
-
- @if $model == ryb {
- $hue-offset : (ryb-hue($color1) - ryb-hue($color2)) * $decimal;
- @if (ryb-hue($color1) - ryb-hue($color2)) * .5 < -90deg {
- $hue-offset : (ryb-hue($color1) + 360deg - ryb-hue($color2)) * $decimal;
- }
- @if (ryb-hue($color1) - ryb-hue($color2)) * .5 > 90deg {
- $hue-offset : (ryb-hue($color1) - 360deg - ryb-hue($color2)) * $decimal;
- }
- }
-
- $saturation-offset : (saturation($color1) - saturation($color2)) * $decimal;
- $lightness-offset : (lightness($color1) - lightness($color2)) * $decimal;
-
- @if $model == ryb {
- $color1: ryb-adjust-hue($color1, $hue-offset * -1);
- }
- @else {
- $color1: adjust-hue($color1, $hue-offset * -1);
- }
-
- $color1: set-saturation($color1, saturation($color1) - $saturation-offset);
- $color1: set-lightness($color1, lightness($color1) - $lightness-offset);
-
- @return $color1;
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_mixins.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_mixins.scss
deleted file mode 100644
index 7e3a8f4..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_mixins.scss
+++ /dev/null
@@ -1,29 +0,0 @@
-////////////////////////////////////////////
-// From Jina Bolton and Eric Meyer -- http://codepen.io/jina/pen/iosjp
-@function cs-stripes($position, $colors) {
- $colors: if(type-of($colors) != 'list', compact($colors), $colors);
- $gradient: ();
- $width: 100% / length($colors);
-
- @for $i from 1 through length($colors) {
- $pop: nth($colors,$i);
- $new: $pop ($width * ($i - 1)), $pop ($width * $i);
- $gradient: join($gradient, $new, comma);
- }
-
- @return linear-gradient($position, $gradient);
-}
-
-////////////////////////////////////////////
-// Color tester
-
-@mixin cs-test($colors, $height: 2em, $element: "body:before") {
- #{$element} {
- content: "";
- display: block;
- height: $height;
- @include background(cs-stripes(left, ($colors)));
- position: relative;
- z-index: 999999999999;
- }
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_ryb.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_ryb.scss
deleted file mode 100644
index 1a6de0e..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_ryb.scss
+++ /dev/null
@@ -1,76 +0,0 @@
-$ryb-interpolation: #FF0000 #FF4900 #FF7400 #FF9200 #FFAA00 #FFBF00 #FFD300 #FFE800 #FFFF00 #CCF600 #9FEE00 #67E300 #00CC00 #00AF64 #009999 #0B61A4 #1240AB #1B1BB3 #3914AF #530FAD #7109AA #A600A6 #CD0074 #E40045;
-
-// RYB color interpolation
-@function find-ryb($hue) {
-
- // remove units on $hue
- @if unit($hue) == deg { $hue: $hue / 1deg; }
-
- // return an interpolated hue
- @return hue(cs-interpolate($hue));
-}
-
-// Find the RYB hue instead of RGB hue of a color.
-
-// map of the RYB offset
-$ryb-offset: 0 1 2 3 5 6 7 8 9 10 11 13 14 15 16 17 18 19 19 20 21 21 22 23 23 24 25 25 26 27 27 28 28 29 29 30 30 31 31 32 32 32 33 33 34 34 35 35 35 36 36 37 37 37 38 38 38 39 39 40 40 40 41 41 41 42 42 42 43 43 43 44 44 44 45 45 45 46 46 46 47 47 47 47 48 48 48 49 49 49 50 50 50 51 51 51 52 52 52 53 53 53 54 54 54 55 55 55 56 56 56 57 57 57 58 58 59 59 59 60 60 61 61 62 63 63 64 65 65 66 67 68 68 69 70 70 71 72 72 73 73 74 75 75 76 77 77 78 79 79 80 81 82 82 83 84 85 86 87 88 88 89 90 91 92 93 95 96 98 100 102 104 105 107 109 111 113 115 116 118 120 122 125 127 129 131 134 136 138 141 143 145 147 150 152 154 156 158 159 161 163 165 166 168 170 171 173 175 177 178 180 182 184 185 187 189 191 192 194 196 198 199 201 203 205 206 207 208 209 210 212 213 214 215 216 217 218 219 220 221 222 223 224 226 227 228 229 230 232 233 234 235 236 238 239 240 241 242 243 244 245 246 247 248 249 250 251 251 252 253 254 255 256 257 257 258 259 260 260 261 262 263 264 264 265 266 267 268 268 269 270 271 272 273 274 274 275 276 277 278 279 280 282 283 284 286 287 289 290 292 293 294 296 297 299 300 302 303 305 307 309 310 312 314 316 317 319 321 323 324 326 327 328 329 330 331 332 333 334 336 337 338 339 340 341 342 343 344 345 347 348 349 350 352 353 354 355 356 358 359 360;
-
-// loop through the map to find the matching hue.
-@function ryb-hue($color) {
- @for $i from 1 through length($ryb-offset) {
- @if nth($ryb-offset, $i) > hue($color) {
- @return $i - 2deg;
- }
- }
-}
-
-// Changes the hue of a color.
-@function ryb-adjust-hue($color, $degrees) {
-
- // Convert precentag to degrees.
- @if unit($degrees) == "%" {
- $degrees: 360 * ($degrees / 100%);
- }
-
- // Start at the current hue and loop in the adjustment.
- $hue-adjust: (ryb-hue($color) + $degrees) / 1deg;
-
- @return hsl(hue(cs-interpolate($hue-adjust)), saturation($color), lightness($color));
-}
-
-@function ryba($red, $yellow, $blue, $alpha) {
- $hue: 0;
- $saturation: 0;
- $lightness: percentage(($red + $yellow + $blue) / (255 * 3));
- @if $red == $yellow and $yellow == $blue {
- @return hsla(0, 0, $lightness, $alpha);
- }
- @if $red >= $yellow and $red >= $blue {
- $hue: 0;
- }
- @elseif $yellow >= $red and $yellow >= $blue {
- $hue: 360 / 3;
- }
- @elseif $blue >= $red and $blue >= $yellow {
- $hue: 360 / 3 * 2;
- }
- @return hsla(hue(cs-interpolate($hue)), 100%, 50%, 1);
-}
-
-@function ryb($red, $yellow, $blue) {
- @return ryba($red, $yellow, $blue, 1);
-}
-
-@function set-ryb-hue($color, $hue) {
- @return hsla(hue(cs-interpolate($hue)), saturation($color), lightness($color), alpha($color));
-}
-
-// Returns the complement of a color.
-@function ryb-complement($color) {
- @return ryb-adjust-hue($color, 180deg);
-}
-
-// Returns the inverse of a color.
-@function ryb-invert($color) {
- @return ryb-adjust-hue(hsl(hue($color), saturation(invert($color)), lightness(invert($color))), 180deg);
-}
\ No newline at end of file
diff --git a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_tint-shade.scss b/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_tint-shade.scss
deleted file mode 100644
index d1198d0..0000000
--- a/themes/learn2-git-sync/scss/vendor/color-schemer/color-schemer/_tint-shade.scss
+++ /dev/null
@@ -1,9 +0,0 @@
-// Add percentage of white to a color
-@function tint($color, $percent) {
- @return mix(white, $color, $percent);
-}
-
-// Add percentage of black to a color
-@function shade($color, $percent) {
- @return mix(black, $color, $percent);
-}
diff --git a/themes/learn2-git-sync/shortcodes/GoogleSlidesShortcode.php b/themes/learn2-git-sync/shortcodes/GoogleSlidesShortcode.php
deleted file mode 100644
index 598b8e2..0000000
--- a/themes/learn2-git-sync/shortcodes/GoogleSlidesShortcode.php
+++ /dev/null
@@ -1,34 +0,0 @@
-shortcode->getRawHandlers()->add('googleslides', function(ShortcodeInterface $sc) {
-
- // Get shortcode content and parameters
- $str = $sc->getContent();
-
- $googleslidesurl= $sc->getParameter('url', $sc->getBbCode());
-
- if ($googleslidesurl) {
- $output = '
';
-
- return $output;
-
- } else {
-
- if ($str) {
-
- return '
';
-
- }
- }
-
- });
- }
-}
diff --git a/themes/learn2-git-sync/shortcodes/H5PShortcode.php b/themes/learn2-git-sync/shortcodes/H5PShortcode.php
deleted file mode 100644
index 0af8804..0000000
--- a/themes/learn2-git-sync/shortcodes/H5PShortcode.php
+++ /dev/null
@@ -1,36 +0,0 @@
-shortcode->getHandlers()->add('h5p', function(ShortcodeInterface $sc) {
-
- // Get shortcode content and parameters
- $str = $sc->getContent();
-
- $h5purl= $sc->getParameter('url', $sc->getBbCode());
-
- if ($h5purl) {
- $output = '
';
-
- return $output;
-
- } else {
-
- if ($str) {
- $output = '
';
-
- return $output;
- }
-
- }
-
- });
- }
-}
diff --git a/themes/learn2-git-sync/shortcodes/PDFShortcode.php b/themes/learn2-git-sync/shortcodes/PDFShortcode.php
deleted file mode 100644
index a3e3a42..0000000
--- a/themes/learn2-git-sync/shortcodes/PDFShortcode.php
+++ /dev/null
@@ -1,35 +0,0 @@
-shortcode->getHandlers()->add('pdf', function(ShortcodeInterface $sc) {
-
- // Get shortcode content and parameters
- $str = $sc->getContent();
-
- $pdfurl= $sc->getParameter('url', $sc->getBbCode());
-
- if ($pdfurl) {
- $output = '
';
-
- return $output;
-
- } else {
-
- if ($str) {
-
- return '
';
-
- }
-
- }
-
- });
- }
-}
diff --git a/themes/learn2-git-sync/templates/chapter.html.twig b/themes/learn2-git-sync/templates/chapter.html.twig
deleted file mode 100644
index 3cdcd5b..0000000
--- a/themes/learn2-git-sync/templates/chapter.html.twig
+++ /dev/null
@@ -1,11 +0,0 @@
-{% extends 'docs.html.twig' %}
-
-{% block content %}
-
-
-
- {{ page.content|raw }}
-
-
-
-{% endblock %}
diff --git a/themes/learn2-git-sync/templates/docs.html.twig b/themes/learn2-git-sync/templates/docs.html.twig
deleted file mode 100644
index 4d594c9..0000000
--- a/themes/learn2-git-sync/templates/docs.html.twig
+++ /dev/null
@@ -1,23 +0,0 @@
-{% extends 'partials/base.html.twig' %}
-{% set tags = page.taxonomy.tag %}
-{% if tags %}
- {% set progress = page.collection({'items':{'@taxonomy':{'category': 'docs', 'tag': tags}},'order': {'by': 'default', 'dir': 'asc'}}) %}
-{% else %}
- {% set progress = page.collection({'items':{'@taxonomy':{'category': 'docs'}},'order': {'by': 'default', 'dir': 'asc'}}) %}
-{% endif %}
-
-{% block navigation %}
-
- {% if not progress.isFirst(page.path) and not (progress.nextSibling(page.path).url == progress.prevSibling(page.path).url) %}
-
- {% endif %}
-
- {% if not progress.isLast(page.path) and not (progress.nextSibling(page.path).url == progress.prevSibling(page.path).url) %}
-
- {% endif %}
-
-{% endblock %}
-
-{% block content %}
- {% include 'partials/page.html.twig' %}
-{% endblock %}
diff --git a/themes/learn2-git-sync/templates/partials/base.html.twig b/themes/learn2-git-sync/templates/partials/base.html.twig
deleted file mode 100644
index d843457..0000000
--- a/themes/learn2-git-sync/templates/partials/base.html.twig
+++ /dev/null
@@ -1,106 +0,0 @@
-{% set theme_config = attribute(config.themes, config.system.pages.theme) %}
-
-
-
- {% block head %}
-
- {% if header.title %}{{ header.title }} | {% endif %}{{ site.title }}
- {% include 'partials/metadata.html.twig' %}
-
-
-
-
-
- {% block stylesheets %}
- {% do assets.addCss('theme://css-compiled/nucleus.css', 102) %}
- {% do assets.addCss('theme://css/custom.css', 100) %}
- {% do assets.addCss('theme://css/font-awesome.min.css',100) %}
- {% do assets.addCss('theme://css/featherlight.min.css') %}
-
- {% if browser.getBrowser == 'msie' and browser.getVersion >= 8 and browser.getVersion <= 9 %}
- {% do assets.addCss('theme://css/nucleus-ie9.css') %}
- {% do assets.addCss('theme://css/pure-0.5.0/grids-min.css') %}
- {% do assets.addJs('theme://js/html5shiv-printshiv.min.js') %}
- {% endif %}
- {% endblock %}
-
- {% block javascripts %}
- {% do assets.addJs('jquery',101) %}
- {% do assets.addJs('theme://js/modernizr.custom.71422.js',100) %}
- {% do assets.addJs('theme://js/featherlight.min.js') %}
- {% do assets.addJs('theme://js/clipboard.min.js') %}
- {% do assets.addJs('theme://js/jquery.scrollbar.min.js') %}
- {% do assets.addJs('theme://js/learn.js') %}
- {% endblock %}
-
- {% block assets deferred %}
- {{ assets.css()|raw }}
- {{ assets.js()|raw }}
- {% endblock %}
-
- {% endblock %}
-
-
- {% block sidebar %}
-
- {% endblock %}
-
- {% block body %}
-
-
-
-
-
-
- {% block topbar %}{% if theme_config.github.position == 'top' or config.plugins.breadcrumbs.enabled %}
-
- {% if theme_config.github.position == 'top' and not ('search' in page.name) %}
-
- {% include 'partials/github_link.html.twig' %}
-
- {% endif %}
-
- {% if config.plugins.breadcrumbs.enabled %}
- {% include 'partials/breadcrumbs.html.twig' %}
- {% endif %}
-
- {% endif %}{% endblock %}
-
- {% block content %}{% endblock %}
-
- {% block footer %}
- {% if theme_config.github.position == 'bottom' and not ('search' in page.name) %}
- {% include 'partials/github_note.html.twig' %}
- {% endif %}
- {% endblock %}
-
-
- {% block navigation %}{% endblock %}
-
- {% endblock %}
- {% block analytics %}
- {% if theme_config.google_analytics_code %}
- {% include 'partials/analytics.html.twig' %}
- {% endif %}
- {% endblock %}
-
- {% block bottom %}
- {{ assets.js('bottom')|raw }}
- {% endblock %}
-
- {# added global scripts - hibbittsdesign.org #}
- {% include 'partials/scripts.html.twig' %}
-
-
-
diff --git a/themes/learn2-git-sync/templates/partials/github_link.html.twig b/themes/learn2-git-sync/templates/partials/github_link.html.twig
deleted file mode 100644
index 4c9f4f4..0000000
--- a/themes/learn2-git-sync/templates/partials/github_link.html.twig
+++ /dev/null
@@ -1,55 +0,0 @@
-{% if theme_config.github.tree %}
- {% set git_repo_link_url = theme_config.github.tree %}
- {% if 'github' in git_repo_link_url %}
- {% set git_repo_link_icon = "github" %}
- {% elseif 'gitlab' in git_repo_link_url %}
- {% set git_repo_link_icon = "gitlab" %}
- {% elseif 'bitbucket' in git_repo_link_url %}
- {% set git_repo_link_icon = "bitbucket" %}
- {% else %}
- {% set git_repo_link_icon = "git" %}
- {% endif %}
- {% if theme_config.github.icon %}
- {% set git_repo_link_icon = theme_config.github.icon %}
- {% endif %}
- {% set git_repo_edit_link_url = git_repo_link_url ~ ('/'~page.filePathClean)|replace({'/user/':'/'}) %}
-
-
- {{ 'THEME_LEARN2_GIT_EDIT_THIS_PAGE'|t }}
-{% else %}
- {% if not config.plugins['git-sync'].enabled %}
- {% set admin_panel_appearance_url = grav.base_url ~ '/admin/plugins/' %}
-
-
- {{ 'THEME_LEARN2_GIT_ADD_SETUP_GIT_SYNC_PLUGIN'|t }}
- {% else %}
- {% if config.plugins['git-sync'].enabled and config.plugins['git-sync'].repository is empty %}
- {% set admin_panel_appearance_url = grav.base_url ~ '/admin/plugins/git-sync' %}
-
-
- {{ 'THEME_LEARN2_GIT_SETUP_GIT_SYNC_PLUGIN'|t }}
- {% else %}
- {% set git_sync_repo = config.plugins['git-sync'].repository %}
- {% set git_sync_repo_link = (git_sync_repo | replace({'.git': '/'})) %}
- {% if 'github' in git_sync_repo_link %}
- {% set git_repo_link_icon = "github" %}
- {% set git_repo_edit_link_url = git_sync_repo_link ~ 'blob/master' ~ (page.filePathClean)|replace({'user/':'/'}) %}
- {% elseif 'gitlab' in git_sync_repo_link %}
- {% set git_repo_link_icon = "gitlab" %}
- {% set git_repo_edit_link_url = git_sync_repo_link ~ 'blob/master' ~ (page.filePathClean)|replace({'user/':'/'}) %}
- {% elseif 'bitbucket' in git_sync_repo_link %}
- {% set git_repo_link_icon = "bitbucket" %}
- {% set git_repo_edit_link_url = git_sync_repo_link ~ 'src/master' ~ (page.filePathClean)|replace({'user/':'/'}) %}
- {% else %}
- {% set git_repo_link_icon = "git" %}
- {% set git_repo_edit_link_url = git_sync_repo_link ~ 'blob/master' ~ (page.filePathClean)|replace({'user/':'/'}) %}
- {% endif %}
- {% if theme_config.github.icon %}
- {% set git_repo_link_icon = theme_config.github.icon %}
- {% endif %}
-
-
- {{ 'THEME_LEARN2_GIT_EDIT_THIS_PAGE'|t }}
- {% endif %}
- {% endif %}
-{% endif %}
diff --git a/themes/learn2-git-sync/templates/partials/github_note.html.twig b/themes/learn2-git-sync/templates/partials/github_note.html.twig
deleted file mode 100644
index a7aa262..0000000
--- a/themes/learn2-git-sync/templates/partials/github_note.html.twig
+++ /dev/null
@@ -1,7 +0,0 @@
-
-
- {{ 'THEME_LEARN2_GIT_NOTE'|t }}
-
- {% include 'partials/github_link.html.twig' %}
-
-
diff --git a/themes/learn2-git-sync/templates/partials/logo.html.twig b/themes/learn2-git-sync/templates/partials/logo.html.twig
deleted file mode 100644
index d17cce1..0000000
--- a/themes/learn2-git-sync/templates/partials/logo.html.twig
+++ /dev/null
@@ -1,5 +0,0 @@
-{% if not (theme_config.hide_site_title) %}
-
- {{config.site.title}}
-
-{% endif %}
diff --git a/themes/learn2-git-sync/templates/partials/page.html.twig b/themes/learn2-git-sync/templates/partials/page.html.twig
deleted file mode 100644
index d2614a1..0000000
--- a/themes/learn2-git-sync/templates/partials/page.html.twig
+++ /dev/null
@@ -1,6 +0,0 @@
-
-
{{ page.title }}
-
- {{ page.content|raw }}
-
-
diff --git a/themes/learn2-git-sync/templates/partials/presentation_iframe.html.twig b/themes/learn2-git-sync/templates/partials/presentation_iframe.html.twig
deleted file mode 100644
index c55c5ac..0000000
--- a/themes/learn2-git-sync/templates/partials/presentation_iframe.html.twig
+++ /dev/null
@@ -1,3 +0,0 @@
-
-
-
diff --git a/themes/learn2-git-sync/templates/partials/scripts.html.twig b/themes/learn2-git-sync/templates/partials/scripts.html.twig
deleted file mode 100644
index 3ed88cc..0000000
--- a/themes/learn2-git-sync/templates/partials/scripts.html.twig
+++ /dev/null
@@ -1 +0,0 @@
-
diff --git a/themes/learn2-git-sync/templates/partials/search.html.twig b/themes/learn2-git-sync/templates/partials/search.html.twig
deleted file mode 100644
index 830ee44..0000000
--- a/themes/learn2-git-sync/templates/partials/search.html.twig
+++ /dev/null
@@ -1,13 +0,0 @@
-{% if config.plugins.simplesearch.enabled %}
-
-
-
-
-
-{% endif %}
-{% if config.plugins.tntsearch.enabled %}
-
-{% endif %}
diff --git a/themes/learn2-git-sync/templates/partials/sidebar.html.twig b/themes/learn2-git-sync/templates/partials/sidebar.html.twig
deleted file mode 100644
index b5ced23..0000000
--- a/themes/learn2-git-sync/templates/partials/sidebar.html.twig
+++ /dev/null
@@ -1,72 +0,0 @@
-{% macro loop(page, parent_loop) %}
- {% import _self as self %}
-
- {% if parent_loop|length > 0 %}
- {% set data_level = parent_loop %}
- {% else %}
- {% set data_level = 0 %}
- {% endif %}
- {% for p in page.children.visible %}
- {% set parent_page = p.activeChild ? ' parent' : '' %}
- {% set current_page = p.active ? ' active' : '' %}
-
-
-
- {% if data_level == 0 %}{{ loop.index }}. {% endif %} {{ p.menu }}
-
- {% if p.children.count > 0 %}
-
- {{ self.loop(p, parent_loop|default(0)+loop.index) }}
-
- {% endif %}
-
- {% endfor %}
-{% endmacro %}
-
-{% macro version(p) %}
- {% set parent_page = p.activeChild ? ' parent' : '' %}
- {% set current_page = p.active ? ' active' : '' %}
-
- {% if p.activeChild or p.active %}
-
- {% else %}
-
- {% endif %}
- {{ p.menu }}
-
-{% endmacro %}
-
-{% import _self as macro %}
-
-
diff --git a/themes/learn2-git-sync/templates/partials/versions.html.twig b/themes/learn2-git-sync/templates/partials/versions.html.twig
deleted file mode 100644
index 9fda56b..0000000
--- a/themes/learn2-git-sync/templates/partials/versions.html.twig
+++ /dev/null
@@ -1,19 +0,0 @@
-
- Version:
-
- {% set langobj = grav['language'] %}
- {% for key in langswitcher.languages %}
- {% if key == langswitcher.current %}
- {% set lang_url = page.url %}
- {% set active = ' selected="selected"' %}
- {% else %}
- {% set lang_url = base_url_simple ~ langobj.getLanguageURLPrefix(key)~langswitcher.page_route ?: '/' %}
- {% set active = '' %}
- {% endif %}
- {{ (key / 10)|number_format(1) }}
- {% endfor %}
-
-
-
diff --git a/themes/learn2-git-sync/templates/search.html.twig b/themes/learn2-git-sync/templates/search.html.twig
deleted file mode 100644
index a6df13c..0000000
--- a/themes/learn2-git-sync/templates/search.html.twig
+++ /dev/null
@@ -1,11 +0,0 @@
-{% embed 'partials/base.html.twig' with { github_link_position: false } %}
-
- {% block content %}
- {{ page.content|raw }}
-
- {% include 'partials/tntsearch.html.twig' with { in_page: true, placeholder: 'THEME_LEARN2_ADVANCED_SEARCH_PLACEHOLDER'|t }%}
- {% endblock %}
-
- {% block footer %}{% endblock %}
-
-{% endembed %}
diff --git a/themes/learn2-git-sync/thumbnail.jpg b/themes/learn2-git-sync/thumbnail.jpg
deleted file mode 100644
index 6c1e46a..0000000
Binary files a/themes/learn2-git-sync/thumbnail.jpg and /dev/null differ